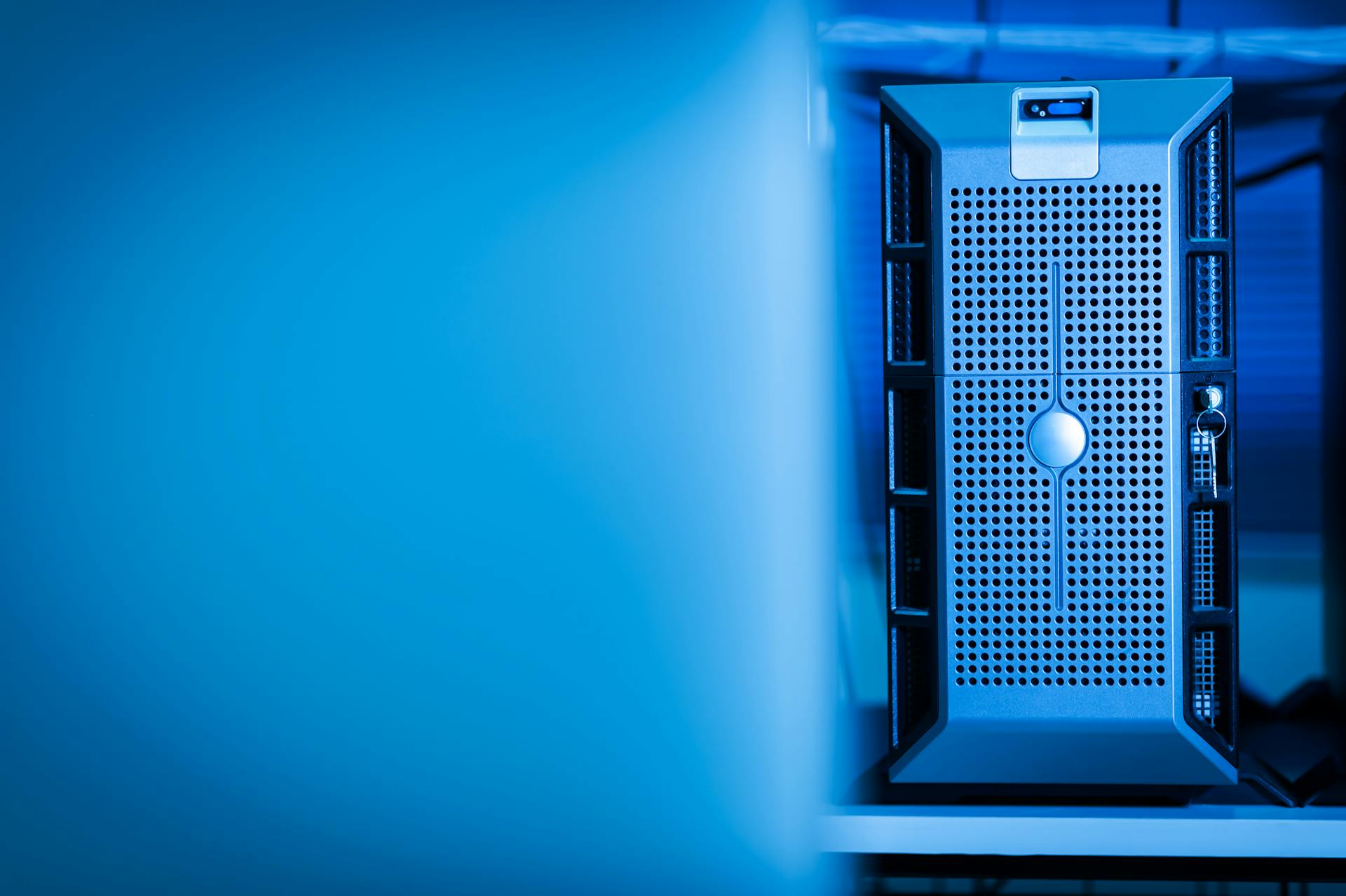
Developing Azure Functions in Python is a great way to build scalable and efficient serverless applications. You can use the Azure Functions Core Tools to create, deploy, and manage your functions.
To get started, you'll need to install the Azure Functions Core Tools by running the command `npm install -g azure-functions-core-tools@3` in your terminal. This will give you access to the `func` command, which you'll use to create and manage your functions.
One of the key benefits of using Azure Functions is its ability to automatically scale to meet changing workloads. This means you don't have to worry about provisioning or managing servers, allowing you to focus on writing code.
Expand your knowledge: Azure Functions Core Tools
Function Configuration
Azure Functions Python allows you to create serverless functions that can be triggered by various events, including HTTP requests, timer triggers, and messages from Azure Storage queues.
You can configure the function to run in a specific runtime version, such as Python 3.8 or 3.9. This can be done by specifying the runtime version in the function's configuration.
The function can also be configured to use a specific handler, which is the entry point of the function. For example, you can use the `run` function as the handler, or you can specify a custom handler using the `__init__.py` file.
Expand your knowledge: Azure Key Vault C#
Database Connection
Connecting to a database is a crucial step in setting up your Azure Functions.
You can integrate Azure Functions with Azure Cosmos DB for various use cases, including IoT, e-commerce, and gaming.
To connect to Azure Cosmos DB, you need to create an account, database, and container first.
Then, you can connect your function code to Azure Cosmos DB using triggers and bindings.
Azure Cosmos DB's change feed functionality is particularly useful for event-driven architectures, providing a persistent event store as a message broker for state-changing events.
This allows downstream microservices to reliably and incrementally read inserts and updates, such as order events.
To implement more complex app logic, you can use the Python library for Cosmos DB, which offers an asynchronous I/O implementation.
If this caught your attention, see: How to Connect to Azure Cosmos Db Using Connection String
SDK Type Bindings (Preview)
SDK Type Bindings (Preview) allow you to interact with data types implemented by the underlying Azure SDKs and frameworks.
To use SDK type bindings, you must use the Python v2 programming model. This is a requirement for working with these bindings.
See what others are reading: Azure Sdk for Python
Currently, only synchronous SDK types are supported. This means you can't use asynchronous types with SDK type bindings.
To enable SDK type bindings for the Blob storage extension, you need to add the azure-functions-extensions-bindings-blob extension package to your requirements.txt file.
Here's a list of required packages to add to your requirements.txt file:
- azure-functions
- azurefunctions-extensions-bindings-blob
Once you've added the package, you need to import the SDK type bindings in your function_app.py file. You can do this by adding the following code:
```python
import azurefunctions.extensions.bindings.blob as blob
```
Scaling and Performance
Scaling and performance are crucial for any function configuration. For best practices, see the Python scaling and performance article.
To ensure efficient scaling, consider the Python scaling and performance article.
Function apps can be scaled based on the Python scaling and performance article.
Version
When creating a function app in Azure, you can request a specific Python version using the --runtime-version option of the az functionapp create command.
The available Python versions vary depending on the Functions runtime version. For example, if you're using Functions version 4.x, you can choose from Python 3.11, 3.10, 3.9, 3.8, or 3.7.
Here's a breakdown of the supported Python versions for each Functions runtime version:
The Python version is set when the function app is created, and it can't be changed for apps running in a Consumption plan.
Configure Local Environment
To configure your local environment for Azure Functions, you'll need an Azure account with an active subscription, which is free to create.
You'll also need one of the following tools for creating Azure resources: the Azurite storage emulator or an actual Azure Storage account. The Azurite storage emulator is assumed in this example.
A supported Python version is required, which you can check by installing the Core Tools on macOS using Homebrew.
Here are the tools you'll need to configure your local environment:
- An Azure account with an active subscription
- One of the following tools for creating Azure resources: Azurite storage emulator or actual Azure Storage account
- A supported Python version
Select Authorization Level Controls
Selecting the right authorization level for your function is crucial to ensure it's secure and accessible to the right people. You can set the authorization level while configuring the function's hosting settings in the local.settings.json file during development or in the Azure Portal when deploying your function to the Azure platform.
There are three main authorization levels to choose from: Anonymous, Admin, and the default level which allows anyone to use the function without authentication or authorization.
Recommended read: Azure Function Local Settings Json
The Anonymous Authorization level makes the function user-friendly by allowing anyone to invoke it without specific authentication or authorization. This is a convenient option for public-facing functions.
The Admin Authorization level requires the caller to have an Azure AD token with an administrator role, making it a more secure implementation. Only those with admin rights or authorized users can invoke the function.
Here's a quick summary of the authorization levels:
Function Development
Function Development is a crucial aspect of Azure Functions Python. The recommended folder structure for a Python functions project is straightforward, with the main project folder containing files like local.settings.json, requirements.txt, and host.json.
You can create a local function by running the func init command, which creates a Python v2 functions project in the virtual environment. This command generates various files, including configuration files named local.settings.json and host.json.
To add a function to your project, use the func new command with the --name argument specifying the unique name of your function and the --template argument specifying the function's trigger. For example, you can add an HTTP trigger endpoint named HttpExample to the function_app.py file, which is accessible without authentication.
Related reading: Azure Auth Json Website Azure Ad Authentication
Programming Model
Azure Functions expects a function to be a stateless method in your Python script that processes input and produces output. By default, the runtime expects the method to be implemented as a global method called `main()` in the `__init__.py` file.
You can also specify an alternative entry point. To do this, you need to bind data to the function from triggers and bindings via method attributes that use the `name` property defined in the `function.json` file.
The `function.json` file describes a simple function that's triggered by an HTTP request named `req`. This is an example of how to declare the attribute types and return type in the function by using Python type annotations.
Azure Functions expects a function to be a stateless method in your Python script that processes input and produces output. The runtime expects the method to be implemented as a global method in the `function_app.py` file.
You can use Python type annotations to bind the input and outputs to your methods. These annotations help you to use the IntelliSense and autocomplete features that are provided by many Python code editors.
Here are some key differences between the two programming models:
To learn about known limitations with the v2 model and their workarounds, see Troubleshoot Python errors in Azure Functions.
Provisioning the Essentials
To provision the essentials for function development, you'll need to set up a resource group, storage account, and service plan. Azure Functions require a storage account and a service plan, so let's opt for the Linux consumption plan (Y1 dynamic) for its scalability.
The choice of os_type and sku_name will affect the app_settings below, so choose the combination that suits your needs. You can select the desired trigger type when creating a new function in the Azure Portal or Visual Studio Code with the Azure Functions extension.
Here's a breakdown of the provisioning process:
- Set up a resource group: This will be the container for all your Azure resources.
- Create a storage account: This will be used to store your function's data.
- Choose a service plan: This will determine the pricing and scalability of your function.
Here's a summary of the key settings:
Blueprints
Blueprints are a game-changer for function development. They allow you to break up your function app into modular components, making it easier to define functions in multiple Python files and divide them into different components per file.
Using blueprints provides several benefits, including extensible public function app interfaces to build and reuse your own APIs. This means you can create reusable APIs that can be easily integrated into your function app.
To create blueprints, you can define an HTTP-triggered function in a separate file, such as http_blueprint.py, and add it to a blueprint object. Then, in your function_app.py file, you can import the blueprint object and register its functions to the function app.
Durable Functions also supports blueprints, allowing you to register your orchestration, activity, and entity triggers and client bindings using the azure-functions-durableBlueprint class. This can be done in a similar way to registering functions in a regular blueprint.
Here are the benefits of using blueprints:
- Lets you break up the function app into modular components
- Provides extensible public function app interfaces to build and reuse your own APIs
By using blueprints, you can make your function app more maintainable, scalable, and reusable. This is especially useful when working on large-scale projects with multiple developers.
Triggers and Inputs
In Azure Functions, inputs are divided into two categories: trigger input and other input. Although they're defined using different decorators, their usage is similar in Python code.
Connection strings or secrets for trigger and input sources map to values in the local.settings.json file when they're running locally, and they map to the application settings when they're running in Azure.
You can define a Blob Storage input binding using the AzureBlobTrigger decorator, which is used to retrieve an entry from Azure Blob Storage based on the ID in the route URL.
When the function is invoked, the HTTP request is passed to the function as req, and an entry is retrieved from the Azure Blob Storage account based on the ID in the route URL and made available as obj in the function body.
Here are the different types of triggers you can use in Azure Functions:
- Blob trigger: Triggers the function when an event results in the manipulation of Azure Blob Storage.
- CosmosDB trigger: Triggers the function when any modifications are made to your collection in Cosmos DB.
- EventHub trigger: Triggers the function when events come from an Azure Event Hub.
- Queue trigger: Triggers the function when a message is received from an Azure Storage Queue.
- Timer trigger: Triggers the function at specific time-based events.
You can use the AzureFunctionsHTTPTrigger decorator to define an HTTP trigger, which is used to handle HTTP requests and return HTTP responses.
To create a function project and add an HTTP triggered function, you can use the func init and func new commands, which will create a Python v2 functions project and add an HTTP trigger endpoint to the function_app.py file.
A fresh viewpoint: Create Sample Azure Function for .net Frame Work
Http Streams (Preview)
Http Streams (Preview) is a feature that lets you accept and return data from your HTTP endpoints using FastAPI request and response APIs. This feature makes it possible to handle large data streams.
Additional reading: Http Trigger Azure Function
To use Http Streams, you need to use the Python v2 programming model. This is currently in preview and requires you to use the Python v2 programming model.
You'll need to meet the following requirements:
- Azure Functions runtime version 4.34.1, or a later version.
- Python version 3.8, or a later supported version.
Http Streams are disabled by default, so you need to enable this feature in your application settings and update your code to use the FastAPI package.
To enable Http Streams, you'll need to add the azurefunctions-extensions-http-fastapi extension package to your requirements.txt file. You'll also need to add some code to your function_app.py file to import the FastAPI extension.
Here's an interesting read: Fastapi Azure Function
Logging from Created Threads
Logging from created threads is a crucial aspect of function development. You need to ensure that logs from these threads are visible to you, and that's where the context argument comes in.
To see logs coming from your created threads, you need to include the context argument in the function's signature. This argument contains an attribute thread_local_storage that stores a local invocation_id.
This invocation_id can be set to the function's current invocation_id to ensure the context is changed. This is a clever trick to get the logs from your created threads.
By setting the invocation_id, you can ensure that the logs from your created threads are tied to the correct function invocation. This makes it much easier to debug and troubleshoot your code.
Here's a quick summary of the steps to follow:
By following these simple steps, you can ensure that logs from your created threads are visible and easily identifiable. This is a great way to improve the debugging and troubleshooting process for your functions.
Package Management
Package management is crucial for function development. You need to manage your dependencies correctly to avoid conflicts.
When developing locally, add the required packages to the requirements.txt file. This file should include the names and versions of the packages you need. For example, if you want to install the requests package from PyPI, you can add it to the requirements.txt file.
You can install packages by using pip, just like this: pip install -r requirements.txt. This command will install all the packages listed in the requirements.txt file.
To prevent issues running in an App Service plan, don't name your directories the same as any Python native modules. For instance, avoid naming a directory "logging" if you're using the built-in logging module.
In an App Service plan, dependencies defined in requirements.txt are given precedence over built-in Python modules. This can cause conflicts when built-in modules have the same names as directories in your code.
Here's a quick reference to the packages you should avoid including in your requirements.txt file:
Adding Your Code
You can add your function code to a new Python script in the function folder, giving it a name like myfunction.py.
The recommended folder structure for a Python functions project includes a folder for each function, with a code file and a binding configuration file, function.json.
Readers also liked: Azure Code
To start, create a new Python script in the function folder and add the code you want, such as a Flask-based HTTP trigger.
When you deploy your project to a function app in Azure, the entire contents of the main project folder should be included in the package, but not the folder itself.
You can use a Python worker extension library in your function by adding the extension package in the requirements.txt file, installing the library into your app, and adding the necessary application settings.
A function project is a container for one or more individual functions that each responds to a specific trigger, and all functions in a project share the same local and hosting configurations.
Here are the basic steps to create a local function:
1. Run the func init command to create a Python v2 functions project in the virtual environment.
2. Add a function to your project by using the func new command, specifying the unique name of your function and the trigger type.
You can also create a new function by running the func new command and following the prompts to choose the trigger type and authentication level.
Take a look at this: .net 8 Azure Functions
Here's a summary of the basic steps to create a function:
- Create a new Python script in the function folder
- Add the code you want, such as a Flask-based HTTP trigger
- Run the func start command to run the function locally
- Deploy the function to Azure using the Deploy to Function App... option.
Frequently Asked Questions
What version of Python does Azure Functions support?
Azure Functions supports Python 3.7 to 3.11 for serverless hosting on Linux. Learn more about the supported Python versions and runtime options.
What versions of Python does Azure Functions support?
Azure Functions supports Python versions 3.7 through 3.11 for serverless hosting on Linux. Learn more about the supported runtimes and hosting options.
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-reference-python
- https://learn.microsoft.com/en-us/azure/azure-functions/create-first-function-cli-python
- https://medium.com/@saurabh.dasgupta1/developing-and-deploying-a-python-azure-function-step-by-step-83c5066a2531
- https://www.geeksforgeeks.org/how-to-create-azure-function-in-visual-studio-code-using-python/
- https://www.linkedin.com/pulse/azure-function-python-deployment-terraform-huaifeng-qin-jfjtc
Featured Images: pexels.com