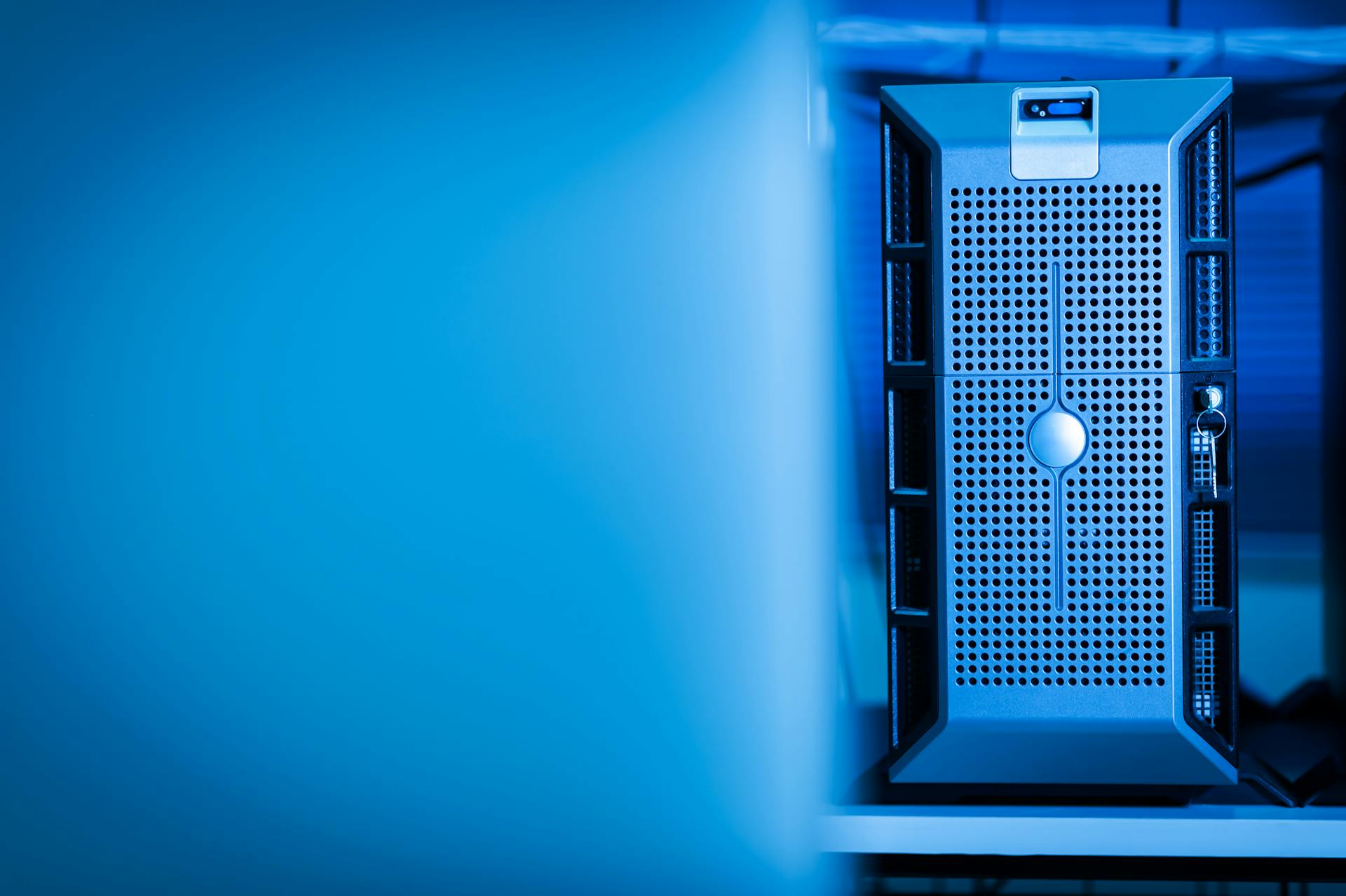
If you're new to Azure, you might be wondering what Azure REST API is all about. Simply put, it's a way to interact with Azure services using HTTP requests.
Azure REST API provides a standardized interface to access Azure resources, allowing you to automate tasks and integrate Azure services with your applications.
To get started with Azure REST API, you'll need to create an Azure subscription and obtain an API key, which is used for authentication and authorization.
The API key is used to sign your HTTP requests, ensuring that only authorized requests are processed by Azure services.
Authentication
Authentication is a crucial step in accessing Azure REST APIs. You have several options to authenticate your application, including using personal access tokens, Microsoft Authentication Library (MSAL), or OAuth.
The type of authentication you choose depends on the type of application you're building. For instance, if you're creating a client-side application that allows user interaction, you can use MSAL. If you're building a GUI-based web application, OAuth is a good choice.
Curious to learn more? Check out: Building Web Apis with Asp.net Core
Here's a breakdown of the authentication methods for different types of applications:
In addition to these methods, you can also use key-based authentication or role-based access control through Microsoft Entra ID. Key-based authentication relies on API keys, while role-based access control requires an established tenant in Microsoft Entra ID with security principals and role assignments.
To obtain an access token, you can use the OAuth2 /authorize and /token endpoints. You can also use the Microsoft Authentication Libraries (MSAL) for platform- and language-specific authentication.
Related reading: Microsoft Azure Dev
API Basics
To call Azure REST APIs, you must issue requests over HTTPS on the default port 443.
Requests must be issued over HTTPS (on the default port 443) to ensure secure communication. This is a standard practice for online APIs.
The Request URI must include the api-version, formatted as shown in this example: GET https://[search service name].search.windows.net/indexes?api-version=2024-07-01.
API requests must include either an api-key or a bearer token for authenticated connections. This ensures that your requests are authorized and secure.
You can optionally set the Accept HTTP header to specify the expected response format. If not set, the default is assumed to be application/json.
Here are the key points to remember:
- HTTPS on port 443
- api-version in Request URI
- api-key or bearer token for authentication
- Optional Accept HTTP header
Uri
A URI is the foundation of a REST request, and it's essential to get it right. The scheme of a URI must be HTTPS to ensure a secure channel for transmitting sensitive information.
The host, resource path, and any required query-string parameters make up the remainder of your service's request URI. For example, Azure Resource Manager provider APIs use https://management.azure.com/, and Azure classic deployment model uses https://management.core.windows.net/.
The request URI is determined by its related REST API specification. This means you need to check the API specification to know the correct URI for your service.
You'll often see query-string parameters in a URI, like the api-version parameter. This parameter is required and specifies the version of the API to use. For instance, to use the Azure DevOps API version 7.1, you'd set the api-version parameter to '7.1'.
Here's a breakdown of the URI parameters you might encounter:
The request URI is bundled in the request message header, along with other required fields. This includes the Authorization header, which contains the OAuth2 bearer token, and the Content-Type header, which specifies the MIME type of the request body.
Client Libraries
You can access the client libraries for these REST APIs, which will help you integrate them into your applications.
The .NET conceptual documentation and .NET reference documentation are available for developers who want to work with the APIs using .NET.
Go, Node.js, and Python are also supported, with client libraries available for each of these programming languages.
If you want to use the APIs in a web browser, you can use the Swagger 2.0 client library.
The Web Extensions SDK is another option for developers who want to integrate the APIs into their web applications.
Here are the client libraries mentioned, listed in a table for easy reference:
Calling APIs with Curl
Calling APIs with Curl is a straightforward process that can be used in various scenarios, including DevOps automation.
Curl is a command-line tool that can be used to call Azure REST APIs, making it a great option for unattended scripts.
You might consider using curl in DevOps automation scenarios, as mentioned in the blog post "Calling Azure REST API via curl".
Curl can be used to call Azure REST APIs in a variety of ways, but it's most commonly used for unattended scripts.
The process of calling an Azure REST API using curl is described in the blog post "How to call Azure REST APIs with curl".
Curious to learn more? Check out: Nextjs Api Call Another Api
API Response
An API response is a crucial part of the Azure REST API process, and understanding it can help you troubleshoot and improve your applications.
The response message is made up of five components: the response URI, HTTP response message header fields, optional HTTP response message body fields, HTTP response message header fields, and optional HTTP response message body fields.
To process the response message, you need to parse the response header and, optionally, the response body. Most programming languages and frameworks return this information to your application in a typed/structured format.
You're interested in confirming the HTTP status code in the response header, which is similar to the example provided in the HTTPS GET example. The response body contains the actual data, encoded in a format such as JSON, like the list of Azure subscriptions and their properties.
For successful operations, you should receive a response header confirming the operation, like the example for the HTTPS PUT operation. The response body contains the actual data, confirming the content of the newly added resource group.
If this caught your attention, see: Nextjs Response Json
Here are the key components of an API response:
API Configuration
To register a client that accesses an Azure Resource Manager REST API, you'll need to follow a few steps.
To register a client application with Microsoft Entra ID, you'll need to create a Microsoft Entra application and service principal that can access resources. This can be done using the portal, PowerShell, or CLI versions for automating registration.
To configure Azure Resource Manager Role-Based Access Control (RBAC) settings for authorizing the client, you'll need to set permission requests to allow the client to access the Azure Resource Manager API.
Here are the key steps to configure API access:
- Register an application with the Microsoft identity platform
- Configure an application to expose a web API
- Configure a client application to access a web API
API Configuration
To register your client application with Microsoft Entra ID, you'll need to follow a specific process. This involves creating a Microsoft Entra application and service principal that can access resources.
Most Azure services require your client code to authenticate with valid credentials before calling the service's API. This is where Microsoft Entra ID comes in, providing an access token as proof of authentication.
A different take: Web Application Programming Interface
To register a client that accesses an Azure Resource Manager REST API, you'll need to register the client application with Microsoft Entra ID. This involves setting permission requests to allow the client to access the Azure Resource Manager API and configuring Azure Resource Manager Role-Based Access Control (RBAC) settings for authorizing the client.
Here are the steps to register a client application:
- Register the client application with Microsoft Entra ID.
- Set permission requests to allow the client to access the Azure Resource Manager API.
- Configure Azure Resource Manager Role-Based Access Control (RBAC) settings for authorizing the client.
API and Version Mapping
API configuration can be complex, but understanding how different APIs and versions interact is crucial for success.
The Azure DevOps Server REST API has undergone several changes over the years, with each version introducing new features and capabilities.
Here's a quick mapping of REST API versions and their corresponding TFS releases. All API versions will work on the server version mentioned as well as later versions.
Recent changes to the engineering system and documentation generation process aim to provide clearer, more in-depth, and more accurate documentation for users of these REST APIs.
Frequently Asked Questions
How do I host a REST API on Azure?
To host a REST API on Azure, start by cloning a sample application and following the steps to deploy it to Azure, including creating a resource group, App Service plan, and web app. This process allows you to easily set up and manage your REST API on Azure's scalable and secure platform.
What is REST API?
REST API is a standard way for web applications to communicate with each other, using HTTP requests to manage data. It's a simple yet powerful tool for building scalable and efficient web services.
What is Azure rest?
Azure REST refers to the RESTful APIs provided by Azure, which enable developers to interact with Azure services using standard HTTP methods such as GET, POST, PUT, and DELETE. By using Azure REST, developers can easily access and manage Azure resources in a flexible and scalable way.
How to call rest API in Azure?
To call a REST API in Azure, obtain an access token and include it in the "Authorization" header as "Bearer {access-token}". This allows you to authenticate and make API calls to Azure services.
Sources
- https://learn.microsoft.com/en-us/rest/api/azure/
- https://learn.microsoft.com/en-us/rest/api/searchservice/
- https://learn.microsoft.com/en-us/rest/api/azure/devops/
- https://learn.microsoft.com/en-us/rest/api/azure/devops/core/projects/create
- https://learn.microsoft.com/en-us/azure/azure-resource-manager/management/manage-resources-rest
Featured Images: pexels.com