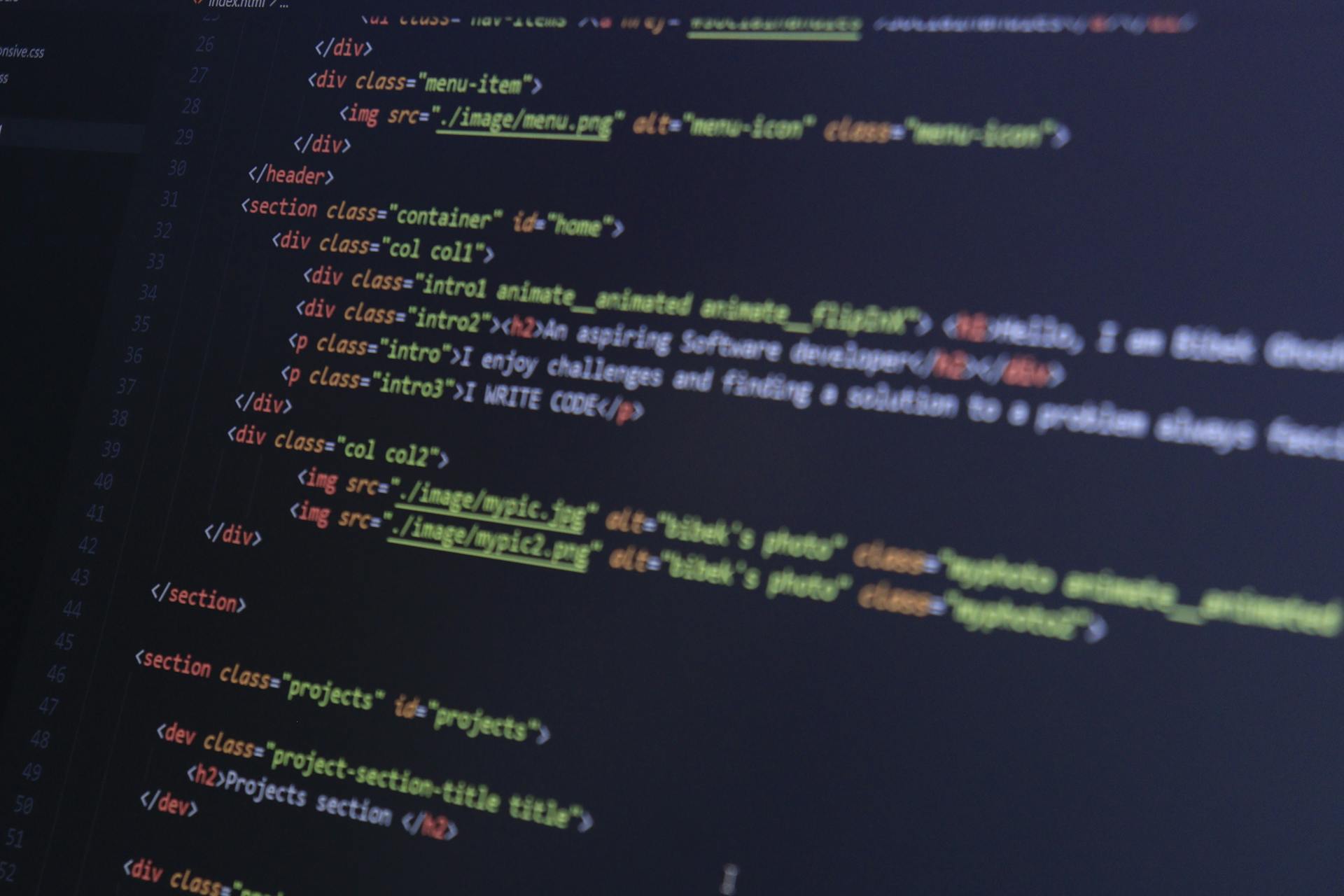
Building a Web API from scratch requires a solid foundation in ASP.NET Core, which is a cross-platform framework for building web applications.
To start, you'll need to create a new project in Visual Studio, selecting the ASP.NET Core Web Application template.
Next, you'll need to choose a project template, such as the Web API template, which will create a basic API project structure for you.
The API project will have a Controllers folder where you can create API controllers, such as the WeatherForecastController, which returns a list of weather forecasts.
API controllers are the core of your API, and they're where you'll define the actions that will be exposed to clients.
A fresh viewpoint: Azure Web App Asp.net V4.8
Getting Started
To get started with building web APIs with ASP.NET Core, you'll need to set up your development environment correctly. You can install Visual Studio 2022 with the ASP.NET and web development workload, which is a prerequisite for ASP.NET Core development.
You'll also need to install Visual Studio Code, C# for Visual Studio Code, and the .NET 8.0 SDK. The .NET CLI is used for ASP.NET Core development functions, and it's compatible with macOS, Linux, and Windows, as well as any code editor.
For more insights, see: Web Mobile Development
Here are the specific tools you'll need to install:
- Visual Studio 2022 with the ASP.NET and web development workload
- Visual Studio Code
- C# for Visual Studio Code (latest version)
- .NET 8.0 SDK
By default, the architecture of the .NET binaries to install will represent the currently running OS architecture, so you don't need to specify a different architecture unless you want to.
Prerequisites
To get started with ASP.NET Core development, you'll need to have the right tools installed on your computer.
First, you'll need to install Visual Studio 2022 with the ASP.NET and web development workload. This will give you the necessary tools to create and manage ASP.NET Core projects.
You can also use Visual Studio Code, which is a free, open-source code editor that's compatible with ASP.NET Core development. To use Visual Studio Code, you'll need to install C# for Visual Studio Code (the latest version) and the .NET 8.0 SDK.
The .NET 8.0 SDK is required for ASP.NET Core development functions, such as project creation, and can be used on macOS, Linux, or Windows with any code editor. However, you may need to make minor changes if you're using a code editor other than Visual Studio Code.
A unique perspective: Web Dev Tools
The Id property functions as the unique key in a relational database.
Here's a list of the prerequisites you'll need to get started with ASP.NET Core development:
- Visual Studio 2022 with the ASP.NET and web development workload.
- Visual Studio Code
- C# for Visual Studio Code (latest version)
- .NET 8.0 SDK
Note that the architecture of the .NET binaries to install will default to the currently running OS architecture. If you want to specify a different OS architecture, you can use the dotnet tool install, --arch option.
Introduction to
APIs are the foundation for modern web applications in today's age.
APIs promote accessible communication with other apps, enabling data exchange and functionality integration.
Web APIs facilitate communication over the Internet, enabling web applications to interact with external services and resources.
Discover more: Building Python Web Apis with Fastapi
Controllers and Routing
Controllers are the heart of ASP.NET Core Web API, allowing you to define API endpoints and handle HTTP requests. A controller class inherits from ControllerBase, and is decorated with the [ApiController] attribute.
To create a controller, you can right-click on the Controller folder and select Add -> Controller, then choose the API Controller - Empty template. This will give you a basic controller class with a constructor that you can use to inject dependencies.
The [Route] attribute is used to define the URL path for a controller, and can be combined with the [HttpGet] attribute to specify the HTTP method and route template. For example, [Route("api/[controller]")] indicates that the controller responds to requests at the "api/[controller]" URL path, where [controller] is the name of the controller class minus the "Controller" suffix.
Here are some key facts to keep in mind when working with controllers and routing:
- The [HttpGet] attribute denotes a method that responds to an HTTP GET request.
- The URL path for each method is constructed by replacing [controller] with the name of the controller, and appending any route template specified in the [HttpGet] attribute.
- The [Route] attribute is used to define the URL path for a controller, and can be combined with the [HttpGet] attribute to specify the HTTP method and route template.
Scaffold a Controller
Scaffolding a controller is an efficient way to create a new controller in your ASP.NET Core project. You can right-click the Controllers folder and select Add > New Scaffolded Item. From there, you can choose API Controller with actions, using Entity Framework, and then select Add.
In the Add API Controller with actions, using Entity Framework dialog, if the scaffolding operation fails, you can select Add to try scaffolding a second time. This can be useful if you encounter any issues during the process.
Discover more: Django Rest Framework Deserialize
To scaffold a controller, you'll also need to add NuGet packages required for scaffolding. This includes the scaffolding engine, which is installed using the dotnet-aspnet-codegenerator command.
Here's a step-by-step process to scaffold a controller:
1. Right-click the Controllers folder and select Add > New Scaffolded Item.
2. Choose API Controller with actions, using Entity Framework, and then select Add.
3. In the Add API Controller with actions, using Entity Framework dialog, select Add to try scaffolding a second time if the operation fails.
4. Add NuGet packages required for scaffolding.
5. Install the scaffolding engine using the dotnet-aspnet-codegenerator command.
The generated code will include the [ApiController] attribute, which indicates that the controller responds to web API requests. The controller will also use Dependency Injection (DI) to inject the database context into the controller.
Asynchronous Programming
Asynchronous programming is a crucial technique for improving the performance and scalability of your web APIs with ASP.NET Core.
By allowing the server to handle more requests simultaneously, asynchronous programming enables your web APIs to efficiently handle high loads and provide a responsive user experience.
Implementing asynchronous methods is a key strategy for achieving this goal, and it can significantly enhance the performance and scalability of your web APIs.
Asynchronous programming improves scalability by allowing the server to handle more requests simultaneously.
Consider reading: Hacking Apis Breaking Web Application Programming Interfaces
Key Features
ASP.NET Core Web API is an incredibly powerful framework for building robust and scalable applications. Its cross-platform capabilities allow it to run on Windows, Linux, and macOS, providing flexibility in deployment.
This cross-platform functionality is a game-changer for developers, as it enables them to deploy their applications on a wide range of operating systems.
One of the standout features of ASP.NET Core Web API is its high-performance architecture, which offers excellent speed and efficiency. This is especially important for applications that require fast data processing and response times.
The framework's modularity is another key benefit, allowing developers to include only the necessary components and resulting in lightweight and efficient applications.
Here are the key features of ASP.NET Core Web API:
- Cross-platform: ASP.NET Core is designed to run on Windows, Linux, and macOS.
- Performance: With a revamped, high-performance architecture, ASP.NET Core Web API offers excellent speed and efficiency.
- Modularity: The framework is modular, allowing developers to include only the necessary components.
This modularity is a major advantage, as it enables developers to build applications that are tailored to their specific needs and requirements.
API Endpoints
API Endpoints are the heart of a web API, and in ASP.NET Core, we use attributes like [HttpPost] to define the type of request an endpoint accepts.
When creating a new resource, the PostTodoItem create method returns an HTTP 201 status code if successful, which is the standard response for an HTTP POST method.
This status code is accompanied by a Location header that specifies the URI of the newly created to-do item, which is generated by referencing the GetTodoItem action using the C# nameof keyword.
Here are the key characteristics of the PostTodoItem create method:
- Returns an HTTP 201 status code if successful.
- Adds a Location header to the response.
- References the GetTodoItem action to create the Location header's URI.
The PutTodoItem
The PutTodoItem method is a crucial part of any API, and it's similar to the PostTodoItem method, but with a key difference: it uses HTTP PUT instead. The response is 204 (No Content), which is a standard response for a successful PUT request.
According to the HTTP specification, a PUT request requires the client to send the entire updated entity, not just the changes. This is in contrast to the PostTodoItem method, which returns an HTTP 201 status code if successful.
Suggestion: Nextjs Response Json
To support partial updates, you should use HTTP PATCH, not PUT. This is an important consideration when designing your API endpoints.
Here are some key differences between the PutTodoItem and PostTodoItem methods:
By understanding the differences between these two methods, you can design more effective API endpoints that meet the needs of your clients.
Implementing Versioning
API versioning is crucial for maintaining backward compatibility, allowing you to evolve your API without breaking existing clients.
To ensure backward compatibility, you can introduce new features and improvements while keeping older versions functional.
API versioning enables you to evolve your API, but it requires careful planning and implementation.
Here are the versioned API endpoints for testing:
- For version 1.0: https://localhost:5001/api/v1/products
- For version 2.0: https://localhost:5001/api/v2/products
By following these steps, you can effectively set up and manage API versioning in your API, ensuring it remains backward compatible while allowing for new enhancements and features.
Security and Authorization
Securing your Web API is essential to protect against unauthorized access and ensure data integrity. You can do this by implementing authentication and authorization.
To start, you can add encryption attributes to model properties, which can help protect sensitive data. This is a key strategy for enhancing the security of your ASP.NET Core Web API.
Implementing JWT authentication, configuring middleware, and protecting API endpoints are also crucial steps in securing your API. You can use authorization policies to control access to specific resources within your API, either based on roles or claims.
Prevent Over-Posting
Preventing over-posting is a crucial aspect of security in our apps. This is achieved by limiting the data that's input and returned using a subset of the model, known as a Data Transfer Object (DTO).
A DTO is used to prevent over-posting, hide properties that clients are not supposed to view, omit some properties to reduce payload size, and flatten object graphs that contain nested objects.
To demonstrate the DTO approach, let's consider the TodoItem class, which includes a secret field that needs to be hidden from this app. However, an administrative app could choose to expose it.
You can post and get the secret field, but you can't post or get it if it's not exposed. This is a key benefit of using a DTO.
Here are some reasons why DTOs are useful:
- Prevent over-posting.
- Hide properties that clients are not supposed to view.
- Omit some properties in order to reduce payload size.
- Flatten object graphs that contain nested objects.
Endpoint Authorization
Endpoint authorization is crucial for protecting your API endpoints from unauthorized access. This can be achieved by adding authorization attributes to your API endpoints.
To do this, you can configure your API to use JWT authentication and configure middleware to protect your endpoints. For example, in ASP.NET Core 8, you can use JWT authentication and protect your API endpoints in the ProductController.
Authorization policies can be implemented to control access to specific resources within your API. This can be done using role-based or claim-based authorization.
Here are some key strategies for enhancing the security of your ASP.NET Core Web API:
- Add encryption attribute to model properties to protect sensitive data.
- Implement authentication methods such as JWT, OAuth, and API keys.
- Configure authentication middleware to secure your API endpoints.
- Use authorization policies to control access to specific resources.
Securing your API endpoints is essential to protect against unauthorized access and ensure data integrity. By implementing these security measures, you can safeguard your API against common threats and provide a robust and secure development environment.
Importance of Versioning in Backward Compatibility
API versioning is crucial for maintaining backward compatibility, allowing you to evolve your API without breaking existing clients.
By introducing versioning, you can ensure that older versions of your API remain functional even as you introduce new features and improvements.
This is especially important in ASP.NET Core 8, where you can set up and manage API versioning effectively.
To achieve backward compatibility, you can test your versioned API endpoints, such as For version 1.0: https://localhost:5001/api/v1/productsFor version 2.0: https://localhost:5001/api/v2/products
By doing so, you can ensure that your API remains compatible with existing clients while allowing for new enhancements and features.
Consider reading: Azure Api Version
Performance and Scalability
When building web APIs with ASP.NET Core, performance and scalability are crucial to ensure a smooth user experience. Caching is a key strategy to improve performance by reducing the load on the database. Caching helps store frequently accessed data in memory.
Implementing caching can be as simple as adding a memory cache service to your application. This allows you to store data in memory, reducing the need for database queries. By implementing caching, you can see significant improvements in performance.
To further optimize performance, consider using pagination for large data sets. Pagination reduces the amount of data returned in a single request, improving performance and user experience.
For another approach, see: Django Rest Framework Pagination
Improving Performance with Caching
Implementing caching strategies can significantly improve performance by reducing the load on the database.
Caching helps reduce the load on the database by storing frequently accessed data in memory.
One effective approach is to use in-memory caching, which can be achieved by adding a memory cache service to your application.
This can be done by implementing caching in your controller to store frequently accessed data in memory.
By using caching, you can improve performance and provide a better user experience, especially when dealing with large data sets.
Pagination is another technique that can help reduce the amount of data returned in a single request, improving performance and user experience.
However, caching can be more effective in certain scenarios, such as when dealing with frequently accessed data.
Optimizing database queries and data access is also crucial for efficient data retrieval and minimizing database load.
But even with optimized queries, caching can still provide significant performance improvements.
Caching can be used in conjunction with pagination to further improve performance and user experience.
Monitoring and Analytics
Monitoring and analytics are crucial for maintaining a healthy and performant API. This involves logging, monitoring API performance, and collecting analytics data to gain insights into usage patterns and potential issues.
Logging is essential for tracking API requests and errors, allowing you to identify and fix problems quickly. The ASP.NET framework recommends using querystring and repeaters for secure logging.
Monitoring API performance is vital for detecting bottlenecks and optimizing your API's response time. You should use tools like querystring asp net to monitor API performance.
Collecting analytics data helps you understand how users interact with your API, enabling you to make data-driven decisions. This includes analyzing usage patterns, identifying trends, and detecting potential security threats.
Securing communication and preventing security threats is critical for maintaining a performant API. Ensure that your implementation aligns with the security practices recommended for the ASP.NET framework, such as using querystring asp net and repeaters asp net.
Worth a look: Web Usage Statistics
Advanced Topics
As you delve into the advanced topics of ASP.NET Core Web API, you'll discover that customizing the middleware pipeline can greatly enhance your API's functionality. Middleware customization allows you to add additional functionality to your API, such as logging, authentication, and request/response manipulation.
Custom middleware components can be created to handle specific tasks, like logging API requests and responses.
The middleware execution order is crucial to consider when customizing the pipeline, as it affects how your API processes incoming requests.
By customizing the middleware pipeline, you can improve your API's performance and scalability.
To ensure seamless communication between your API and clients with different requirements, you need to configure and customize content negotiation. Content negotiation enables clients to request data in different formats, such as JSON or XML.
Configuring content negotiation allows you to specify the formats that your API supports, and clients can then request data in those formats.
Hypermedia as the Engine of Application State (HATEOAS) can also enhance your API's discoverability and navigation. By incorporating hypermedia links into API responses, you provide clients with information on available actions.
HATEOAS makes it easier for clients to navigate your API and discover available resources and actions.
By addressing these advanced topics, you can improve your API's functionality, customization, and discoverability, ultimately leading to better performance and scalability.
Sources
- https://learn.microsoft.com/en-us/aspnet/core/tutorials/first-web-api
- https://medium.com/net-core/build-a-restful-web-api-with-asp-net-core-6-30747197e229
- https://wirefuture.com/post/building-web-apis-with-asp-net-core-8-a-step-by-step-guide
- https://www.clariontech.com/blog/building-asp.net-web-api-with-.net-core
- https://www.saffrontech.net/blog/asp-net-core-web-api
Featured Images: pexels.com