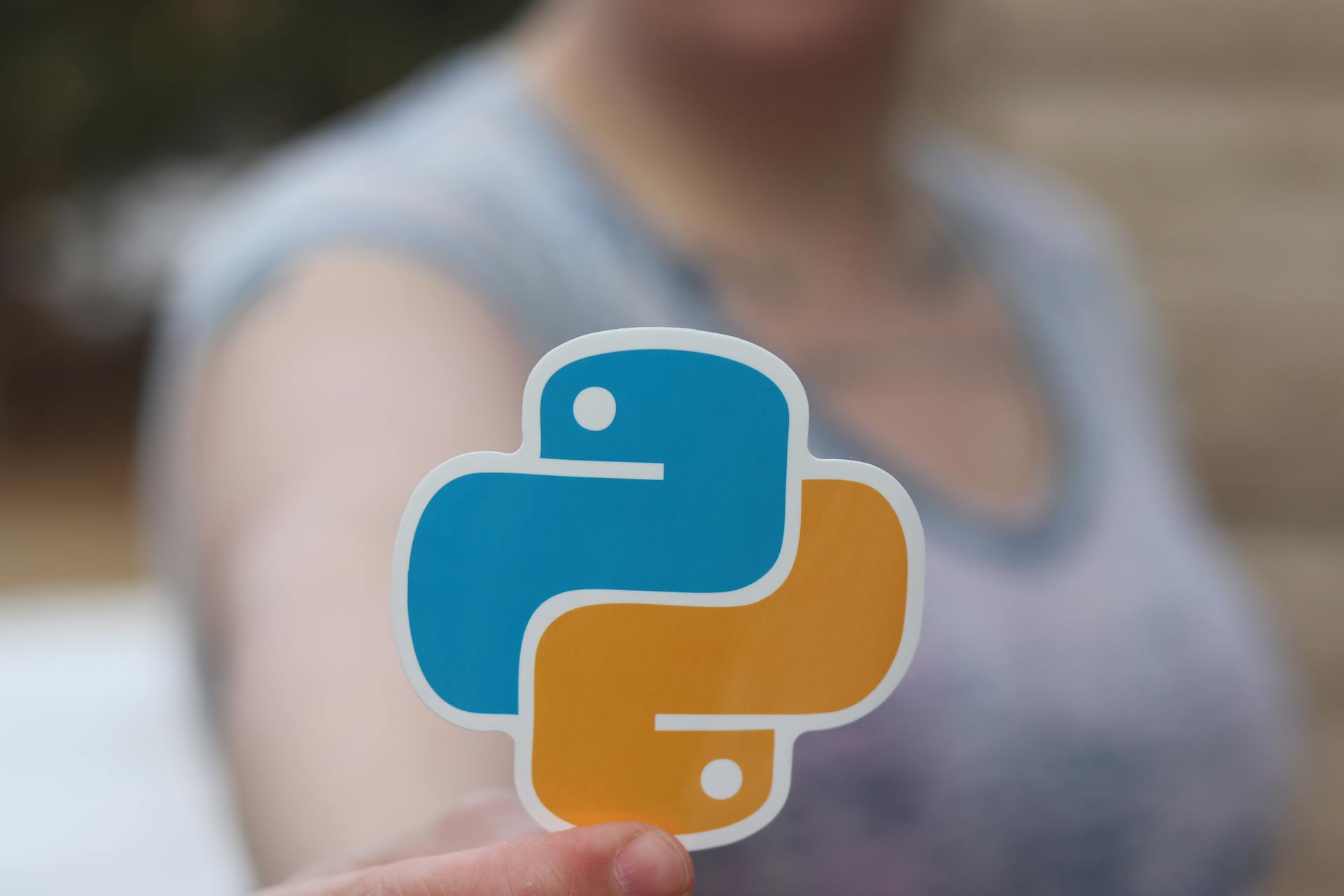
Django Rest Framework Deserialize is a powerful tool for converting JSON data into Python objects.
The DRF serializer's `parse()` method is where the deserialization magic happens, and it's responsible for converting the JSON data into Python objects.
The `parse()` method takes a JSON string as input and returns a Python object, which can be a dictionary, list, or even a custom object.
This process is made possible by the `parser` attribute of the serializer, which is an instance of `rest_framework.parsers.JSONParser`.
Check this out: Azure Data Factory Framework
Deserialization
Deserialization is a crucial step in Django REST Framework (DRF) that allows you to convert data received through API requests back into Python objects.
Deserialization is very similar to serialization, and the deserialize function takes the same format argument as serialize, a string or stream of data, and returns an iterator.
The objects returned by the deserialize iterator are special DeserializedObject instances that wrap a created – but unsaved – object and any associated relationship data. Calling DeserializedObject.save() saves the object to the database.
On a similar theme: Azure Data Factory Rest Api
If the pk attribute in the serialized data doesn’t exist or is null, a new instance will be saved to the database. This ensures that deserializing is a non-destructive operation even if the data in your serialized representation doesn’t match what’s currently in the database.
To make sure that deserialized objects are “appropriate” for saving, you can examine the deserialized objects before calling save().
DeserializedObject instances can be inspected as deserialized_object.object, and you can save the object directly if you trust your data source.
However, if fields in the serialized data do not exist on a model, a DeserializationError will be raised unless the ignorenonexistent argument is passed in as True.
Here are some key differences between deserialization and serialization:
Deserialization of natural keys is also possible, which allows you to use a set of fields to uniquely identify an object, rather than its primary key. This can be achieved by defining a default Manager for a model with a get_by_natural_key() method.
For example, if you have a model called Person with a natural key consisting of the first and last name, you can use the get_by_natural_key() method to resolve a serialized data representation of a Person object into its primary key.
However, if you have a model with no primary key, you can use the get_by_natural_key() method to populate the deserialized object’s primary key.
Deserialization of objects with no primary key will always check whether the model’s manager has a get_by_natural_key() method and if so, use it to populate the deserialized object’s primary key.
When dealing with natural foreign keys, you may encounter forward references, where an object has a foreign key referencing another object that hasn’t yet been deserialized. To handle this situation, you need to pass handle_forward_references=True to serializers.deserialize(). This will set the deferred_fields attribute on the DeserializedObject instances, which you can later call save_deferred_fields() on.
Serialization
Serialization is a crucial part of Django REST framework's deserialization process. Serializers convert complex data types into Python data types that can be easily rendered into JSON, XML, or other content types.
Serializers are used to convert Django model instances into Python data types. They provide deserialization, allowing parsed data to be converted back into complex types after first validating the incoming data.
Serializer fields handle converting between primitive values and internal datatypes. They also deal with validating input values, as well as retrieving and setting the values from their parent objects.
Serializer fields have several arguments that can be used to manipulate their behavior. These include read_only, write_only, required, default, allow_null, source, validators, error_messages, label, help_text, and initial.
Here are the main types of serializer fields:
- BooleanField: A boolean field used to wrap True or False values.
- CharField: CharField is used to store text representation.
- EmailField: EmailField is also a text representation and it validates the text to be a valid e-mail address.
- RegexField: As the name defines, RegexField matches the string to a particular regex, else raises an error.
- UUIDField: A field that ensures the input is a valid UUID string.
- JSONField: A field class that validates that the incoming data structure consists of valid JSON primitives.
Serializer fields also have several core arguments that can be used to manipulate their behavior. These include read_only, write_only, required, default, allow_null, source, validators, error_messages, label, help_text, and initial.
Some common serializer fields include:
Serializer fields can also be used to validate input values. For example, the EmailField field validates the text to be a valid e-mail address.
Serializer fields can be used to customize how data is retrieved for serialization and how data is assigned during deserialization. This can be done using the source argument, which allows you to access nested data and map a serializer field to a different attribute or method on the model instance.
Serializers
Serializers are the backbone of Django REST framework's serialization process. They convert complex data types, such as Django model instances, into Python data types that can be easily rendered into JSON, XML, or other content types.
A serializer can be used to convert data in both directions: from the complex data type to the Python data type, and vice versa. This process is known as deserialization.
Serializers in Django REST framework are a part of the Django REST framework, a powerful and flexible toolkit for building Web APIs. They are used to provide deserialization, allowing parsed data to be converted back into complex types after first validating the incoming data.
Serializer fields handle converting between primitive values and internal datatypes. They also deal with validating input values, as well as retrieving and setting the values from their parent objects.
Some common serializer fields include:
- BooleanField: A boolean field used to wrap True or False values.
- CharField: CharField is used to store text representation.
- IntegerField: IntegerField is basically a integer field that validates the input against Python’s int instance.
Serializer fields can be customized with various arguments, such as:
- `source`: The name of the attribute that will be used to populate the field.
- `validators`: A list of validator functions which should be applied to the incoming field input.
- `error_messages`: A dictionary of error codes to error messages.
Serializers also support various serialization formats, including JSON, XML, and YAML. The format used depends on the requirements of the project.
In addition to serializer fields, Django REST framework also provides a way to define custom validation logic for serializers. This can be done using field-level validations or object-level validations.
Serialization Basics
Serialization Basics is where it all starts. A serializer is used to convert complex data types, such as Django model instances, into Python data types that can be easily rendered into JSON, XML, or other content types.
Serializer fields handle converting between primitive values and internal datatypes, validating input values, and retrieving and setting values from their parent objects. They are crucial for customizing how data is retrieved for serialization and how data is assigned during deserialization.
Serializer fields are similar to Django Form fields and Django model fields, requiring certain arguments to manipulate their behavior. The most common arguments used in serializer fields include read_only, write_only, required, default, allow_null, source, validators, error_messages, label, help_text, and initial.
These arguments can be used to control how serializer fields behave, such as whether they are read-only or write-only, whether they are required, and what default value to use if no input value is supplied.
Suggestion: Serializers Django Rest Framework
Serialization Formats
Serialization formats in Django are quite versatile, supporting several formats that can be used to serialize data.
Django supports a number of serialization formats, some of which require you to install third-party Python modules.
Here are some of the serialization formats supported by Django:
JSON is one of the most commonly used serialization formats in Django.
A string of the form YYYY-MM-DD as defined in ECMA-262 is used to represent dates in JSON.
A string of the form HH:MM:ss.sss as defined in ECMA-262 is used to represent time in JSON.
A string representing a duration as defined in ISO-8601 is used to represent time deltas in JSON.
Here's an interesting read: Nextjs Response Json
Validations in Serializers
Validations in Serializers are crucial for ensuring the data being serialized is accurate and consistent.
You can define custom validation logic in serializers by using field-level validations, where you define validation logic within the field definition.
For example, the RegexField matches the string to a particular regex, else raises an error. This can be used to validate user input.
Object-level validations allow you to define validation logic that applies to the entire object.
This can be useful for ensuring that certain conditions are met before the serializer can proceed with serialization.
You can use the error_messages argument to specify custom error messages for validation errors.
For instance, you can define a custom error message for a RegexField that raises an error if the input does not match the regex pattern.
Here is an example of how you can use the error_messages argument:
This can be especially helpful when working with user input, as it allows you to provide clear and concise error messages to the user.
Data Types
Data Types are crucial for deserializing data in Django Rest Framework.
In Django Rest Framework, you can use the built-in serializers to handle different data types, such as integers, floats, and strings.
For example, if you have a model field that is an integer, you can use the IntegerField in your serializer to deserialize the data correctly.
In the example of deserializing a user's age, if the API endpoint expects a string, you can use the CharField to handle the deserialization of the string into an integer.
Natural Keys and Forward References
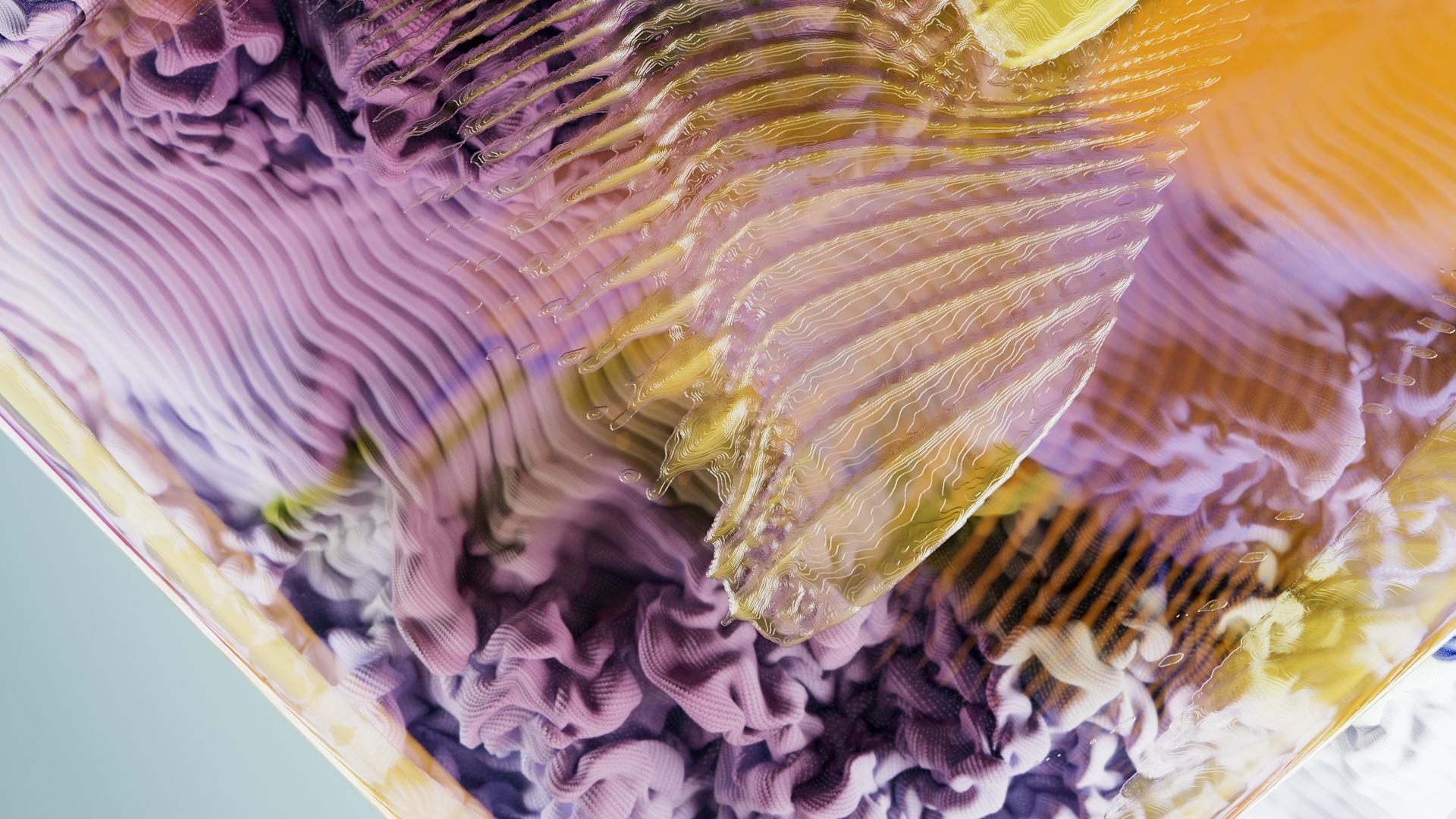
Natural keys are a more humane way to refer to objects, especially when dealing with relationships between objects. They allow you to use meaningful data, like a pair of first and last names, to identify an object instead of a primary key value.
To add natural key handling to a model, you define a default Manager with a get_by_natural_key() method. This method is used to resolve the natural key into the primary key of an actual object.
Whatever fields you use for a natural key must be able to uniquely identify an object, which often means having a uniqueness clause on the field or fields in your natural key. However, uniqueness doesn't need to be enforced at the database level.
Sometimes you'll need to deserialize data where an object has a foreign key referencing another object that hasn't yet been deserialized, known as a "forward reference". To handle this situation, you need to pass handle_forward_references=True to serializers.deserialize().
Numeric
Numeric data types are essential in programming, and they're used to store and manipulate numbers.
An IntegerField is a numeric data type that stores integers, which are whole numbers without decimal points.
FloatField is another type, which stores floating-point numbers, allowing for decimal points.
DecimalField is similar, but it's represented in Python by a Decimal instance, making it more precise than FloatField.
Date and Time
Date and time fields are used to represent different aspects of time. There are four main types of date and time fields: DateTimeField, DateField, TimeField, and DurationField.
A DateTimeField represents a date and time. It's a combination of the two, giving you a precise moment in time.
A DateField, on the other hand, only represents a date. It doesn't include the time component.
A TimeField is similar to a DateField, but it only represents the time of day. It's useful when you need to schedule events without specifying the date.
A DurationField represents a time interval. The validated data for this field contains a datetime.timedelta instance, which is represented as a string in a specific format. This format is '[DD] [HH:[MM:]]ss[.uuuuuu]'.
Choice and Composite
Choice and Composite fields are essential for working with Serializers in Django Rest Framework.
ChoiceField is a field that can accept a value out of a limited set of choices, used by ModelSerializer to automatically generate fields if the model field includes a choices=… argument.
MultipleChoiceField allows you to accept a set of zero, one or many values, chosen from a limited set of choices.
To work with Serializers with confidence, it's crucial to try out these fields and analyze their core arguments.
Composite Fields include ListField, which validates a list of objects, and DictField, which validates a dictionary of objects with string keys.
ListField can be used to validate a list of integers, while DictField can be used to create a field that validates a mapping of strings to strings.
HStoreField is a preconfigured DictField compatible with Django's postgres HStoreField, offering efficient storage of key-value pairs.
Choice Selection
Choice Selection is a crucial aspect of working with Serializers. ChoiceField is a field that can accept a value out of a limited set of choices.
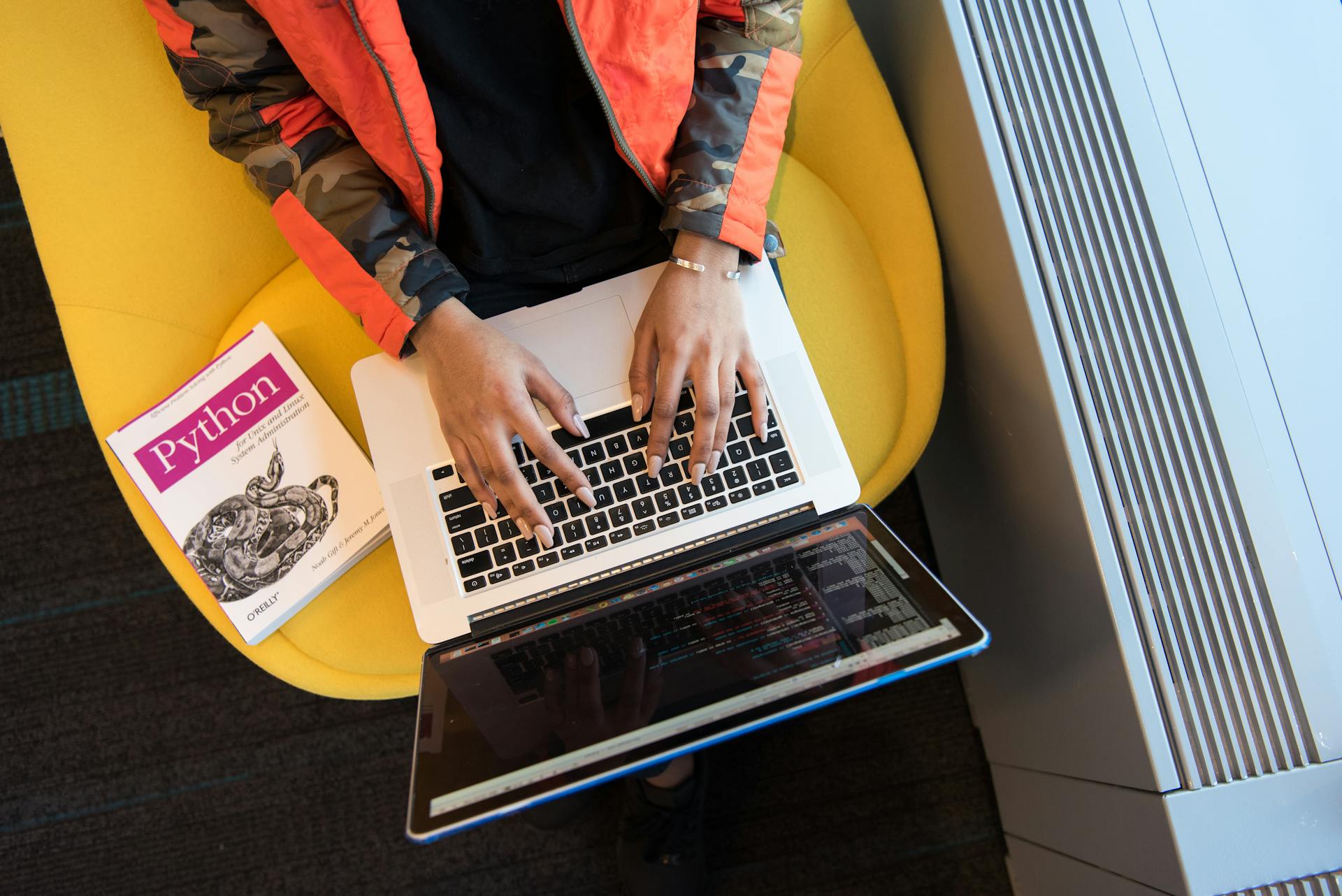
This field is used by ModelSerializer to automatically generate fields if the corresponding model field includes a choices=… argument. I've found that using ChoiceField can save a lot of time and effort when working with complex data.
MultipleChoiceField is another type of field that can accept a set of zero, one or many values, chosen from a limited set of choices. This field is particularly useful when you need to handle multiple selections.
To work with Serializers with confidence, it's essential to try out ChoiceField and MultipleChoiceField at least once and analyze their core arguments.
Composite
Composite fields are a great way to validate complex data in your application.
You can use ListField to validate a list of objects, which is useful when you need to ensure that a certain data structure is followed.
For example, you can use ListField to validate a list of integers.
DictField is another type of composite field that validates a dictionary of objects, where the keys are always assumed to be string values.
This makes it easy to validate a mapping of strings to strings.
HStoreField is a preconfigured DictField that's compatible with Django's postgres HStoreField, offering efficient storage of key-value pairs.
It's well-suited for scenarios where you have a dynamic set of keys with string values.
Documentation Search
Documentation Search is a crucial step in understanding how to deserialize data in Django Rest Framework. You can search for specific information in the DRF documentation using the search bar at the top of the page.
The DRF documentation is well-organized and covers various topics, including serialization and deserialization. You can find detailed information on the `Serializer` class, which is used to define the structure of your data.
One useful resource is the "Serializer" section, where you can learn about the different fields and methods available for serializing and deserializing data. The `to_representation` method, for example, allows you to customize how data is serialized.
The DRF documentation also includes examples of how to use serializers with different data types, such as integers and strings. You can find these examples in the "Examples" section.
To get started with deserializing data, you'll want to create a `Serializer` class that defines the structure of your data. This can be done using the `ModelSerializer` class, which provides a convenient way to serialize and deserialize model instances.
Intriguing read: Django Framework Examples
Sources
- https://www.django-rest-framework.org/api-guide/serializers/
- https://medium.com/django-unleashed/django-rest-framework-serialization-deeper-look-part-1-cf40108f9deb
- https://www.geeksforgeeks.org/serializers-django-rest-framework/
- https://docs.djangoproject.com/en/5.1/topics/serialization/
- https://medium.com/@mathur.danduprolu/serializer-and-modelserializer-in-drf-b5b5c194295d
Featured Images: pexels.com