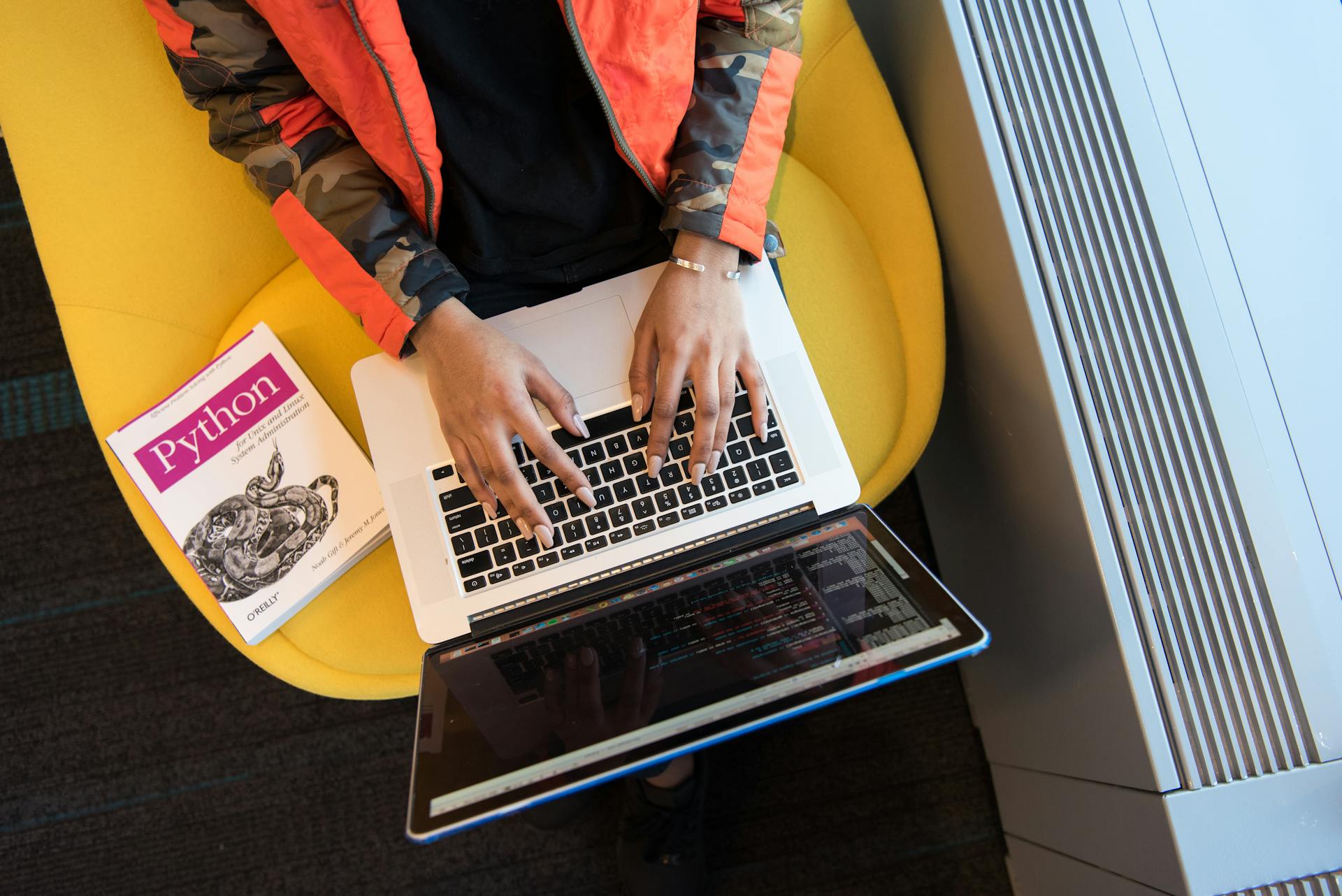
If you're looking to build Python web APIs, Django is an excellent choice. It's a high-level framework that provides a robust set of tools for building scalable and maintainable APIs.
One of the key benefits of using Django is its built-in support for authentication and authorization. This allows you to easily manage user access to your API, which is a crucial aspect of any web application.
Django also provides a flexible and modular architecture, making it easy to structure and organize your code. This is particularly useful for large-scale projects where complexity can quickly become overwhelming.
By leveraging Django's built-in features and tools, you can save time and effort when building your Python web API, and focus on delivering a high-quality product to your users.
Project Setup
To set up a Django project, start by creating a structure for your application. You can do this at any location on your system. Create a virtual environment to isolate package dependencies locally within the project directory using a command like `env\Scripts\activate` on Windows.
A virtual environment is essential to avoid installing Django into a global Python environment and gives you control over the libraries used in an application. You can create a virtual environment in your project folder using a command like `python3 -m venv .venv` on Linux or macOS.
Here are the steps to create a project environment:
- Create a project folder for your tutorial, such as `hello_django`.
- Run the following command to create a virtual environment named `.venv` based on your current interpreter:
- Open the project folder in VS Code and select the Python: Select Interpreter command from the Command Palette.
- Run Terminal: Create New Terminal from the Command Palette to activate the virtual environment.
- Update pip in the virtual environment by running `python -m pip install --upgrade pip`.
- Install Django in the virtual environment by running `python -m pip install django`.
Install
To set up your project environment, you'll want to create a virtual environment. This is done by creating a project folder, such as hello_django, and then using a command to create a virtual environment named .venv.
You'll need to install a virtual environment on your system, which can be done using the command `sudo apt-get install python3-venv` on Linux, or by running `python3 -m venv .venv` on macOS or Windows.
Once you've created the virtual environment, you can open the project folder in VS Code by running `code .` or by using the File > Open Folder command.
Broaden your view: Install Django Project
To select the virtual environment in VS Code, open the Command Palette and select the Python: Select Interpreter command. This will present a list of available interpreters, from which you can select the virtual environment in your project folder.
The selected environment will appear on the right side of the VS Code status bar, with a ('.venv': venv) indicator to let you know you're using a virtual environment.
To ensure you're using the correct environment, update pip by running `python -m pip install --upgrade pip` in the VS Code Terminal, and then install Django by running `python -m pip install django`.
Here's a quick rundown of the steps:
- Create a project folder and virtual environment.
- Open the project folder in VS Code.
- Select the virtual environment in VS Code.
- Update pip and install Django in the virtual environment.
A Project Environment
To set up a project environment for your Django project, start by creating a virtual environment. This can be done by running the command `env\Scripts\activate` on Windows, or `python3 -m venv .venv` on Linux or macOS, to create a virtual environment named `.venv` based on your current interpreter.
A virtual environment is a self-contained environment that allows you to isolate package dependencies locally within the project directory. This is especially useful when working on multiple projects that require different versions of the same package.
To activate the virtual environment, run the command `source .venv/bin/activate` on Linux or macOS, or `.venv\Scripts\Activate.ps1` on Windows. This will activate the environment, and you'll know it's activated when the command prompt shows `(.venv)` at the beginning.
Here are the steps to create a virtual environment:
- On your file system, create a project folder for this tutorial, such as `hello_django`.
- In that folder, use the following command to create a virtual environment named `.venv` based on your current interpreter:
+ Linux: `python3 -m venv .venv`
+ macOS: `python3 -m venv .venv`
+ Windows: `py -3 -m venv .venv`
- Open the project folder in VS Code by running `code .`, or by running VS Code and using the File > Open Folder command.
- In VS Code, open the Command Palette and select the Python: Select Interpreter command.
- Select the virtual environment in your project folder that starts with `./.venv` or `.\.venv`.
- Run Terminal: Create New Terminal from the Command Palette, which creates a terminal and automatically activates the virtual environment by running its activation script.
By following these steps, you'll be able to create a virtual environment for your Django project and isolate package dependencies locally within the project directory.
Django Basics
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design.
It's designed to handle large amounts of data and traffic, making it a popular choice for complex web applications.
Django's batteries-included approach means it comes with a lot of built-in features, including an ORM, authentication and authorization, and admin interface.
This allows developers to build robust and scalable applications quickly and efficiently.
Django's ORM provides a high-level interface for interacting with the database, abstracting away the underlying database technology.
This makes it easy to switch between different databases, such as MySQL and PostgreSQL.
Django's admin interface is a powerful tool for managing data in the database, providing a user-friendly interface for adding, editing, and deleting data.
It's a great way to quickly get up and running with a new project, and it's highly customizable.
Django's authentication and authorization system provides a robust way to manage user accounts and permissions.
It's designed to be highly secure and flexible, making it suitable for a wide range of applications.
Worth a look: Google Data Studio Examples
Testing and Debugging
Testing and debugging are crucial steps in building a Django API. You can test your API by navigating to the URL http://localhost:8000/users using curl.
To make testing and debugging easier, you can create a customized launch profile in VS Code. This allows you to run the server and test the app without typing python manage.py runserver each time.
To create a launch profile, switch to the Run view in VS Code and click on the create a launch.json file link. Select the Django option from the dropdown and VS Code will populate a launch.json file with a Django run configuration.
Here are the steps to follow:
- Start the debugger by selecting the Run > Start Debugging menu command or pressing F5.
- Ctrl+click the http://127.0.0.1:8000/ URL in the terminal output window to open the browser and see that the app is running properly.
You can use the debugger with page templates by setting breakpoints in the template code and running the app in the debugger. This allows you to step through the template code and examine variables in the Variables pane.
Suggestion: Tailwind Css Examples Code
Test Your API
Testing your API is a crucial step in ensuring it's working as expected. You can start the built-in server from the command line.
To access your API, navigate to the URL http://localhost:8000/users using a tool like curl. This will allow you to test the API's endpoints and verify that they're returning the correct data.
Testing the API's endpoints is an essential part of the process. You can use curl to send requests to the API and inspect the responses.
Debugger with Templates
You can have programming errors inside templates just like any other procedural code.
The Python Extension for VS Code provides template debugging when you have "django": true in the debugging configuration.
To use the debugger with page templates, you need to set breakpoints on the procedural code lines in your template files.
Set breakpoints on both the {% if message_list %} and {% for message in message_list %} lines in your template file.
Run the app in the debugger and open a browser to the home page. The debugger will break into the debugger in the template on the {% if %} statement and show all the context variables in the Variables pane.
You can use the Step Over (F10) command to step through the template code and examine each value in message and step to lines like{{ message.log_date | date:'d M Y' }}.
You can also work with variables in the Debug Console panel, but Django filters like date are not presently available in the console.
You might like: Examples of a Web Page Layout
Here's a summary of the steps to use the debugger with templates:
- Set breakpoints on procedural code lines in your template files.
- Run the app in the debugger and open a browser to the home page.
- Use the Step Over (F10) command to step through the template code.
- Work with variables in the Debug Console panel.
Static Files and Templates
Static files are an essential part of any Django project, and they're used to store things like images, CSS files, and JavaScript files.
To refer to static files in a template, you need to create a folder named static within your app's folder, and then create a subfolder named after your app. For example, if your app is named "hello", you would create a folder named "hello" within the static folder.
The reason for this extra subfolder is to prevent file collisions when deploying your project to a production server. This way, your app's static files are collected into a single folder, and they won't interfere with files from other apps in the same project.
Here's an example of how to refer to a static file in a template: {% static 'hello/site.css' %}. This tells Django to look for the file "site.css" in the "hello" subfolder of the static folder.
A base page template in Django contains shared parts of a set of pages, including references to CSS files, script files, and so forth. It also defines block tags with content that extended templates are expected to override.
Here's an interesting read: Host Django Site
Generate URLs
Generating URLs is a crucial step in making your Django app accessible to users. You can generate URLs by opening the urls.py file in a text editor and replacing the default sample code with the code provided in the article.
To create a URL pattern, you need to specify a route that maps to a specific view. For example, in the article, we create a URL pattern that maps the root URL of the app to the views.home function.
Here's a step-by-step guide to creating a URL pattern:
1. Open the urls.py file in a text editor and add the following code:
```python
from django.urls import path
from hello import views
urlpatterns = [
path("", views.home, name="home"),
]
```
This code specifies a URL pattern that maps the root URL of the app to the views.home function.
2. Save the modified file and make sure to include the app's URL patterns in the project's urls.py file.
Expand your knowledge: Next Js App Router Example
Serve Static Files
Serving static files in Django can be a bit tricky, but it's essential for a smooth user experience. To serve static files, you need to include django.contrib.staticfiles in your INSTALLED_APPS list in settings.py.
You can't just add static files anywhere in your project, though. In the project's web_project/urls.py, you need to add the following import statement: from django.contrib.staticfiles.urls import staticfiles_urlpatterns. Then, add the following line at the end: urlpatterns += staticfiles_urlpatterns().
Static files are served as-is, so you'll need to make sure they're in the right place. In your app, create a folder named static, and within that, create a subfolder named hello (matching your app name). This ensures that when you deploy to a production server, your static files won't collide with files from other apps.
Here's a quick rundown of the steps:
- Create a static folder in your app
- Inside the static folder, create a subfolder named hello
- In the static/hello folder, add your static files (like CSS or image files)
- In your template, use the {% load static %} tag to refer to your static files
To collect all your static files into one place, run the command python manage.py collectstatic. This will copy all your static files into a single folder, making it easier to serve them in production. Just remember to run collectstatic every time you change your static files!
In your settings.py, define a location where static files will be collected: STATIC_ROOT = BASE_DIR /'static_collected'. Then, run python manage.py collectstatic to copy your static files into the specified folder.
Discover more: How to Run Django Project
Frequently Asked Questions
Is Django for frontend or backend?
Django is primarily used for backend web development, with some support for front-end development. It's ideal for building web apps quickly and efficiently with Python.
What can Django framework do?
Django can be used to build a wide range of websites, from simple blogs to complex social networks. It's a versatile framework that can deliver content in various formats, making it a great choice for web development projects.
Sources
- https://developer.mozilla.org/en-US/docs/Learn/Server-side/Django
- https://opensource.com/article/19/11/python-web-api-django
- https://codment.com/famous-websites-built-with-the-django-framework/
- https://code.visualstudio.com/docs/python/tutorial-django
- https://developer.mozilla.org/en-US/docs/Learn/Server-side/Django/skeleton_website
Featured Images: pexels.com