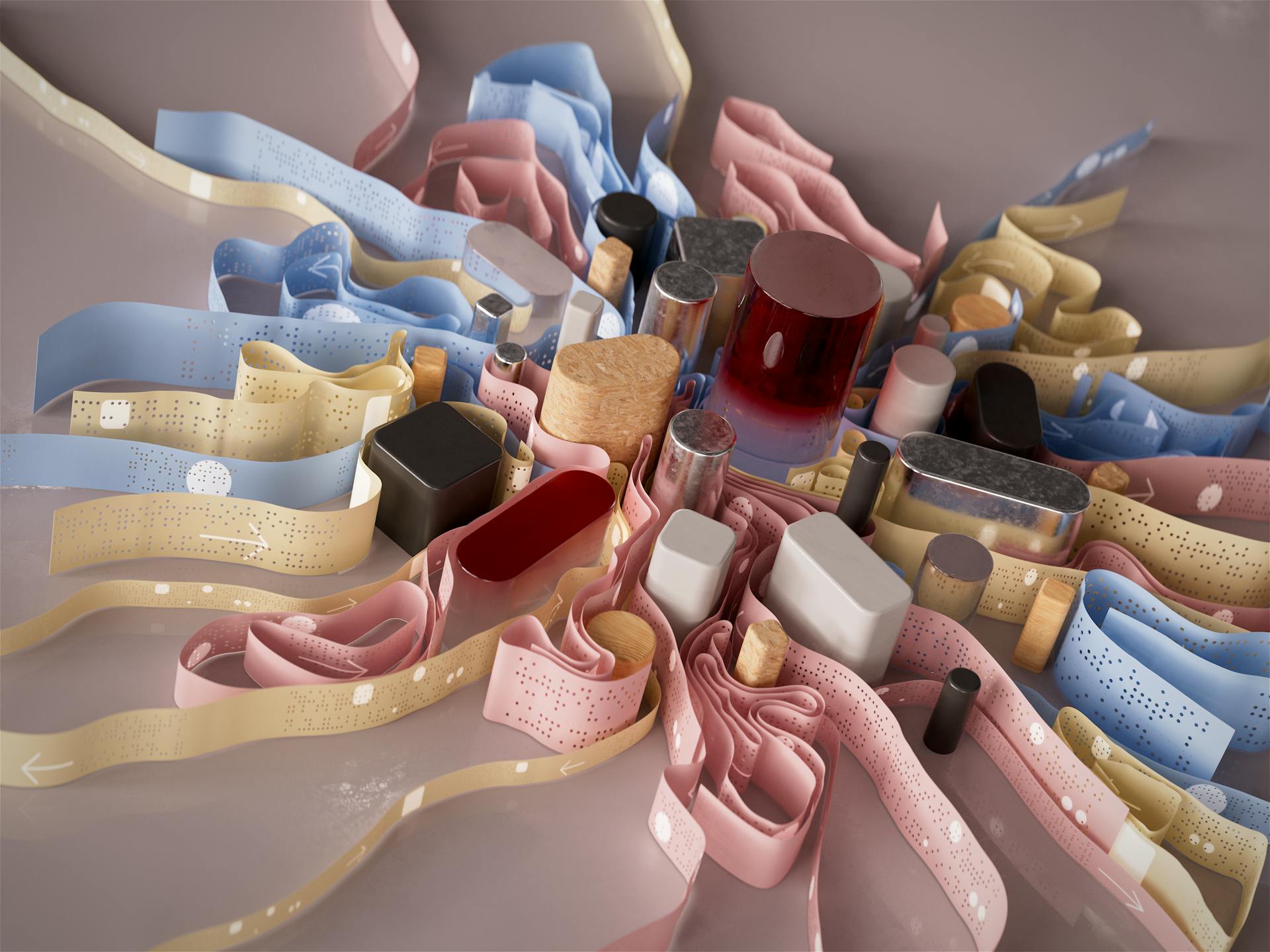
Django Rest Framework (DRF) serializers are a crucial part of building robust and scalable APIs. They help convert complex data types, such as models, into JSON or other formats that can be easily consumed by clients.
Serializers can be used to validate and sanitize data, ensuring that it conforms to the expected structure and format. This is particularly important when dealing with user input, as it helps prevent common web vulnerabilities like SQL injection.
In DRF, serializers are typically created using the `serializers.ModelSerializer` class, which provides a convenient way to define field mappings and validation rules. For example, you can use the `fields` attribute to specify which model fields should be included in the serialized output.
By using serializers effectively, you can simplify your API development process and improve the overall quality of your code.
See what others are reading: Azure Data Factory Rest Api
Serializer Basics
To create a basic serializer, you need to import the serializers class from rest_framework.
You can define fields for a serializer just like creating a form or model in Django.
Importing the serializers class from rest_framework is the first step in creating a serializer.
Serializers are in charge of transforming objects into data formats that front-end frameworks and javascript can understand.
The process of creating a serializer is similar to creating a form or model in Django.
Related reading: Creating Restful Webservices in Java
Outputs
Outputs are a crucial part of working with Django Rest Framework serializers. You can customize the output to suit your needs by overriding specific functions.
To change the serialization output, you can use the to_representation() function. This allows you to add or modify data before it's sent.
The to_internal_value() function is used for deserialization, allowing you to modify the data as it's being converted from JSON to Python objects.
If you need to rename a serializer output field, you can simply add a new field to your serializer and pass it to the fields property.
Here are the key functions to remember:
- to_representation(): changes the serialization output
- to_internal_value(): changes the deserialization output
By using these functions, you can customize your serializer outputs to fit your application's needs.
Serializer Configuration
Serializer configuration is a crucial aspect of Django REST framework serialization. You can set fields as read-only to boost performance during write operations by adding `read_only=True` to the field or specifying them in the `read_only_fields` Meta option.
Setting multiple fields as read-only can be done using `read_only_fields` in the Meta class. This is a more efficient way than explicitly adding `read_only=True` to each field.
You can also use `write_only_fields` Meta option to designate fields as write-only, allowing you to control which fields are included in API output and which are ignored during create and update operations.
Here are the different ways to customize fields in serializer configuration:
- Use `read_only=True` to set a field as read-only.
- Specify multiple fields as read-only using `read_only_fields` in the Meta class.
- Use `write_only_fields` to designate fields as write-only.
Context
You can pass additional data to your serializers using the serializer context property. This data can be used inside the serializer, such as in the to_representation method or when validating data.
The context property is accessed via the self.context dictionary. You can think of it as a way to store extra information that's relevant to the serialization process.
To pass data to the serializer context, you use the context keyword when instantiating the serializer. This keyword is a dictionary that contains the additional data you want to pass.
The context dictionary can be used within any serializer field logic, such as a custom to_representation method, by accessing the self.context attribute. This is a powerful feature that allows you to customize the serialization process based on the specific needs of your application.
Discover more: Azure Data Factory Framework
Write-Only Fields
Designating fields as write-only can be a great way to simplify your serializer configuration. You can use the write_only_fields Meta option to achieve this.
This option allows you to specify multiple fields as write-only at once, rather than adding the write only=True attribute to each field individually.
By using write_only_fields, you can keep your code organized and maintainable.
Nested Serialization
Nested Serialization is a powerful feature in Django Rest Framework (DRF) that allows you to serialize complex data models with ease.
There are two main ways to handle nested serialization with ModelSerializer: Explicit definition and using the depth field.
You can explicitly define nested serialization by creating a serializer for each related model. For example, if you have a Comment model that is related to a Post model, you can create a CommentSerializer and a PostSerializer, and then use the PostSerializer in the CommentSerializer.
Alternatively, you can use the depth field to specify the number of relationships that need to be traversed in depth before returning to a flat representation. The depth field needs to be set to an integer value that represents the number of relationships.
Readers also liked: Serial Number
Here are some examples of how to use the depth field:
By setting the depth field to a specific value, you can control the level of nested serialization and prevent infinite recursion.
Nested objects can be tricky to serialize, but DRF provides a way to handle them using the depth field. For example, if you have a Thing model and a Wrapper model that references the Thing model, you can set the depth field to serialize the Thing model's contents.
However, if you want to create a writable nested relationship, you may need to override the fields in the serializer. For example, if you have a Wrapper model with a foreign key field that references the Thing model, you may need to override the field to make it writable.
You can limit the default fields used in a model serializer by using the fields or exclude options. For example, you can use the fields option to include only specific fields in the serializer, or use the exclude option to exclude specific fields.
By using these techniques, you can effectively handle nested serialization in your DRF application and provide a rich and complex API for your users.
Advanced Usage
You can create customized subclasses of ModelSerializer or HyperlinkedModelSerializer, allowing you to use a different set of default fields if needed.
This type of advanced usage is only required if you have certain serializers that need to be repeated. The ModelSerializer class is the same as a regular Serializer class, except that it's designed to work with Django models.
To override the get_serializer_class() of your ViewSet, you can use a different Serializer in your create and update actions, as shown in the example of using multiple serializers.
Creating customized subclasses is a powerful way to tailor your serializers to specific needs, but it's not always necessary.
API Call Relationships
Relational fields in Django Rest Framework serializers can be a bit tricky, but the default representation is straightforward: it uses the primary keys of associated instances.
The RelatedField in serializers can be used to express the relationship's target by utilizing its __unicode__ method.
When serializing a wrapper, you might need to pass just the PK of the model object to use the serializer as-is.
NestedSerializers are mentioned in the DRF 3.2.0 release notes, but their purpose is unclear.
Serializer Wrapping
Serializer wrapping is a powerful technique in Django Rest Framework (DRF) that allows you to create a new object with a nested object pointing to an existing one.
To achieve this, you can't just pass the ID of the existing object to the serializer's create() method, as it won't be reflected in the validated_data.
You can try passing the existing object itself to the serializer's create() method, but it won't work either.
Nested objects can be tricky to handle in DRF, but with the right approach, you can get the desired result.
You can use the depth parameter in the serializer to include the nested object's contents in the response.
However, DRF's ModelSerializer does not support writable nested relationships by default, which means you need to do some extra work to make it work.
If you create a ModelSerializer for the wrapper model without overriding any fields, DRF will create a new Thing object and a Wrapper object with a thing field pointing to that new Thing object.
Sources
- https://testdriven.io/blog/drf-serializers/
- https://www.geeksforgeeks.org/serializers-django-rest-framework/
- https://dev.to/abdenasser/my-personal-django-rest-framework-serializer-notes-2i22
- https://www.scaler.com/topics/django/serializers-in-django/
- https://cheat.readthedocs.io/en/latest/django/drf_serializers.html
Featured Images: pexels.com