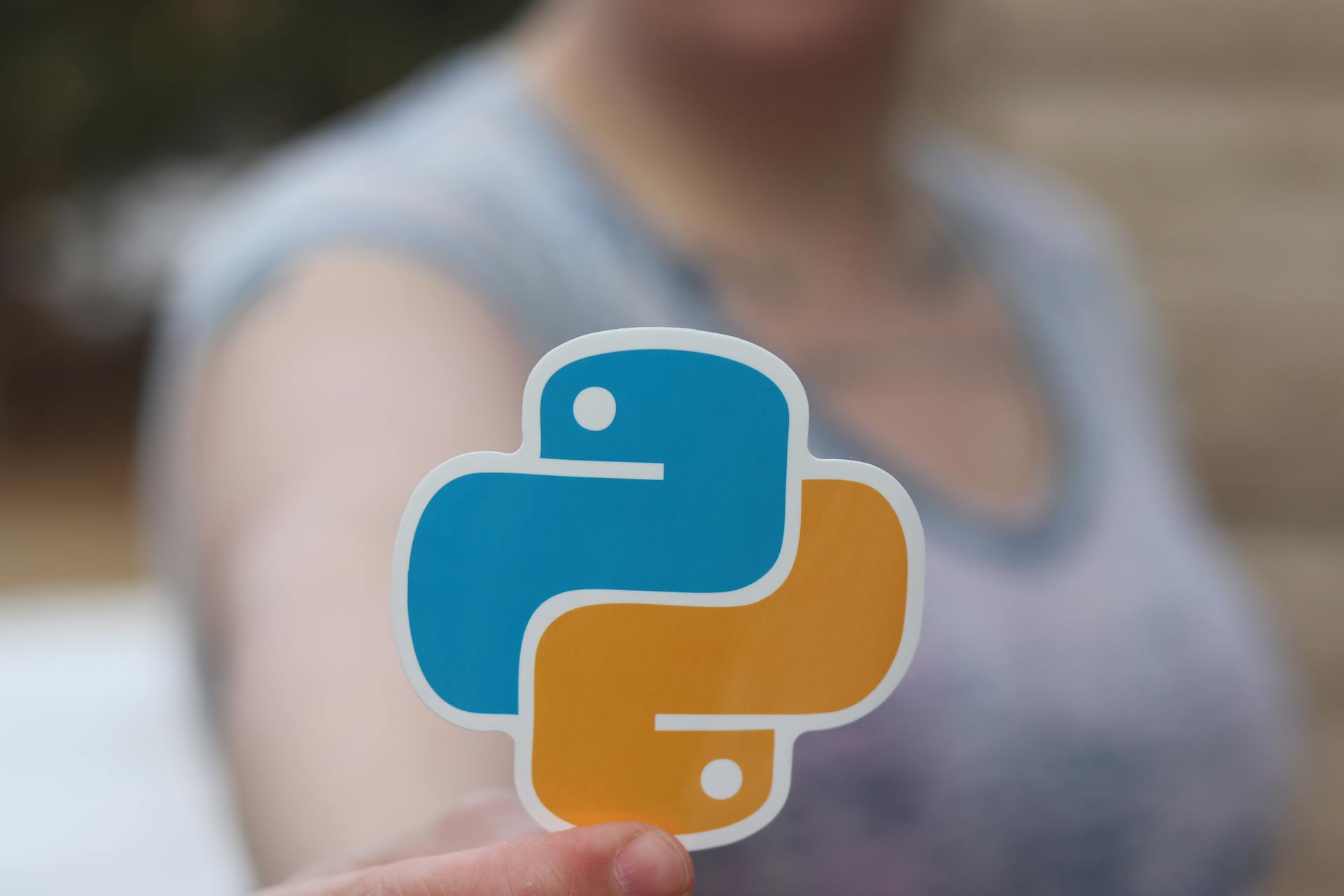
Building a Python web API with FastAPI is a straightforward process. You can start by installing the required packages, including FastAPI and Uvicorn, using pip.
FastAPI is built on top of standard Python type hints, making it easy to create robust and maintainable APIs. With its powerful features and tools, you can build high-performance APIs quickly.
To begin building your API, you'll need to create a new FastAPI application instance. This is done by importing the FastAPI class and creating an instance of it, like so: `app = FastAPI()`.
FastAPI's auto-documentation feature allows you to automatically generate API documentation, making it easy to share and collaborate on your API.
Getting Started
To get started with building Python web APIs with FastAPI, you'll need to set up a virtual environment. This is done by creating a folder for your project, navigating to it in your terminal, and running the command `python -m venv env` to create a virtual environment named `env`.
You should then activate the virtual environment by running the command `source env/bin/activate` on Linux or macOS, or `env\Scripts\activate` on Windows. This will indicate that you're in the virtual environment with `(env)` at the beginning of your terminal prompt.
To install FastAPI and its dependencies, you can use the pip package manager by running the command `pip install fastapi`. You'll also need to install a server like Uvicorn, which you can do by running `pip install uvicorn`.
Project Setup
To set up your project, create a virtual environment by running the command `python -m venv env` in your terminal. This isolates your project from the rest of your system, allowing you to use different versions of packages without conflicts.
A virtual environment is a way of keeping your project organized, especially as it grows in complexity. You can create a folder for your project and navigate to it in your terminal.
To activate your virtual environment, run the command `source env/bin/activate` on Linux or macOS, or `env\Scripts\activate` on Windows. You should see `(env)` at the beginning of your terminal prompt, indicating that you are in the virtual environment.
Now that your virtual environment is set up, install the required dependencies, including FastAPI and Uvicorn. You can do this by running `pip install fastapi` and `pip install uvicorn` in your terminal.
Here are the specific packages you need to install:
With these packages installed, you can start creating your FastAPI project. Create a file named `main.py` in your project folder and open it in your preferred code editor. Then, write the following code to import the FastAPI class and create an app instance: `from fastapi import FastAPI; app = FastAPI()`.
What Is?
FastAPI is a modern, high-performance Python web framework designed for building APIs quickly and easily.
It was created as an alternative to other popular frameworks like Flask and Django, offering improved performance and productivity through its unique features.
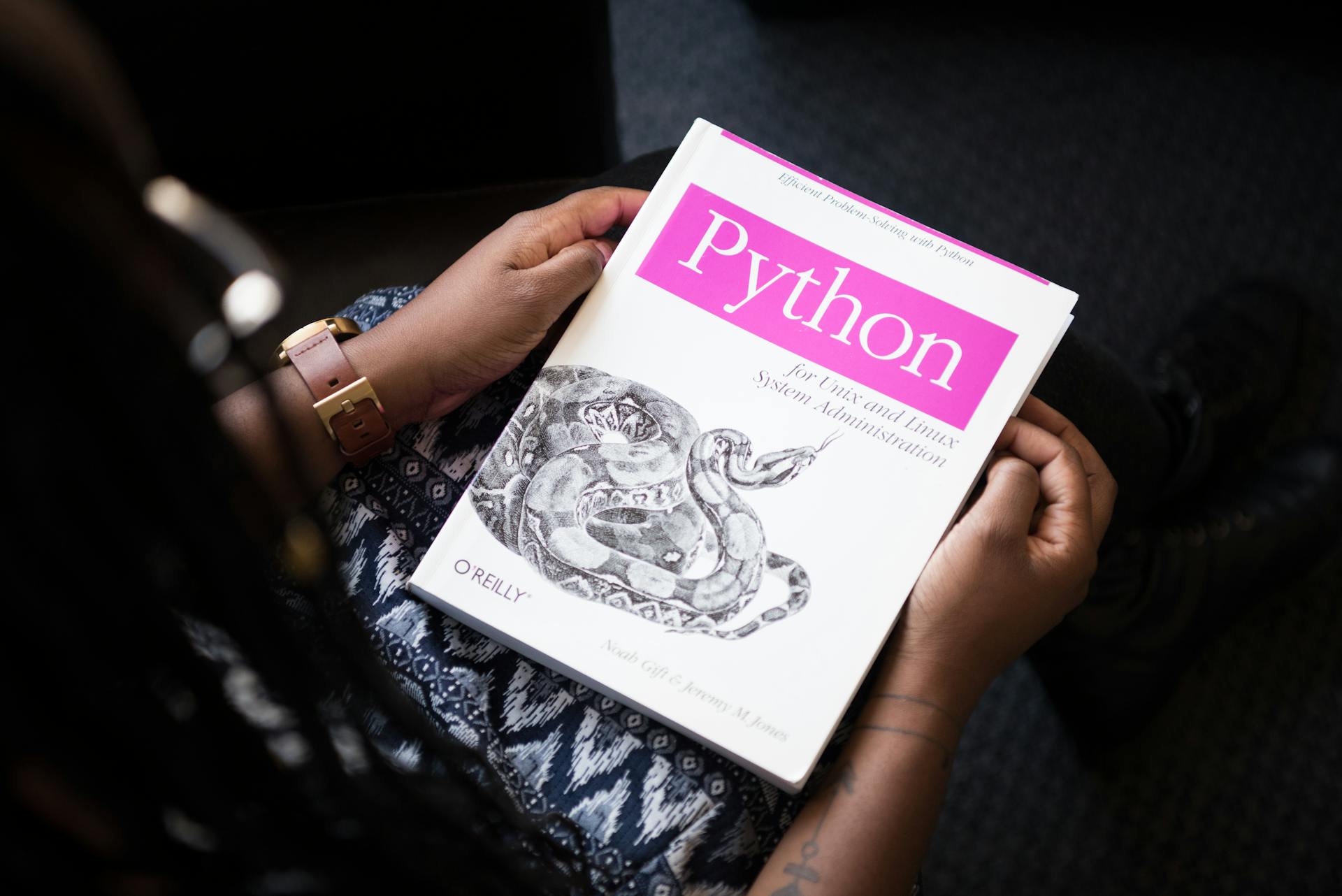
FastAPI leverages Python type hints to provide a more robust and developer-friendly experience, ensuring automatic validation, serialization, and documentation.
Its unique approach helps minimize code repetition and errors, making the development process smoother and more efficient.
FastAPI supports asynchronous programming, which allows for handling multiple tasks simultaneously without blocking the execution of other tasks.
This makes it highly suitable for applications requiring real-time data processing or those with high concurrency demands.
FastAPI integrates seamlessly with popular data validation libraries like Pydantic, which ensures data integrity and consistency across the application.
FastAPI includes built-in support for OpenAPI and JSON Schema, which means developers can automatically generate interactive API documentation.
This feature is invaluable for both development and client-facing stages, providing clear and concise documentation that can be easily navigated and tested.
How Can We Help?
So you're looking to get started with building Python Web APIs, but not sure where to begin? iTechnolabs can help you design and plan your API architecture, ensuring it meets both current and future requirements.
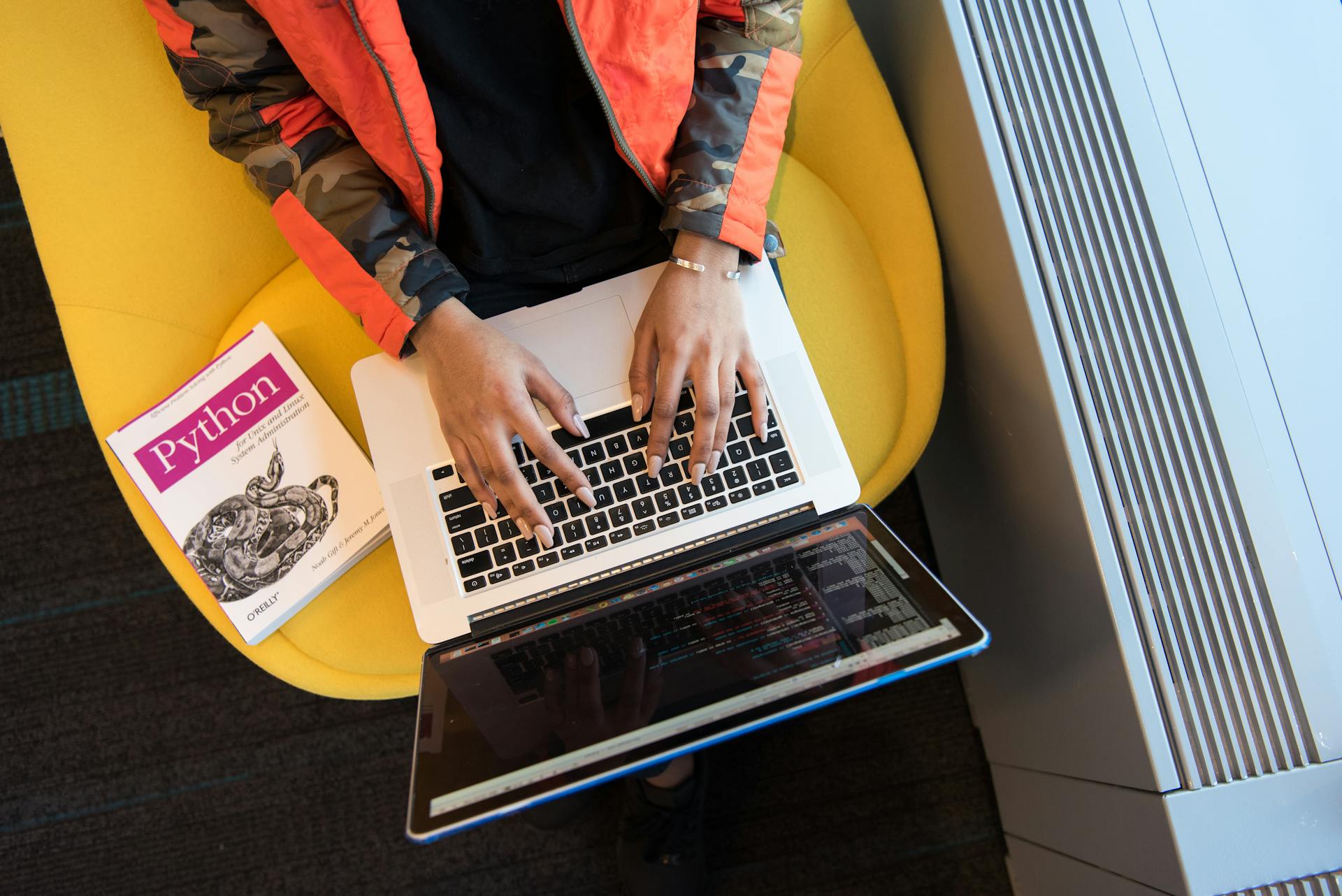
We offer expert consultation to provide guidance on best practices, architectural patterns, and technology recommendations. This ensures your API is well-structured and scalable.
Our team of experienced developers can assist you in developing and deploying Web APIs using the FastAPI framework. We follow industry best practices to ensure your API is secure, scalable, and easy to maintain.
We can also help you create thorough and automatic API documentation for ease of use. This includes utilizing pydantic models to ensure your API data is validated and type-checked, preventing errors and making your API more reliable and robust.
Here are some of the specific services we offer to help you get started:
- Expert Consultation: Get guidance on designing and planning your API architecture.
- Development Services: We'll help you develop and deploy your Web API using FastAPI.
- Code Optimization: We'll optimize your API code for performance and efficiency.
- Security Implementation: We'll integrate robust security features to protect your API.
- Scalability Solutions: We'll design scalable solutions to grow with your application needs.
- Documentation Support: We'll assist in creating thorough and automatic API documentation.
- Validation and Type Checking: We'll utilize pydantic models to ensure data integrity.
- Testing and Debugging: We'll conduct extensive testing and debugging to ensure your API functions correctly.
Designing and Implementing Endpoints
To create a robust API, you need to design and implement your endpoints thoughtfully. Start by defining the main resources or entities your API will deal with, such as posts, comments, users, and tags. This will help you determine the operations or actions your API will support for each resource.
Next, decide on the parameters or filters your API will accept for each operation. For example, you might want to specify the page number, page size, sort order, or search query for getting a list of posts. The data models or schemas you use for each resource are also crucial, defining the fields and types of a post, such as the title, content, author, date, and tags.
To design your API schema, think about the following aspects:
- What are the main resources or entities that your API will deal with?
- What are the operations or actions that your API will support for each resource?
- What are the parameters or filters that your API will accept for each operation?
- What are the data models or schemas that your API will use for each resource?
- What are the status codes and error messages that your API will return for each operation?
Once you have a clear idea of your API schema, you can start writing the code for your FastAPI app. FastAPI makes it easy to design the API schema, as it is based on the OpenAPI specification, which is a widely used and standardized format for describing web APIs.
To implement your API endpoints, you can use Python decorators to register functions as API endpoints, specifying the HTTP method, path, and parameters. For example, the following code defines an endpoint for getting a list of posts:
```python
@app.get("/posts")
async def read_posts(page: int = 1, size: int = 10):
posts = await get_posts(page, size)
return {"posts": posts}
```
This code imports FastAPI and Pydantic, creates an app instance, defines a data model for a post using Pydantic, defines a sample list of posts, and defines an endpoint for getting a list of posts using the `@app.get` decorator. The decorator takes the path as an argument, and the function takes the parameters as arguments, annotated with the `Query` class, which validates and parses the query parameters.
You can test this endpoint by visiting `http://localhost:8000/posts` or `http://localhost:8000/posts?page=2&size=2` in your browser, or by using the Swagger UI or ReDoc interfaces.
Managing Data and Requests
FastAPI makes it easy to manage data and requests with its built-in support for path parameters. You can attach short data directly to the URL path, making it convenient to access data sent through the URL path.
To implement path parameters, you can use the example provided, where the name is a path parameter extracted from the URL and passed as a parameter to the get_name function.
FastAPI also supports query parameters, which are similar to path parameters but are appended to the URL after a question mark. You can override their default values by providing different values in the URL.
Here are the types of data you can pass in a request:
- Path parameters
- Query parameters
- Body parameters
- Headers
- Cookies
You can implement body parameters by defining a pydantic model that inherits from BaseModel, as shown in the example. This will define the request body parameters and provide more context to FastAPI.
Using Query Parameters
Query parameters are a great way to pass information to your API endpoints. They are appended to the URL with a question mark.
You can use query parameters to filter or sort the results of your API. For example, you can use a query parameter to get a list of posts by a specific author.
To define a query parameter, you can use the Query class from FastAPI. The Query class validates and parses the query parameter, using the arguments that are passed to it.
For example, the following code defines an endpoint for getting a list of posts by a specific author, using a query parameter:
You can test this endpoint by visiting http://localhost:8000/posts?author=Alice or http://localhost:8000/posts?author=David in your browser.
Here are some common query parameter types:
By using query parameters, you can make your API more flexible and user-friendly.
Managing Bodies
You can attach short data directly to the URL path using path parameters. This is done by attaching the data to the URL path, and FastAPI will extract it and pass it as a parameter to the endpoint function.
The data sent through the URL path can be accessed conveniently, making it a convenient way to pass data to an endpoint.
To implement query parameters in a FastAPI's endpoint, you can append the parameters to the URL after a question mark. This is different from path parameters, which are attached directly to the URL path.
You can override default values by providing different values in the URL.
You can encode data and append it to the request made to the endpoint using body parameters. This is implemented by defining a pydantic model that inherits from BaseModel, which defines the request body parameters.
FastAPI will automatically deserialize and validate the incoming data against your model when you use a pydantic model as a request body.
You can use both path parameters and a request body in the same endpoint function, allowing you to access data from different sources.
Here's a summary of the different ways to manage bodies in FastAPI:
You can declare path parameters and a request body at the same time, and FastAPI will take care of doing all the data validation, serialization, and documentation for you.
Error Handling and Security
Error handling is crucial for building reliable and robust Python web APIs with FastAPI. Proper error handling improves the reliability and robustness of your API.
Anticipating and handling errors and exceptions is essential, as they can disrupt the normal flow of your program. Errors and exceptions can occur when the client sends invalid or incomplete data, the server cannot connect to the database or another service, the server runs out of memory or disk space, or the server encounters an unexpected bug or logic error in the code.
You can improve the reliability and robustness of your API by using built-in and custom exceptions to raise and catch errors, and by using HTTP status codes to indicate the outcome of the request.
Error Handling
Error handling is crucial for any web API to ensure reliability and robustness. Improving the reliability and robustness of your API is one of the reasons why error handling is important.
You might encounter errors and exceptions when the client sends invalid or incomplete data to your API, or when the server cannot connect to the database or another service. The server running out of memory or disk space, or encountering an unexpected bug or logic error in your code, can also cause errors and exceptions.
To handle errors and exceptions properly, you should use built-in and custom exceptions to raise and catch errors. This allows you to provide meaningful and helpful feedback to the client and the developer.
Here are some reasons why error handling is important:
- Improving the reliability and robustness of your API.
- Providing meaningful and helpful feedback to the client and the developer.
- Preventing security breaches and data leaks.
- Debugging and troubleshooting your code more easily.
To indicate the outcome of the request, you can use HTTP status codes. For example, you can use the 400 Bad Request status code when the client sends invalid or incomplete data to your API.
Auth and Authorization
Auth and Authorization is a crucial aspect of web API security. Authentication is the process of verifying the identity of the client making the request, while authorization is about granting or denying access to resources and operations based on the client's identity and permissions.
You can implement authentication and authorization in your web API using various methods, such as JSON Web Tokens (JWT), OAuth2, Basic Auth, API keys, and more. FastAPI's built-in support for OAuth2 with JWT makes it easy to add authentication and authorization to your web API.
To create a user model and database for storing user information, you can use a library like Pydantic to define the user model. For example, you can create a user model with fields like username, email, and password.
Here are the steps to create a user model and database:
- Create a user model using Pydantic
- Define the fields for the user model, such as username, email, and password
- Create a database to store user information
You can also create endpoints for registering new users and logging in existing users. For example, you can create an endpoint for registering new users by sending a POST request to a URL like /register with a JSON body containing the user's information.
Here are the steps to create an endpoint for registering new users:
- Create an endpoint for registering new users
- Send a POST request to the endpoint with a JSON body containing the user's information
- Hash the user's password before storing it in the database
Additionally, you can create a dependency function for verifying and decoding JWT tokens. This function can be used to authenticate users and authorize access to specific endpoints.
Here are the steps to create a dependency function for verifying and decoding JWT tokens:
- Create a dependency function for verifying and decoding JWT tokens
- Use the function to authenticate users and authorize access to specific endpoints
- Use the function to verify the JWT token sent in the Authorization header
You can also create a permission-based system for restricting access to certain endpoints. For example, you can create an endpoint that requires a user to be an administrator to access.
Here are the steps to create a permission-based system:
- Create a permission-based system for restricting access to certain endpoints
- Define the permissions required for each endpoint
- Use the permission-based system to restrict access to endpoints based on the user's role or permissions
Testing and Documentation
Testing and documentation are crucial steps in building a robust Python web API with FastAPI. Testing helps you find and fix bugs, errors, and vulnerabilities in your code, while documentation makes it easy for other developers to understand your API's structure and usage.
FastAPI automatically generates documentation, including information about path parameters, which is a huge time-saver. This documentation is interactive, making it easy for users to explore and understand your API.
To ensure proper functionality and error handling, it's essential to test a variety of input values for any path parameters when writing tests for your endpoint. This is especially important when using FastAPI, which can automatically generate documentation for your API.
Testing and Documenting
Testing and documenting your web API is crucial for ensuring its quality, functionality, and usability. This involves testing your API to find and fix bugs, errors, and vulnerabilities in your code, as well as verifying that it meets the requirements and expectations of your users.
Testing your web API helps you detect and prevent bugs and errors in your code, ensure that your code meets the specifications and requirements of your API, refactor and improve your code without breaking its functionality, and measure and improve the quality and coverage of your code.
You can write unit and integration tests for your API endpoints using pytest and TestClient. Unit tests check the behavior of a single unit of code, while integration tests check the interaction and integration of multiple units of code.
Here are some benefits of writing unit and integration tests for your web API:
- Detect and prevent bugs and errors in your code.
- Ensure that your code meets the specifications and requirements of your API.
- Refactor and improve your code without breaking its functionality.
- Measure and improve the quality and coverage of your code.
FastAPI automatically generates documentation for your API, including information about path parameters. This makes it easy for other developers to understand the structure and usage of your API.
Learn More About
Learning more about FastAPI can take your development skills to the next level. You can learn about query parameters to customize requests, which is a great way to tailor your API to specific needs.
Dependency injection is another powerful feature of FastAPI that allows you to handle reusable logic for permissions, database sessions, and more. It's a game-changer for complex applications.
Security utilities in FastAPI make it easy to integrate authentication and authorization based on standards. This ensures your API is secure and reliable.
Background tasks in FastAPI are perfect for simple operations like sending an email notification. They run in the background, so you can focus on other tasks.
FastAPI also supports concurrency and improves performance with async and await. This means you can handle multiple requests at the same time, making your API more efficient.
If you need real-time communication, FastAPI's WebSockets feature is the way to go. It's ideal for advanced use cases that require live updates.
To build bigger applications, you can use FastAPI with multiple files. This makes it easy to organize your code and keep track of your project.
Here are some key features to explore further:
- Query parameters
- Dependency injection
- Security utilities
- Background tasks
- async and await
- WebSockets
- Bigger applications in multiple files
Advanced Features
FastAPI's advanced features make building robust and scalable web APIs a breeze.
With the ability to add custom authentication and authorization, you can secure your API with ease.
You can also use FastAPI's built-in support for asynchronous programming to handle multiple requests concurrently, making it perfect for high-traffic applications.
By leveraging these features, you can create a web API that's both fast and secure.
Advanced Concepts
APIs can become complex, requiring more than just basic functionality. You'll need to validate credentials before performing requests or handling files. In the inventory app, you'll be adding validation middleware to ensure secure data handling.
APIs may need to persist data, which is achieved through a database. The inventory app will utilize a database to store and manage data.
File upload functionality is another advanced concept. The inventory app will be able to handle file uploads, allowing users to upload files as needed.
These advanced concepts will take your inventory app to the next level, providing a more robust and feature-rich experience.
Editor Features
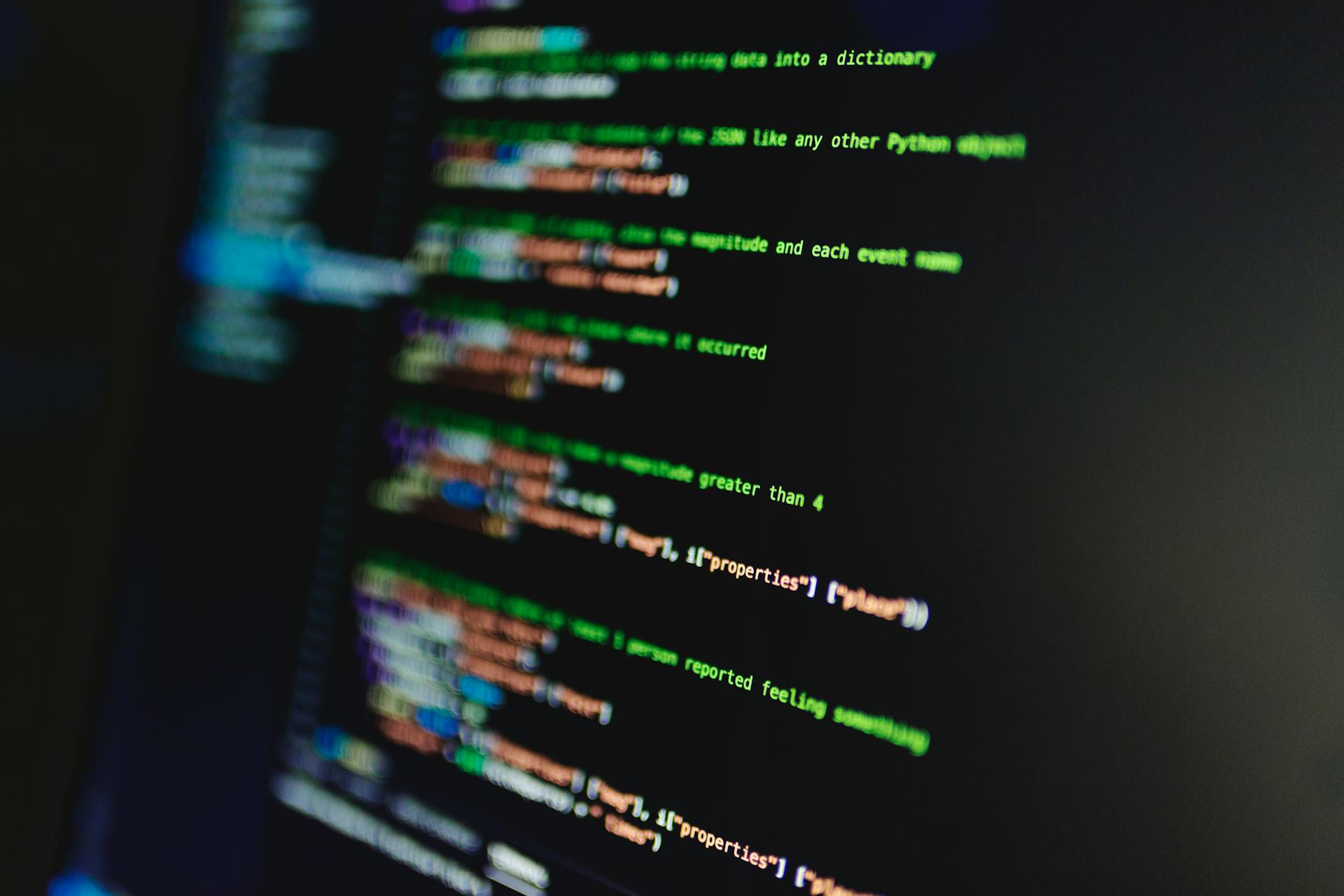
Using Pydantic models can significantly enhance your development experience by reducing the need to constantly refer to documentation.
You can get autocompletion for your model's attributes in your IDE or code editor, making writing code faster and more error-free.
Pydantic models offer validation and type-checking, which further aids in writing robust and reliable code.
This is especially useful when working with JSON data, as you can get type hints and completion everywhere inside your function.
You'll get error checks for incorrect type operations, such as trying to sum a string with a float, which can warn you of potential errors in your code.
The Pydantic framework was built around this design, and it was thoroughly tested at the design phase to ensure it would work with all editors.
You can get the same editor support with PyCharm and most other Python editors, including Visual Studio Code.
Here are some editors that offer great support for Pydantic models:
- Visual Studio Code
- PyCharm
- Most other Python editors
If you use PyCharm, you can use the pydantic PyCharm plugin to improve your editor support, and if you use VS Code, you'll get the best developer experience with Pylance.
Comparing Python Frameworks
FastAPI, a relatively new addition to the backend API ecosystem, competes with established Python giants such as Flask and Django.
FastAPI provides two alternative ways to show API documentation, including ReDoc, which is an automatic documentation provider. This is in addition to the standard OpenAPI documentation.
Django is a feature-rich Python backend framework that includes a variety of built-in libraries, making it suitable for complex web applications. It has powerful features like ORM, authentication mechanisms, and routing capabilities.
FastAPI shines as a nimble microframework that was purposefully designed to be lightweight, compensating for its lack of a large library ecosystem by being extremely fast.
Here's a comparison of some key features of FastAPI and other popular Python frameworks:
Flask is a lightweight framework used by Python developers to quickly build web applications. It stands out for its design, giving developers more control and flexibility when structuring their applications.
Pyramid is another Python framework that adheres to the "use only what you need" philosophy, providing a minimalistic core that can be supplemented with various add-ons and libraries. This modular approach enables developers to pick and choose which components they need for their specific use cases.
Conclusion and Next Steps
Congratulations on completing this guide to building Python web APIs with FastAPI! You now have valuable insight into using this powerful tool for your backend application.
FastAPI is a user-friendly and highly effective API development tool that provides flexibility and exceptional performance.
With FastAPI, you can build scalable and efficient APIs that meet your development requirements. Its microframework design makes it an excellent choice for API development.
You should now be equipped to tackle your next project with confidence, using the skills and knowledge you've gained from this guide.
Sources
- https://github.com/PacktPublishing/Building-Python-Web-APIs-with-FastAPI
- https://refine.dev/blog/introduction-to-fast-api/
- https://gpttutorpro.com/fastapi-best-practices-tips-and-tricks-for-building-better-web-apis/
- https://itechnolabs.ca/creating-python-web-apis-with-fastapi/
- https://realpython.com/fastapi-python-web-apis/
Featured Images: pexels.com