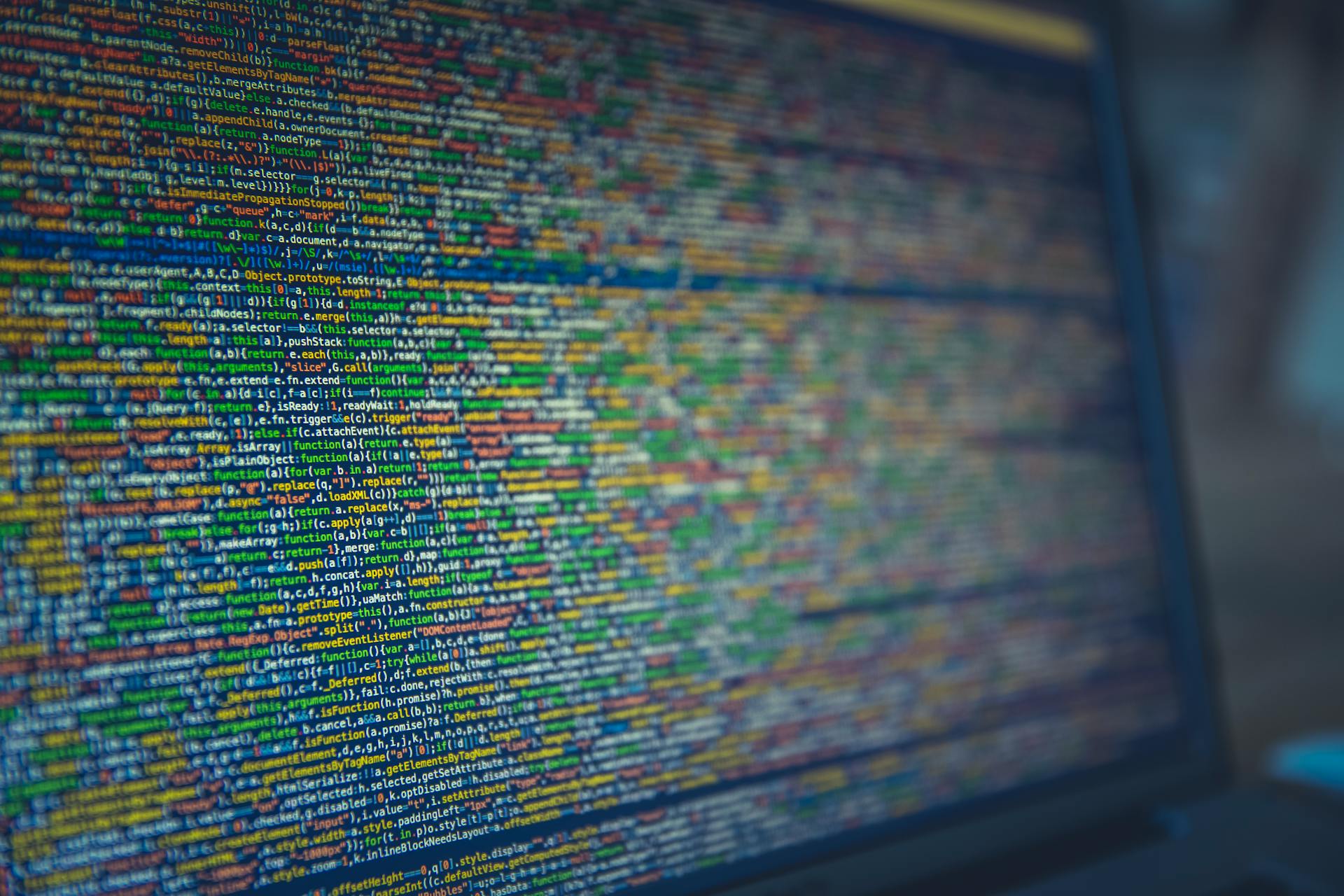
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It's ideal for building web APIs quickly and efficiently.
FastAPI is designed to be highly scalable and performance-oriented, making it a great choice for building high-traffic APIs.
With FastAPI, you can build APIs that are both fast and secure, thanks to its built-in support for features like authentication and rate limiting.
Next.js, on the other hand, is a popular React-based framework for building server-side rendered (SSR) and statically generated websites. It's known for its simplicity and ease of use.
By combining FastAPI and Next.js, you can create a full-stack web development experience that's both fast and scalable.
Consider reading: Is Django a Framework
Initial Project Setup
To get started with your FastAPI Next.js project, you'll want to set up your initial project structure. Create a directory to store all your code, and for this tutorial, we'll keep everything in one repository for simplicity.
A good place to start is by creating a directory for your FastAPI application in the project root. This will be the main hub for your back-end code.
Create and activate a Python virtual environment to isolate your project's dependencies. This is a good practice to keep your project's dependencies organized and avoid conflicts with other projects.
You'll need to install some dependencies to get started. Here are the ones you'll need:
- fastapi – the web framework
- uvicorn – the ASGI server
- gunicorn – the WSGI server
- psycopg2 – the PostgreSQL driver
- sqlalchemy – the Python SQL toolkit and object relational mapper
- databases – asyncio support for databases
- alembic – the database migration tool
These dependencies will provide the foundation for your back-end application. Make sure to install them using pip, and you'll be ready to start building your project.
Security
Security is a top priority in any web application, and FastAPI Next.js is no exception. To protect against cross-site request forgery (CSRF) attacks, NextAuth implements a signed double submit cookie technique.
This involves generating a cryptographically secure random value, putting it in a cookie, and including the same value in another channel of communication, such as the headers of the request or a hidden form field. An attacker won't know the random value, and even if they manipulate a user into sending a request, they won't be able to put the value into the second channel, allowing the server to reject the request.
To validate the CSRF token, the server calculates a hash of the random value with a secret only known to the server concatenated to it. This ensures that the CSRF value is set by the server, not an attacker. The token is then verified by checking if it matches the value set in the other channel, providing a reasonable guarantee that the request is legitimate.
Broaden your view: Next Js Csrf
Csrf Protection
CSRF Protection is a crucial security measure that prevents malicious actors from performing unwanted actions on behalf of an authenticated user.
CSRF attacks can be devastating, allowing an attacker to nuke your account by manipulating you into navigating to a link that sends your JWT cookie to the API.
The double submit cookie technique is used for CSRF prevention, involving generating a random value, putting it in a cookie, and including the same value in the headers of the request or a hidden form field.
This technique is vulnerable to defeat, so NextAuth uses a signed double submit cookie, which involves the server calculating a hash of the random value with a secret only known to the server concatenated to it.
The CSRF token in the cookie is equivalent to the token and its hash concatenated with a | as a separator between them.
To validate the CSRF token, we need to separate the token and its hash by splitting on | on the value in the cookie, verify that the token was indeed set by the server, and compare the token in the cookie with the value set in the other channel.
If the token and its hash match, we can be reasonably sure the request is legit, but we still need to write a dependency to use it in FastAPI, which involves a bit too much code to put in this post.
Using the Tokens
You can use the tokens in FastAPI by specifying dependencies that can be used on routes, allowing you to share logic and reuse code.
FastAPI has a powerful dependency injection system that makes it easy to share logic and reuse code.
To decrypt the token in Python, you'll want to use a library that supports AES256GCM in "dir" mode, such as python-jose.
This library supports decrypting JWTs with just two arguments: an encrypted payload and a key.
The key is derived from NEXTAUTH_SECRET using HKDF, which can be implemented using the cryptography library.
You can call the HKDF function like this to get the derived key.
To protect routes with NextAuth tokens, you can use the dependency injection system to specify routes that require a valid token cookie.
The contents of the JWT are also available as a dictionary in the route handler, allowing you to use the contents of the token for whatever you need.
Sources
- https://www.geeksforgeeks.org/server-side-google-authentication-using-fastapi-and-reactjs/
- https://code.visualstudio.com/docs/python/tutorial-fastapi
- https://www.travisluong.com/how-to-build-a-full-stack-next-js-fastapi-postgresql-boilerplate-tutorial/
- https://tom.catshoek.dev/posts/nextauth-fastapi/
- https://stackoverflow.com/questions/78727205/405-method-not-allowed-error-in-vercel-fastapi-backend-but-works-locally
Featured Images: pexels.com