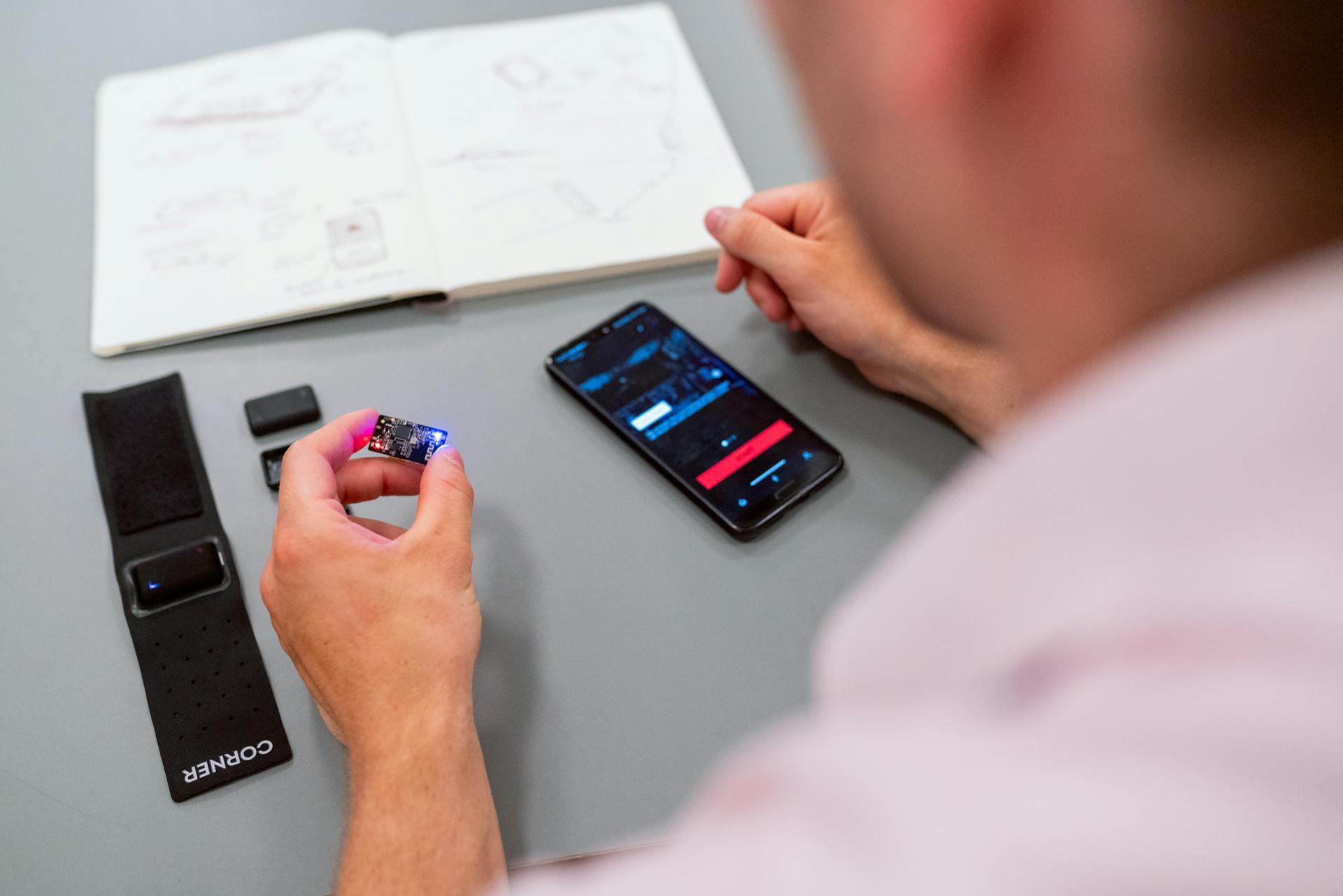
CSRF attacks are a serious concern for Next.js developers.
A CSRF attack occurs when an attacker tricks a user into performing an unintended action on a web application that the user is authenticated to.
To prevent CSRF attacks in Next.js, we can use the built-in CSRF protection feature.
This feature uses a token-based approach to validate requests. The token is included in a hidden form field and verified on the server-side.
Next.js provides a simple way to enable CSRF protection by setting the "csrf" option to true in the Next.config.js file.
Take a look at this: Nextjs Client Session Token
Understanding Attacks
CSRF attacks exploit the trust between users and web applications, taking advantage of the implicit trust placed in an authenticated user's session.
A CSRF attack is a malicious exploit where an attacker tricks an unsuspecting user into submitting a web request that the user did not intend to submit or may not even be aware of.
CSRF attacks can result in undesirable consequences, such as making unauthorized financial transactions.
The success of a CSRF attack relies on the trust established between the targeted website and the victim's browser.
A CSRF attack usually consists of five steps: authentication of the victim, attack setup, victim interaction, automatic request, and trust exploitation.
Here are the five steps of a CSRF attack:
- Authentication of the victim: The attacker checks that the victim is authenticated as a legitimate user of the targeted website, with an active session on the website.
- Attack setup: The attacker creates a malicious webpage or uses an already compromised one. The attacker then builds a malicious request that targets the vulnerable web application and includes all the necessary parameters and instructions to perform a malicious operation on behalf of the victim.
- Victim interaction: The attacker tricks the victim into visiting the malicious webpage, often using social engineering techniques such as false emails, phishing or alluring links.
- Automatic request: The malicious webpage or link automatically sends a request to the targeted web application in the victim's browser, transmitting the disguised malicious request along with the victim's session cookies.
- Trust exploitation: Since the victim is already authenticated on the targeted website, the server considers the request legitimate and executes the illegitimate operation, such as completing a purchase, changing account settings or deleting data.
These attacks can be difficult to detect because the victim is already authenticated on the targeted website.
Countermeasures to Attacks
To better safeguard the security of web applications, it is essential to protect against CSRF attacks. Fortunately, several countermeasures can be employed to add an extra layer of protection.
One commonly used technique is to include a token in every form submission, which can be verified on the server-side to ensure the request is legitimate. This token is unique to each user session and can be easily generated and stored.
Another effective countermeasure is to use the Synchronizer Token Pattern, which involves generating a token on the server-side and embedding it in the form. This token is then verified on the server-side to ensure the request is legitimate.
A different take: Nextjs Form
In addition to these techniques, it's also a good idea to use HTTP-only cookies, which can help prevent CSRF attacks by making it harder for attackers to access sensitive information.
By employing these countermeasures, developers can significantly reduce the risk of CSRF attacks and ensure the security of their web applications.
Security Features
Securing API routes is crucial for building a reliable and trustworthy Next.js application. API routes are vulnerable to various security threats, including unauthorized access, data tampering, and denial-of-service (DoS) attacks.
To mitigate these threats, you can implement essential security measures, such as setting the Content-Security-Policy header to specify allowed sources for resources like scripts, styles, and images. This helps prevent cross-site scripting (XSS) attacks by blocking the execution of scripts from untrusted sources.
SameSite cookies are also an important security feature, as they restrict cookies from being sent in cross-origin requests, thereby preventing CSRF attacks. However, it's essential to note that older browsers may not fully support SameSite cookies, so you should check for browser compatibility and consider alternative CSRF protection techniques for older browsers.
On a similar theme: Protected Routes in Next Js
Same Site Cookies
Same Site Cookies are a powerful security feature that helps prevent cross-site request forgery (CSRF) attacks by restricting cookie transmission in cross-origin requests. This is achieved by setting the SameSite attribute to either "Strict" or "Lax".
By setting SameSite cookies, you can prevent attackers from automatically inserting session cookies in cross-origin requests, a technique commonly used in CSRF attacks. This is especially important for web applications that handle sensitive user data.
SameSite cookies are supported by most contemporary browsers, but older ones may not be fully compatible. So, it's essential to check for browser compatibility before implementing this security feature.
To test the effectiveness of SameSite cookies, you can use tools like Fiddler. When testing with Fiddler, you should receive an error response if the cookies are not being transmitted correctly.
Content Security Policy
Content Security Policy is a security header that allows you to specify a set of rules that define the sources from which the application can load resources.
This is designed to mitigate cross-site scripting (XSS) attacks by preventing the execution of scripts from untrusted sources.
By specifying the allowed sources for various resources, you can ensure that your application only loads resources from trusted locations.
The Content-Security-Policy header can be used to prevent the execution of scripts from untrusted sources, making it a powerful tool in the fight against XSS attacks.
By defining the allowed sources for resources like scripts, styles, and images, you can significantly reduce the risk of XSS attacks on your application.
API Security
Securing API routes is crucial for building a reliable and trustworthy Next.js application.
API routes are vulnerable to various security threats, including unauthorized access, data tampering, and denial-of-service (DoS) attacks. Securing API routes requires essential security measures to protect them from these threats.
API routes can be protected with authentication and authorization mechanisms to ensure only authorized users can access them. This can be achieved using Next.js built-in authentication features or third-party libraries.
API routes are also vulnerable to CSRF attacks, which can be mitigated by using CSRF tokens or headers to verify the authenticity of requests.
Discover more: Using State in Next Js
Protection
To implement CSRF protection in a Next.js application, you can use the Edge-CSRF library, which uses middleware to provide a layer of protection.
You'll need to install the edge-csrf library by running a command in your terminal. This library generates a token using the cookie strategy from expressjs/csurf and the crypto logic from pillarjs/csrf.
The token can be accessed from the X-CSRF-Token HTTP response header on the server-side or client-side and should be included with subsequent requests. You can extract the token from the X-CSRF-Token header and pass it as the value to a hidden input element.
To configure the CSRF middleware protection, you can pass an object with configuration options as an argument to the initialization function. This allows you to customize the default cookie set by the edge-csrf library.
CSRF attacks involve an attacker tricking a user into performing unintended actions on a web application. To protect against CSRF attacks, you can use the csurf library to generate and validate CSRF tokens.
You can use the Edge-CSRF library to generate a token and include it with subsequent requests. This helps prevent attackers from tricking users into performing unintended actions on your web application.
Take a look at this: Nextjs Authentication Middleware
Cross Site Request Forgery (CSRF)
Cross Site Request Forgery (CSRF) is a type of attack that exploits the trust between a user and a website to perform unauthorized actions on the user's behalf.
CSRF attacks can lead to serious security breaches, such as account takeover, data theft, and malware installation.
The Referer header is a component of the HTTP protocol that identifies the URL of the webpage that referred the user to the current page, but attackers can modify or remove it.
Validating the Referer header can help prevent CSRF attacks, but it should only be used in conjunction with other security measures as a backup defense mechanism.
SameSite cookies can also be used to prevent CSRF attacks by restricting cookies from being sent in cross-origin requests.
However, SameSite cookies are not supported by older browsers, so it's essential to check for browser compatibility before implementing this security measure.
CSRF attacks can be carried out with the user's credentials, making them difficult to detect and mitigate.
You might like: Next Js Header
Implementation
To implement CSRF protection in your Next.js application, you'll need to use a library like Edge-CSRF or implement it yourself.
First, let's look at using Edge-CSRF. You can install it by running the command `npm install edge-csrf` in your terminal.
To enable CSRF protection, the Edge-CSRF library generates a token using the cookie strategy from expressjs/csurf and the crypto logic from pillarjs/csrf. This token can be accessed from the X-CSRF-Token HTTP response header on the server-side or client-side and should be included with subsequent requests.
You can configure the CSRF middleware protection by passing an object with configuration options as an argument to the initialization function. This includes the ability to customize the default cookie set by the edge-csrf library, set the secret byte length, and set the salt byte length, among other things.
If you prefer to implement CSRF protection yourself, you can create a middleware.ts file in the root folder of your project. This file will contain the code that generates and validates the CSRF token.
Here's an interesting read: Middleware Next Js
Here's a step-by-step guide to implementing CSRF protection yourself:
- Create a middleware.ts file in the root folder of your project.
- Filter middleware to grab only GET requests.
- Create a secure cookie with a short expiration date with a value that is signed by the secret key only known by the server-side available environment key.
- Update the NextResponse headers with X-CSRF-Token header with the raw value.
- On the server-side of the page you want to add CSRF protection, grab the http value using headers().get('X-CSRF-Token') and pass it to the client-side component.
- On the client-side of the page, create a hidden input with the value of the X-CSRF-Token header.
Remember to update your route endpoint to include the CSRF token in the request.
Sources
- https://www.telerik.com/blogs/protecting-nextjs-applications-cross-site-request-forgery-csrf-attacks
- https://community.nodebb.org/topic/16608/invalid-csrf-token-when-authenticating-via-third-party-app
- https://www.ory.sh/nextjs-authentication-spa-custom-flows-open-source/
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
- https://www.yagiz.co/securing-your-nextjs-13-application
Featured Images: pexels.com