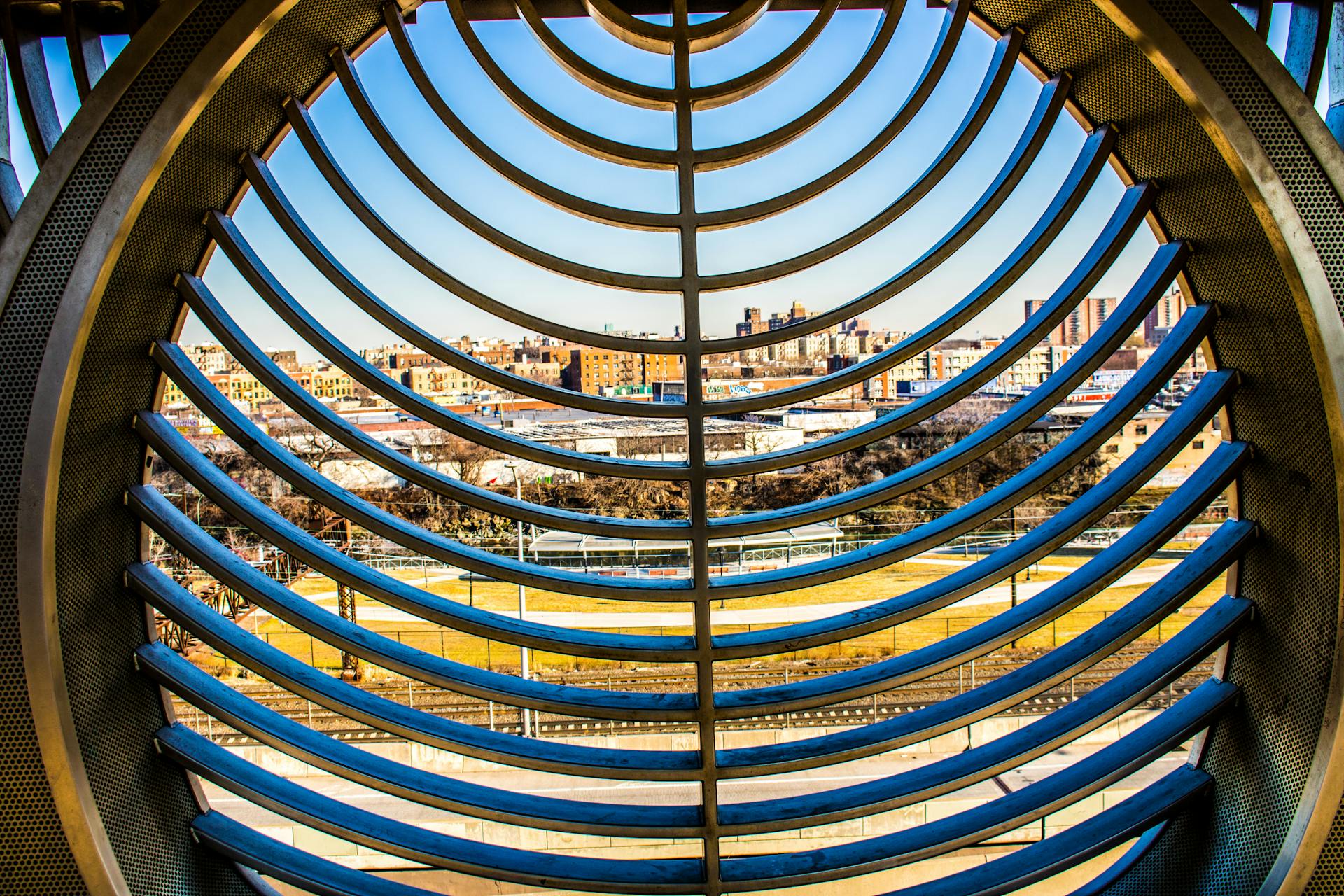
To avoid CSS grid overflow issues, it's essential to understand how the grid container and its items interact. A grid container's overflow behavior can be controlled using the overflow property.
When a grid item's content exceeds its allocated space, it can be clipped, visible, or hidden, depending on the overflow property set on the grid container. This can be particularly problematic if not handled correctly.
To prevent grid overflow, make sure to set the grid container's overflow property to 'auto' or 'scroll' to enable scrolling or clipping of excess content. This can be done using CSS code like grid-container { overflow: auto; }.
By following these best practices, you can ensure a smooth and intuitive user experience, even when dealing with large amounts of content.
Discover more: Css Grid Rows Auto
Grid Container
The grid container is a crucial concept in CSS grid layout. It's the element on which display: grid is applied.
In CSS grid layout, the grid container is the direct parent of all the grid items. It's the element that has display: grid applied, and therefore creates a new grid formatting context for the direct children.
For more insights, see: Pseudo Element Css
The grid container is essentially the container that holds all the grid items. This means that any child elements within the grid container can be positioned using grid properties like grid-column and grid-row.
In CSS grid layout, the grid container is what sets the stage for the grid system. It's the foundation upon which all the grid items are built.
For more insights, see: Css Grid Align Items
Grid Items
Grid items are the direct descendants of the grid container. They can be positioned within the grid using various properties.
The simplest way to place grid items is to use the numbered lines of the grid. These lines are based on the grid of the grid container.
The properties used to place items by line number are: grid-column-start, grid-column-end, grid-row-start, and grid-row-end. They have shorthands, such as grid-column and grid-row, which allow you to set both start and end lines at once.
To place an item, set the start and end lines of the grid area it should be placed into. Chrome DevTools can give you a visual guide to the lines in order to check where your item is placed.
For your interest: 2 Column Grid Css
The line numbering follows the writing mode and direction of the component. This means that the placement of the items stays consistent to the way that text flows.
You can use the grid-column and grid-row properties to place a grid item onto the grid. The syntax is: the first value specifies the starting line number, followed by a forward slash, followed by the second value, which specifies the end line number.
Here are the properties used to place items by line number:
- grid-column-start
- grid-column-end
- grid-row-start
- grid-row-end
They have shorthands, such as:
- grid-column
- grid-row
Grid Tracks and Gaps
Grid tracks and gaps are crucial components of CSS Grid overflow. Grid tracks are created by defining grid-template-columns and grid-template-rows properties.
Grid-template-columns property creates column tracks, and each value of this property creates a column track. For example, grid-template-columns: 200px 1fr 2fr creates three column tracks, where column 1 has a fixed width of 200px, column 2 is 1fr wide, and column 3 is 2fr wide.
Suggestion: Css Grid Template Areas
The fr unit is a flexible unit that takes up a fraction of the available space. To calculate the width of a column with an fr unit, you need to subtract all non-fr values and gaps. For instance, in the example grid-template-columns: 200px 1fr 2fr, column 1 has a fixed width of 200px, leaving 900px for the remaining columns. With 3 fractional units in total, 1fr equals 300px, making column 2 300px wide and column 3 600px wide.
Grid-template-rows property creates row tracks, and each value of this property creates a row track. For example, grid-template-rows: 100px 300px explicitly defines the first two rows, where row 1 is 100px high and row 2 is 300px high.
Grid gaps can be specified using the column-gap, row-gap, grid-column-gap, or grid-row-gap properties. These properties set the size of the grid lines, which can be thought of as the width of the gutters between the columns/rows. The gutters are only created between the columns/rows, not on the outer edges.
Here's a summary of the grid gap properties:
- column-gap and row-gap specify the size of the grid lines between columns and rows, respectively.
- grid-column-gap and grid-row-gap are deprecated and will be removed in favor of column-gap and row-gap.
Grid Units and Sizing
Fractional units in CSS Grid, like 1fr, essentially mean "portion of the remaining space". They're much more flexible than percentage values, which can become rigid when combined with other units like padding.
Fractional units are calculated by subtracting all non-fr values and gaps, then dividing the remaining space among the fractional units. For example, if you have three fractional units with a total remaining space of 900px, each 1fr unit would equal 300px.
Intrinsic sizing keywords, like min-content, max-content, and fit-content(), provide additional methods of sizing boxes in CSS. The min-content keyword makes a track as small as it can be without the track content overflowing, while max-content makes it as wide as the longest word in the track.
Here's a quick rundown of the intrinsic sizing keywords:
- min-content: makes a track as small as it can be without content overflowing
- max-content: makes a track as wide as the longest word in the track
- fit-content(): makes a track wrap its content once it reaches a specified size
The minmax() function allows you to set a minimum and maximum size for a track, which can be useful for distributing remaining space among tracks. For example, using minmax(auto, 1fr) will give the content enough room, then distribute the available space.
You might like: Css Grid Extra Space
Auto-Fill and Auto-Fit
Auto-Fill and Auto-Fit allow you to create responsive grid layouts without media queries.
You can use the auto-fill or auto-fit keywords to create as many tracks as will fit in your container, while also allowing for flexible tracks that can grow or shrink as needed.
In the demo, you can see how the grid changes as you change the size of the viewport, and how the tracks are not flexible, resulting in a gap on the end until there is enough space for another 200 pixel track.
With the minmax() function, you can request as many tracks as will fit with a minimum size of 200 pixels and a maximum of 1fr, creating a two-dimensional responsive layout.
There is a subtle difference between auto-fill and auto-fit. The auto-fill keyword creates empty tracks, while the auto-fit keyword collapses the tracks down to 0 size, allowing the flexible tracks to grow to consume the space.
Intriguing read: Css Grid Space between
Here are the differences between auto-fill and auto-fit:
In the end, the choice between auto-fill and auto-fit depends on the desired behavior, and both keywords otherwise act in exactly the same way once the first track is filled.
Worth a look: Css Grid Auto-fit
Fr Units
Fr Units are a flexible way to size grid tracks, taking up a portion of the remaining space. They're represented by the 'fr' unit, which is short for fractional unit.
You can use multiple 'fr' units in a single grid template to distribute space proportionally. For example, 1fr and 2fr will share the remaining space in a ratio of 1:2.
The 'fr' unit works similarly to flex: auto in flexbox, distributing space after the items have been laid out. This means you can use it to create columns that all get the same share of available space.
To calculate the size of an 'fr' unit, you subtract all non-'fr' values and gaps from the available space. Then, you divide the remaining space by the total number of 'fr' units to determine the size of each unit.
Consider reading: Css Grid Fr
For instance, if you have three columns with a fixed width of 200px, and two 'fr' units, the remaining space would be 900px. Dividing 900px by 3 gives you 300px, which is the size of each 'fr' unit.
You can combine 'fr' units with fixed sizes to create more complex grid layouts. For example, 200px 1fr will create a column with a fixed width of 200px, and the second column will take up the remaining space.
The 'fr' unit is a powerful tool for creating responsive grid layouts that adapt to different screen sizes. By using 'fr' units, you can create columns that resize dynamically based on the available space.
You might like: Css Grid Column Width
Grid Placement and Ordering
CSS Grid Layout is based on a grid of numbered lines, making it easy to place items onto the grid. You can use these lines to position items from one line to another.
The properties grid-column-start, grid-column-end, grid-row-start, and grid-row-end allow you to place items by line number. There are also shorthands, grid-column and grid-row, which enable you to set both start and end lines at once.
If this caught your attention, see: Grid Row Css
You can use Chrome DevTools to get a visual guide to the lines and check where your item is placed. The line numbering follows the writing mode and direction of the component.
To place an item, set the start and end lines of the grid area where it should be placed. This will create an explicit grid, which is a grid that you have defined and given size to the tracks.
Grid lines mark the start or end of a column or row track, with the count starting at 1. You can use these line numbers to place a grid item onto the grid.
Here's a breakdown of the grid placement properties:
You can also use the "span" keyword to place an item, which tells the grid layout how many columns or rows the item should span.
If this caught your attention, see: Css Grid Colspan
Grid Shorthand Properties
Grid shorthand properties can be a bit confusing at first, but they're actually quite useful. They allow you to set many grid properties in one go, which can save you time and make your code more concise.
Worth a look: Css Grid Properties
You can use these properties to set the grid template, grid columns, and grid rows all at once. For example, you can use the grid-template-rows property to set the number of rows and their sizes in a single line of code.
Whether you prefer to use shorthand properties or longhand properties is up to you, but it's worth noting that shorthand properties can make your code more efficient and easier to read.
A different take: Grid Col Span Css
Shorthand Properties
Shorthand properties can look a little confusing, but they're actually a convenient way to set multiple grid properties at once.
There are two shorthand properties that allow you to do this. These properties can be a bit overwhelming at first, but breaking down how they work together can help clarify things.
You can use them or stick to longhands, it's up to you.
Auto-Fill Keywords
You can use the auto-fill keyword to create as many tracks as will fit in your container, but keep in mind that the tracks are not flexible.
The auto-fill keyword is useful for creating a two-dimensional responsive layout, but it does leave a gap on the end until there is enough space for another track.
There's a subtle difference between auto-fill and auto-fit, with auto-fill creating empty tracks when there aren't enough items to fill them.
Auto-fill and auto-fit keywords otherwise act in exactly the same way once the first track is filled.
To create a responsive grid layout without media queries, you can use the auto-fill or auto-fit keyword with the repeat() function, like this: grid-template-columns: repeat(auto-fill, minmax(200px, 1fr)).
Auto-fill expands grid items to fill the available space, while auto-fit does not.
Intriguing read: Css Grid Repeat
Grid Functionality and Limits
Grid functionality is determined by the grid-template-areas property, which defines the grid's layout and structure.
The grid is divided into tracks, which are the rows and columns that make up the grid. The grid-template-columns property defines the number and size of these tracks.
The grid's overflow behavior is influenced by the grid-template-rows property, which specifies the size and number of grid rows.
Grid tracks can be fixed, auto, or fractional, and their size is determined by the grid-template-columns and grid-template-rows properties.
The grid's overflow can be hidden, visible, or clipped, depending on the grid-template-areas property and the size of the grid's content.
A unique perspective: Css Text Ellipsis
Grid Construction and Setup
CSS Grid will create a single-column layout by default, but we can specify columns using the grid-template-columns property.
To define columns, pass two or more values to grid-template-columns, such as 25% and 75%, to slice the element up into multiple columns.
Columns can be defined using any valid CSS length-percentage value, including pixels, rems, or viewport units.
The fr unit, short for "fraction", allows columns to be flexible and grow or shrink as required to contain their contents.
Percentage-based columns are rigid and won't shrink below their minimum content size, while fr-based columns will adapt to their contents.
Here's a comparison of percentage-based and fr-based columns:
The fr unit distributes extra space between columns, first calculating column widths based on their contents, and then distributing any leftover space based on the fr values.
This is similar to flex-grow, where extra space is distributed among flex items based on their grow factor.
You might enjoy: Flex Grid Css
Grid Concepts and Terminology
Grid is a new layout system in CSS, and it comes with its own set of terminology.
Grid terminology can be overwhelming at first, but it's actually quite straightforward. Grid has a real layout system, which is a big deal for CSS.
Some key terms to know include grid, which is the container that holds the layout. Grid containers are created using the grid-template-areas property.
Grid items are the content that's placed inside the grid container. They can be images, text, or other HTML elements. Grid items can be placed inside the grid container using the grid-column and grid-row properties.
Grid tracks are the rows and columns that make up the grid. They're created using the grid-template-columns and grid-template-rows properties.
A fresh viewpoint: Tailwind Css Layout
Frequently Asked Questions
How do I prevent content overflow in CSS Grid?
To prevent content overflow in CSS Grid, use the auto-fit(minmax(
Featured Images: pexels.com