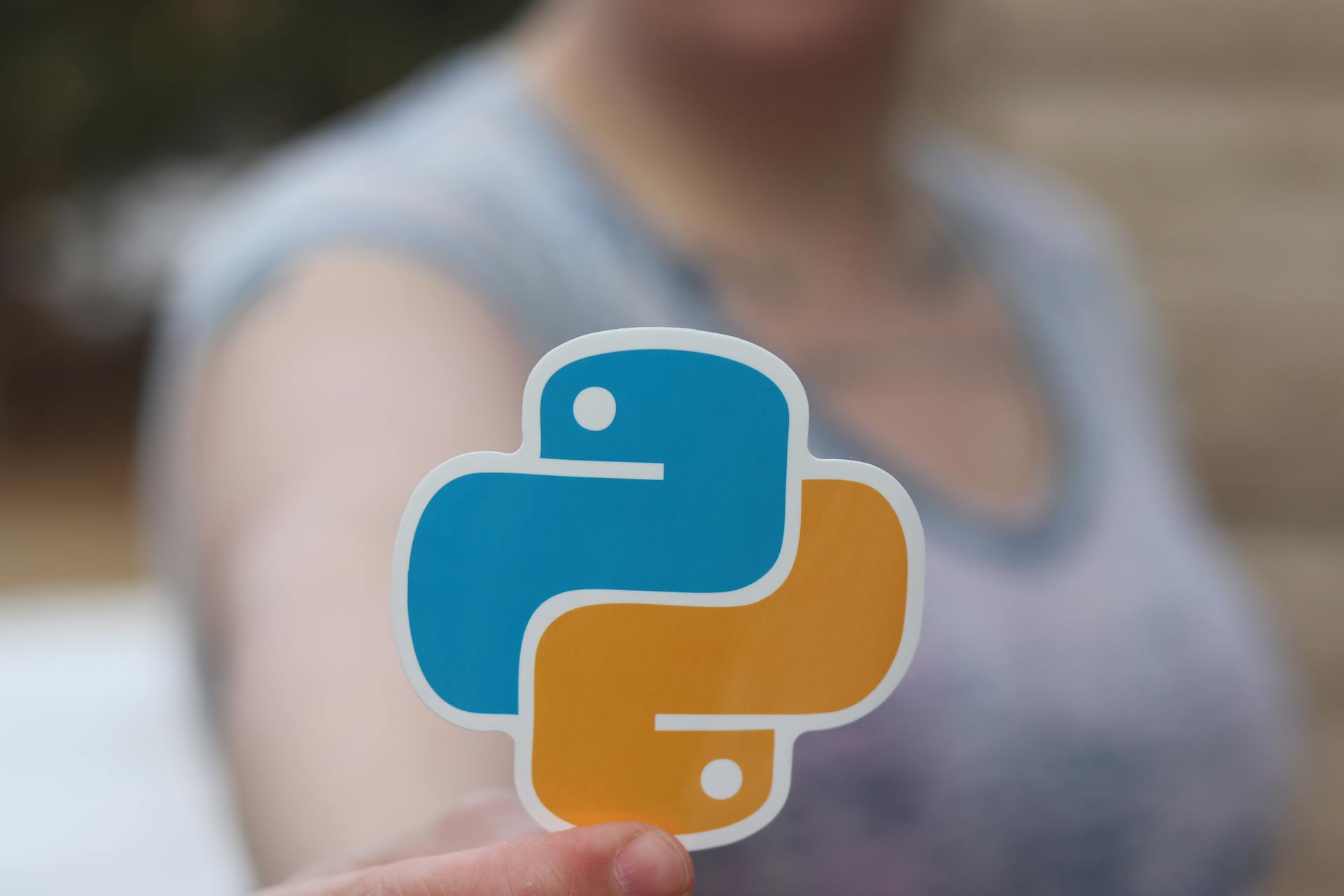
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It's perfect for building robust and scalable web applications.
In this tutorial, we'll take you from scratch to deployment, covering the basics of Django and its ecosystem. You'll learn how to set up a new project, create models, views, and templates, and deploy your application to a production environment.
Django's batteries-included approach means you don't need to look for third-party libraries to get started. The framework provides an ORM (Object-Relational Mapping) system that abstracts the underlying database, allowing you to focus on writing code.
With Django, you can build complex web applications quickly, thanks to its modular design and extensive libraries.
Suggestion: Web Page Design Tutorial
Project Setup
To set up a project in Django, start by creating a virtual environment in your project folder. You can do this by running a command in your terminal, such as `python3 -m venv .venv` on Linux or macOS, or `py -3 -m venv .venv` on Windows.
Create a new directory for your project, and then navigate to it in your terminal. You can then activate the virtual environment by running the command `source .venv/bin/activate` on Linux or macOS, or `.venv\Scripts\Activate.ps1` on Windows.
Once your virtual environment is set up, you can install Django by running the command `python -m pip install django`. You can then create a new Django project by running the command `django-admin startproject web_project .`, which creates a new directory with your project name and sets up a basic Django project structure within it.
Check this out: Windows Azure Active Directory Tutorial
A Project Environment
Creating a project environment is a crucial step in setting up your Django project. This involves creating a virtual environment where Django is installed, which helps avoid installing it in a global Python environment and gives you control over the libraries used in your application.
You can create a virtual environment using the `venv` module, which is included in Python 3.3 and later versions. To do this, navigate to your project folder and run the command `python3 -m venv .venv` (on Linux or macOS) or `py -3 -m venv .venv` (on Windows).
Once you've created the virtual environment, you can activate it by running the command `source .venv/bin/activate` (on Linux or macOS) or `.venv\Scripts\Activate.ps1` (on Windows).
Here are the steps to create a project environment:
- Create a new virtual environment using `venv`.
- Activate the virtual environment using `source` or `.venv\Scripts\Activate.ps1`.
- Update pip in the virtual environment by running `python -m pip install --upgrade pip`.
- Install Django in the virtual environment by running `python -m pip install django`.
By following these steps, you can create a self-contained environment for your Django project that's isolated from the global Python environment. This helps ensure that your project works as expected and avoids conflicts with other projects or libraries.
Collectstatic Command
In Django, you typically collect all static files from your apps into a single folder using the collectstatic command.
To do this, you need to add a line to your web_project/settings.py file that defines a location where static files are collected. This line should be STATIC_ROOT = BASE_DIR /'static_collected'.
Running the command python manage.py collectstatic copies your static files into the top level static_collected folder.
You should run collectstatic any time you change static files and before deploying into production. This ensures that your static files are up to date and ready to be served by a dedicated static file server.
Templates and Styles
In Django, templates are used to separate presentation logic from application logic. To refer to static files in a template, you need to create a static folder in your app directory, with a subfolder matching your app name. This is because when you deploy your project to a production server, you collect all static files into a single folder served by a dedicated static file server.
A base page template in Django contains shared parts of a set of pages, including references to CSS files and script files. This template defines one or more block tags with content that extended templates are expected to override. For example, the `layout.html` file defines blocks named "title" and "content".
To include styles in your templates, you can add CSS files to your static folder. For instance, the `site.css` file in the `static/hello` folder contains styles for the "message" class, which is used to render text in blue.
Here's an interesting read: Css Stylesheet Tutorial
Refer to Static Files in Templates
To refer to static files in a template, you need to create a static folder in your app's directory. This folder should be named after your app, which in this case is "hello". Within the static folder, create a subfolder named "hello" to ensure your static files don't collide with files from other apps in the project.
You'll also need to create a CSS file, such as "site.css", and add it to the static/hello folder. In your template, use the {% load static %} tag to load the static files, and then refer to the CSS file using the {% static %} tag.
Here's a step-by-step guide to setting up static files in your template:
1. Create a static folder in your app's directory and name it after your app.
2. Within the static folder, create a subfolder named after your app.
3. Add a CSS file, such as "site.css", to the static/hello folder.
Consider reading: Most Popular Css Frameworks
4. In your template, add the {% load static %} tag to load the static files.
5. Refer to the CSS file using the {% static %} tag, like this: {% static 'hello/site.css' %}
By following these steps, you can easily refer to static files in your templates and style your web pages with CSS.
You might like: Learn Tailwind Css
Debugger with Templates
You can debug page templates with the Python Extension for VS Code. To do this, you need to have "django": true in the debugging configuration.
The Python Extension for VS Code provides template debugging when you have "django": true in the debugging configuration. You can set breakpoints on both the {% if message_list %} and {% for message in message_list %} lines in your templates.
To debug templates, follow these steps: set breakpoints on both the {% if message_list %} and {% for message in message_list %} lines, run the app in the debugger, and open a browser to the home page. The debugger will break into the debugger in the template on the {% if %} statement and show all the context variables in the Variables pane.
You can step through the template code using the Step Over (F10) command. This will let you examine each value in message and step to lines like{{ message.log_date | date:'d M Y' }}. You can also work with variables in the Debug Console panel.
Here are the steps to set up template debugging:
1. Set breakpoints on both the {% if message_list %} and {% for message in message_list %} lines.
2. Run the app in the debugger and open a browser to the home page.
3. Use the Step Over (F10) command to step through the template code.
4. Work with variables in the Debug Console panel.
5. Select Continue (F5) to finish running the app and view the rendered page in the browser.
Database and Models
Django's default database is SQLite, but it's not recommended for high-traffic sites or multi-server scenarios. Instead, consider using production-level data stores like PostgreSQL, MySQL, and SQL Server.
For development work, Django includes a db.sqlite3 file that's suitable for low to medium traffic sites with fewer than 100 K hits/day. However, for larger projects, you'll need to switch to a more robust database.
To define models in Django, you'll create Python classes in the app's models.py file, derived from django.db.models.Model. Each model will have a unique ID field named id and other fields defined using types from django.db.models. For example, you can use CharField for limited text, TextField for unlimited text, and EmailField for email addresses.
Broaden your view: Azure Cosmos Db Tutorial
Types of Databases
Django comes with a default database called SQLite, which is great for development work.
SQLite works fine for low to medium traffic sites with fewer than 100 K hits/day.
However, it's not recommended for higher volumes and is limited to a single computer.
For production-level work, consider using a data store like PostgreSQL, MySQL, or SQL Server.
These databases are designed to handle higher traffic and can be used in multi-server scenarios like load-balancing and geo-replication.
You can also use the Azure SDK for Python to work with Azure storage services like tables and blobs.
Database Through Models
A Django model is a Python class derived from django.db.models.Model, which represents a specific database object, typically a table. You place these classes in an app's models.py file.
To create a model, you define class variables represented by instances of field classes, such as CharField, DateField, and IntegerField. For example, the ToDoItem model has two class variables: text and due_date.
You work with your database almost exclusively through the models you define in code. Django's migrations handle all the details of the underlying database automatically as you evolve the models over time.
Here's a general workflow:
- Make changes to the models in your models.py file.
- Run python manage.py makemigrations to generate scripts in the migrations folder that migrate the database from its current state to the new state.
- Run python manage.py migrate to apply the scripts to the actual database.
Migrations are Django's way of propagating changes in your models into the database schema. They are designed to be mostly automatic, but you'll need to know when to make migrations and when to run them.
Here's a step-by-step guide to handling migrations:
- Understanding Migrations: Migrations are Django’s way of propagating changes in your models (like adding a new field, deleting a model, etc.) into the database schema.
- Making Migrations: Once you have defined or updated your models, you need to tell Django to create migrations for these changes.
- Review Migration Scripts: Before applying the migrations, it’s a good practice to review the migration scripts that Django has generated.
- Apply Migrations: To apply the migrations to your database, run: python manage.py migrate
- Migration Dependencies: Sometimes, your migrations depend on each other, especially when you’re working with multiple apps.
- Rolling Back Migrations: If you need to undo a migration, you can use the migrate command with the name of the app and the migration you want to revert to.
Views and URLs
Views are functions or classes that specify how web requests are processed and which web responses are returned. In Django, views are defined in the app's directory, specifically in the views.py file.
By convention, views are defined in the app's directory, specifically in the views.py file. You can define views as functions or classes, and they can inherit from Django's built-in view classes, such as ListView.
To configure views, you need to map them to URLs using the URL dispatcher. The URL dispatcher is used to direct HTTP requests to the appropriate view based on the request URL.
If this caught your attention, see: Google Cloud Functions Framework
Here are the standard actions in a ViewSet:
- list: handles GET requests to the root of the URL, returning a list of all instances.
- create: handles POST requests, allowing clients to create a new instance of a model.
- retrieve: handles GET requests to a specific instance, returning that particular instance.
- update: handles PUT requests, used to update an existing model instance.
- destroy: handles DELETE requests, allowing clients to delete an existing instance.
Custom actions can be added to ViewSets using the @action decorator, allowing you to add custom logic to your views.
Configure URL Routing
Configuring URL routing is a crucial step in building a Django REST API. In Django, the URL dispatcher is used to direct HTTP requests to the appropriate view based on the request URL.
You can configure URL routing by using a URLconf, which is a set of patterns that Django tries to match the requested URL to find the right view. In your Django project's urls.py file, you will include the URL patterns for your app.
To set up URL patterns, you can use a DefaultRouter, which automatically generates URL patterns for the standard actions in your viewsets. For example, if you have a BlogPostViewSet, the router will generate URL patterns for listing all blog posts, retrieving a single blog post, creating a new blog post, etc.
Here's an example of how to set up a DefaultRouter:
```python
from django.urls import include, path
from rest_framework.routers import DefaultRouter
from myapp.views import BlogPostViewSet
router = DefaultRouter()
router.register(r'blogposts', BlogPostViewSet)
urlpatterns = [
path('', include(router.urls)),
]
```
In this example, the router registers the BlogPostViewSet and generates URL patterns for the standard actions.
If you have custom actions in your viewsets, the router will also generate appropriate URL patterns for these actions. For example, if you have a custom action called "like" in your BlogPostViewSet, the router will generate a URL pattern for this action.
Here's an example of how to use a router with custom actions:
```python
from django.urls import include, path
from rest_framework.routers import DefaultRouter
from myapp.views import BlogPostViewSet
router = DefaultRouter()
router.register(r'blogposts', BlogPostViewSet)
urlpatterns = [
path('', include(router.urls)),
]
```
In this example, the router registers the BlogPostViewSet and generates URL patterns for the standard actions and the custom "like" action.
To test your URLs, you can use Django's shell and test framework. You can also use a tool like curl to test your URLs from the command line.
By configuring your URL routing properly, you ensure that your Django REST API is well-structured and that each endpoint is correctly mapped to its corresponding view. This step is crucial for the functionality of your API, as it defines how clients interact with your application and access its resources.
Here's a summary of the key points to keep in mind when configuring URL routing:
- Use a URLconf to define the URL patterns for your app
- Use a DefaultRouter to automatically generate URL patterns for the standard actions in your viewsets
- Use the router to generate URL patterns for custom actions in your viewsets
- Test your URLs using Django's shell and test framework or a tool like curl
Serve Static Files
Serving static files in Django is a crucial step in making your web app visually appealing and functional. Static files are pieces of content that your web app returns as-is for certain requests, such as CSS files.
The INSTALLED_APPS list in settings.py needs to contain django.contrib.staticfiles for serving static files. This is included by default, so you don't need to add it manually.
To serve static files in a production server like Gunicorn, a simple approach is to use the Django development server. However, for a full treatment of static files, you should check the Django documentation.
To get started with serving static files, you need to ready your app. This involves adding an import statement and a line of code to your project's web_project/urls.py file.
Here are the steps to ready your app:
- Import the staticfiles_urlpatterns function from django.contrib.staticfiles.urls
- Add the staticfiles_urlpatterns() function to the end of your url patterns list
Once you've added the staticfiles_urlpatterns() function, you can refer to static files in your templates. This involves creating a static folder and a subfolder named after your app.
Here's a step-by-step guide to referring to static files in a template:
- Create a static folder in your app's directory
- Create a subfolder named after your app inside the static folder
- Inside the subfolder, create a CSS file with the desired styles
- Load the static tag in your template and link to the CSS file
- Use the message style in your template to render the content in blue
Running Migrations
Running migrations is a crucial step in keeping your database schema in sync with your Django models. You can run migrations in PyCharm by using the manage.py utility.
To start, you need to type makemigrations followed by Enter in the terminal tab. This will create a migration file in the todo/migrations directory, specifically 0001_initial.py.
Once you've created the migration file, you can apply the changes to your database by typing migrate and pressing Enter. This will create tables in the database for the two new models.
Recommended read: Create Responsive Website Tutorial
API and Authentication
Implementing authentication and permissions is a critical step in building a robust Django REST API. It ensures that only authenticated users can access certain endpoints and perform actions they’re permitted to.
Django REST Framework supports various authentication schemes, including Basic Authentication, Token Authentication, and OAuth. To set up Token Authentication, you need to add 'rest_framework.authtoken' to your INSTALLED_APPS and run python manage.py migrate to create the necessary database tables.
To implement permissions, you can set permission classes in your views to control access. Django REST Framework provides built-in permission classes like IsAuthenticated, IsAdminUser, and IsAuthenticatedOrReadOnly. For example, you can set permission_classes=[IsAuthenticated] in your view to ensure that only authenticated users can access the endpoints.
Here are some key points to consider:
- Choose an authentication scheme and configure it in your Django settings.
- Set up permissions in your views to control access.
- Customize permission classes if the built-in ones don't fit your needs.
- Test your API's authentication and permissions to ensure they're working as expected.
Creating API Views
Creating API views is a crucial step in building a robust and efficient API. Django REST Framework's ViewSets provide a high-level abstraction over traditional Django views and are particularly suited for standard database operations.
Related reading: Django Rest Api Framework
To create a ViewSet, you can create a class that inherits from django.rest_framework.viewsets.ModelViewSet. This class will automatically provide list, create, retrieve, update, and destroy actions.
For example, if you have a BlogPost model and a corresponding BlogPostSerializer, your ViewSet might look like this: class BlogPostViewSet(viewsets.ModelViewSet): queryset = BlogPost.objects.all() serializer_class = BlogPostSerializer
Handling GET requests is also an important part of creating API views. The list action in a ViewSet handles GET requests to the root of the URL, returning a list of all instances. The retrieve action handles GET requests to a specific instance, like /api/blogposts/1/, returning that particular instance.
Here's a summary of the actions provided by a ViewSet:
- List: Handles GET requests to the root of the URL, returning a list of all instances.
- Create: Handles POST requests, allowing clients to create a new instance of a model.
- Retrieve: Handles GET requests to a specific instance, returning that particular instance.
- Update: Handles PUT requests, updating an existing model instance.
- Destroy: Handles DELETE requests, deleting an existing instance.
You can customize the behavior of a ViewSet by overriding action methods like create(), update(), or destroy(). You can also add custom actions using the @action decorator.
Implement Authentication and Permissions
Implementing authentication and permissions is a critical step in building a robust Django REST API. This ensures that only authenticated users can access certain endpoints, and users can only perform actions they're permitted to.
Django REST Framework supports various authentication schemes like Basic Authentication, Token Authentication, and OAuth. To set up authentication, you need to choose an authentication scheme and configure it in your Django settings.
To implement permissions, you can set permission classes to control access in your views. Django REST Framework provides a set of built-in permission classes like IsAuthenticated, IsAdminUser, and IsAuthenticatedOrReadOnly. For example, you can use IsAuthenticated to ensure that only authenticated users can access the endpoints defined in a viewset.
A custom permission class is a Python class that extends rest_framework.permissions.BasePermission and overrides the .has_permission() and/or .has_object_permission() methods. If the built-in permissions don't fit your needs, you can define custom permission classes to tailor your API's access control.
To secure sensitive information, ensure that tokens and credentials are transmitted securely, preferably over HTTPS. Be mindful of storing sensitive data on the client side.
Here are the steps to implement authentication and permissions effectively:
- Understanding Authentication and Permissions: Determine the identity of a user and control access to different parts of your API.
- Setting Up Authentication: Choose an authentication scheme and configure it in your Django settings.
- Implementing Permissions: Set permission classes to control access in your views.
- Custom Permissions: Define custom permission classes if the built-in permissions don't fit your needs.
- Token Generation and Handling: Generate tokens for token-based authentication and handle them securely.
- Securing Sensitive Information: Transmit tokens and credentials securely over HTTPS.
- Testing Authentication and Permissions: Write tests to check if the API responds correctly to authenticated and unauthenticated requests.
Sources
- https://code.visualstudio.com/docs/python/tutorial-django
- https://www.jetbrains.com/help/pycharm/creating-and-running-your-first-django-project.html
- https://www.moesif.com/blog/technical/api-development/Django-REST-API-Tutorial/
- https://www.djangoproject.com/start/
- https://www.geeksforgeeks.org/django-tutorial/
Featured Images: pexels.com