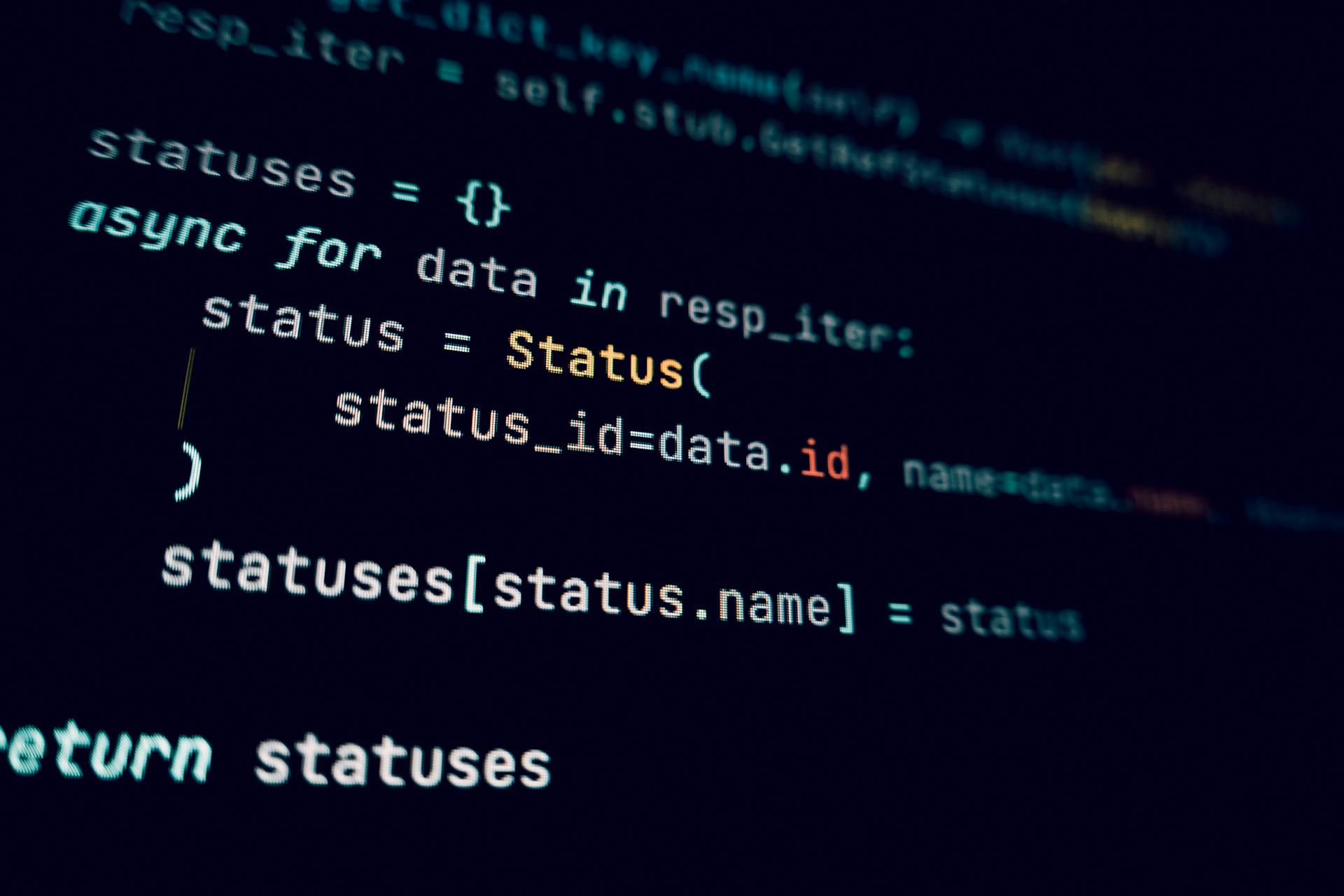
Developing a Django Rest API framework involves several key steps. You should start by creating a new Django project and a new Django app within it.
To begin, run the command `django-admin startproject myproject` to create a new Django project, and then navigate into the project directory with `cd myproject`. Next, create a new Django app with `python manage.py startapp myapp`.
Django comes with a built-in authentication system that can be used to create user accounts and manage user sessions. This system is based on the built-in User model, which is a subclass of AbstractBaseUser.
To enable authentication in your Django project, you need to add `'django.contrib.auth'` to the `INSTALLED_APPS` setting in your `settings.py` file.
Curious to learn more? Check out: Nextjs Api Router
Setting Up
First, create a virtual environment to isolate dependencies, ideally in your project's folder. You can do this by running the command python -m venv django_env.
To activate the virtual environment, run source ./django_env/bin/activate, which will turn it on.
You'll know the virtual environment is on because its name will become part of the shell prompt.
Navigate to an empty folder in your terminal and install Django and Django REST framework with the commands below.
Install Django and Django REST framework with the commands below: pip install django and pip install djangorestframework.
Create a new app for your API by running python manage.py startapp todo.
Run your initial migrations of the built-in user model with the command python manage.py migrate.
Add rest_framework and todo to the INSTALLED_APPS inside the todo/todo/settings.py file.
Include rest_framework and URLs as shown below in your main urls.py file: from django.urls import path, include from rest_framework import routers from rest_framework.authtoken.views import obtain_auth_token.
Consider reading: Building Python Web Apis with Fastapi
Serializers
Serializers are a crucial part of Django REST API framework, and they're used to convert complex data such as querysets and model instances into native Python datatypes that can be easily rendered into JSON, XML, or other content types.
Serializers provide deserialization as well, allowing parsed data to be converted back into complex types after first validating the incoming data. This process is essential for any API, and Django REST framework can take it over completely.
To create a serializer, you can use the ModelSerializer class, which is provided by Django REST framework. This class allows you to generate a serializer for any model in just two lines of code.
Here's a step-by-step guide to creating a serializer:
1. Import the serializer from rest_framework
2. Import the model from your models.py file
3. Create a model serializer class that inherits from serializers.ModelSerializer
4. Specify the model and fields to be serialized in the class Meta
Here's an example of how to create a serializer for the Offer model:
```
class OfferSerializer(serializers.ModelSerializer):
class Meta:
model = Offer
fields = ['id', 'address', 'size', 'type', 'price', 'sharing', 'text']
```
In this example, we're specifying the Offer model as the base model and the list of fields to be serialized.
Serializers can be used to "translate" Django model instances or querysets into other formats, usually JSON or XML, so that they can be sent in the body of an HTTP response.
Readers also liked: Azure Data Factory Framework
Here's a summary of the key points to remember when working with serializers:
- Serializers are used to convert complex data into native Python datatypes
- ModelSerializer class is used to generate a serializer for any model
- Specify the model and fields to be serialized in the class Meta
- Serializers provide deserialization as well
By following these steps and using the ModelSerializer class, you can easily create serializers for your Django models and use them to interact with your API.
Views
Views are a crucial part of Django REST API framework, allowing you to handle HTTP requests and return responses. They can be function-based or class-based.
In function-based views, you define a function that takes a request object as an argument and returns a response object. For example, in the rental/views.py file, you can see a function-based view called offer_list that handles GET and POST requests. It fetches all offers, serializes them, and returns the response.
Function-based views are useful for simple APIs, but for more complex APIs, class-based views are preferred. Class-based views are defined as classes that inherit from Django's built-in view classes, such as View or APIView.
In class-based views, you define methods for handling different HTTP methods. For example, in the Detail view, you can see a class-based view that handles GET, PUT, and DELETE requests. It fetches the object, serializes it, and returns the response.
Worth a look: Nextjs View Transition Api
Here are some key differences between function-based and class-based views:
Class-based views are more flexible and scalable, making them a better choice for complex APIs. However, they can be more complex to implement, especially for beginners.
In addition to function-based and class-based views, you can also use viewsets, which are a set of views that provide a full CRUD (Create, Read, Update, Delete) set of operations. Viewsets are defined as classes that inherit from Django's built-in viewset classes, such as ModelViewSet.
Viewsets are useful for creating RESTful APIs that provide a full CRUD set of operations. They can be configured to handle different HTTP methods and provide a flexible way to handle requests and return responses.
In summary, views are a crucial part of Django REST API framework, and you can choose between function-based, class-based, and viewset-based views depending on your API's complexity and requirements.
See what others are reading: Why Are Apis Important
App Model Creation
Creating models for your Django app is a crucial step in building a robust API.
In Django, models are essentially classes that define the structure of your database tables. You can create models using the Django ORM (Object-Relational Mapping) system.
To create a model, you need to define its fields, which are the columns in your database table.
For example, if you're building a blog API, you might create a model for the Post class with fields like title, content, and author.
Creating models for your Django app is a crucial step in building a robust API.
In Django, models are essentially classes that define the structure of your database tables.
You can create models using the Django ORM system, which allows you to interact with your database using Python code.
To create a model, you need to define its fields, which are the columns in your database table.
For instance, in the example above, the Post class has fields like title, content, and author.
You can use these fields to create a model that represents your data and interacts with your database.
Creating an endpoint for your class-based view is also an important step in building a robust API.
You might like: Creating Restful Webservices in Java
You can create an endpoint by defining a URL pattern that maps to your view function.
For example, if you have a class-based view like the one in the example above, you can create an endpoint for it.
This is done by creating a URL pattern that maps to the view's as_view() method.
So, to summarize, creating models for your Django app involves defining classes that represent your database tables and defining fields that represent the columns in those tables.
Authentication and Permissions
Authentication and Permissions are crucial components of any Django REST API framework. You can implement authentication and permissions in your Django apps in multiple ways, including using the Simple JWT package or djoser.
To install the packages, you can run the following command: `pip install djangorestframework simplejwt djoser`. Then, you need to configure your Django project settings by adding djoser to INSTALLED_APPS and configuring the REST_FRAMEWORK settings.
For example, you can add the following code to your settings.py file:
Additional reading: Install Django Project
```python
INSTALLED_APPS = [
...
'djoser',
...
]
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': (
'rest_framework_simplejwt.authentication.JWTAuthentication',
),
}
```
You can also use a custom permission class to restrict access to certain views. For instance, you can create a class that inherits from rest_framework.permissions.BasePermission and overrides the has_object_permission method to check if the requesting user is the author of the object.
Here's an example of a custom permission class:
```python
class IsAuthorOrReadOnly(permissions.BasePermission):
def has_object_permission(self, request, view, obj):
return request.method in permissions.SAFE_METHODS or obj.author == request.user
```
This permission class will grant permission to read-only methods (GET, HEAD, OPTIONS) and will also grant permission to the author of the object to update it.
To enable authentication and permissions in your views, you can add the permission_classes attribute to the view class. For example:
```python
class OfferList(generics.ListCreateAPIView):
queryset = Offer.objects.all()
serializer_class = OfferSerializer
permission_classes = [permissions.IsAuthenticatedOrReadOnly]
def perform_create(self, serializer):
serializer.save(author=self.request.user)
```
This view will require authentication for create and update operations, but will allow anyone to read the list of offers.
Intriguing read: Django Rest Framework Serializer
Here's a summary of the key points to keep in mind when implementing authentication and permissions in your Django REST API framework:
By following these guidelines and using the examples provided, you can implement robust authentication and permissions in your Django REST API framework.
Frequently Asked Questions
Is Django good for REST API?
Yes, Django is a great choice for building REST APIs, thanks to its robustness and ease of use. It's the de facto standard for building APIs with Django, making it a popular and reliable option.
What is API in Django rest framework?
An API in Django Rest Framework is a software interface that enables different applications to communicate with each other, allowing for data exchange and functionality sharing. In this context, we'll explore how to create a Django REST API to manage user data.
Is Django a rest framework?
Django REST framework is a toolkit for building Web APIs, not the Django framework itself. It's a powerful tool that helps developers create robust and user-friendly APIs.
Are Django and Django Rest Framework different?
Yes, Django and Django Rest Framework are different, with Django being a Python web development framework and Django Rest Framework being a library that helps build REST APIs within Django. This distinction makes Django a broader platform for web development, while Django Rest Framework is a specialized tool for API design.
Sources
- https://tests4geeks.com/blog/django-rest-framework-tutorial/
- https://blog.logrocket.com/django-rest-framework-create-api/
- https://www.geeksforgeeks.org/how-to-create-a-basic-api-using-django-rest-framework/
- https://djangopackages.org/grids/g/rest/
- https://www.jetbrains.com/help/pycharm/building-apis-with-django-rest-framework.html
Featured Images: pexels.com