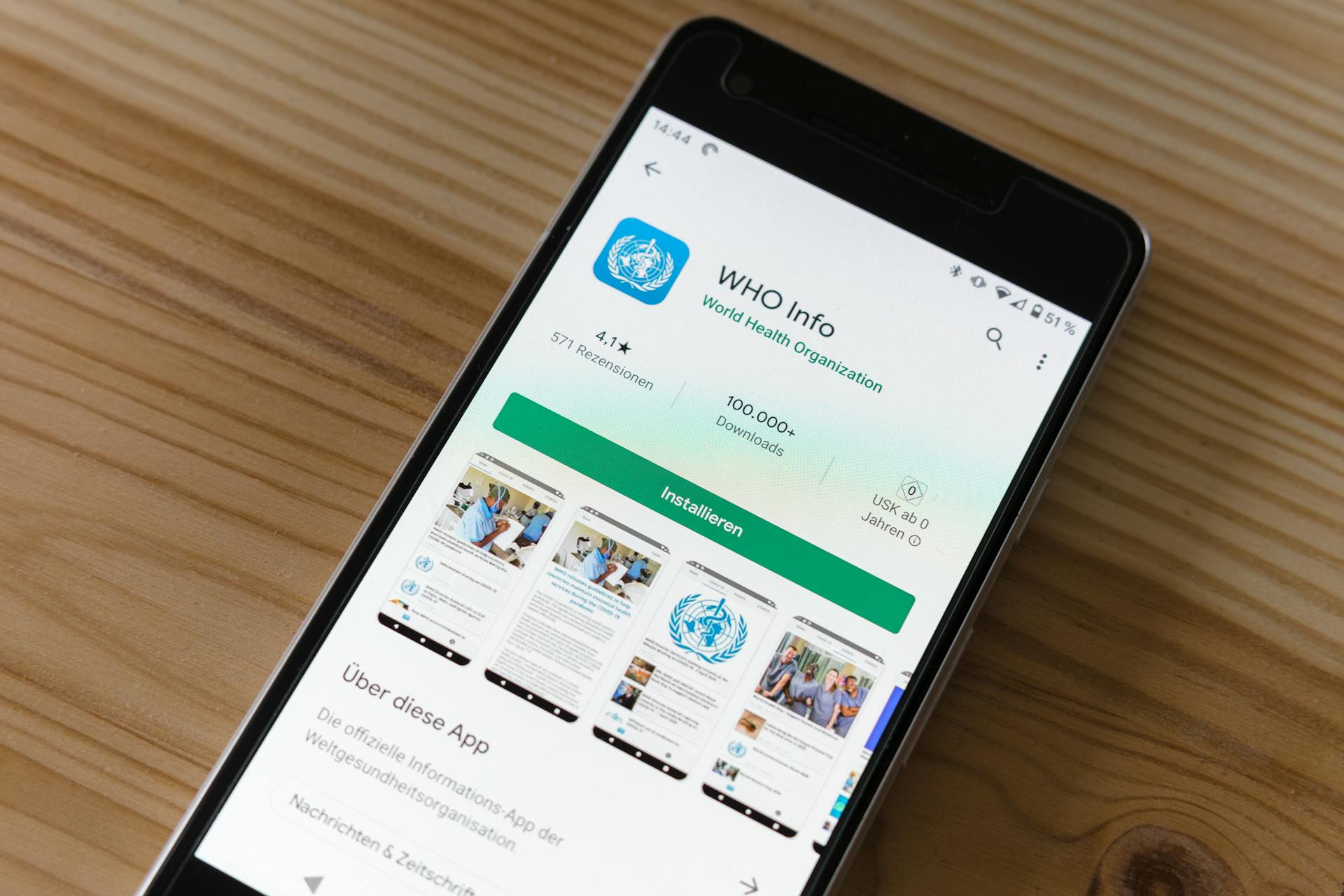
Implementing the Dropbox Android SDK is a straightforward process that can be completed in a few steps. First, you'll need to add the Dropbox Android SDK to your project by including the Dropbox SDK library in your Android project.
To do this, you'll need to add the following line of code to your project's build.gradle file: `implementation 'com.dropbox.core:android-sdk:4.8.0'`. This line specifies the version of the Dropbox SDK you want to use.
The Dropbox Android SDK is a powerful tool that allows you to integrate Dropbox functionality into your Android app. With it, you can enable users to upload and download files from Dropbox directly within your app.
You might like: Windows Azure Sdk
Getting Started
To get started with the Dropbox Android SDK, you'll need to download the SDK. Download the dropbox-android-sdk-1.6.3.jar and json_simple-1.1.jar files and place them in your project's Libs folder.
First, you'll need to download the latest version of dropbox-android-sdk from the official page. You can then extract the files and import them into your project's libs folder.
If this caught your attention, see: Azure Sdk Java
You'll need to add a dependency in your application's gradle file to use the Dropbox API. To do this, click on File -> Project Structure and select the Dependencies tab.
The Dropbox API requires you to download and import the dropbox-android-sdk and json_simple-1.1.jar files. These files should be placed in your project's Libs folder.
API Integration
API Integration is a crucial step in developing an Android app that utilizes the Dropbox Android SDK. To integrate the Dropbox API, you'll need to download the SDK and add it to your project.
The Dropbox API allows apps to store and sync files with Dropbox, create a private Dropbox, and access user files. By integrating the Dropbox chooser SDK, users can access their Dropbox files and download them to their local storage.
To implement the Dropbox API in your Android project, you'll need to download the dropbox-android-sdk-1.6.3.jar and json_simple-1.1.jar files and place them in your Libs folder. If you're using Android Studio, you'll need to add a dependency in your application's Gradle file.
Worth a look: How to Download Files from Dropbox to Android Phone
The Dropbox API requires you to apply for a Dropbox developer app, which will give you an App Key and App Secret. These values are used to authenticate your app with the Dropbox API. You'll also need to create a Session, which allows your app to authenticate with the Dropbox API.
Here's a summary of the steps to integrate the Dropbox API:
- Download the dropbox-android-sdk-1.6.3.jar and json_simple-1.1.jar files
- Add the dependency in your application's Gradle file (if using Android Studio)
- Apply for a Dropbox developer app and obtain an App Key and App Secret
- Create a Session to authenticate with the Dropbox API
By following these steps, you'll be able to integrate the Dropbox API into your Android app and provide users with a seamless experience for storing and syncing files with Dropbox.
Android App Implementation
To implement Dropbox in your Android app, you'll need to download the SDK and add it to your project. You can download the SDK from the Dropbox developer console, where you'll also find your app key and app secret.
In your Android project, you'll need to add the Dropbox SDK to your Libs folder, as shown in Example 4. You'll also need to add the necessary permissions to your AndroidManifest.xml file, including the internet permission.
Suggestion: Gdrive Android
Once you've set up the SDK, you can create a session that allows your app to authenticate with the Dropbox API. This is done by calling the buildSession() method, as shown in Example 3. You'll also need to add the necessary code to handle the authentication process, including the onActivityResult() method.
Here's a summary of the steps to implement Dropbox in your Android app:
By following these steps, you'll be able to implement Dropbox in your Android app and allow users to upload and download files from their Dropbox account.
Step 8 Dashboard
You'll be redirected to your dashboard screen, which contains all the useful parameters to access your Dropbox account from your device.
The dashboard screen includes the App Key and App Secret, which provide a unique key that you need to pass in your application to access your Dropbox account from mobile devices.
Note that the Permission type will be Full Dropbox access, allowing you to access all your Dropbox files and folders from your app.
Now that you have your App Key and App Secret, you can use them to authenticate your app and access your Dropbox account.
Related reading: What Is the Dropbox App
Android App Project Implementation
To implement Dropbox in your Android app project, you'll need to download the Dropbox SDK. Specifically, you'll need to download the dropbox-android-sdk.jar file and place it in your project's Libs folder.
First, create an account on the Dropbox website and generate an app key and app secret. These will be used to authenticate your app with Dropbox. You can find more information on how to do this in Example 1, where it's explained that you need to go to www.dropbox.com/developers/apps and login to create a new app.
Once you have your app key and app secret, you'll need to create a new class in your Android project to handle the Dropbox API. This class will need to extend the AsyncTask class, as explained in Example 5, where it's shown how to create a new class called "UploadFile.java" that extends AsyncTask.
To authenticate with Dropbox, you'll need to create a session using the AppKeyPair class, as shown in Example 8, where it's explained how to create a new AppKeyPair object using your app key and app secret. You'll also need to handle the authentication process in your Android app's onCreate() method, as explained in Example 9, where it's shown how to create a new AndroidAuthSession object and start the authentication process.
Check this out: How Do I Create a Dropbox Account
Here's a summary of the steps you'll need to take to implement Dropbox in your Android app project:
- Download the Dropbox SDK and place it in your project's Libs folder
- Create an account on the Dropbox website and generate an app key and app secret
- Create a new class in your Android project to handle the Dropbox API
- Create a session using the AppKeyPair class
- Handle the authentication process in your Android app's onCreate() method
By following these steps, you should be able to successfully implement Dropbox in your Android app project.
File Management
File Management with Dropbox Android SDK is a breeze. You can search files in a user's Dropbox account by initializing the default page data, checking for the path and query parameters, and assigning them to variables.
The path is crucial, as it needs to be an existing path in the user's Dropbox, starting with a forward slash to indicate the root directory. The query is the file name the user wants to find, and it doesn't have to be exact.
To send the search request, you'll need to pass along the mode, which can be filename, filename_and_content, or deleted_filename. Once you get the response, you can extract the matches and display them in a table, showing the file name, revisions, and download links.
Searching Files
Searching files in your Dropbox account is a straightforward process. To initiate a search, you need to pass the path and query as query parameters. The path must be an existing directory in your Dropbox account, such as /Files, /Documents, or /Public, and must start with a forward slash.
The query is the name of the file you're looking for, and it doesn't have to be the exact file name. You can also specify the mode of search, which can be filename, filename_and_content, or deleted_filename.
When you send the request, you'll get a response back, which you'll need to extract the response body from. This body will contain an array of matches found, along with the path and query you passed in.
If the number of matches is greater than 0, you can render the search results table, which displays the filename, a link for showing the revisions in the file, and a download link for downloading the file.
Explore further: Do I Need Dropbox
Uploading Files
Uploading Files is a crucial part of any file management system. You can upload files to Dropbox using the app's upload feature.
The upload view is a form that asks for the path to which the file will be uploaded and the file itself. This form is rendered in the resources/views/admin/upload.blade.php file.
Once the form is submitted, it's processed by the doUpload method, which checks if a file has been uploaded and if a path has been specified. If it hasn't been set, the user is redirected back to the upload page with an error message.
If the path and file are present, the app checks if the file has a valid mime type and size. If so, the request parameters are added, including the path to where the file will be saved in the user's Dropbox.
The mode option is used to specify the action to be performed if the file already exists. This can be either add, overwrite, or update. The autorename option should be set to true if the mode is set to add, so the new file will be automatically renamed if a file with the same name already exists.
Related reading: Dropbox Upload Speed Slow
The mute option is used to tell Dropbox whether to notify the user or not. The default for this option is false, which means the user will be notified by the Dropbox app when a file is uploaded.
To make the file upload request, the app uses the content client as opposed to the API client. The upload options are passed in the Dropbox-API-Arg header as a JSON string, while the binary data for the file itself is passed in the request body. The Content-Type is set to application/octet-stream, as you're passing in binary data to the request body.
Once you get a response back, the app converts it to an array and checks for the filename. If it's present, the user is redirected back to the upload page and informed that the file was uploaded.
Sources
- http://www.theappguruz.com/blog/10-steps-integrate-dropbox-api-android
- https://www.technetexperts.com/dropbox-api-integration-in-android-app-development/
- https://examples.javacodegeeks.com/android/android-dropbox-implementation-example/
- https://www.codemzy.com/blog/dropbox-long-lived-access-refresh-token
- https://www.sitepoint.com/build-your-own-dropbox-client-with-the-dropbox-api/
Featured Images: pexels.com