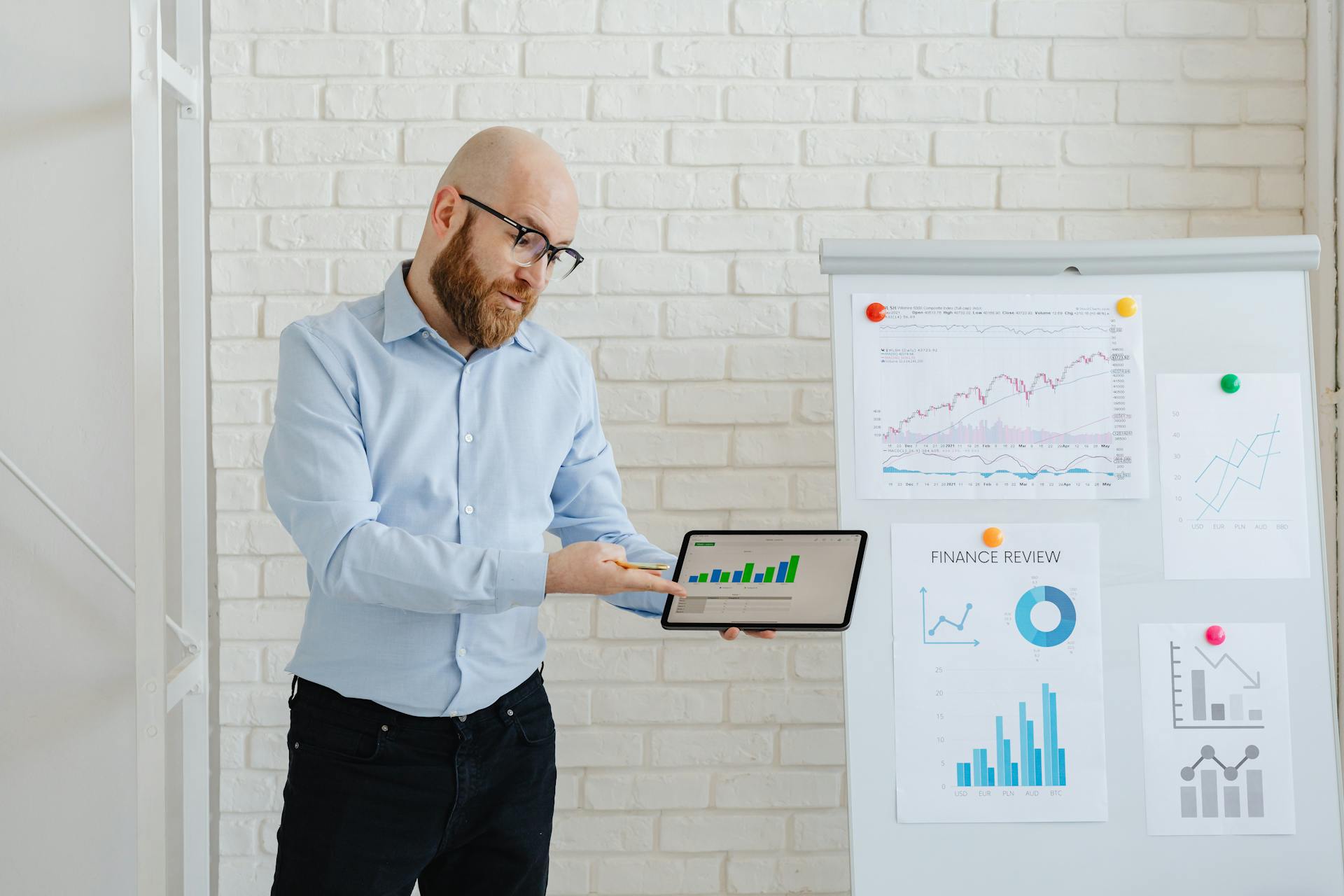
To integrate a Google Drive Service Account API, you need to create a service account in the Google Cloud Console.
A service account is a special type of Google account that belongs to your application instead of being tied to an individual user.
You can create a service account by going to the Google Cloud Console, clicking on the select a project dropdown menu, and then clicking on the "Create Service Account" button.
To generate a private key file for your service account, you need to click on the "Keys" tab and then click on the "Add key" button.
A unique perspective: How to Make Shared Google Drive
Setting Up Google Drive Service Account
To set up a Google Drive service account, you'll need to create a service account and obtain a JSON key associated with it. You can find a tutorial on how to do this in another article.
First, head over to the Google Cloud Platform and create a new service account. This will allow you to access your Google Drive data automatically, without prompting for permission.
For your interest: Azure Service Account
Once you have your service account set up, you can enable the Google Drive API on your Google Cloud Platform project. This is done by clicking on the Enabled APIs and services link in the left navigation menu, and then clicking the Enable APIs and services button.
To grant access to your service account, sign in to your Google Ads account as an administrator, navigate to Admin > Access and security, and click the + button under the Users tab. Type in the service account email and select the appropriate level of account access.
If you're accessing your own Google Drive account, you can use a service account to access your data without asking for permission. Simply add the service account email to the folder you want to access, just like you would with any other user.
To send requests to Google Drive, you'll need to create a "BaseClientService" using your credentials. This will allow you to perform requests to Google Drive.
Here are the steps to create a service account:
- Create a project in the Google Cloud Platform to associate the account with.
- Enable the Google Drive API.
- Create the service account, including entering the service account name and ID.
- Generate a JSON key for the service account.
Remember to store the JSON key securely, as this is the only copy of the key.
Managing Access and Permissions
To set up a service account, you need to create a service account and credentials, and download the service account key in JSON format. Note the service account ID and email.
You can grant access to your Google Drive account using a service account, which is automatic and doesn't require user permission. This is useful when you own the data already.
To grant access, simply add the service account email to the folder you want to give access to, just like you would for any other user.
A service account also has its own Google Drive account, so you can upload data to it. However, you won't be able to see the files on the website version of Google Drive.
To access the files, create a directory on the service account and grant your personal Google account full access to it. This way, you'll be able to see the files on your web site version of Google Drive.
The service account will own the files it uploads, so it will have to give you permission to access them as well.
Additional reading: Data Engineering with Google Cloud Platform
Authentication and Authorization
You'll need to authenticate your service account to access Google Drive data. OAuth2 is one type of authentication that allows you to access another user's data, and the code for it is slightly different from a service account.
To authenticate, you have two options: OAuth2 or a service account. A service account can only impersonate users in the same Google Workspace.
For authentication to work, you'll need to enable the Google Drive API on your Google Cloud Platform project. This is done by clicking on the Enabled APIs and services on the left navigation menu and then clicking on the button to enable the API.
Curious to learn more? Check out: Microsoft Azure Infrastructure as a Service
Enable Google Drive API
To enable the Google Drive API, you need to start by clicking on the Enabled APIs and services on the left navigation menu.
Once you've located the Enabled APIs and services section, click on the button at the top named Enable APIs and services.
This will allow you to access the Google Drive API, which is a crucial step in the authentication and authorization process.
See what others are reading: Azure Api Manager
Types of Authentication
Authentication is the key to accessing Google Drive API data. There are two types of authentication: OAuth2 and a service account.
OAuth2 allows you to access another user's data, which is useful for certain applications. The code for OAuth2 is slightly different from a service account.
A service account can be set up to access your own data, making it a convenient option for personal projects.
Discover more: Google Cloud Platform Data Centers
Authorization Using Impersonation
Authorization using impersonation is a viable option for accessing Google Ads accounts. This approach involves impersonating a user who has access to the account using a service account.
Only Google Workspace customers can use this method. A service account can only impersonate users with email addresses in the same Google Workspace.
Impersonation requires the service account to have the necessary permissions to access the Google Ads account. This ensures that the impersonated user has the required access rights.
For example, a service account can impersonate a user with an email address like "[email protected]" if they are both in the same Google Workspace.
Worth a look: Google Workspace Delegation of Google Drive
Security and Implementation
Protecting your Google Drive service account's key file is crucial, especially since it can impersonate any user in your domain. This key file is the key to accessing Google services, so keep it safe.
A good practice is to allow service accounts to access only the minimum required set of APIs, which limits the amount of data an attacker can access if the service account's key file is compromised.
Recommended read: Google Drive Shared File Easy Transfer to My Drive
Security Concerns
Protecting your service account's key file is crucial since it allows the service account to impersonate any user in the domain.
This key file is like the master key to your entire Google Workspace domain, so it's essential to keep it safe.
Allowing service accounts to access only the minimum required set of APIs is a good practice that limits the amount of data an attacker can access if the service account's key file is compromised.
By doing so, you're essentially setting up a firewall to prevent unauthorized access to sensitive data.
Intriguing read: Azure Email
Implementation
To implement authentication with Google APIs, you can define a generic function to create a service authenticated with credentials from a service account. This function can be used to authenticate with different APIs.
For example, you can use the "https://www.googleapis.com/auth/drive.metadata.readonly" scope to access Google Drive API v3. If you want to use other scopes, you can find the complete list of scopes for Google Drive API v3 here.
You'll need to share files and folders with the service account's email to access them from your script. To do this, go to the Google Cloud Console, navigate to IAM & Admin > Service Accounts, and copy the service account's email.
For more insights, see: Google Drive Scopes
Service Account Details
A service account is a way to access your Google Drive data without asking for permission from the user, making it perfect for automated tasks.
You can create a service account by creating a project in the Google Cloud Platform and enabling the Google Drive API.
To create a service account, go to the Google Cloud Console and click Menu ☰ > IAM & Admin > Create a Project, then enter a project name and click Create.
A service account has its own Google Drive account, so you can upload data to it, but you won't be able to see it on the website unless you grant your personal account access to the service account's directory.
You can grant your personal account access to the service account's directory by adding your email to the directory like you would add any other user.
To create a service account, you need to create a project, enable the Google Drive API, and then create the service account by clicking Menu ☰ > IAM & Admin > Service Accounts and following the prompts.
A service account can be tricky to work with because it owns the files it uploads, so you'll need to give your personal account permission to access them.
Check this out: Google Super Admin Google Drive Individual Accounts
Frequently Asked Questions
What is the difference between a Google Account and a service account?
A Google Account is a personal account for humans, while a service account is a special account for applications to access Google APIs without human interaction
How to view Google service account Drive?
To view a Google service account's permissions, navigate to the Service Accounts page in the Google Cloud console, select a project, and click on the service account's email address. From there, you can view the permissions tab to see the account's Drive permissions.
What is a service account in Google API?
A service account is a special Google account that allows applications to access Google APIs without human authorization, using a secure key file. This key file enables automated access to Google APIs, making it ideal for programmatic interactions.
Sources
- https://www.labnol.org/google-api-service-account-220404
- https://medium.com/@matheodaly.md/using-google-drive-api-with-python-and-a-service-account-d6ae1f6456c2
- https://developers.google.com/google-ads/api/docs/oauth/service-accounts
- https://www.daimto.com/google-drive-authentication-c/
- https://blog.zephyrok.com/google-drive-api-with-service-account-in-python/
Featured Images: pexels.com