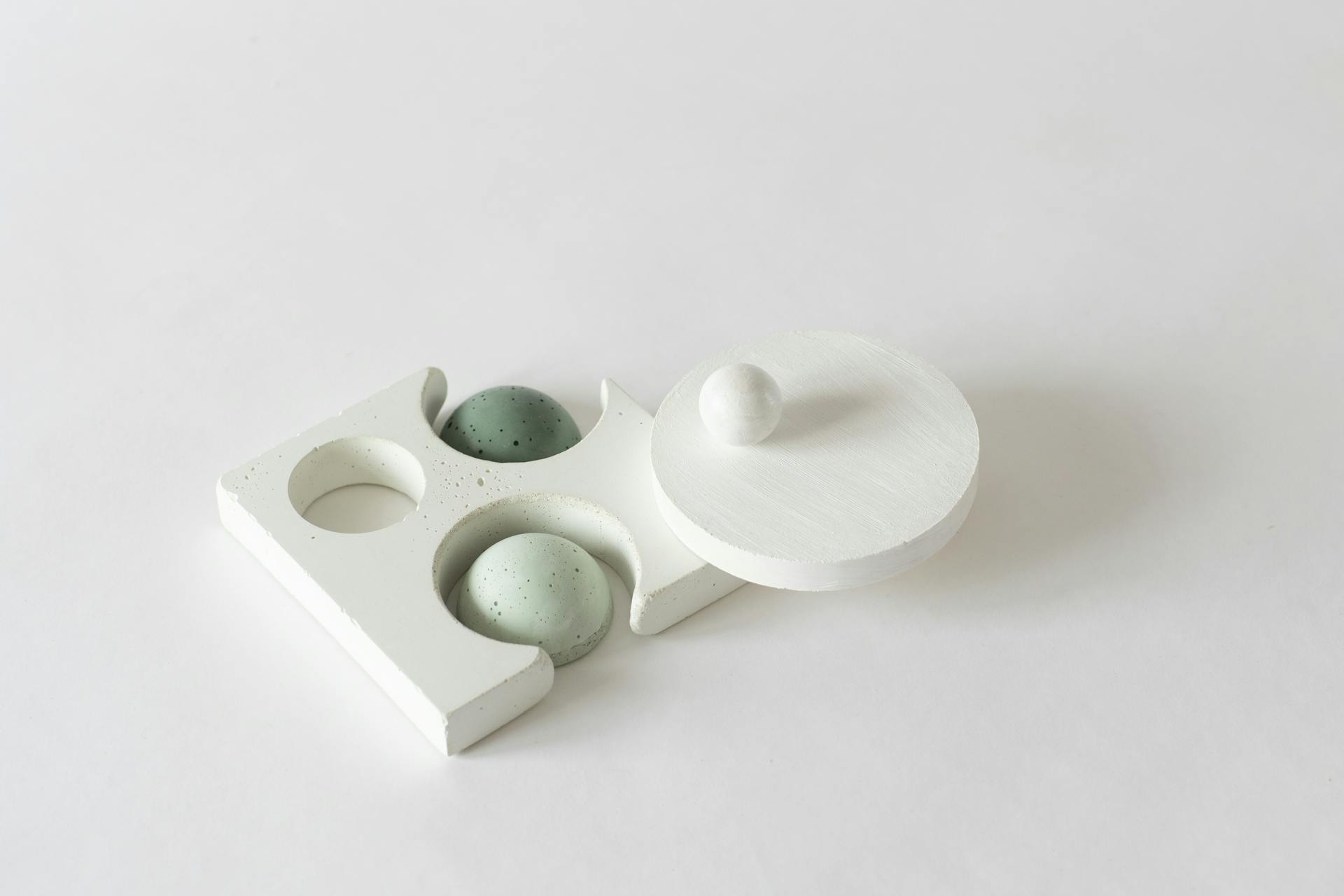
Django and Tailwind CSS are a match made in heaven. By integrating Tailwind CSS into your Django app, you can give it a sleek and modern look that will impress your users.
With Tailwind CSS, you can use its utility-first approach to create a responsive design that adapts to different screen sizes. For example, in the article, we learned how to use Tailwind's grid system to create a responsive layout that looks great on both desktop and mobile devices.
Tailwind CSS also provides a wide range of pre-defined classes that you can use to style your app's elements. We saw how to use these classes to create a consistent design language throughout the app, from buttons to forms.
By following these steps, you can give your Django app a professional-looking design that will make a great first impression on your users.
Discover more: Django Nextjs
Installation and Setup
To get started with Tailwind CSS in your Django app, you'll first need to install it. You can do this by running the following commands in your terminal: npm init -y, npm install tailwindcss, and npx tailwindcss init. This will create a new package.json file, install Tailwind CSS, and generate a tailwind.config.js file.
Explore further: How to Install Tailwind Css in Vscode
You'll also need to update the content array in tailwind.config.js to include paths to your Django templates. This might look something like this: "paths": ["templates/**/*.{html,css}"], depending on where your templates are located.
To ease execution of build and minify tasks, you can add the following scripts to your package.json file: "build": "tailwindcss -i input.css -o output.css", "minify": "cssmin output.css", and "watch": "tailwindcss -i input.css -o output.css --watch". You'll need to adapt the paths according to your Django static folder location.
You can then configure your template paths in tailwind.config.js to parse your content. This could be something like "paths": ["templates/**/*.{html,css}"], depending on where your templates are located.
To keep your output.css file up to date, you can run npm run tailwind-watch while you're coding. This will regenerate your output.css file as soon as you add a new Tailwind class to your code.
Here are some key steps to keep in mind:
- Create a new directory within your Django project to install Tailwind CSS.
- Configure your template paths in tailwind.config.js.
- Add scripts to your package.json file to ease execution of build and minify tasks.
- Run npm run tailwind-watch while coding to keep your output.css file up to date.
- Don't forget to include the npm run tailwind-build script in your deployment process.
You can also use the django-tailwind plugin, which produces similar results to the npm method. To install this plugin, run pip install django-tailwind. Then, go and start tailwind in dev mode.
You might enjoy: Django Cms
Integrating with Django
To integrate Tailwind CSS with Django, you'll need to add 'tailwind' to your INSTALLED_APPS in settings.py. This is a straightforward step that sets the foundation for using Tailwind in your Django project.
For a more seamless experience, consider using the Django-Tailwind package, which is well-documented and up-to-date. With this package, you can install the python package django-tailwind from pip and start tailwind in dev mode using the latest release, which supports the latest version of Tailwind CSS.
To get started with using Tailwind directives in your Django templates, create an input.css file in your static directory and include the necessary Tailwind directives. This will allow you to use Tailwind classes in your HTML templates.
Discover more: Making Analytics Website in Django
Django Starter
To get started with integrating Tailwind CSS into your Django project, you'll need to add it to your INSTALLED_APPS in settings.py. This is the first step in using the Tailwind + Django starter.
The middleware should be listed after any that encode the response, such as Django's GZipMiddleware. This ensures that the middleware can insert the required script tag on HTML responses.
To enable live reload, you'll need to include django_browser_reload in your root url.py: path("__reload__/", include("django_browser_reload.urls")).
Here's a step-by-step guide to setting up the Tailwind + Django starter:
1. Add 'tailwind' to INSTALLED_APPS in settings.py
2. Include django_browser_reload in your root url.py
3. Run the command to install all necessary dependencies for Tailwind CSS
Integrating Django Templates
To integrate Tailwind CSS into your Django templates, you need to create an input.css file in your static directory. This file will contain the Tailwind directives.
You can include the following Tailwind directives in the input.css file, making sure to replace the path to your static css assets:
Create the input.css file in your static directory, e.g., /path-to/static/css/input.css, and include the following Tailwind directives:
note: make sure to replace /path-to/static/css/ with the actual path to your static css assets.
Once you have created the input.css file, you need to load the CSS in your templates. This is done by including the following code at the top of your template:
Here's an interesting read: Html Tailwind Css Templates
This will load the CSS and make it available for use in your templates.
You can also use a template like the one provided by Django Tailwind, which is located under your tailwind app name/templates/base.html. You can extend this template or delete it if you have your own layout.
In any case, you should now be able to use Tailwind CSS classes in your HTML.
Take a look at this: Why Use Tailwind Css
Using Tailwind Features
To use Tailwind features, you'll need to install Tailwind CSS, which can be done by running a command on the terminal.
This command will install all the Tailwind dependencies, getting you one step closer to customizing your Django app's look.
After installing Tailwind, you'll need to initialize it, which can be done by running another command that will generate a config file named "tailwind.config.js".
Additional reading: Tailwind Css with Vite
Using Tailwind CSS
First, you need to install Tailwind CSS. Run the command on the terminal to install all the tailwind dependencies.
To install Tailwind CSS, create a new directory within your Django project and install it like in any vanilla JS project setup.
Configure your template paths in tailwind.config.js by specifying the right place to parse your content. This could be something like configuring the paths to your Django templates.
You can prepare npm scripts to ease execution of build / minify tasks in your package.json file. Adapt the paths according to your Django static folder location.
To keep your output.css file up to date, run npm run tailwind-watch while you're coding. This will ensure that your output.css file is regenerated as soon as you add a new tailwind class to your code.
Don't forget to add the output.css file to .gitignore.
To actually use tailwindCSS classes, include the outputted css file into a Django template file along with Django's call to load the static files.
You can include the following npm scripts to your package.json file to ease execution of build / minify tasks:
- tailwind-watch: npm run tailwind-watch
- tailwind-build: npm run tailwind-build
Finally, don't forget to include the npm run tailwind-build script in your deployment process. This will build the output and remove unused classes to ensure a lower file size.
Forms
Forms can be a bit ugly even when using Tailwind in a Django project.
You can improve the form style by using tailwindcss/forms, a plugin that provides a basic reset for form styles.
This plugin makes form elements easy to override with utilities, which is a game-changer for customizing your forms.
To get started, update your frontend/tailwind.config.js file to tell Tailwind CSS which CSS classes are used by your project's forms.
Six Answers
There are at least three different methods to install Tailwind with Django properly. You can choose the one that best suits your needs.
You have the option to use a package manager like npm or yarn to install Tailwind. This method is often the most straightforward.
Using a package manager can save you time and effort in the long run. It also allows you to easily manage your dependencies.
Another method is to use a tool like Tailwind CLI. This can be a good option if you prefer a more hands-on approach.
Tailwind CLI provides a lot of flexibility and control over the installation process. It's a great choice if you want to customize your installation.
Finally, you can also use a Django package like django-tailwindcss. This method is often the most convenient.
Compiling and Configuring
Create a new file named tailwind.config.js in your project's root directory and add the necessary content to configure Tailwind CSS for your Django project.
To create a PostCSS configuration, create a new file named postcss.config.js in the project's root directory and include the necessary content.
To compile assets using Tailwind CSS, run the command in your terminal to compile the assets.
Here's a quick rundown of the steps to configure Tailwind CSS:
- npm init -y: Creates a new package.json file with default settings for a Node.js project
- npm install tailwindcss: Installs Tailwind CSS into the project as a dependency.
- npx tailwindcss init: Generates a tailwind.config.js file, allowing customization of Tailwind CSS settings for the project.
Remember to update the content array in tailwind.config.js to include paths to your Django templates, replacing path-to/css/output.css with the actual path used in the package.json scripts.
Handling Auto-Reload Locally
Handling auto-reload locally is a game-changer for development. You'll want to use Django-browser-reload, which works as expected out of the box.
To get started, make sure you have npm properly installed on your system. You can check the DjangoTailwindStarter template on GitHub for a minimal setup.
If you're using a Mac, be aware that you might encounter a "Permission denied" error when running npm run-script build. This issue doesn't occur on Windows.
For another approach, see: Tailwind Css Npm
Also, don't forget to create a package.json file using npm init -y before installing TailwindCSS and other dependencies.
Here are the essential steps to set up auto-reload locally:
- Install Django-browser-reload and follow the setup instructions.
- Use npm-watch to build the output automatically when either the input CSS or the config is changed.
By following these steps, you'll be able to auto-reload your Django development server when an HTML file is changed and saved.
Configuring
Configuring is a crucial step in the process. You'll need to create a new file named tailwind.config.js in your project's root directory.
To configure Tailwind CSS, you'll need to create a new file named tailwind.config.js. Add the following content to this file.
You can also create a new file named postcss.config.js in the project's root directory. Include the following content in this file.
To configure Tailwind CSS, you can follow these steps:
- Npm init -y creates a new package.json file with default settings for a Node.js project.
- Npm install tailwindcss installs Tailwind CSS into the project as a dependency.
- Npx tailwindcss init generates a tailwind.config.js file, allowing customization of Tailwind CSS settings for the project.
To update the content array in tailwind.config.js, you'll need to include paths to your Django templates. Replace path-to/css/output.css with the path used in the package.json scripts.
Compile Assets
Compiling Assets is a crucial step in the process. You'll need to run a command in your terminal to compile the assets using Tailwind CSS.
To do this, you'll use the following command: run the following command to compile the assets using Tailwind CSS.
Why and How
Making your Django app look pretty with Tailwind CSS is a game-changer. Tailwind CSS allows you to write more concise and maintainable CSS code.
To get started, you need to install Tailwind CSS in your Django project. This can be done by running the command `npm install tailwindcss postcss autoprefixer` in your terminal.
Tailwind CSS is designed to be highly customizable, with a wide range of pre-defined classes that can be used to style your app. This makes it easy to create a consistent look and feel across your app.
One of the key benefits of using Tailwind CSS is that it allows you to write CSS in a more functional way. This means that you can use utility-first classes to style your app, rather than writing custom CSS code.
For example, you can use the `bg-purple-500` class to set the background color of an element to a deep purple color. This is just one of many utility classes available in Tailwind CSS.
By using Tailwind CSS, you can create a beautiful and consistent UI for your Django app with minimal effort.
For more insights, see: Tailwind Css Class
Frequently Asked Questions
What is the best CSS to use with Django?
For seamless integration with Django, consider using Tailwind CSS, which offers a harmonious development process with minimal code complexity.
How to add CSS style in Django?
To add CSS style in Django, create a static files directory with a styling file named 'style' inside it. This simple step will help you give your Django project a visually appealing design.
Can I combine Tailwind and CSS?
Yes, you can combine Tailwind with CSS, as it's a PostCSS plugin that works seamlessly with popular preprocessors like Sass, Less, and Stylus. Tailwind integrates well with CSS preprocessors, making it easy to use in your existing workflow.
Sources
- https://www.geeksforgeeks.org/how-to-use-tailwind-css-with-django/
- https://stackoverflow.com/questions/63392426/how-to-use-tailwindcss-with-django
- https://saashammer.com/blog/render-django-form-with-tailwind-css-style/
- https://www.tailwindtap.com/blog/how-to-use-tailwind-css-with-python-django
- https://www.readysaas.app/blog/how-to-setup-tailwind-in-django-project
Featured Images: pexels.com