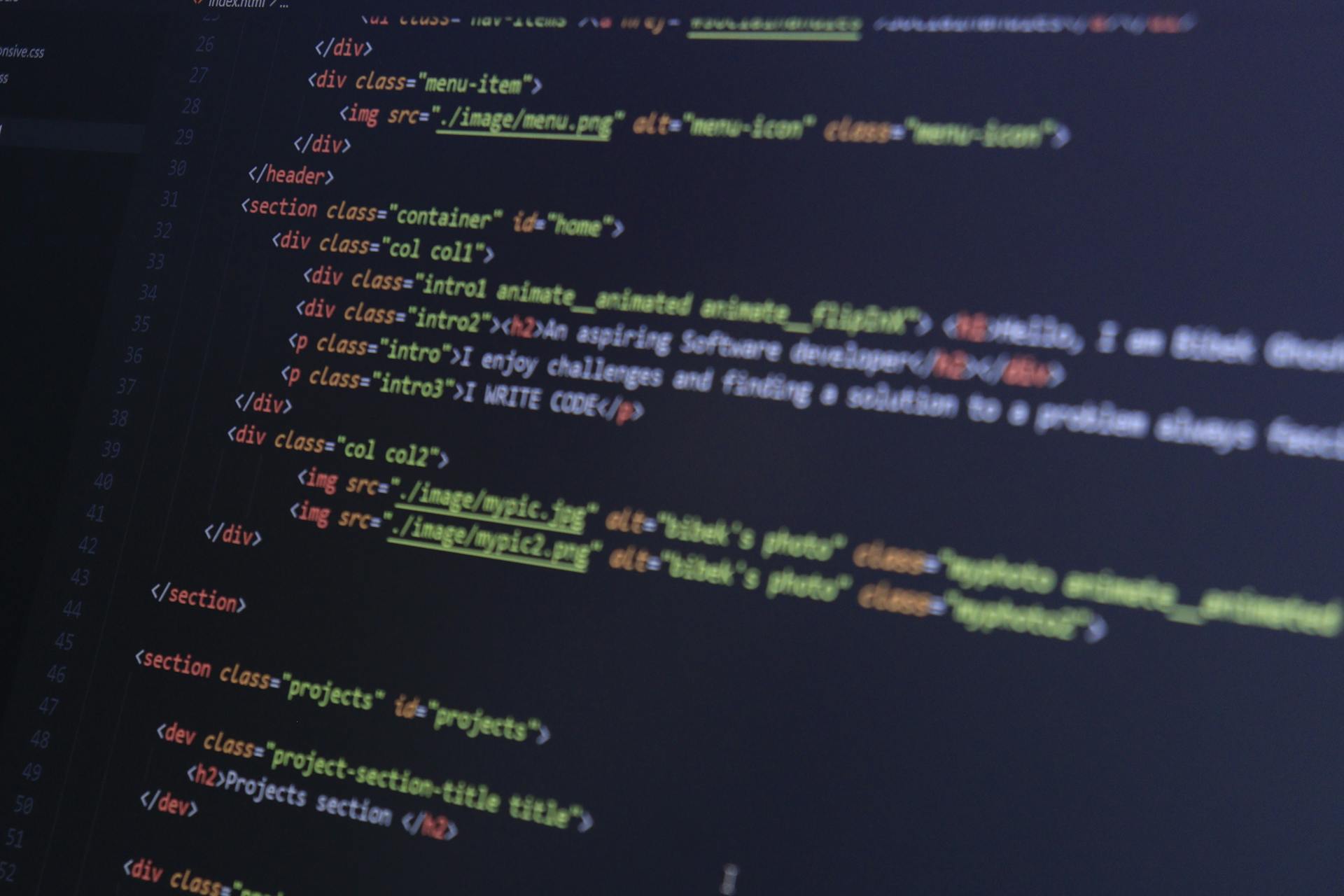
Creating a Django analytics website with user management and data visualization is a fantastic way to track and analyze your website's performance.
To get started, you'll need to install Django and the necessary packages, such as Django REST framework for building APIs.
One of the first steps is to create a new Django project and install the required packages.
In the article, we'll cover how to create a user management system using Django's built-in authentication and authorization system.
Intriguing read: Install Django Project
Setting Up the App
To set up the app, you'll need to install Django and create a new project.
First, install Django using pip: $ pip install django.
Next, create a new Django project by running the command: django-admin startproject analytics_project.
Navigate to the project directory: cd analytics_project.
Create a new Django app for your analytics website: python manage.py startapp analytics_app.
Install the necessary packages, including Django Rest Framework, by running: pip install djangorestframework.
Make sure to add 'rest_framework' to the INSTALLED_APPS list in settings.py.
Finally, run migrations to set up the database: python manage.py migrate.
If this caught your attention, see: Azure Web App Asp.net V4.8
Database and Models
In Django, models are classes that define the way Django creates a table. The class name refers to a table name, and the class attributes refer to each table's fields.
To create a database for our analytics website, we need to define seven models: Analytics, Event, RegisterEvent, LoginEvent, ViewPageEvent, EditProfileEvent, and LogoutEvent. These models will be used to save user actions on the site and record analytics or numbers from various events.
The Event class is the foundation of our database, defining the Event table to save user actions on the site.
For more insights, see: Event Web Page Design
Database Connection
Connecting your database to Arctype is a straightforward process. First, you'll need to create an account and download Arctype, then you'll be directed to an initial database setup page.
To connect an existing database, simply click "I have an existing database" and then select your database type. This will lead you to a series of screens where you'll need to enter your database credentials, which should match the connection details in your settings.py.
If your database is running on localhost, you can use the default values. With Arctype connected to your database, you'll be ready to start filling it with data.
For more insights, see: How Much Traffic Does a Website Need to Make Money
Creating Models
Creating models is a crucial step in building your database. You'll define the structure of your data by creating classes that represent tables in your database.
In Django, these classes are called models, and they're used to create tables in your database. The class name refers to the table name, and the class attributes refer to each table's fields.
To create models, you'll need to define the fields that make up each table. In the example of creating Django models for analytics data, seven models are created: Analytics, Event, RegisterEvent, LoginEvent, ViewPageEvent, EditProfileEvent, and LogoutEvent.
Each model class defines a table to save specific data, such as user actions or site analytics. The Event class, for instance, defines the Event table to save user actions on the site.
The class attributes in each model class define the fields or columns in the corresponding table. By creating these models, you'll be able to easily manage and interact with your data in your Django application.
For your interest: Host Django Site
Grouping User Events
Grouping User Events is a crucial step in making sense of the data in your events table. As your site grows, this table will contain thousands of rows, making it hard to run analytics.
To tackle this, you can run a loop through the events table and check for the various event types before saving them into their respective model tables. This approach is more effective than trying to analyze the data in its current state.
You'll want to check if the data already exists in the table before saving it. This ensures you don't duplicate any data and saves storage space.
Running this loop will go through all the rows in the events table, making it a time-consuming process. However, it's a necessary step in getting your data organized.
If this caught your attention, see: Data Text Html Charset Utf 8 Base64
Why Use Arctype?
Arctype is a game-changer when it comes to querying SQL data. With its autocomplete functionality, you can run queries with ease, no need to memorize every SQL command.
Arctype's save functionality is also a huge time-saver, allowing you to save your commonly used queries for later use. This means you can focus on analyzing your data, not rewriting the same queries over and over.
Arctype dashboards enable seamless interaction with your data, making it easy to create eye-catching visualizations.
On a similar theme: Data Lake Analytics
User Management
In a Django analytics website, user management is crucial for tracking and analyzing user behavior.
To manage users, you can use Django's built-in User model, which is a base model for creating custom user models.
Custom user models can be created by inheriting from AbstractBaseUser, as shown in the "Custom User Model" section.
The User model is used to authenticate users, which is essential for tracking user behavior.
The User model has several fields, including id, username, first_name, last_name, email, and password.
These fields can be accessed and manipulated using Django's ORM.
To add a new user, you can use the User.objects.create() method, as demonstrated in the "Creating Users" section.
Take a look at this: Can I Use Google Analytics on Any Website
Similarly, to retrieve a user, you can use the User.objects.get() method, as shown in the "Retrieving Users" section.
User management is also important for assigning permissions and roles to users.
Django's built-in Permission model can be used to manage permissions, as explained in the "Managing Permissions" section.
By effectively managing users, you can gain valuable insights into user behavior and improve your analytics website.
Data Visualization
Data Visualization is a crucial part of any analytics website, and Django makes it easy to implement. To get started, you'll need to install Django and Chart.js, a popular JavaScript library for creating interactive graphs.
To create a chart, you'll need to define the data and labels in your views.py file, as shown in Example 1. This is where you'll specify the chart type, data, and labels.
Here's a simple structure of the ChartJS library, as described in Example 3: it works with HTML canvas and requires a JavaScript object with arrays for labels and datasets.
Readers also liked: Cloudfront Aws Webflow Example
In Django, you can use the ChartData class to return data to the chart, as shown in Example 1. This class takes in the chart data and labels, and returns a JSON response.
To display the chart on your website, you'll need to create a canvas element in your HTML template, as shown in Example 1. This is where the chart will be rendered.
Here's a list of the modules required to implement data visualization in Django:
- Django: install Django
- Django Rest Framework: install Django Rest Framework using pip
You can also use dynamically-linked values to create a doughnut chart that changes based on a table selection, as shown in Example 2. This involves creating a table with selectable rows and using the {{input_date}} dynamic value to update the date queried for the doughnut chart results.
Building the Dashboard
The views.py file is where we'll implement all the functionalities for your dashboard, so let's dive into it. It's where we'll define how the user interacts with the dashboard.
You might enjoy: Website Analytics Dashboard
We'll start by going through each application view, as mentioned in the Django project setup. This will help us understand the different components of the dashboard.
The views.py file is crucial in building the dashboard functionality. It's where we'll write the code that will handle user requests and display the necessary data.
Each view will handle a specific task, such as displaying a list of items or showing detailed information about a particular item. This is in line with the concept of modular code that we discussed earlier.
The views.py file will use Django's built-in views to handle HTTP requests and return HTTP responses. This is a fundamental aspect of building web applications with Django.
Readers also liked: Css in Html File
ListView and Routing
In a Django project, it's common to have multiple apps, each with its own urls.py file. This separation of concerns makes it easier to manage routing.
To include another app's urls.py file, you can use the include function in your project's urls.py file.
In the example of the billionaires app, the urls.py file is set up with a URL path that references a class-based view, BillionairesView. This view is a ListView, which is used to display a list of objects from the database.
Here's an interesting read: Web App Designs
Creating User Index View
Creating the User Index View is a crucial part of building a comprehensive user profile page in Django.
This view records page views for users' pages and allows users to update their profile information.
To start, we check if any data exists in the Event model that contains the same session key.
If the query returns true, we create an instance of the Event object with the session key and the current time.
We also collect data needed to update the user's profile, sent in a POST request, and save it in the user's profile while recording an event for this edit action.
The user's information is then passed to be rendered on the profile page in the context dictionary variable.
This includes data points like the number of users, the number of page views, the number of active sessions, the number of inactive sessions, and the logout register.
Implementing the ListView
The ListView is a great place to start when working with Django views. The ListView is a class-based view (CBV) that allows you to display a list of objects from your database.
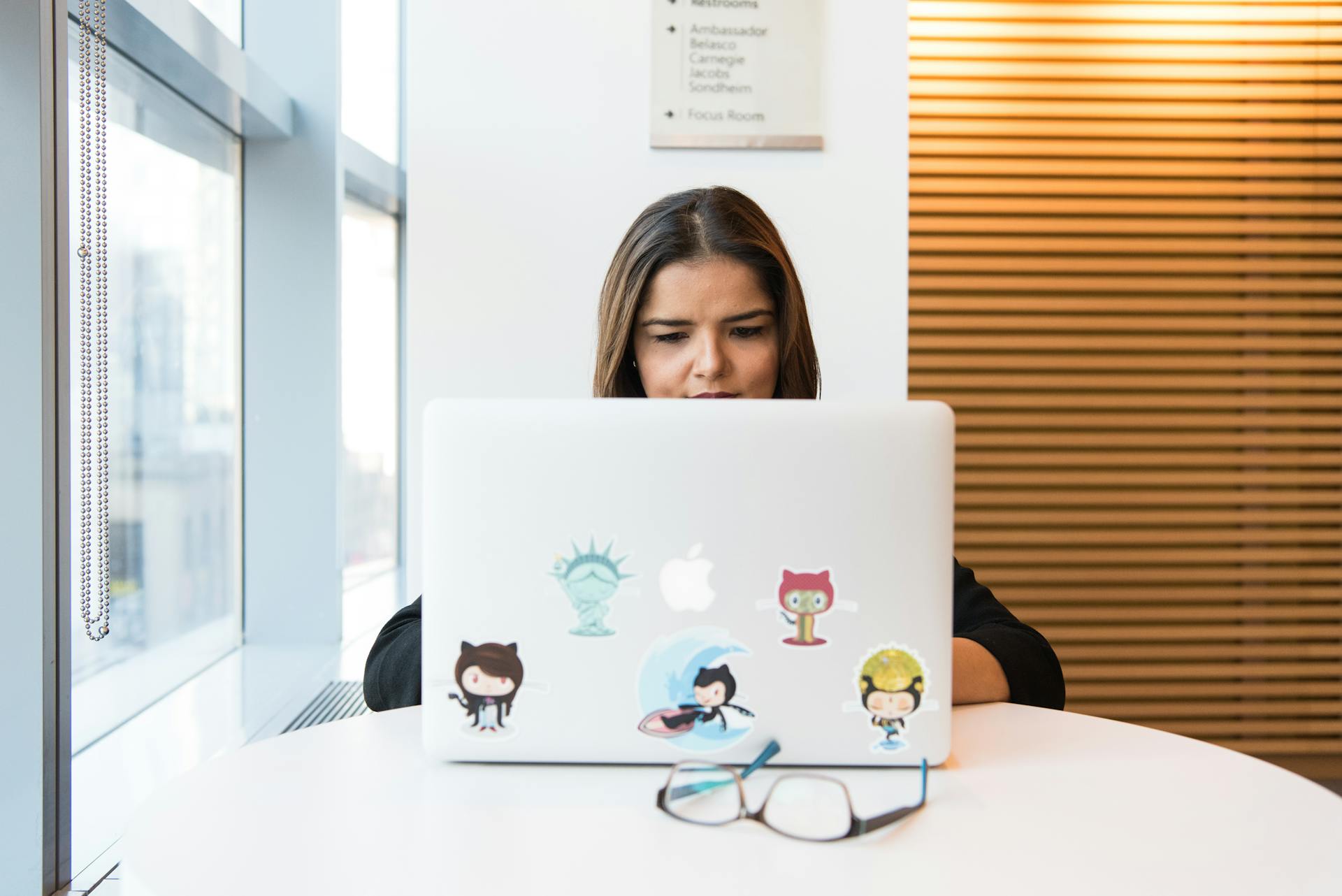
To implement the ListView, you need to create a view that inherits from ListView. This view will handle the logic of retrieving data from your database and displaying it in your template.
The BillionairesView is an example of a ListView that displays a list of billionaires' names and worth. You can use Django's template variable in double curly braces to access the data from your database.
To display the data, you can use the object_list method, which is an inbuilt method that lists all the objects in the database table. This method is useful when you don't want to store the data in a context name.
The ListView is a powerful tool for displaying data in a flexible and efficient way. It's a great choice for many use cases, and it's easy to use once you get the hang of it.
To use the ListView, you need to pass the model and template directory to the view. In this case, the model is Billionaires and the template directory is billionaires/charts.html.
The ListView also allows you to use Django's aggregate function to perform calculations on your data. For example, you can use the Max, Avg, Min, and Count functions to get the maximum, average, minimum, and count of the money column.
By using the ListView and Django's template variable, you can easily display data from your database in a readable and organized way. This is especially useful when working with large datasets.
Readers also liked: Html Css Box Model
URL Routing
URL Routing is a crucial part of Django development, and it's done through the use of URLs.py files.
To separate concerns and make it easier to manage routing, each app should have its own urls.py file. This is how it's done in the project urls.py, where the include function is used to include the billionaires urls.py file.
In the billionaires urls.py, the path function is imported and used to define a URL path. This path points to a class-based view, specifically BillionairesView, which is imported from views.py.
The URL path is given a name, 'billionaires', which can be used as a href tag to reference the URL. This name will not be affected even if the URL pattern changes, as it references any active pattern at the time.
Here's an interesting read: Html File
Libraries and Tools
To start building your analytics website in Django, you need to import the required Django libraries. This should be done at the top of your application, as it sets the foundation for the rest of your project.
These libraries will help you create a solid analytics application from scratch. You should start with the basics, just like you would with any new project.
You can import these libraries in a way that's easy to understand and manage.
Importing Libraries
Importing Libraries is a crucial step in creating any application, and I've found that starting with the basics is key.
To begin, you'll need to import all of the Django libraries needed to create your application. If you're trying to create your own analytics application from scratch, you should start with these libraries.
Importing the required libraries at the top of your code is a good practice to follow. This helps keep your code organized and easy to read.
Adding Posthog
Adding Posthog to your Django app can be done in two ways: by adding the snippet or using the Python library. This flexibility allows you to choose the method that best suits your needs.
To add the snippet, you'll need to follow the instructions provided, which involves integrating Posthog into your app. This approach is straightforward and easy to implement.
Using the Python library is another viable option, allowing you to leverage the power of Posthog within your Django app. By doing so, you can track user behavior with precision.
PostHog can be added to a basic Django app to track user behavior, making it an essential tool for any developer.
If this caught your attention, see: Python Website Hosting
Installation
To start making an analytics website in Django, you need to install the necessary packages. Python 3.7 is the recommended version to use for this project.
First, ensure that Python is downloaded to your system. Next, create a virtual environment for your project using the myvenv command. This is a good practice to keep different projects organized.
To activate the virtual environment in Windows using Git Bash, you need to type the activation code in your terminal.
Now, it's time to download the necessary packages using pip install. You'll need to download each package one at a time. Aside from mysqlclient, every other package is for deploying your app on Heroku.
After downloading those packages, create a Django project by typing the project creation code in your terminal. This will create a new project with a root folder, which is where you'll spend most of your time working.
Remember, in Django, there's a difference between a "project" and an "app". They're created with different commands.
Broaden your view: Web App Dev
Frequently Asked Questions
Can I create a website using Django?
Yes, you can create a website using Django, a high-level Python framework that allows you to build robust and scalable web applications. With Django, you can quickly develop a website that meets your needs.
How to create a dynamic website using Django?
To create a dynamic website using Django, start by setting up a new project and app, then follow a series of steps to configure URLs, views, templates, and database settings. By following these steps, you can build a fully functional and interactive website with Django.
Sources
- https://www.geeksforgeeks.org/data-visualization-using-chartjs-and-django/
- https://posthog.com/tutorials/django-analytics
- https://medium.com/database-dive/build-an-analytics-dashboard-with-django-and-arctype-669276d7a565
- https://masteringbackend.com/posts/building-a-basic-analytical-app-with-django-and-chartjs
- https://www.analyticsvidhya.com/blog/2021/12/using-django-framework-for-making-exciting-data-science-projects-an-example-use-case/
Featured Images: pexels.com