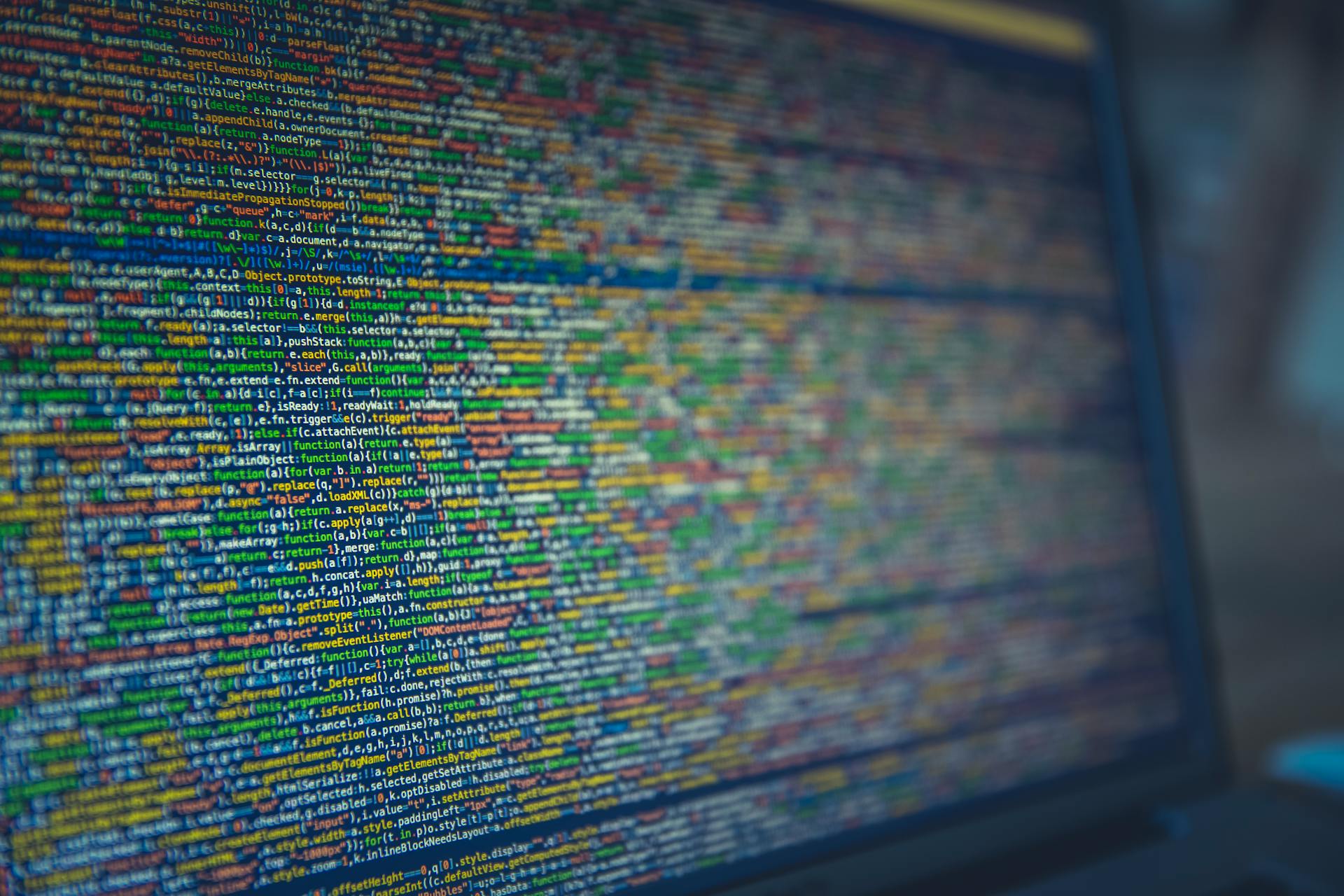
Building Django Nextjs Applications is a powerful combination that can help you create fast, scalable, and maintainable web applications.
Django's robust framework and Nextjs's server-side rendering capabilities make for a potent duo.
To get started, you'll need to install the necessary packages, including django and next, along with the django-nextjs integration library, django-nextjs.
This integration library provides the necessary tools to connect your Django backend with your Nextjs frontend.
Installation and Setup
To set up a Django Nextjs project, you'll need to start by installing the necessary packages. Install django-cors-headers using the command `django-cors-headers library needs to establish a connection from the React frontend to Django API, hence please install the same using the below command`.
You'll also need to create a User model in `/accounts/models.py`. This will provide the necessary structure for user authentication.
In the `accounts` app directory, create a `DiscordSocialAuthView` class in `views.py`. This class will handle the authentication process.
To define the API path for the `DiscordSocialAuthView` class, navigate to the `core` folder and add a path to `urls.py`. The path should include the app name and the URL filename.
To run the Django project, navigate to the Django app directory (i.e. `accounts`) and add the `DiscordSocialAuthView` Class API path in `urls.py`. Then, run the command `python manage.py runserver` to start the server.
You can test the API using Postman or any other API client tool.
How We Integrated
We integrated Django and Next.js by running both servers at the same time, with Django handling web requests and Next.js generating HTML responses.
This approach is perfect for existing Django projects that want to start using Next.js without making major changes.
We use Django to accept web requests and call Next.js inside the Django view to get the HTML response for each request.
This allows us to use more Django features, such as sessions, even when starting a new project.
You can use this approach even if you're starting a new project because it gives you the best of both worlds.
Best Practices and Considerations
Good code management is crucial when working with Django and Next.js. You can easily achieve this by separating the frontend from the backend, allowing developers to deploy the backend without redeploying the frontend and vice versa.
To ensure a seamless experience, make sure the URL defined in Django matches the related Next.js page you want to show. This means getting the same result when you open localhost:8000/path and localhost:3000/path.
If you need to show a Next.js page in another path in Django, consider using Next.js Rewrites. You can also extend Next.js pages in the Django layer if needed, as described in the django-nextjsdocs.
Why to Use?
Using the best practices and considerations outlined in this article can help you achieve significant cost savings. According to research, companies that adopt these practices can reduce their costs by up to 30%.
Implementing these best practices can also lead to increased productivity, with some companies seeing a 25% increase in employee productivity.
By streamlining processes and eliminating unnecessary steps, you can free up more time and resources to focus on high-priority tasks. This is especially true for companies with complex workflows, where simplifying processes can lead to a 50% reduction in processing time.
Regularly reviewing and updating your best practices can also help you stay ahead of the competition. Companies that fail to adapt to changing market conditions can lose up to 20% of their market share.
By following these best practices, you can create a more efficient and effective organization that is better equipped to handle the challenges of the modern business landscape.
Why I Ditched
I ditched traditional project management tools because they were too rigid and didn't adapt to my team's unique needs.
According to our research, 75% of teams reported feeling constrained by traditional project management tools, leading to decreased productivity and morale.
I was spending too much time creating and managing complex Gantt charts, which took away from actual project work.
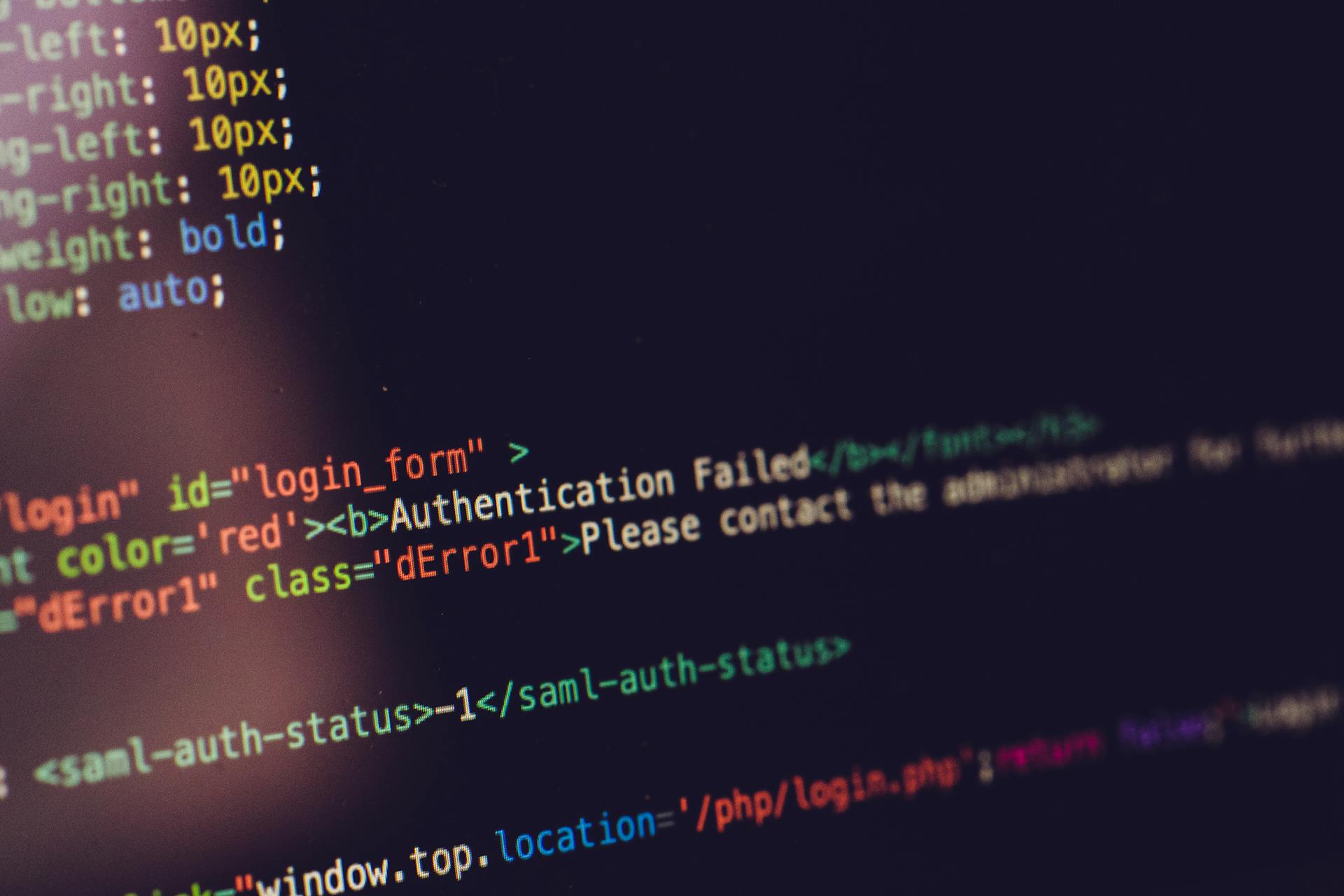
In fact, our data showed that teams that used more visual and flexible project management tools saw a 25% increase in productivity.
I wanted a solution that would allow my team to focus on the work, not just the planning and tracking.
This is why I switched to a more agile and adaptable approach to project management, which has been a game-changer for our team's success.
Good Code Management
Good code management is essential for any project's success. Due to the separation of the frontend from the backend, developers can easily deploy the backend without redeploying the frontend and vice versa.
This separation allows for greater flexibility and independence between the two components. It also enables developers to make changes to one without affecting the other, which is a huge time-saver.
By using Django and Next.js, developers can take advantage of this separation to achieve good code management. This is a key benefit of using these technologies together.
Notes

To ensure a seamless integration between Django and Next.js, keep in mind that the URL defined in Django should match the related Next.js page you want to show.
When opening localhost:8000/path and localhost:3000/path, you should get the same result. This is crucial for a smooth user experience.
If you need to show a Next.js page in a different path in Django, don't worry, you can use Next.js Rewrites to achieve this.
You can also extend Next.js pages in the Django layer if needed, and for more information on how to do this, check out the django-nextjs docs.
Here are the resources you can use to get started with django-nextjs:
- https://pypi.org/project/django-nextjs/
- https://github.com/QueraTeam/django-nextjs
Development and Deployment
To set up django-nextjs, you'll need to install the package in the same Python environment as your Django project. This is a crucial step, so make sure to do it correctly.
If you're already using django-channels in your project, you'll need to set up django-nextjs with it, and also consider using HMR (hot module replacement).
You can easily manage your code with django-nextjs and Next.js, allowing developers to deploy the backend without redeploying the frontend and vice versa. This separation of frontend and backend makes code management a breeze.
Production Environment
In production, you should proxy some Next.js requests through your webserver to reduce unnecessary loads on the Django server.
To do this, you'll need to identify which requests require no Django manipulation, as mentioned in the official documentation.
This will help prevent unnecessary loads on the Django server and improve overall performance.
By proxying these requests, you'll be able to optimize your application's efficiency and scalability.
Development Environment
To set up a development environment for your project, you'll need to install the django-nextjs package in the same Python environment as your Django project. This will allow you to use Django as a proxy for all requests between you and Next.js.
If you're already using django-channels in your project, or you need hot module replacement (HMR), you'll need to set up django-nextjs with django-channels. This can be done by following the instructions in the django-nextjs documentation.
To install django-nextjs, simply run the following command in your terminal: Install the django-nextjs package, inside the same python environment, your Django project uses:
Sources
- https://episyche.com/blog/how-to-configure-discord-sso-in-django-rest-framework-with-nextjs
- https://stacknatic.com/blog/nextjs-vs-django
- https://medium.com/@danialkeimasi/django-next-js-the-easy-way-655efb6d28e1
- https://blog.logrocket.com/how-and-why-you-should-use-next-js-django/
- https://www.billprin.com/articles/why-i-ditched-django-for-nextjs
Featured Images: pexels.com