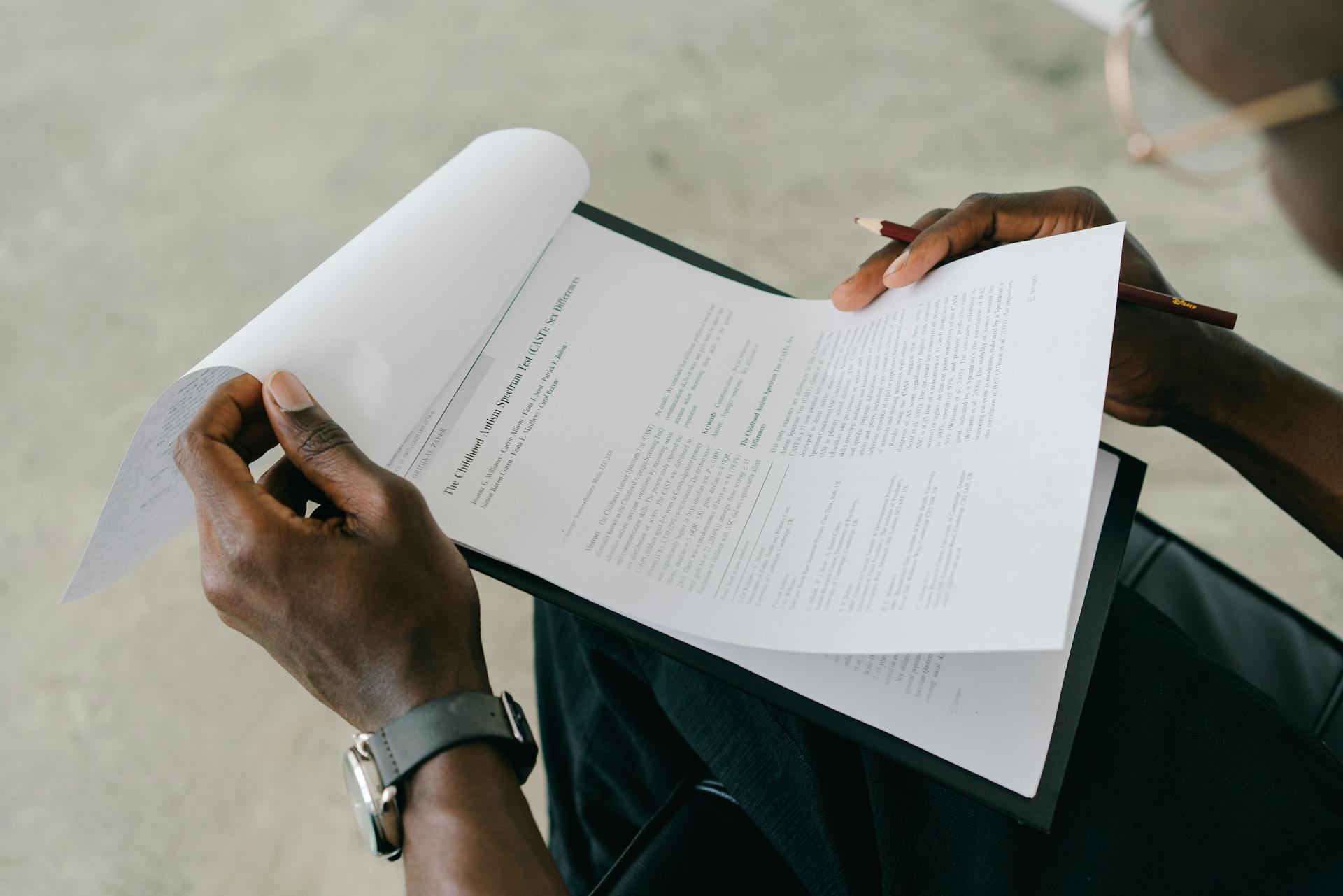
Simulating a "Choose File" HTML element can be a bit tricky, but don't worry, it's achievable with the right approach.
To start, you can use the HTML5 input type "file" to create a file input element. This is the standard way to allow users to select files on most modern browsers.
By using the input type "file", you can also leverage its built-in features, such as the ability to display a file name, size, and type. This can be particularly useful for user feedback and validation purposes.
One key consideration when simulating a "Choose File" HTML element is to ensure that the input element is properly styled to match the desired design.
If this caught your attention, see: Text Type in Html
Input Type
There are several types of input fields that can be used for the file input, including text, password, and hidden fields.
A text input field can be used to create a basic file input, but it's not ideal as it can be easily bypassed by users.
Using a hidden input field can be a good alternative, as it can be used to store the file path of the selected file.
In HTML, the type attribute of the input element is used to specify the type of input field, and for the file input, it's set to "file".
The accept attribute of the input element can also be used to specify the types of files that can be selected, such as images, videos, or audio files.
A file input can also be styled using CSS to make it look more like a button or a link, which can make it more user-friendly.
For your interest: Html File Type
Customizing the File Input
You can customize the file input by adding features to its appearance when not in plain mode. This can be done through various methods.
To globally change the Browse label, you can add custom styles to your global stylesheets, or use the browse-text prop to set the content of the custom file browse button text. Only plain text is supported, not HTML or components.
Check this out: Html Video File Not Found
By hiding the default HTML file upload button and styling the label element, you can create a custom file upload button that looks and works like the original. This can be achieved by adding the hidden attribute to the tag and styling the label element to look like a button.
If this caught your attention, see: Html Text Element
Customize Browse Button Label
You can globally change the Browse label by adding custom styles to your global stylesheets. This is a good approach for multi-language sites, and you can use the :lang() function to make it work.
To change the label, you can add something like this to your global stylesheets. This will update the label for all file input fields on your site.
Alternatively, you can use the browse-text prop to set the content of the custom file browse button text. Note that only plain text is supported, and HTML and components are not allowed.
By using the browse-text prop, you can customize the label for a specific file input field. This can be useful if you want to have different labels for different fields.
Limiting File Types
Limiting File Types is a crucial aspect of customizing the file input. You can limit the file types by setting the accept prop to a string containing the allowed file type(s).
To specify more than one type, separate the values with a comma. For example, if you want to accept both image and video files, you can set the accept prop to "image/*, video/*".
Not all browsers support or respect the accept attribute on file inputs, so you may need to consider alternative solutions. BootstrapVue uses an internal file type checking routine for drag and drop, which filters out files that don't have the correct IANA media type or extension.
For another approach, see: Itext Insert Image in Header File from Html
JavaScript and File Upload
JavaScript is a powerful tool that can enhance the input element and display required information in a smart and pleasant way. Our toolbelt is indeed rich and powerful, making it easy to use JavaScript to rescue our fake widget.
Using a little bit of JavaScript can display the user selection in our fake widget. The following snippet can be used to display the user selection:
After a user has chosen a file from her local filesystem, the input should preview the selection.
A fresh viewpoint: External Javascript File Html
File Selection and Management
When selecting files for simulation, it's essential to understand the different types of files that can be used.
The "HTML File Input" section explains that the HTML file input element allows users to select files from their device.
To manage files effectively, it's crucial to consider the "Multiple File Selection" feature, which allows users to select multiple files at once, as mentioned in the "Multiple File Selection" section.
This feature can be particularly useful when dealing with large collections of files, such as images or documents.
Single File
In single file mode, the v-model is null by default. This indicates that no file is selected.
If a file is selected, the return value is a JavaScript File object instance. You can work with this object to access the file's metadata and contents.
On single file mode, if the user cancels the "Browse" dialog, the v-model remains null. This is a key difference between single file and other modes.
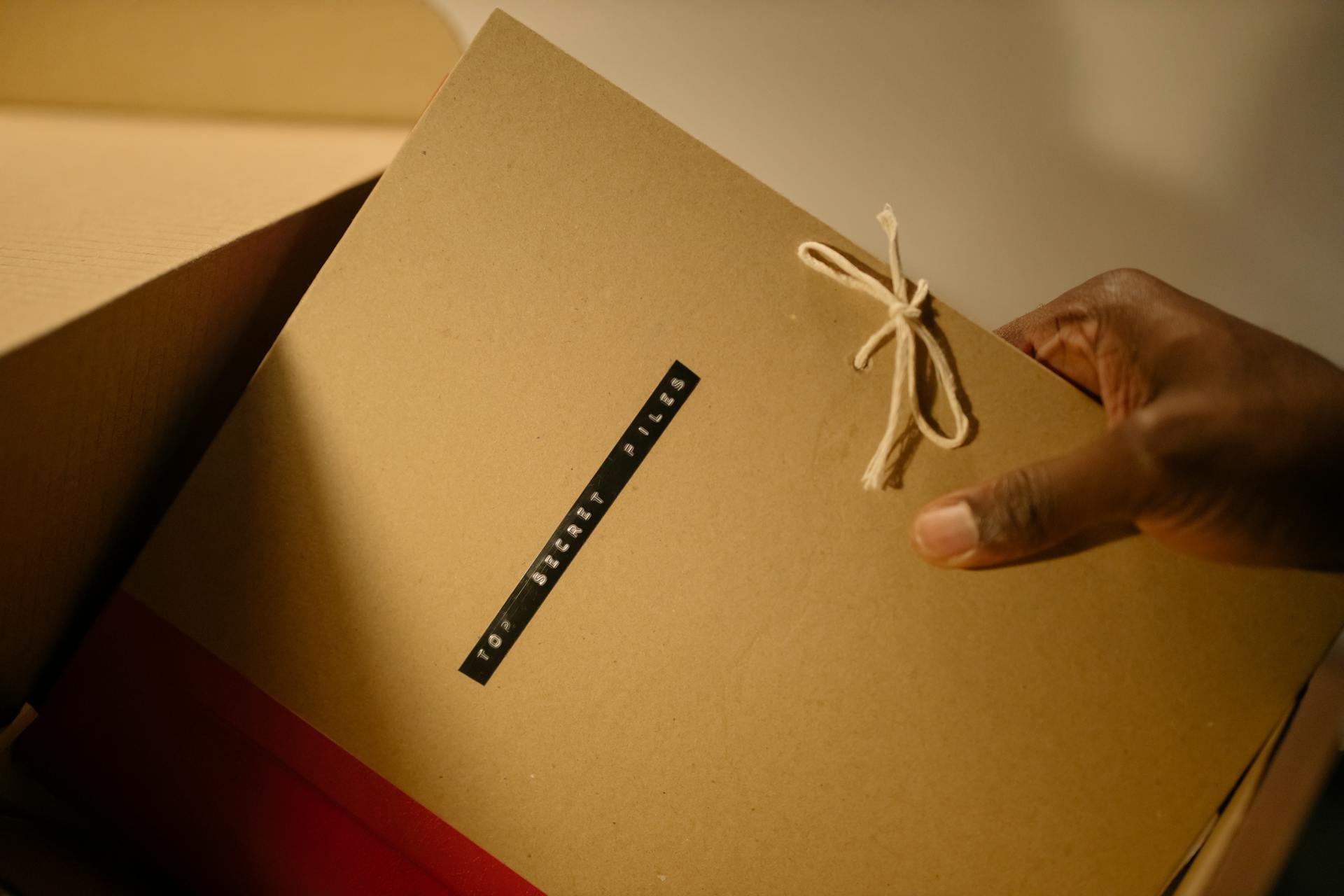
In this mode, you can use the File object to get the file's name, size, and other properties. This can be helpful for displaying file information to the user.
Single file mode is a great choice when you only need to select a single file at a time. It's simple and easy to use, and it gets the job done.
Clearing File Selection
Clearing File Selection is a common requirement when working with file inputs. You can clear the file selection by setting the v-model to null for single mode or an empty array [] for multiple/directory mode.
In some cases, you might need to use a reset() method to clear the file input. This method is provided by the
To use the reset() method, you'll need to obtain a reference to the
Additional reading: Html Form File Upload
<b-form-file>
The
To use the
Here are some of the key properties of the
By using these properties, you can customize the behavior of the
Style Label and Hide Default Button
To style the label element and hide the default HTML file upload button, add the hidden attribute to the tag. This effectively hides the default button in the browser.
We then style the label element to look more like a button. By doing so, we create a custom button that works like the original file upload button.
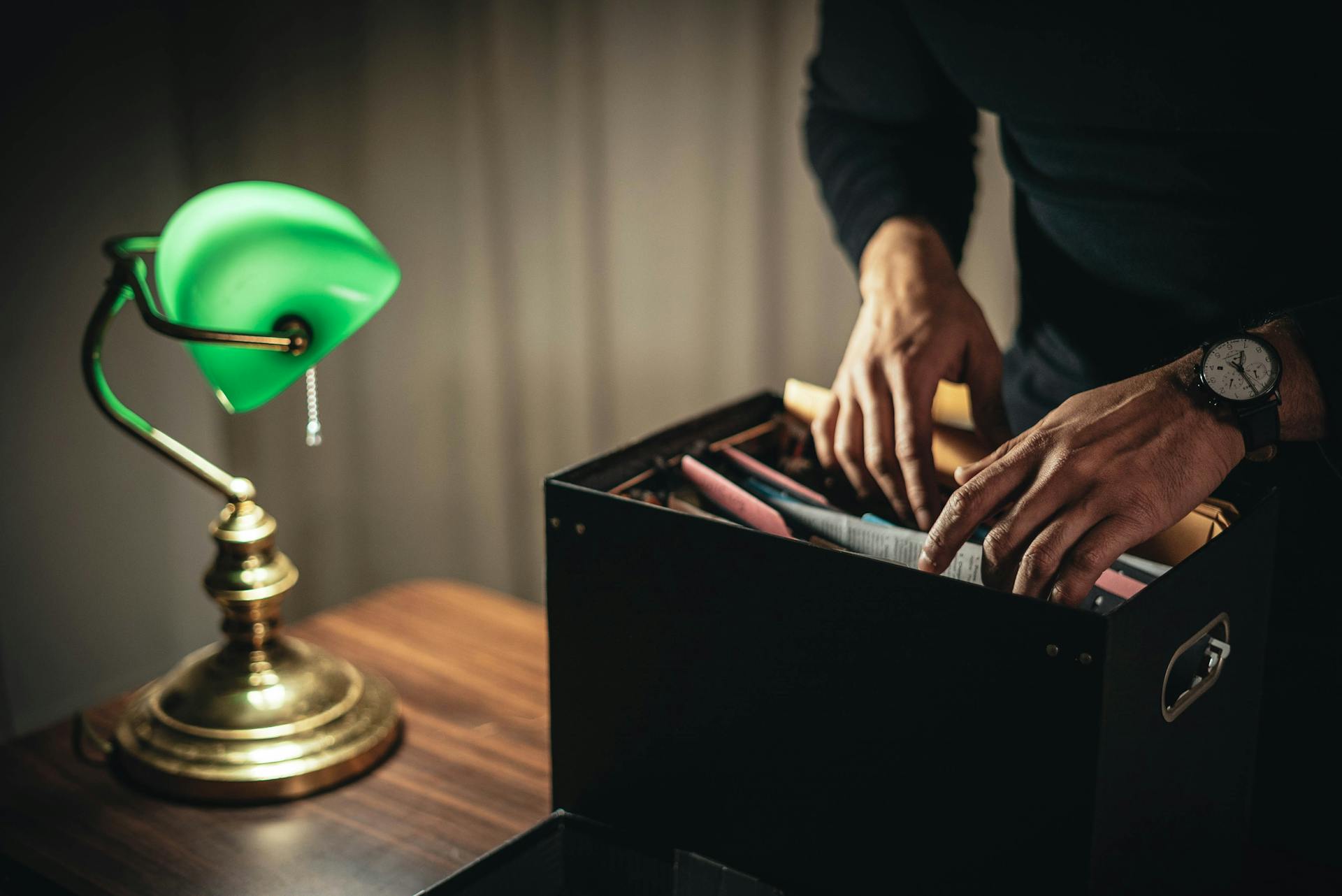
To display the name of the file being uploaded, include a span tag with an id of file-chosen right after the custom file upload button. This allows us to see the name of the file being uploaded.
In the JavaScript file, listen to the change event on the original file upload button, which we've hidden. This returns a file object containing details like name and file size.
Properties
The
The accept property is used to set the value on the file input's accept attribute, and it defaults to an empty string. This means you can use it to specify the types of files that can be uploaded, such as images, videos, or documents.
You can also use the autofocus property to attempt to auto-focus the control when it is mounted, or re-activated when in a keep-alive. This property is a boolean value that defaults to false.
The browse-text property is used to set the text content for the file browse button, and it defaults to 'Browse'. This means you can easily change the text that appears on the button to something more descriptive or user-friendly.
The directory property is a boolean value that enables directory mode on browsers that support it. This property defaults to false, which means it's not enabled by default.
Here's a summary of the properties:
The multiple property is a boolean value that allows multiple files to be selected, and it defaults to false. This means if you set it to true, the v-model will be an array of files.
The name property is used to set the value of the name attribute on the form control, and it defaults to an empty string. This means you should set it to a unique value to identify the form control.
The no-drop property is a boolean value that disables drag and drop mode, and it defaults to false. This means if you set it to true, users won't be able to drag and drop files into the form control.
The placeholder property is used to set the placeholder attribute value on the form control, and it defaults to 'No file chosen'. This means you can easily change the text that appears in the form control when no file is selected.
A different take: Html Drag Drop File Upload
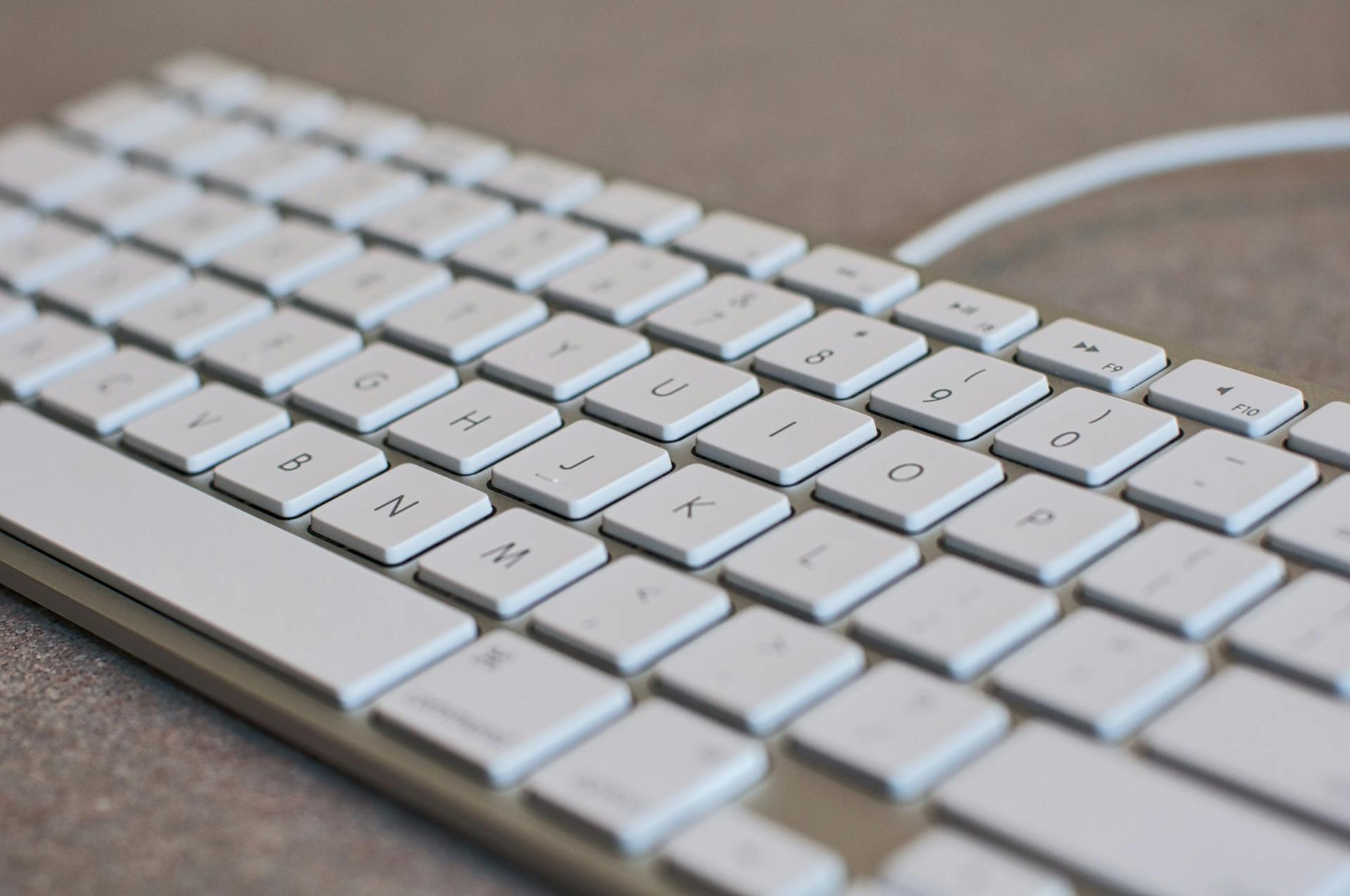
The plain property is a boolean value that renders the form control in plain mode, rather than custom styled mode, and it defaults to false. This means if you set it to true, the form control will have a more basic appearance.
The required property is a boolean value that adds the required attribute to the form control, and it defaults to false. This means if you set it to true, the form control will be marked as required.
The size property is used to set the size of the component's appearance, and it defaults to an empty string. This means you can set it to 'sm', 'md', or 'lg' to change the size of the form control.
The state property is a boolean value that controls the validation state appearance of the component, and it defaults to null. This means you can set it to true for valid, false for invalid, or null for no validation state.
The value property, or v-model, is used to set the current value of the file input, and it defaults to null. This means you can set it to a single File object or an array of File objects (if multiple or directory is set).
Properties and Behavior
The file input property "accept" is a string that determines which file types are allowed to be selected. You can set it to a specific file type, such as "image/*" for images, or leave it blank for no restrictions.
The "autofocus" property is a boolean that attempts to auto-focus the control when it's mounted or re-activated. This doesn't set the "autofocus" attribute on the control, so it's not a visual cue.
The file input has a "browse-text" property that sets the text content for the file browse button. By default, it's set to "Browse".
You can enable the "directory" mode by setting the "directory" property to true. This allows the user to select a directory of files, but it's only supported in certain browsers.
The "disabled" property is a boolean that disables the component's functionality and places it in a disabled state. This is useful when you want to prevent the user from selecting files.
Additional reading: Html File Upload Multiple Files
Here's a summary of the properties that affect the file input's behavior:
The "multiple" property is a boolean that allows multiple files to be selected. If set to true, the "v-model" will be an array of file objects.
The "name" property sets the value of the "name" attribute on the form control. You can use this to identify the file input in your form data.
The "no-drop" property is a boolean that disables drag and drop mode. This is useful when you want to prevent the user from dragging and dropping files into the file input.
The "no-drop-placeholder" property sets the text to display as the placeholder when files are being dragged and it isn't allowed to drop them. By default, it's set to "Not allowed".
The "no-traverse" property is a boolean that determines whether to return files as a flat array when in "directory" mode.
The "placeholder" property sets the "placeholder" attribute value on the form control. By default, it's set to "No file chosen".
The "plain" property is a boolean that renders the form control in plain mode, rather than custom styled mode.
The "required" property is a boolean that adds the "required" attribute to the form control.
The "size" property sets the size of the component's appearance. You can choose from "sm", "md" (default), or "lg".
The "state" property controls the validation state appearance of the component. You can set it to true for valid, false for invalid, or null for no validation state.
The "value" property, also known as "v-model", sets the current value of the file input. It can be a single file object or an array of file objects, depending on the "multiple" or "directory" properties.
Frequently Asked Questions
How to add choose file option in HTML?
To add a "choose file" option in HTML, use the tag. Adding the
How to select a file using JavaScript?
To select a file using JavaScript, use the `` element, which is supported in every major browser and allows users to select files using their operating system's built-in file selection UI. This element can also be used to select multiple files by adding the `multiple` attribute.
How to test file upload in React Testing Library?
To test file upload in React Testing Library, use the `upload` method from `@testing-library/user-event`, passing the input element and a File object as arguments. This allows you to simulate file uploads in your test cases with ease.
Sources
- https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file
- https://dev.to/faddalibrahim/how-to-create-a-custom-file-upload-button-using-html-css-and-javascript-1c03
- https://medium.com/@emanuele.bertoldi/lets-fix-that-ugly-html5-file-input-81c3f1d8ec78
- https://uploadcare.com/blog/how-to-upload-files-using-js/
- https://bootstrap-vue.org/docs/components/form-file
Featured Images: pexels.com