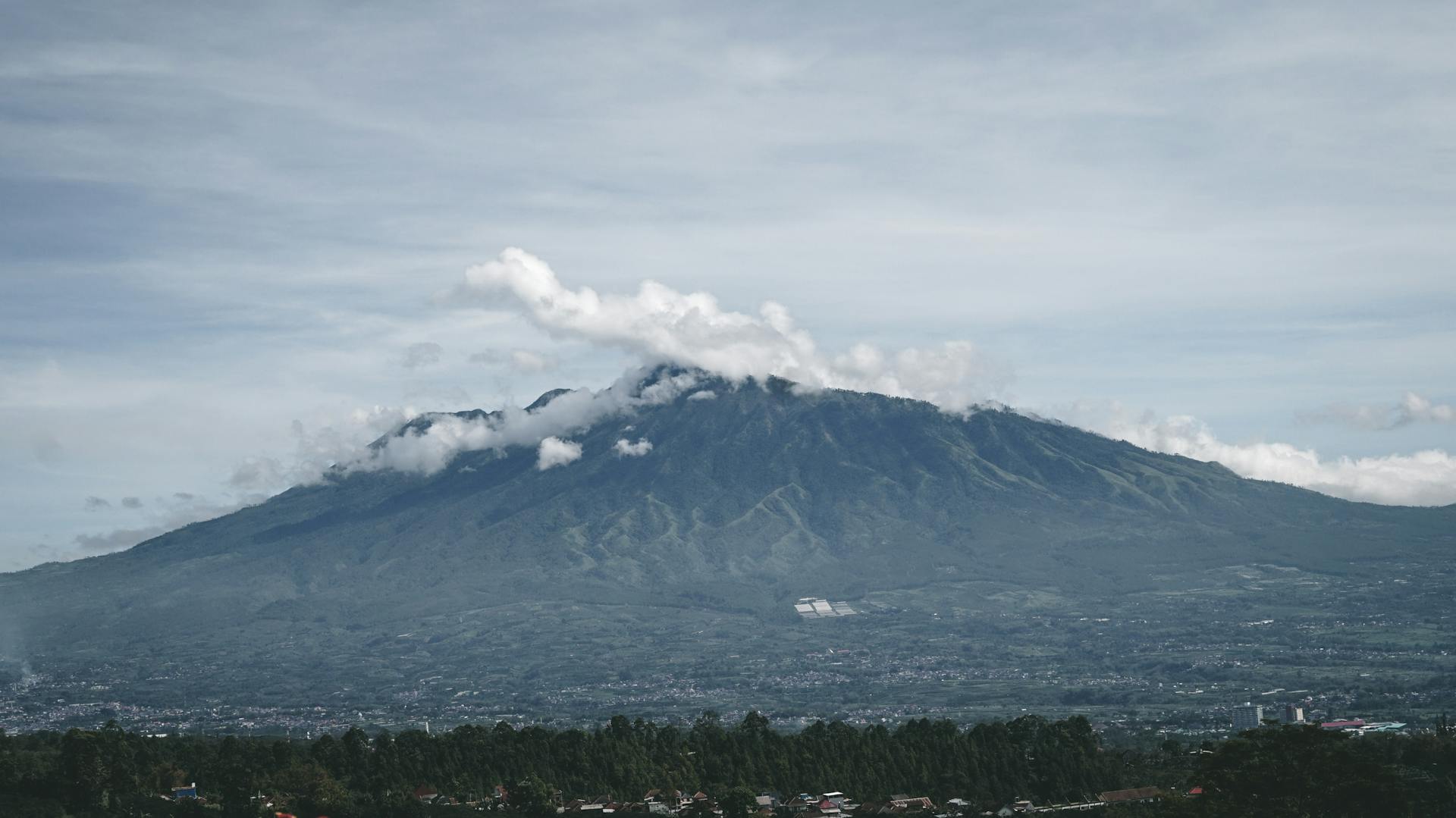
To get started with Java Google Cloud Storage, you'll need to create a project in the Google Cloud Console. This will give you access to the Cloud Storage API.
You'll then need to enable the Cloud Storage API for your project. This will allow you to interact with Cloud Storage using the Java SDK.
Next, you'll need to install the Google Cloud SDK on your machine. This includes the Java SDK, which you'll use to interact with Cloud Storage.
Once you have the SDK installed, you can start using the Java client library to interact with Cloud Storage. This library provides a simple and intuitive way to access Cloud Storage from your Java application.
For your interest: Do I Need Onedrive on My Android Phone
Uploading and Managing Files
Uploading files to Google Cloud Storage is a straightforward process. You can create a file, such as a text file called sample.txt, with contents like "hello world".
To upload a file, you can use Java code in your App class, and then check the GCP console to see if the file was uploaded successfully. The generation number of your file will change each time you upload a file to the same object location.
You can also update files that are already present in your bucket by running the same code and changing the contents of the file. The MD5 hash will only change if the contents of the file itself have changed.
Intriguing read: Google Drive Qr Code Generator
Uploading and Managing Files
Uploading files is a breeze with Cloud Storage. You can create a sample file to upload, like a text file called sample.txt with the text "hello world".
To upload a file, you'll need to write Java code in your App class. This code will send the file to your Cloud Storage bucket. You can then check the GCP console to see if the file was uploaded successfully.
You can also update files that are already present in your bucket. Simply change the contents of the file you're uploading, and the new version will replace the old one. Every object in a GCS bucket has a generation number, which keeps track of different versions of the same file.
The generation number will change each time you upload a file to the same object location. The MD5 hash, on the other hand, will only change if the contents of the file itself have changed.
For your interest: Object Storage Google
If you're using a servlet to handle file uploads, you can get the file chosen by the user and upload it to Cloud Storage using a specific code. Don't forget to include the @MultipartConfig annotation to handle file uploads.
Once you've uploaded a file, you can get its URL using the Blob class. This URL can be used in HTML to display an image or a download link.
Here's a summary of the steps to create a Java code file for uploading and managing files:
- Set APPLICATION_NAME to a unique identifier for your application.
- Set BUCKET_NAME to the name of a bucket in the same project as your client ID.
By following these steps and using the right code, you'll be able to upload and manage files with ease in Cloud Storage.
Deleting Files
Deleting files from your bucket is a straightforward process that can be done by calling the blob.delete() method. This method will throw an exception if you try to delete a file that doesn't exist, so make sure to check if the file exists before trying to delete it.
Cloud storage uses a flat namespace, which means that folders don't actually exist and all files are stored directly within the bucket. This can sometimes make it tricky to keep track of your files, but it's worth getting used to.
You might like: Google Cloud Storage Bucket
Blobstore and Folders
When you upload a file to Google Cloud Storage, you can use a folder-like structure, but it's actually just a part of the file name, not a real folder. This is because the Cloud Storage API gives us an illusion of a traditional file system for convenience.
You can list files within a folder using the storage.list method, but you need to pass the correct options to get the result in the desired format. The options you pass are required to get the result in this format: BlobListOption.prefix("") - this is the prefix that we want to list files for.BlobListOption.currentDirectory() - this means that we only want to list files in the current directory.
If you want to list the files within a specific directory, you can pass the directory name as the prefix, like "my_folder/" to get the files within the my_folder directory.
Readers also liked: List of Web Service Protocols
The Blob Instance
The Blob class represents an object stored in our bucket, which we'll be using to interact with our files on GCS.
This class is essential for almost all operations dealing with existing files, such as downloading, deleting, or reading contents.
To interact with a file, we first need to get the Blob instance for the file that we want to deal with.
A fresh viewpoint: Golang Azure Blob Storage
How Folders Work
You can still upload a file to a folder, but the path is just a part of the file name and doesn't actually create any folder structure. This is done by adding a folder prefix to the file name, like "my_folder/sample.txt".
The Cloud Storage API gives us an illusion of a traditional file system for convenience, allowing us to list files within a folder. This is done using the storage.list method.
To list files in a folder, you need to pass the correct options to the storage.list method. Here are the options you need to pass:
- BlobListOption.prefix("") - this is the prefix that you want to list files for, such as an empty string to list all files in the root directory.
- BlobListOption.currentDirectory() - this means you only want to list files in the current directory, not in subdirectories.
If you want to list files in a specific folder, like "my_folder", you need to pass the correct prefix, like "my_folder/". This will give you the files in that folder, but not in subdirectories.
Authentication and Access
Authentication with Google Cloud Storage requires you to tell the Cloud Storage library who you are. You can do this by creating a JSON credentials file and setting your GOOGLE_APPLICATION_CREDENTIALS environment variable.
To use the GCS client library, you can either use the default GCS bucket, which provides an already configured bucket with free quota, or you must first activate a Cloud project for GCS.
Your project can access multiple buckets, and you need to create these upfront by clicking on Cloud Storage once your project is open in the Google Developers Console, or you can use the gsutil tool provided by GCS.
Take a look at this: How to Use Google One Vpn
Authentication
Authentication is a crucial step in securing your data and ensuring only authorized individuals can access it. To achieve this, you need to tell the Cloud Storage library who you are.
You can view the objects in your bucket, but only you can upload new files to it. This is because anyone can view the objects in your bucket, but only you can upload new files to it.
To authenticate, you need to create a JSON credentials file. Follow these steps to create a JSON credentials file and set your GOOGLE_APPLICATION_CREDENTIALS environment variable.
Worth a look: Google Cloud Bucket How to Store Index File
ACLs and the Client Library
The Google Cloud Storage (GCS) client library doesn't allow you to change the bucket ACL, but it does let you specify an ACL for the objects you create.
This means you can control access to the objects your app creates, even if you can't control access to the entire bucket.
The available ACL settings are described in the documentation for the GcsService.FcsFileOptions object.
You can use these settings to grant read, write, or full access to objects your app creates, depending on the needs of your project and the users who will be accessing the data.
Here are the available ACL settings:
If you don't specify an ACL, the object will use the default ACL set by the bucket owner, which is public-read, meaning anyone with access to the bucket can read the object.
Sources
- https://www.sohamkamani.com/java/google-cloud-storage/
- https://happycoding.io/tutorials/google-cloud/cloud-storage
- https://download.huihoo.com/google/gdgdevkit/DVD1/developers.google.com/appengine/docs/java/googlecloudstorageclient.html
- https://download.huihoo.com/google/gdgdevkit/DVD1/developers.google.com/storage/docs/json_api/v1/json-api-java-samples.html
- https://firebase.google.com/docs/storage
Featured Images: pexels.com