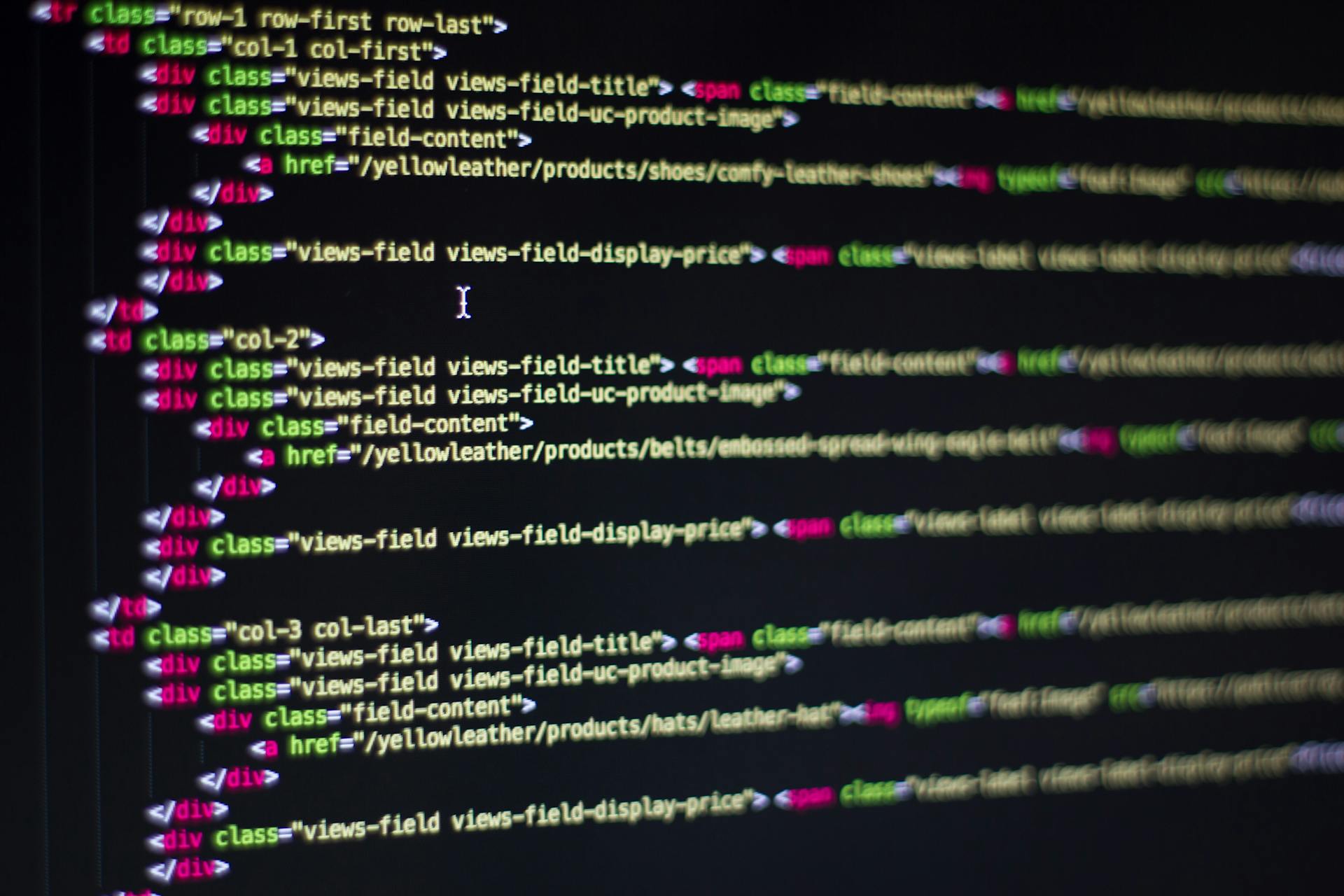
Learning Java programming is a fantastic way to create web applications, and it's a great skill to have as a beginner. Java is an object-oriented language that's widely used for developing web applications.
Java is known for its platform independence, which means your Java code can run on any device that has a Java Virtual Machine (JVM) installed. This is a huge advantage when it comes to developing web applications that need to be accessed from multiple platforms.
Java's vast ecosystem of libraries and frameworks makes it an ideal choice for web development. The Java Servlet and JavaServer Pages (JSP) technologies are two of the most popular frameworks used for building web applications.
If this caught your attention, see: Can Nextjs Be Used on Traditional Web Application
Java Technologies
Java Technologies play a crucial role in building robust web applications. Apache Tomcat is one of the most widely used Java web servers and servlet containers, providing a reliable platform to deploy and manage Java web apps.
For building Java-based web applications, Spring Boot is a popular choice due to its simplicity and ease of use. It follows the principles of the Spring framework, minimizing configuration and boilerplate code. Spring Boot focuses on convention over configuration, allowing developers to quickly create standalone, production-ready applications.
Here are some key Java technologies:
- Apache Tomcat: Java web server and servlet container
- Spring Boot: Simplifies development of Java-based web applications
- JavaServer Faces (JSF): Component-based web framework for user interfaces
- Maven and Gradle: Build automation tools for managing dependencies and project configurations
These technologies are essential for building efficient and scalable web applications in Java.
Technologies to Use
Java is a versatile language with a wide range of technologies to choose from. Apache Tomcat is one of the world's most widely used Java web servers and servlet containers.
For Java web development, you'll want to consider using Spring Boot, which simplifies the development of Java-based web applications. It follows the principles of the Spring framework while minimizing configuration and boilerplate code.
JavaServer Faces (JSF) is a component-based web framework that simplifies the development of user interfaces for Java web applications. It provides a set of reusable UI components and a robust event-driven programming model.
Check this out: Web App Programming Language
Maven and Gradle are popular build automation tools used in Java web development. They simplify the build process, manage dependencies, and facilitate project management.
Here are some key features of these technologies:
Java is also known for its platform independency, meaning it's a "Write Once, Run Anywhere" (WORA) language. The compiled code can run on any machine that supports the Java Virtual Machine (JVM).
Language History
Java was launched in 1991 by James Gosling and his team at Sun Microsystems.
Java was offered to the public in 1995 for usage in various applications.
It's a top three programming language in the world for backend development, according to learncodewith.me.
In 2022, Java was ranked first among the top ten programming languages.
Generics
Generics are an important concept in Java programming that allows us to create classes and methods that work with different data types.
Generics were added to Java in version 5 and have become widely used ever since. By using generics, we can create collections, like lists or maps, that can store different types of objects.
One of the main benefits of generics is type safety, which means we can catch errors at compile time instead of at runtime. The compiler checks if we are using the correct types when working with generic classes or methods, reducing the chances of bugs occurring in our code.
Generics help improve code readability by making it clear what kind of data a class or method is expecting. This makes our code more maintainable and easier to understand for other developers.
You might like: Azure Rgb
Backend Development
Backend development is a crucial part of building web applications, and Java is a popular choice for this task. Java's use cases have grown to include data science applications, machine learning applications, and even IoT applications.
Java has strong support for web development, and it's frequently used at the server side. A Java web application is a collection of dynamic resources and static resources, which can be deployed as a WAR (Web ARchive) file.
Some popular Java web frameworks are GWT, JavaServer Faces, Struts, and the Spring framework. These frameworks usually require a minimum container, such as a web container, to execute Java servlets and JavaServer Pages.
Here are some top companies that use Java for backend development:
Java Servlets are an essential technology for web application development in Java, handling requests from web browsers and creating dynamic websites.
Struts
Struts is a free and open-source web application framework used to create Java EE web applications. It uses and improves the Java Servlet API to encourage developers to use a model-view-controller paradigm.
Craig McClanahan designed Struts and presented it to the Apache Nation. This framework is often used to build complex web applications that require a high level of scalability and maintainability.
Struts provides a lot of built-in functionality, including support for internationalization, validation, and exception handling. It also provides a lot of tools and utilities to make development easier.
Some of the key features of Struts include:
- Model-View-Controller (MVC) architecture
- Internationalization and localization support
- Form validation and processing
- Exception handling and logging
- Support for Java Servlet API
Overall, Struts is a powerful and flexible framework that can help developers build complex and scalable web applications in Java.
Benefits of Backend Development
Java's use cases have now grown to include data science applications, machine learning applications, and even IoT applications. This versatility makes Java a great choice for backend development.
Java's platform independence allows it to run on any device, making it an ideal choice for developing scalable backend infrastructure.
Java's extensive libraries and tools support a wide range of backend development tasks, from database management to web services.
Java's strong focus on security features ensures that backend applications are protected from common threats and vulnerabilities.
Java's large community of developers means there are plenty of resources available to help with any issues that may arise during development.
You might enjoy: Backend Web Programming
Scalability and Robustness
Java's robust type-checking mechanism makes it durable, allowing it to handle complex applications with ease. This is a key benefit of using Java for backend development.
Java's automatic memory management and disposal collection make it highly scalable, enabling developers to speed up web application development. This is particularly useful for large-scale applications.
The Java Virtual Machine (JVM) enables dynamic linking and a secure environment, allowing Java to run anywhere. This is a significant advantage over other programming languages.
Java's automatic memory management and disposal collection make it highly scalable, allowing developers to focus on writing efficient code. This can result in faster development times and more efficient applications.
The JVM's secure environment provides an additional layer of protection against memory-related issues, making Java a more reliable choice for backend development. This is especially important for applications that require high uptime and stability.
Multithreading
Multithreading is a powerful technique that allows your code to run faster and more efficiently by executing multiple tasks simultaneously.
Multithreading is an advanced concept in Java programming that allows for the concurrent execution of multiple threads.
In simpler terms, multithreading means running two or more tasks simultaneously within a program.
Different parts of your code can be executed simultaneously, making your program faster and more efficient.
You might enjoy: Web Design Referral Program
Java provides built-in support for multithreaded programming, making it easier for developers to implement this functionality.
Threads are lightweight processes within a program that can perform tasks independently.
They can execute different functions concurrently or share resources to accomplish a common goal.
Understanding concurrency and parallelism is essential when working with multithreading in Java.
Concurrency refers to the ability of different tasks to run independently but not simultaneously.
Parallelism involves executing tasks simultaneously by utilising multiple processors.
Networking
Networking is a crucial aspect of backend development, and Java is particularly well-suited for this task.
Java offers advanced networking techniques and libraries for secure and efficient data transmission, making it an ideal choice for developing network-centric applications.
Developing email applications and facilitating communication between networked devices are just a couple of examples of what Java can do.
Java can be used for server-side development through technologies like RMI and CORBA, enabling distributed computing and remote method invocation.
These technologies allow developers to create robust and scalable backend systems that can handle complex networking tasks.
Readers also liked: Web Dev Technologies
Top Companies
LinkedIn's application is primarily written in Java, with some C++ parts thrown in for good measure.
The United States leads among enterprises that use Java, accounting for more than 60% of Java clients, with about 64,000 businesses.
Uber works with a large amount of real-time data, tracking drivers and incoming ride requests, which Java helps to sift through and match users swiftly.
Microsoft uses Java for various purposes, including building its proprietary Edge web browser.
Netflix primarily uses Java for practically everything, which is a testament to its reliability and scalability.
Java is used by many other tech titans, including Airbnb, Google, eBay, Spotify, TripAdvisor, Intel, Pinterest, Groupon, Slack, Flipkart, and many more.
Database and Storage
JDBC is a Java API that provides a standard way to interact with relational databases.
To establish a connection to a database using JDBC, several steps need to be followed, including loading the appropriate JDBC driver and creating a connection by calling the DriverManager.getConnection() method.
The DriverManager.getConnection() method requires the database URL, username, and password as parameters, and the URL typically follows the format jdbc:subprotocol:subname.
Here's a breakdown of the steps to execute queries and update data in a database using JDBC:
- Create a statement object using the Connection.createStatement() method
- Use the executeQuery() method to execute SQL queries against the database
- Use the executeUpdate() method to execute SQL statements that modify the data
Database integration is a crucial part of developing web applications with Java, allowing you to connect your application to a database and store data securely.
Database Integration
Database integration is a crucial part of developing web applications with Java. It allows you to connect your application to a database and store data securely.
To connect to a database using JDBC, you need to load the appropriate JDBC driver using the Class.forName() method. This method dynamically loads the driver class into memory.
The driver class name depends on the specific database you are working with. For example, if you're working with a MySQL database, the driver class name would be com.mysql.cj.jdbc.Driver.
To establish a connection, you need to create a connection by calling the DriverManager.getConnection() method. This method requires the database URL, username, and password as parameters.
Here are the basic steps to connect to a database using JDBC:
- Load the JDBC driver using Class.forName()
- Create a connection using DriverManager.getConnection()
- Execute queries and update data using the Connection.createStatement() method
Remember to handle exceptions that may occur during database operations using try-catch blocks to catch and handle SQLExceptions.
Memory Capacity
Memory Capacity is a crucial aspect to consider when it comes to database and storage systems. Java uses a significant amount of memory compared to other languages like C and C++.
This can lead to memory efficiency issues, causing system performance to suffer during cleanup execution.
Worth a look: C Programming Web Server
Advanced Concepts
In Java programming, advanced concepts are essential for building robust and scalable web applications.
Multithreading is a critical concept that allows developers to write efficient code that can handle multiple tasks simultaneously.
Networking is another vital concept that enables Java applications to communicate with other systems and devices over the internet.
File handling is a fundamental concept that allows developers to read and write data to files and directories.
Generics are a powerful feature in Java that enable developers to write reusable and type-safe code.
Reflection is a concept that allows Java applications to inspect and modify their own code at runtime.
If this caught your attention, see: Web Designers Code
Tools
When building a Java web application, you'll want to consider the tools that will make your job easier.
Apache Tomcat is a popular choice for running Java web apps, known for its simplicity and robust performance. It supports Java Servlets, JSP pages, and other Java technologies.
Spring Boot is a powerful framework that simplifies web application development, minimizing configuration and boilerplate code. It's a go-to choice among web developers.
For build automation, Maven and Gradle are two popular tools. Maven uses XML scripts, while Gradle uses a Groovy-based scripting language for a more flexible and customizable build system.
JavaServer Faces (JSF) is a component-based web framework that simplifies user interface development, following the Model-View-Controller (MVC) architecture.
Here are some of the tools you'll want to consider:
- Apache Tomcat
- Spring Boot
- JavaServer Faces (JSF)
- Maven
- Gradle
Understanding Architecture
The client-server model is the foundation of web application architecture, where the client sends requests to a server, which processes the requests and sends back the response.
The client is responsible for initiating the request and displaying the response to the user, and can be a desktop application, a mobile app, or a web browser.
Worth a look: Web Server Programming
In the client-server model, the client and server communicate using a network protocol, such as HTTP, which stands for Hypertext Transfer Protocol.
HTTP requests are composed of methods, such as GET, POST, PUT, and DELETE, which indicate the type of operation to be performed on the server.
The servlet lifecycle is a crucial concept in Java web development, where servlets are Java classes that handle requests and generate responses in a web application.
A servlet's lifecycle includes several methods, such as init(), service(), and destroy(), which are called by the web container at different stages of the servlet's lifetime.
The init() method is called when the servlet is first loaded and initialized by the container, and is typically used for one-time initialization tasks.
The service() method is called for each request and can be overridden to implement specific functionality, making it a key part of a servlet's lifecycle.
The destroy() method is called when the servlet is removed from the container, allowing the servlet to release any resources acquired during its lifetime.
Worth a look: Web Dev Services
Understanding the JSP lifecycle is also essential for Java web developers, where JSP stands for JavaServer Pages, a technology that allows developers to create dynamic web content using Java code embedded within HTML.
The JSP lifecycle consists of four phases: translation, compilation, initialization, and execution, which define the stages a JSP page goes through during its execution.
Worth a look: Hire Mern Stack Developers
Sources
- https://www.oracle.com/technical-resources/articles/java/webapps.html
- https://www.vogella.com/tutorials/JavaWebTerminology/article.html
- https://intellipaat.com/blog/java-web-development/
- https://www.freecodecamp.org/news/java-for-backend-web-development/
- https://profiletree.com/web-application-development-using-java/
Featured Images: pexels.com