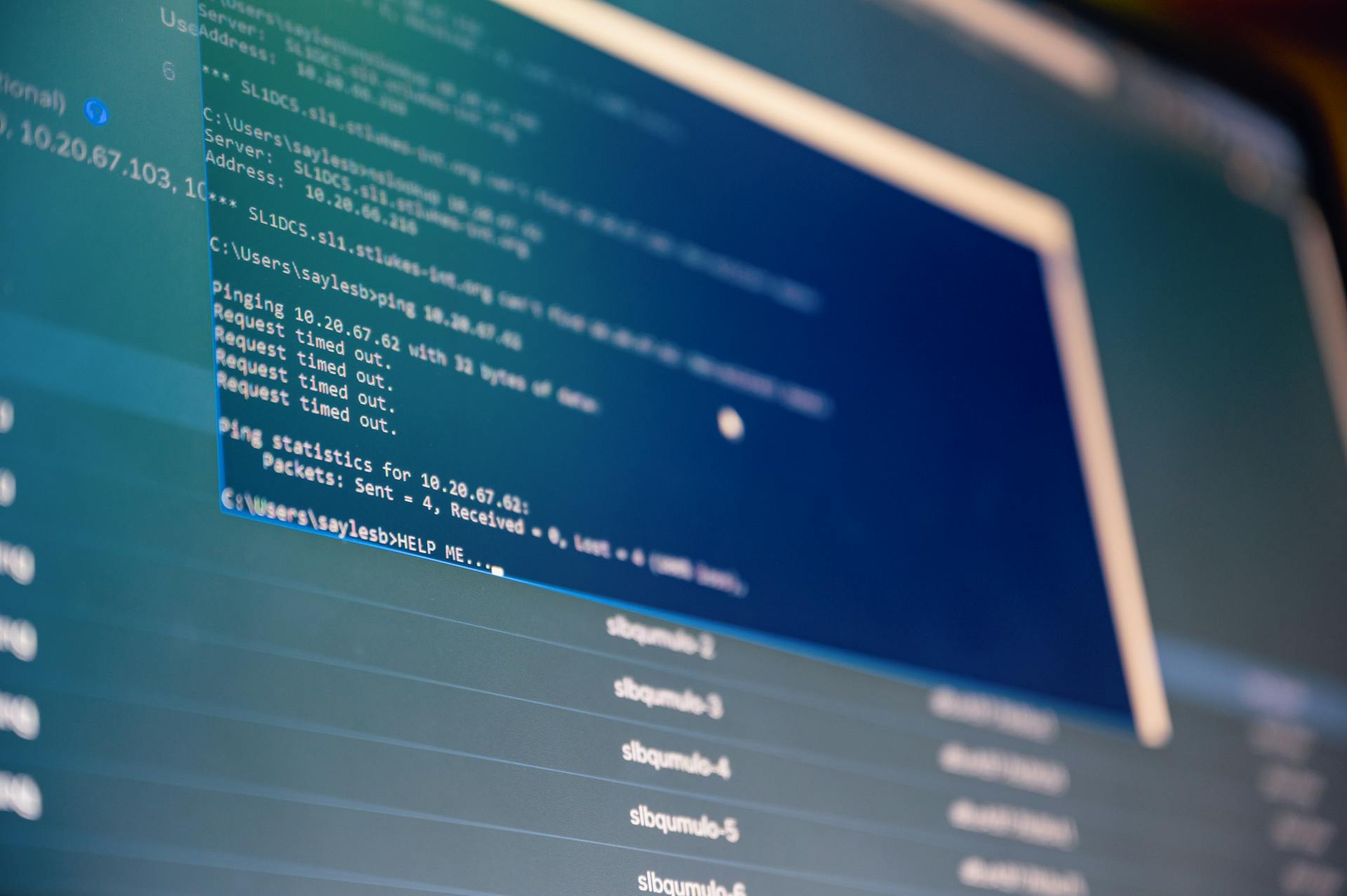
Web development technologies are constantly evolving, but some remain essential for building robust and scalable websites. JavaScript is a versatile language used for client-side scripting, with frameworks like React and Angular offering efficient solutions for complex applications.
Front-end development focuses on creating user interfaces, and CSS is a crucial tool for styling and layout. CSS preprocessors like Sass and Less can simplify and speed up the development process.
Back-end development, on the other hand, deals with server-side logic, and languages like Node.js and Python are popular choices for building robust APIs. Frameworks like Express.js and Django provide a solid foundation for server-side development.
Modern web development often involves working with a combination of these technologies to create seamless user experiences.
Frontend Frameworks
Frontend frameworks are essential for creating stunning websites, and your choice of framework is crucial. You should always select the best tool for the job.
React is a popular JavaScript library for building user interfaces, particularly single-page applications where fast interactions are critical. It uses a virtual DOM for performance optimization and supports server-side rendering.
Angular provides a structured approach to building dynamic web applications, incorporating features like two-way data binding, dependency injection, and a modular architecture. However, the learning curve can be steep, and the framework might be overkill for smaller projects.
Vue.js is a progressive JavaScript framework known for its simplicity and flexibility. It is particularly popular for developing user interfaces and single-page applications. Vue's core library focuses on the view layer only, making it easy to integrate with other projects or libraries.
Here are some key features of popular frontend frameworks:
- React: Virtual DOM, server-side rendering
- Angular: Two-way data binding, dependency injection, modular architecture
- Vue.js: Reactive data binding, component-based architecture
React.js
React.js is a popular JavaScript library for building user interfaces, particularly single-page applications where fast interactions are critical. It's maintained by Facebook and allows developers to create large web applications that can update and render efficiently in response to data changes.
React uses a virtual DOM for performance optimization and supports server-side rendering, making it a versatile choice for modern web development. This means developers can create complex web applications that update quickly and smoothly, without sacrificing performance.
React is also an interface-first way of using JavaScript, which makes it a great choice for developers who want to focus on building user interfaces. As Matt Mullenweg would say, it's essential to learn React.js deeply, especially for WordPress developers.
React's syntax extension, JSX, allows developers to create elements and render them to the Document Object Module (DOM) with ease. This makes it a strong choice for building complex user interfaces.
Here are some key features of React.js:
- Virtual DOM for performance optimization
- Server-side rendering
- Interface-first approach to JavaScript development
- JSX syntax extension for easy element creation
- Support for server-side rendering and static site generation
Overall, React.js is a powerful and versatile library that's well-suited for building modern web applications. Its ease of use, performance optimization features, and interface-first approach make it a great choice for developers of all levels.
CodePen or JSFiddle
CodePen or JSFiddle is a great tool for developers who want to showcase a particular element within a file and demonstrate where changes can be made. They offer an online IDE that can help you create better code.
You can name your "Pen" or "Fiddle" depending on your platform, and save it to share with others. This simple idea can help you bring your half-baked thoughts to life within seconds.
For developers who want to collaborate on a project, online IDEs like CodePen or JSFiddle offer chat or live mic features. This makes it easy to work together in real-time.
Overall, an online IDE is an invaluable tool for developers who want to create better code and collaborate with others.
CSS Frameworks
CSS frameworks are a game-changer for styling websites. They provide prebuilt components that make styling quicker and easier.
Bootstrap is the most popular CSS framework, offering tons of responsive layout tools. It's a great choice for developers who want to create stunning websites.
Tailwind CSS is another popular option, known for its "utility-first" approach. It allows developers to add CSS classes to HTML tags, making it easy to design the frontend of a site without leaving HTML.
The 960 Grid System is a good example of how Tailwind CSS can be used in conjunction with other frameworks. However, it's essential to use patience and discipline when working with Tailwind CSS, as it can lead to spaghetti code if not managed properly.
Here are some popular CSS frameworks to consider:
- Bootstrap
- Tailwind CSS
- Bulma
- Materialize
Materialize, for example, implements Google's Material Design principles, making it a great choice for developers who want to create modern and visually appealing websites.
Backend Frameworks
Backend frameworks play a crucial role in web development, providing a solid foundation for building scalable and efficient applications. They simplify development by offering pre-built functionality and tools, allowing developers to focus on writing code that meets the application's specific needs.
Node.js is a popular choice for building scalable web applications, leveraging its non-blocking, event-driven architecture to handle multiple concurrent connections. Its vast ecosystem of packages through npm makes development speed and code reuse easier.
Here are some key benefits of popular backend frameworks:
- Django, a high-level Python web framework, encourages rapid development and clean design, making it a preferred choice for backend development.
- ASP.NET Core offers a modular architecture, making it flexible and scalable, and supports various development styles, including MVC, web APIs, and Razor Pages.
- Laravel provides an elegant syntax, simplifies database interactions, and offers a powerful templating system, making it a popular choice among PHP developers.
- Spring Boot reduces configuration overhead, runs applications with embedded web servers, and offers tools for monitoring and managing applications in production.
Node.js
Node.js is a runtime environment that allows developers to run JavaScript on the server-side, built on Chrome's V8 JavaScript engine. It's designed for building scalable network applications.
Node.js excels in handling concurrent connections, making it suitable for real-time applications. This is due to its event-driven, non-blocking I/O model.
Asynchronous I/O is a key feature of Node.js, allowing for non-blocking I/O operations. This makes it well-suited for handling multiple concurrent requests.
The Node Package Manager (npm) provides access to a vast repository of libraries and modules. This enhances development speed and code reuse.
Here are some key benefits of using Node.js:
- Asynchronous I/O: Node.js's event-driven architecture allows for non-blocking I/O operations.
- npm (Node Package Manager): A robust package ecosystem provides many libraries and modules.
- Scalability: Node.js is designed for scalability, making it a good fit for applications with a high volume of simultaneous connections.
Node.js is particularly advantageous for projects demanding real-time features and handling numerous concurrent connections. This is because it leverages a vast ecosystem of packages through npm and is designed for scalability.
Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It emphasizes reusability, less code, and the principle of “don’t repeat yourself” (DRY).
Django comes with a robust admin interface, which provides an automatic interface for managing application data. This feature is a huge time-saver, especially for developers who need to manage complex data sets.
The MTV Architecture is a key component of Django, separating concerns using Model, Template, and View. This architecture makes it easier to maintain and scale your application.
Django's ORM (Object-Relational Mapping) simplifies database interactions, making it easier to work with databases without writing raw SQL code. This feature is particularly useful for developers who are new to database management.
Django includes built-in security features, which protect against common web vulnerabilities such as SQL injection and XSS attacks. This provides an extra layer of protection for your application and its users.
Here are some key benefits of using Django:
- Encourages rapid development and clean design
- Emphasizes reusability and less code
- Includes a robust admin interface
- Simplifies database interactions with ORM
- Protects against common web vulnerabilities
Laravel
Laravel is a popular PHP framework that makes development more enjoyable and accessible with its elegant syntax.
One of the key benefits of Laravel is its Eloquent ORM, which simplifies database interactions and makes it easier to work with data.
Laravel's Blade Templating Engine is a powerful and easy-to-use templating system that helps you separate presentation logic from application logic.
Here are some of the key features of Laravel:
- Elegant Syntax: Makes PHP development more accessible and enjoyable.
- Eloquent ORM: Simplifies database interactions.
- Blade Templating Engine: Offers a powerful and easy-to-use templating system.
- Built-In Authentication: Provides tools for user authentication and authorization.
- Artisan CLI: Includes a command-line interface for managing the application.
Laravel is a full-stack framework that can be used for a wide range of projects, from local development environments to API backends for Next.js apps.
Laravel's Homestead is a Vagrant-based local development environment that helps you set up a development environment quickly and easily.
Laravel itself is a Docker-based PHP framework that uses a CLI called Sail to interact with it.
You can use Laravel to build up projects, even if you don't use much of the framework itself, and it's a central tool to your workflow as a PHP developer.
Privacy and Security
Privacy and security are non-negotiable aspects of any web development stack.
Regular updates are essential to ensure the chosen tech stack remains secure and up-to-date.
Strong encryption methods should be integral to the chosen tech stack to protect sensitive data.
A proactive approach to cybersecurity is crucial in today's threat landscape.
Opting for a stack prioritizing robust security features is paramount to safeguard your web application development.
Code Editors Visual Studio Code
Visual Studio Code is an open source code editor developed by Microsoft that offers enough in the box to be considered an IDE.
It's undoubtedly offering enough functionality to capture over half of the market — 55% of web developers use Visual Studio Code daily.
Visual Studio Code supports TypeScript, JavaScript, and Node.js out of the box, and its extension library makes it modular and flexible enough to meet any of your development needs.
You can install linters and fixers for your chosen language, along with Docker and Vagrant extensions, and much more.
The extension ecosystem is so rich that you'll be able to find something to support the language you're using.
Visual Studio Code also offers top-notch integration with other Microsoft products, most notably GitHub.
Design and Prototyping
Design and Prototyping is a crucial part of web development, and it's essential to have the right tools to get the job done. Figma is an oft-mentioned web development tool that lets you collaborate on design.
Figma's drag-and-drop editor makes it easy to build interfaces and user-facing elements, and developers can grab code snippets to implant into their projects. You can also get a homogenized set of tools to help with font and color choices, which can save you time and reduce revisions.
Figma's pricing starts at $12 per "editor" on the standard tier, which rises to $45 per "editor" for enterprise-based teams. This means the price could rise based on how many editors you'd like onboard.
InVision Studio is another popular design and prototyping tool that provides an intuitive layer-based editor and vector support. It's a "screen design" app that makes it easy to create animations and link artboards and screens together.
The free version of InVision Studio gives you almost all the functionality of the paid version, with only a restriction on the number of documents you can save. The Pro tier is valuable for the app's power, costing around $95/user/year.
Figma is particularly valuable for team projects, with features like a central asset repository and reusable components. Team leaders will also appreciate the comprehensive reporting options to see how the team members use the various design systems.
InVision Studio's shared component libraries, global syncing options, and robust Inspect mode make it easy for the entire team to work within the app. This can save you time and reduce errors in the design and prototyping process.
Version Control Systems
Version Control Systems are a must-have for any web development project. They help manage code changes across collaborators and keep a history of what happens.
Git is the most prominent Version Control System, invented by Linux creator Linus Torvalds. It uses a series of commands to add file changes to a "staging area" and then commits them to a repository.
The top version control tools are Git, GitHub, and GitLab. Git is a distributed VCS that lets developers host remote repositories, while GitHub is a popular cloud host for shared Git repositories. GitLab is an open-source alternative to GitHub.
Additional version control systems like Mercurial and Apache Subversion exist, but Git runs the show today. GitLab has a great beginner's guide to using Git on the command line for those new to VCSs.
Here are the top version control tools:
- Git – A distributed VCS to let devs host remote repositories
- GitHub – A popular cloud host for shared Git repositories
- GitLab – An open-source alternative to GitHub
Browsers
Browsers are a crucial part of web development, and most modern browsers include specific development tools to help you analyze backend code. You can use these tools to take back into your project and improve its performance.
The most popular browsers include Chrome, Firefox, and Safari, which rely on layout and JavaScript engines like Blink, Gecko, and WebKit to parse code and translate it to pretty pixels. Chrome uses the Blink rendering engine, while Firefox pioneered the Gecko layout engine.
Here are some fun browser facts:
- Chrome uses the Blink rendering engine
- Firefox pioneered the Gecko layout engine
- Safari adopted the WebKit engine, now called Blink
- Internet Explorer historically used Trident
- Microsoft Edge marks a switch to Blink
Chrome's DevTools are highly regarded for their stellar feature set and diagnostics, and many Chromium-based browsers have the same set of DevTools with similar shortcuts.
Chrome
Chrome is a popular choice for developers, thanks to its impressive set of developer tools. The Chrome Developer Tools, known as DevTools, are highly regarded for their feature set and diagnostics.
The Elements tab is a must-visit, where you can view the page source code. The Performance tab provides valuable insights into page load times, which can't be matched by other browser DevTools.
The Security tab offers useful information for monitoring or researching a client's website. You can also generate Google Lighthouse reports directly from your Chromium-based browser, thanks to Chrome's DevTools.
For beginners, the Console is a great place to start coding, as you can run JavaScript directly in the browser and test out snippets. It's an easy way to get started and find your feet when first learning to code.
Firefox
Firefox is a popular browser with a monthly active user base of around 220 million. It's still going strong despite Google's dominance.
Developers have praised Firefox's debugging capabilities, particularly with Firebug, which was a leader in its time. Firebug is no longer mentioned in the text, but we can see that Firefox has a strong following among developers.
Firefox Developer Tools have a core set of features, including the Inspector, a Debugger, Memory, Storage, and more. These tools are ideal for cross-browser debugging and troubleshooting.
Despite having less packed into its Developer Tools than Chromium-based browsers, Firefox is still a great choice for almost all tasks.
Can I Use...
Can I Use... is a game-changer for frontend developers. It's like having an Ask Jeeves for CSS, where you can type in a CSS selector or property and get instant feedback on whether it's supported by your target browser.
You can use it to check the compatibility of JavaScript and HTML elements as well, but it won't look up server-side languages like PHP or Python. It's a frontend language database, after all.
The service is incredibly valuable for accessibility and designing for multiple devices. It's a simple proposition: pull up a specific element and see at a glance whether your target browser supports it.
You can even find statistics like the release date for the element and a usage percentage on Can I Use... It's a treasure trove of information that can save you a lot of time and hassle.
It's worth noting that Can I Use... might not get regular use, but it's a lifesaver at certain stages of a project. Once you've figured out what your target browsers will support, it shuffles back into your bookmarks and waits for when it's next needed.
Frequently Asked Questions
What are the new dev technologies?
Discover the latest dev technologies, including Progressive Web Apps, AI Chatbots, and Serverless Architecture, that are revolutionizing the way we build and interact with digital products
What are the three types of web developers?
There are three main types of web developers: front-end, back-end, and full stack. Each type focuses on different aspects of website development, from user interface to underlying code and overall management.
What technology is used to build websites?
Websites are built using HTML (HyperText Markup Language) and CSS, which work together to structure and style web pages. Understanding these technologies is essential for creating engaging and user-friendly websites.
Featured Images: pexels.com