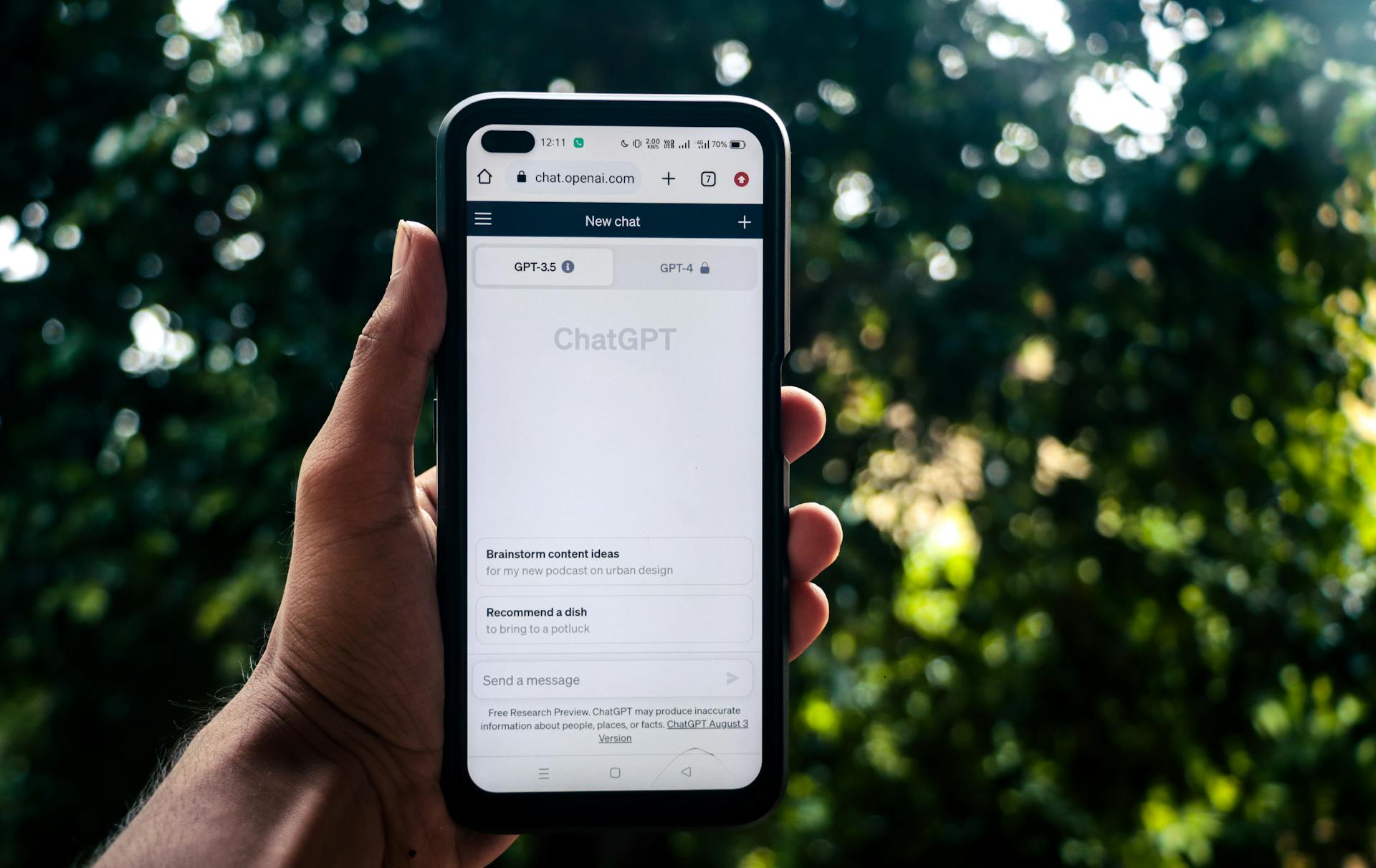
JQueryUI animate is a powerful tool that allows you to add animations to your web applications. It's a part of the JQueryUI library, which is built on top of JQuery.
With JQueryUI animate, you can create smooth and engaging animations that enhance the user experience. You can animate various elements such as width, height, opacity, and more.
One of the key benefits of using JQueryUI animate is that it's easy to use and requires minimal code. As we'll see in the examples, you can create complex animations with just a few lines of code.
You might enjoy: Free Gif for Website
Animation Basics
To animate any element, such as a simple image, you need to understand the basics of animation in jQuery UI.
Directional properties like top, right, bottom, and left have no effect on elements if their position style property is static, which it is by default.
You can animate elements with a non-static position style property, but it's essential to understand the default settings to avoid unexpected results.
The position style property is static by default, so you'll need to set it to a different value, such as relative or absolute, to see any effect from directional properties.
Curious to learn more? Check out: Tailwind Css Animate
Animation Properties
Animation properties can be a bit tricky to work with, but don't worry, I've got you covered. You should animate all properties to a single numeric value, except for properties like width, height, or left, which can be animated but not background-color unless you use the jQuery.Color plugin.
Most non-numeric properties, like background-color, cannot be animated using basic jQuery functionality. However, properties like scrollTop and scrollLeft, as well as custom properties, can be animated.
The units em and % can be specified where applicable, but shorthand CSS properties, like font or background, are not fully supported. For example, if you want to animate the rendered border width, you'll need to set the border style and width in advance, or use the CSS equivalent 'font-size' for font size.
Worth a look: Css3 Animations
Duration
Durations are given in milliseconds, and higher values indicate slower animations, not faster ones.
The default duration is 400 milliseconds, which is a good starting point for most animations.
You can also use the strings 'fast' and 'slow' to indicate durations of 200 and 600 milliseconds, respectively. This can be a quick way to add some variation to your animations without having to specify the exact duration.
Suggestion: Animation Webflow
Using Relative Values
You can use relative values in the animate method to create animations that change over time, like increasing the height and width of an element by a certain amount each time the animation executes.
For example, if you want to increase the height of an element by 150px, you can use the value '+=150px' in the animate method.
This method executes when you click a button, and as you can see, the height and width are increased by 50px each time the button is clicked.
Relative values can be used with any property that can be animated, including height, width, and opacity.
By using relative values, you can create animations that are more dynamic and engaging, and that respond to user input in a more interactive way.
For instance, if you want to increase the height and width of a block by 50px each time the animate method executes, you can use the values '+=50px' for both properties.
This will create a smooth and seamless animation that changes over time, making it more engaging for the user.
See what others are reading: Webflow Scroll Animations
Returns: jQuery
The .animate() method returns a jQuery object. This allows us to chain additional methods to the animation.
The jQuery object returned by .animate() can be used to access the animated elements and their properties. For example, we can use the .css() method to get the current value of a CSS property after the animation has completed.
The returned jQuery object is essential for creating complex animation effects by allowing us to build on the initial animation. It's a powerful tool that opens up a world of creative possibilities.
Animation Options
You can specify the duration of the animation in milliseconds, for example, 500 milliseconds or "slow". The animation can also be queued up, allowing you to chain animations together.
The jQuery.animate() method allows you to define a transition function to adjust the gradient of the animation, also known as an easing function. This can be used to create different styles of animation.
Here's a breakdown of the animation options:
You can also use a callback function to execute code after the animation is complete, for example, alerting a message when the animation is done.
Callback Functions
Callback functions are a powerful tool in animation, allowing you to execute custom code at specific points in the animation process.
You can define callback functions for the start, step, progress, complete, done, fail, and always events, which are called on a per-element basis. This means each callback function is executed once per matched element, not once for the animation as a whole.
The .promise() method is useful for obtaining a promise to which you can attach callbacks that fire once for an animated set of any size, including zero elements.
Here are some examples of callback functions in action:
Note that the step function is particularly useful for enabling custom animation types or altering the animation as it is occurring. It accepts two arguments: now and fx, where now is the numeric value of the property being animated at each step, and fx is a reference to the jQuery.fx prototype object.
Sequential Animations
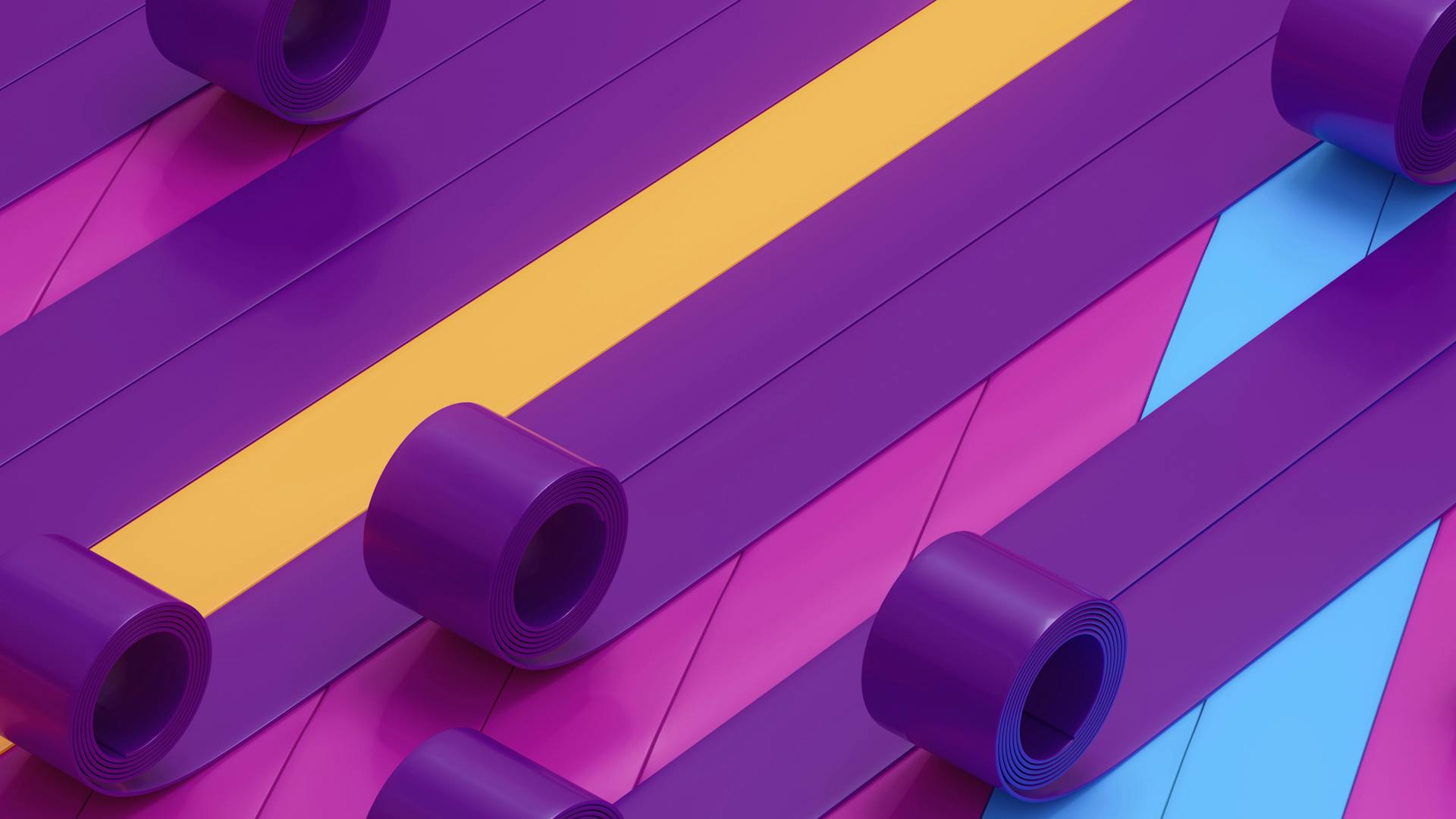
Sequential animations allow you to execute multiple animations one after the other by calling the .animate() method several times in a row.
You can create a sequence of animations by concatenating multiple .animate() methods, as shown in Example 2. For instance, moving an element 250 pixels to the right and then 100 pixels down can be achieved by calling .animate() twice in a row.
This technique is useful for creating complex animations that involve multiple steps. By chaining multiple animations together, you can create a smooth and seamless transition between different states.
Here's an example of how to create a sequence of animations:
- Move an element 250 pixels to the right
- Move the same element 100 pixels down
To achieve this, you would call .animate() twice in a row, like this:
$( ".selector" ).animate( { left: "250px" } );
$( ".selector" ).animate( { top: "100px" } );
Sources
- https://api.jquery.com/animate/
- https://beginnersbook.com/2019/04/jquery-animate-effect/
- https://medium.com/@tyler_brewer2/using-animate-in-jquery-53022c041660
- https://stackoverflow.com/questions/5047498/how-do-you-animate-the-value-for-a-jquery-ui-progressbar
- https://www.ionos.com/digitalguide/websites/web-development/jquery-animate/
Featured Images: pexels.com