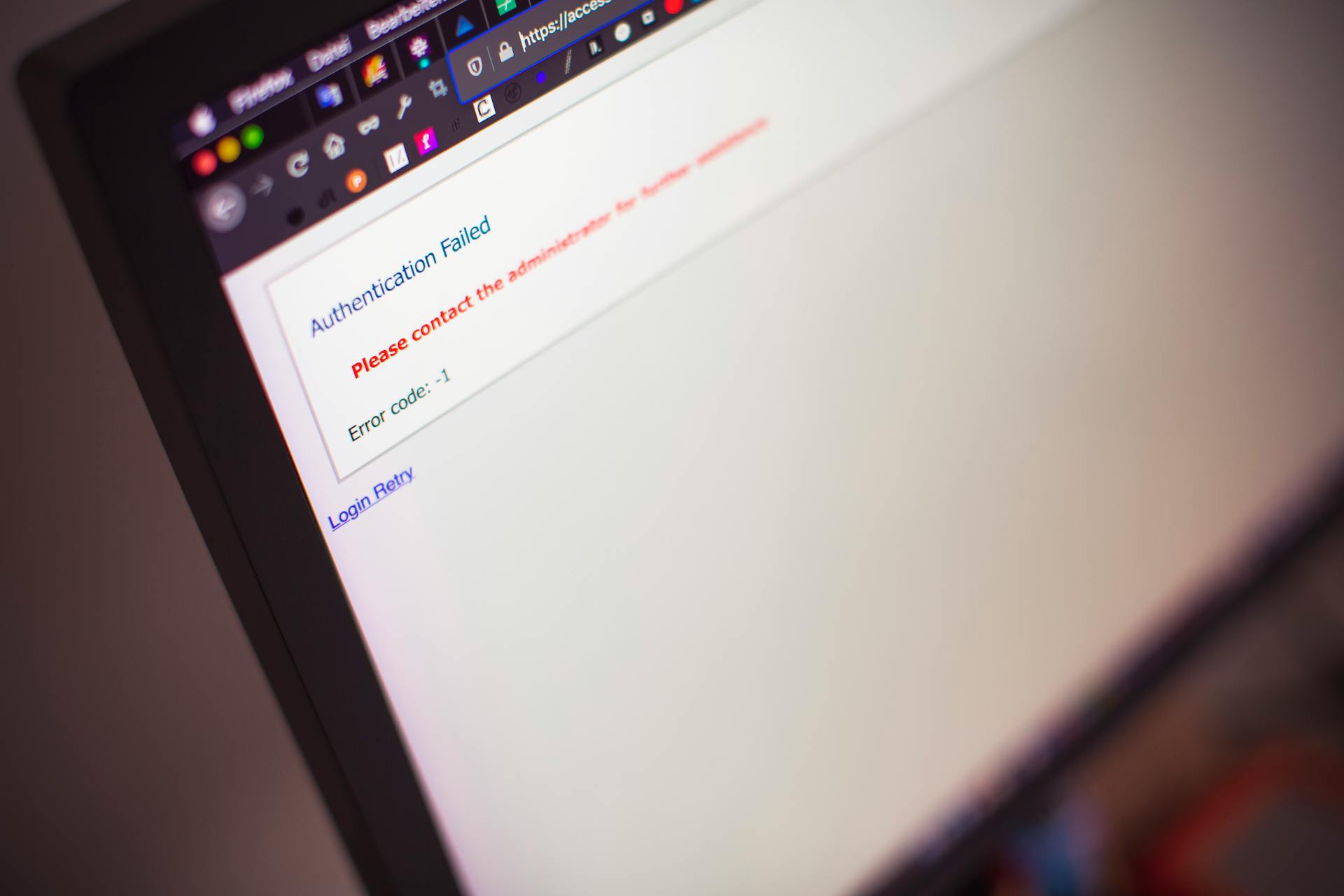
Implementing authentication with Keycloak and Next.js is a straightforward process.
To get started, you'll need to install the Keycloak adapter for Next.js, which can be done using npm or yarn.
This adapter allows you to use Keycloak as an authentication provider in your Next.js application.
You'll then need to configure Keycloak to issue JSON Web Tokens (JWTs) that can be used to authenticate users in your Next.js app.
The Keycloak adapter for Next.js will handle the process of obtaining and verifying these JWTs for you.
By following these steps, you can easily integrate Keycloak authentication into your Next.js application.
For another approach, see: Next.js
Setting Up Keycloak
To set up Keycloak, start by navigating to the Keycloak admin console at http://localhost:8080/auth/admin. Log in using your administrator credentials to access the Master realm.
Once logged in, click on the dropdown menu in the top left corner of the admin console and select "Add realm." Fill out the "Add Realm" form with the following details:
Click on the "Create" button to create the realm.
Realm Setup
To set up a Keycloak realm, you'll need to start by navigating to the admin console. The default URL is usually http://localhost:8080/auth/admin.
Once you're logged in, you'll be on the "Master" realm by default. This is where you'll create a new realm.
To create a new realm, click on the dropdown menu in the top left corner of the admin console. It usually displays "Master." From this dropdown, select "Add realm."
In the "Add Realm" form, you'll need to enter some details. The most important one is the name of your realm. Choose a meaningful name that represents your application or organization.
Here are the details you'll need to enter in the "Add Realm" form:
After entering the details, click on the "Create" button to create the realm.
Using the JavaScript Adapter
You can directly integrate the Keycloak JavaScript adapter into your React application. This approach is straightforward and gets the job done.
To use the JavaScript adapter, you'll need to modify your code, specifically the src/app/page.tsx file, to include the adapter's functionality.
Once you've integrated the adapter, you can access protected data when authenticated and prompt users to sign in when unauthenticated.
Configure
To configure Keycloak with your Next.js app, you'll need to create an OIDC client in Keycloak. This involves setting up a client in the Keycloak admin UI, which can be accessed by clicking Open Console in the Phase Two Dashboard.
You'll need to click Clients in the menu, then Create client, and select OpenID Connect as the client type. Enter a Client ID and a Name for the client.
Under the Capability Config section, leave the defaults as selected. Then, under Login settings, you'll need to add a redirect URI and Web origin. For a local development setup, the redirect URI and Web origin can be set to http://localhost:3000/* and http://localhost:3000, respectively.
Click Save to create the client. You can then download the adapter config for the client by clicking the Action dropdown and selecting Download adapter config. Select Keycloak OIDC JSON as the format option to get the necessary details.
A fresh viewpoint: Nextjs Download
You'll also need to copy the Client secret from the Credential tab for the client to use. This will be used in the configuration of your Next.js app.
To configure the authOptions with KeycloakProvider for next-auth, you'll need to add the following code to your file:
Add the following environment variables to a file named .env.local:
NEXTAUTH_URL=http://localhost:3000
NEXTAUTH_SECRET=your_secret
Replace your_secret with a secret generated by running the command npm run generate-secret. This secret is used to sign and encrypt cookies.
To configure Keycloak, access the admin console at http://localhost:8080/auth/admin. Create a realm for your application, and within the realm, create a client for your Next.js app. Set Valid Redirect URIs to your app's URL and set Web Origins if needed.
To connect Keycloak to PostgreSQL, go to User Federation in the Keycloak admin console and select jdbc. Configure it with your PostgreSQL database details.
To set up identity providers, go to Identity Providers in the Keycloak admin console and select Google or GitHub. Follow the instructions to set up each provider, including creating OAuth credentials in Google and GitHub.
Additional reading: Nextjs Auth
Here's a summary of the Keycloak configuration steps:
Creating a Next.js Client
To create a client for your Next.js application in Keycloak, you can follow these steps. Click on the 'Create' button in the upper right corner of the "Clients" section.
You should fill out the form with the following information: Client ID, Client Protocol, and Root URL. Enter a unique identifier for your Next.js application, such as "nextjs-local" for the Client ID. Choose openid-connect as the Client Protocol, as it's the protocol used for modern web-based single sign-on (SSO). Enter the local development URL of your Next.js application, such as http://localhost:3001, for the Root URL.
Here's a summary of the required information:
Setting Up Users:
Setting up users is an essential step in creating a Next.js client. Users are the individuals who will interact with your application, and defining their roles and permissions is crucial for precise access control.
Within your Keycloak realm, you'll add users and assign them specific roles. These roles determine the privileges users have, so it's essential to establish a structured framework for managing authentication and authorization.
By setting up users and roles, you'll have a clear understanding of who can access what within your application. This will help you ensure that users only have the permissions they need, reducing the risk of unauthorized access.
As you add users, consider defining roles to categorize their privileges. This will make it easier to manage user access and permissions as your application grows.
A unique perspective: Can Nextjs Be Used on Traditional Web Application
Creating a Next.js Client
To create a client in Keycloak, you need to open the Admin UI by clicking Open Console in the Phase Two Dashboard.
You'll then click Clients in the menu, followed by Create client. Leave Client type set to OpenID Connect.
Enter a unique Client ID, such as "nextjs-local", and a Name for the client. Click Next to proceed.
Under Capability Config, leave the defaults as selected. This can be configured further later.
Under Login settings, you'll need to add a redirect URI and Web origin. A valid redirect URI is http://localhost:3000/*, and the corresponding Web origin is http://localhost:3000.
Here are the Keycloak client settings you'll need:
Make sure to save your client settings in Keycloak.
Sources
- https://phasetwo.io/blog/instant-user-managemenet-and-sso-for-nextjs/
- https://medium.com/inspiredbrilliance/implementing-authentication-in-next-js-v13-application-with-keycloak-part-1-f4817c53c7ef
- https://stackoverflow.com/questions/65728505/how-can-i-use-keycloak-in-next-js
- https://blog.stackademic.com/integrating-keycloak-authentication-in-next-js-with-postgresql-db4b55c78d29
- https://www.geeksforgeeks.org/how-to-implement-keycloak-authentication-in-react/
Featured Images: pexels.com