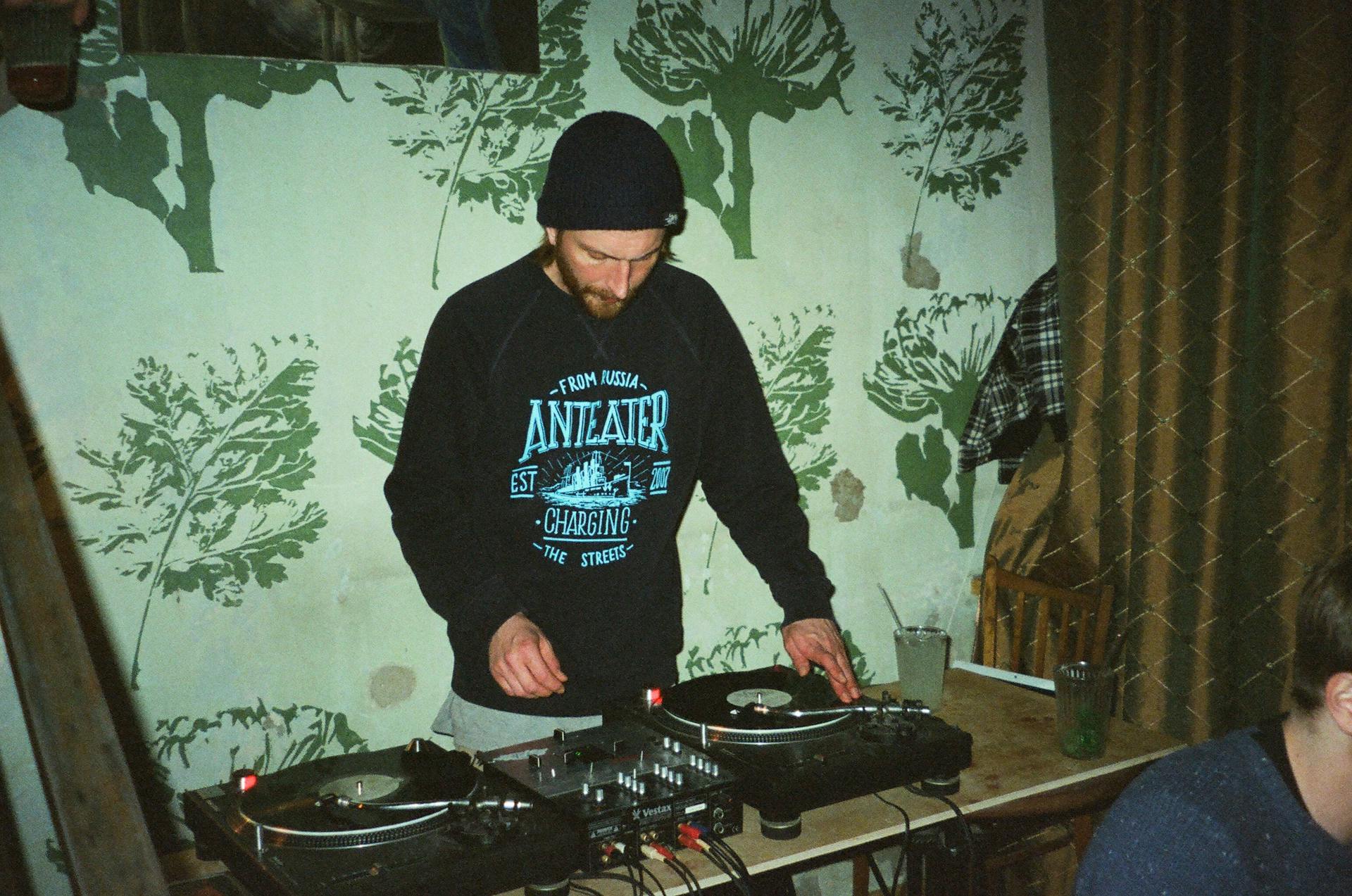
Laravel Mix is a powerful tool for compiling and processing frontend assets in Laravel applications.
It allows developers to write simple CSS and JavaScript code that can be easily compiled into production-ready code.
TailwindCSS is a utility-first CSS framework that provides a set of pre-defined classes for styling HTML elements.
This approach eliminates the need for writing custom CSS code from scratch.
By combining Laravel Mix and TailwindCSS, developers can create efficient and maintainable frontend codebases.
For example, with Laravel Mix and TailwindCSS, you can write a single line of code to generate a responsive navigation bar.
Curious to learn more? Check out: Free Frontend Development Courses
Install
The first step in setting up Laravel Mix with Tailwind CSS is to install them in your project. Open a command prompt and navigate to your Laravel project directory.
To do this, run the following commands: `npm install tailwindcss postcss autoprefixer` and `npm install laravel-mix tailwindcss`. These commands install Tailwind CSS and its dependencies like PostCSS and Autoprefixer.
This process seamlessly integrates Tailwind CSS with Laravel Mix, making it easy to use.
See what others are reading: Tailwindcss Install Css
Configuration
To configure Tailwind CSS, you'll need to create a configuration file at the root of your application. This file will contain some basic options for Tailwind CSS, such as purge, darkMode, theme, variants, and plugins.
You can modify these options to change the default behavior and appearance of Tailwind CSS. For example, you can add paths to your template files in the purge option, which will remove any unused CSS classes from your final output.
To create a minimal tailwind.config.js file, run the command `npx tailwindcss init`. This will create a file at the root of your application that you can then configure to your liking.
In the content key of the tailwind.config.js file, you'll need to add the locations where your files live, such as .php, .blade.php, .js, and .vue files.
To add support for Tailwind CSS, you'll need to add an .option() to the .sass() call in your webpack.mix.js file. This will enable Tailwind CSS to process your CSS files.
Recommended read: React Js Bootstrap Modal
You should also define the tailwindcss constant at the top of the page, and add a .version() call at the end of the file to polish it off a bit.
Remember to add Tailwind CSS to your Laravel Mix configuration by opening the webpack.mix.js file and adding tailwindcss as a PostCSS plugin. This will instruct Laravel Mix to compile the app.js file to public/js and process the app.css file to public/css using Tailwind CSS.
To add Tailwind CSS directives to your CSS file, you'll need to open the app.css file in the resources/css directory and add the following lines: @tailwind base; @tailwind components; @tailwind utilities;.
For your interest: Font Awesome Icons Next Js
How to Use It
To use Laravel Mix with Tailwind CSS, you'll need to compile your CSS/JS files when you make changes. This is done with two commands: one for development and one for production.
The development command includes all Tailwind classes, while the production command purges unused Tailwind classes. You'll also need to run one of these commands when you make changes to your configuration files.
Run the following command to create a Tailwind CSS configuration file: npm run init. This will create a minimal tailwind.config.js file at the root of your application.
In the tailwind.config.js file, you can configure the locations where your files live, such as .php, .blade.php, .js, and .vue files.
To add Tailwind CSS to your Laravel Mix configuration, open the webpack.mix.js file and add tailwindcss as a PostCSS plugin. Your webpack.mix.js file should look something like this: mix.postCss('app.css', 'public/css', tailwindcss);
To use Tailwind CSS in your project, you'll need to add Tailwind CSS directives to your CSS file. These directives tell Tailwind CSS where to inject its base, components, and utilities styles. Add the following lines to your app.css file: @tailwind base; @tailwind components; @tailwind utilities;
Finally, start your build process by running the command npm run dev. This command compiles and bundles your assets using Laravel Mix, creating a final app.css file in public/css containing all Tailwind CSS styles.
For your interest: Using React Js with Bootstrap
Compiling Assets
Laravel Mix is a powerful tool that allows you to compile your assets with ease, and one of the key features is its ability to compile Tailwind CSS.
Laravel Mix uses PostCSS to compile Tailwind CSS, which is a powerful tool for customizing your CSS.
You can configure Laravel Mix to compile your assets by using the mix command in your terminal.
To use the mix command, you need to have the mix.php file in your Laravel project, which is where Laravel Mix is configured.
The mix command can be used to compile your assets in different ways, such as compiling Tailwind CSS and JavaScript files.
For example, you can use the mix.js('resources/js/app.js', 'public/js') command to compile your JavaScript files.
Similarly, you can use the mix.css('resources/css/app.css', 'public/css') command to compile your CSS files.
Laravel Mix also supports watching your assets for changes, which means you can see the changes reflected in your compiled assets without having to recompile them manually.
You can use the mix.watch() command to start watching your assets for changes.
This feature is especially useful when you're working on a project that involves a lot of CSS and JavaScript files.
Recommended read: Javascript Web Page Design
Purging and Optimization
Purging your CSS classes is a great way to keep your production build small. You can use TailwindCSS to purge unused CSS classes.
To start purging, you'll need to run a command at the root of your project to generate a tailwind.config.js file. This file is essential for configuring TailwindCSS.
Once you have the tailwind.config.js file, you can update the purge array to specify the resources you want TailwindCSS to purge on a production run. This will help remove unused CSS classes.
TailwindCSS will automatically look for the tailwind.config.js file in the root of your project, so you don't need to pass it in as you did in the past. However, if you want to do that, you can update your webpack.mix.js file to pass the file.
To configure PurgeCSS, you can add it to your webpack.mix.js file by chaining .purgeCss() onto Mix. This will enable PurgeCSS only on production builds, which is perfect for keeping your build small.
PurgeCSS can be configured with options, but for a simple integration, you can ignore checking CSS between comments, such as the base and components Tailwind imports.
Benefits and Introduction
Laravel Mix and Tailwind CSS are a match made in heaven. Tailwind CSS is very flexible, allowing developers to change or add to the default settings as they want for their project.
This flexibility, combined with its responsive classes, makes it easy to create responsive designs that adapt to different screen sizes. With Tailwind CSS, developers can quickly handle layout changes without writing too many media queries.
Here are some key benefits of using Tailwind CSS in a Laravel project with Laravel Mix:
- Flexibility and Customization
- Responsive Design Made Easy
- Performance Optimization
- Active Community and Documentation
The combination of Tailwind CSS and Laravel Mix also results in better asset compilation, which leads to smaller and cleaner CSS files. This in turn improves the performance of the app.
The Problem with Dynamic Content
Initially, I was very pleased with the reduction in file size after implementing PurgeCSS, but soon realized there was an issue with dynamic content.
The blog uses a CMS package called Canvas, which uses HTML elements to separate post content. You can view the source of this post to see what this page structure looks like.
This issue was caused by PurgeCSS removing the styles that I previously created to make posts look normal. Those rules were not being used by the blog on any static pages.
PurgeCSS deemed them unused, when in fact my posts needed them, resulting in a problem with dynamic content.
Introduction
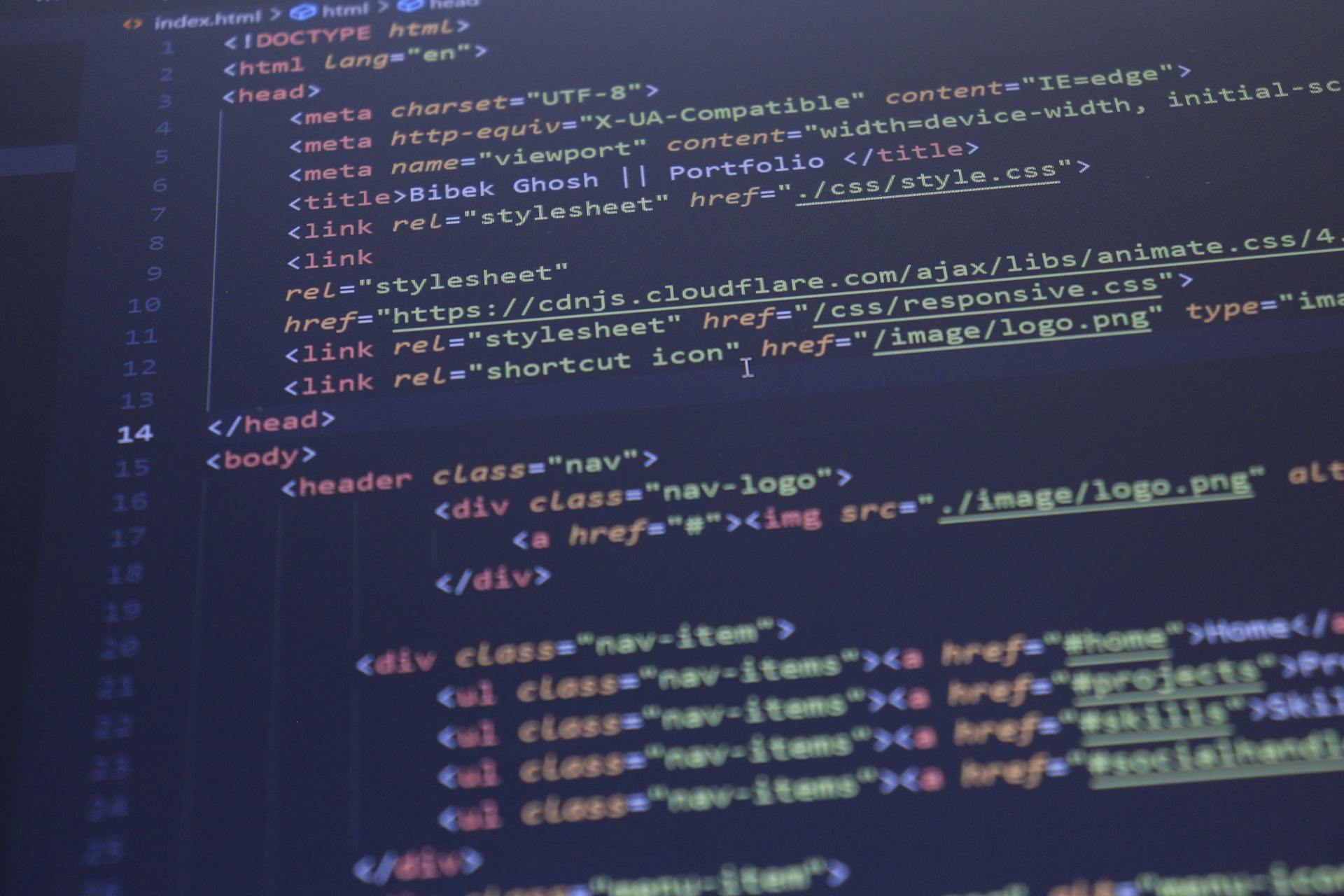
Welcome to this article about the benefits and introduction of Tailwind CSS in a Laravel project. Tailwind CSS is a popular framework that can help you create responsive and customizable designs with ease.
It's great for developers who want to create unique and tailored designs for their Laravel apps. With Tailwind CSS, you can change or add to the default settings as you want, making it a very flexible framework.
One of the main benefits of using Tailwind CSS is that it makes responsive design easy. You can quickly handle layout changes for different screen sizes without writing too many media queries.
Here are some key benefits of using Tailwind CSS in a Laravel project:
- Flexibility and Customization
- Responsive Design Made Easy
- Performance Optimization
- Active Community and Documentation
Tailwind CSS is also great for performance optimization, as it works well with Laravel Mix to compile small and clean CSS files that remove unused styles. This makes the file size less and the performance better.
If you're new to Tailwind CSS, don't worry! There are plenty of resources available to help you get started, including good documentation and a large community of fans who can provide tips and demos.
Sources
- https://ralphjsmit.com/tailwind-sass-laravel
- https://laravel.com/docs/8.x/mix
- https://www.tailwindtap.com/blog/how-to-install-tailwind-css-laravel-mix
- https://devdojo.com/thinkverse/laravel-quickie-how-to-install-and-build-tailwindcss-v2-with-laravel-mix
- https://davidgrzyb.com/optimizing-tailwind-file-size-purgecss-laravel-mix/
Featured Images: pexels.com