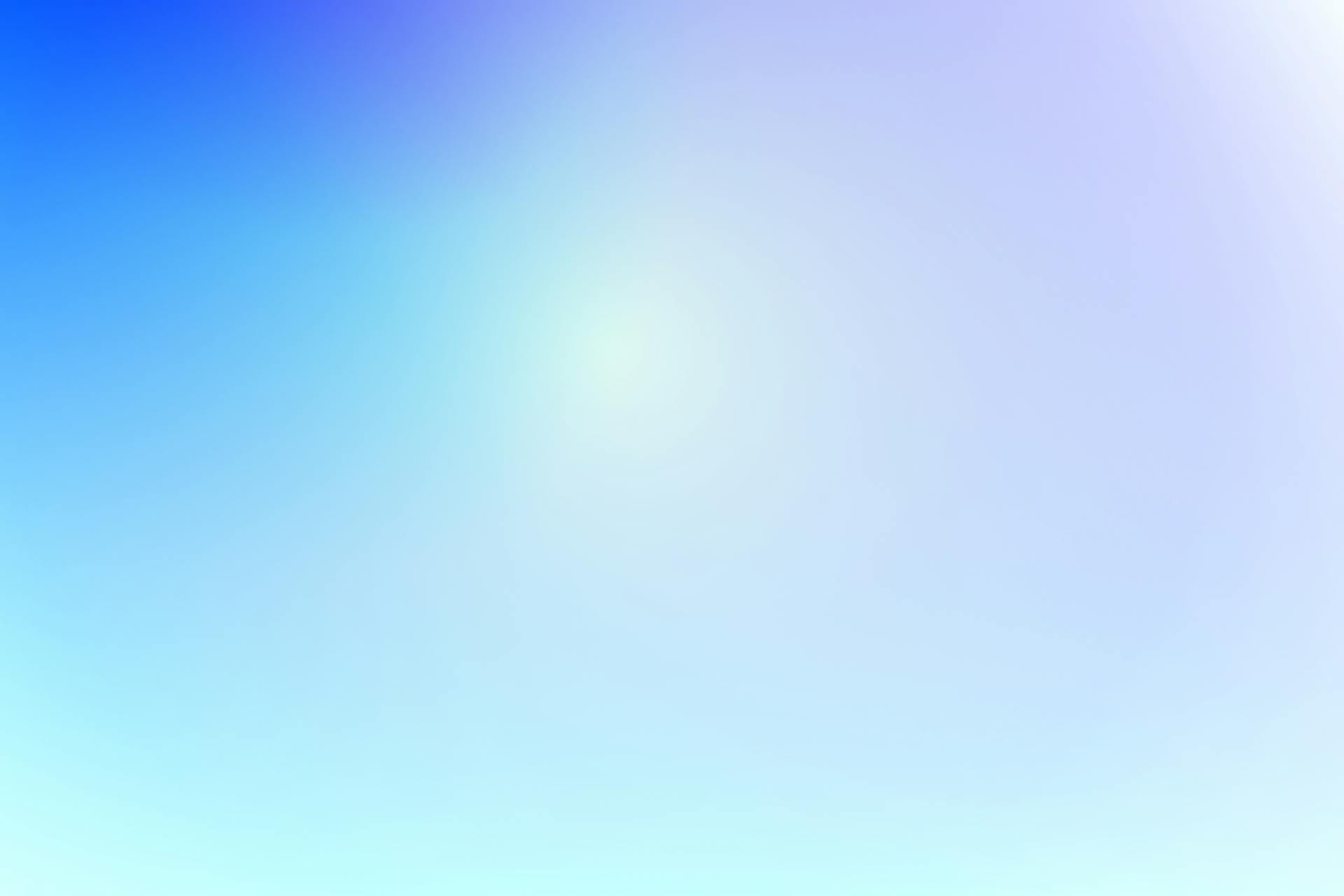
React JS with Bootstrap is a powerful combination for building responsive and interactive web applications. Bootstrap's pre-designed UI components and React's component-based architecture make it a perfect pair for developers.
With Bootstrap, you can create a responsive layout in just a few lines of code, as shown in the "Setting up Bootstrap with React" section. This layout will automatically adapt to different screen sizes and devices.
One of the key benefits of using React JS with Bootstrap is the ability to create reusable UI components. As demonstrated in the "Creating Reusable UI Components with React and Bootstrap" section, you can create a button component that can be used throughout your application.
By combining React JS with Bootstrap, you can build complex web applications with ease.
Expand your knowledge: Responsive Image in Bootstrap
Components and Libraries
Importing individual components like react-bootstrap/Button rather than the entire library can significantly reduce the amount of code sent to the client. This is a key principle to keep in mind when working with React and Bootstrap.
You can use UI components libraries like CoreUI for React, React-bootstrap, or Reactstrap to build and style your React.js applications. These libraries provide additional UI components and customizations.
CoreUI for React, for example, is a comprehensive UI library that extends Bootstrap 5 and includes additional components and features. It is maintained by a team of professionals and offers enterprise-level support.
Here are some popular UI components libraries for React:
- CoreUI for React
- React-bootstrap
- Reactstrap
Importing Components
Importing components is a crucial step in building a React application. Importing individual components like react-bootstrap/Button can significantly reduce the amount of code sent to the client.
Importing the entire library can be unnecessary and lead to bloated code. By importing only what you need, you can make your application more efficient and easier to maintain.
CoreUI for React, React-bootstrap, and Reactstrap are popular libraries that provide Bootstrap 5 components as React components. They can save time and effort by providing a solid foundation for building UI components.
Here's an interesting read: Twitter Bootstrap Components
Here are some key features of these libraries:
- CoreUI for React: Offers enterprise-level support and includes additional components and features.
- React-bootstrap: Provides Bootstrap 5 components as React components with a bootstrap stylesheet.
- Reactstrap: Enables importing individual components with a bootstrap stylesheet.
By choosing the right library and importing only what you need, you can build a robust and maintainable application with ease.
UI Component Libraries
CoreUI for React is a comprehensive UI library for React that extends Bootstrap 5 and includes additional components and features. It's maintained by a team of professionals and offers enterprise-level support.
React-bootstrap is a library that provides Bootstrap 5 components as React components with a bootstrap stylesheet. You can easily add Bootstrap and export the default post to prove it's installed.
Reactstrap is another library that provides Bootstrap 5 components as React components, enabling you to import individual components with a bootstrap stylesheet. It's designed to be simple, lightweight, and easy to use.
These libraries offer a variety of pre-built UI components that can be easily integrated into a React project, saving time and effort by providing a solid foundation for building UI components and ensuring consistency and maintainability across an application.
Worth a look: Bootstrap Ui Kit
Here are some benefits of using Bootstrap 5 and UI components libraries in React.js:
• Speed and efficiency: Bootstrap 5 provides a wide range of pre-designed components that can be easily imported and used in your React.js project.
• Consistent look and feel: Bootstrap 5 provides a consistent design language that ensures your application has a consistent look and feel.
• Cross-browser compatibility: Bootstrap 5 is designed to work seamlessly across all modern browsers.
• Responsive design: Bootstrap 5 is built with a mobile-first approach, making it easy to create responsive applications that look great on any device.
To use Bootstrap 5 in a React.js project, you can import individual components like `react-bootstrap/Button` rather than the entire library, which can significantly reduce the amount of code sent to the client.
You can also import Bootstrap as a dependency using a build tool or a module bundler like webpack or Vite. This will install the most recent version of Bootstrap, and you can include it in your app's entry file by importing both Bootstrap CSS and its associated JavaScript file.
Alternatively, you can import Bootstrap as a styling dependency using a module bundler or webpack. This will install Bootstrap and other supporting libraries like jQuery and popper.js, and you can import Bootstrap in your component by importing the bootstrap as shown below.
Take a look at this: Javascript Web Page Design
Styles and Layout
Styles and layout are crucial aspects of building a great user experience in React JS with Bootstrap. Bootstrap provides a 12-column flexbox grid system that allows for responsive and adaptable layouts. This grid system is based on a series of containers, rows, and columns elements.
Bootstrap offers two containers: container with a fixed, centered width for standard layouts, and container-fluid for full-width layouts. The row element forms horizontal containers for columns, ensuring proper alignment and equal height.
To create a responsive layout, you can use the Bootstrap grid system with the col- class, which is followed by a number from one to 12. For example, col-6 will create a column that spans half the width of its parent row.
Here are the Bootstrap responsive breakpoint classes:
- col-sm-: Applies to small screens, with a minimum width of 576px
- col-md-: Applies to medium screens, with a minimum width of 768px
- col-lg-: Applies to large screens, with a minimum width of 992px
- col-xl-: Applies to extra-large screens, with a minimum width of 1200px
Bootstrap also offers an auto-layout feature that enables users to create responsive layouts without specifying the exact widths of columns. In an auto-layout, columns with a col class will automatically size themselves to be equal width within the same row.
Suggestion: Zurb Foundation Columns
Stylesheets
React-Bootstrap doesn't come with included CSS, so you'll need to add a stylesheet to use its components.
You can include Bootstrap's source Sass files in your main Sass file and then require them on your src/index.js or App.js file, especially in a typical create-react-app application.
This approach works for most bundlers, but you might need to set it up differently depending on your bundler of choice.
To get started with styling, you can install React-Bootstrap and import its components, such as the Button component, which would look like import { Button } from 'react-bootstrap'.
Broaden your view: React Js Deploy Nginx in Azure Web App
Auto-Layout
Auto-layout is a game-changer for creating responsive layouts without specifying exact widths of columns. It's a feature in Bootstrap that enables users to create layouts where columns automatically size themselves to be equal width within the same row.
In an auto-layout, columns with a col class will take up an equal portion of the available width, making it easy to create layouts with multiple columns of equal width.
A unique perspective: Create React App vs Next Js
The syntax for auto-layout is slightly different than the traditional Bootstrap grid system, using the col element in React as a component from the React-Bootstrap library that receives its responsive classes as props.
For example, if you have three columns with col classes inside a row, each will take up an equal portion of the available width, making it easy to create layouts with multiple columns of equal width.
You can use the xs (extra small) and md (medium) props to specify the column widths, and Bootstrap will take care of the rest.
Here's a breakdown of the column widths in an auto-layout:
- xs: extra small screens
- md: medium screens
In an auto-layout, the columns will automatically adjust to the screen size, making it easy to create responsive layouts that work across different devices.
Browser Support
We aim to support all browsers supported by both React and Bootstrap. This ensures a seamless user experience across various devices and platforms.
React and Bootstrap have a wide range of browser support, but we're committed to keeping up with the latest advancements.
Installation and Setup
To install Bootstrap with React, you can use a CDN or install it from npm. If you choose to use a CDN, you can add a link to the Bootstrap CSS file in your public/index.html file. This is the easiest way to add Bootstrap to your React app.
You can also install Bootstrap from npm by running the command `npm install bootstrap` in your terminal. After installing Bootstrap, you need to import it in your React app entry file, which is usually the src/index.js file. You can do this by adding the following code: `import 'bootstrap/dist/css/bootstrap.min.css';`
Alternatively, you can use a package like React Bootstrap or Reactstrap, which provides pre-built Bootstrap components designed to work as React components. These packages can be installed using npm by running the command `npm install react-bootstrap bootstrap` or `npm install reactstrap`.
Broaden your view: Npm Next Js
Browser Globals
We provide react-bootstrap.js and react-bootstrap.min.js bundles with all components exported on the window.ReactBootstrap object. These bundles are available on jsDelivr, as well as in the npm package.
The react-bootstrap.js and react-bootstrap.min.js bundles are two JavaScript files that contain all the components from react-bootstrap. You can access these components by using the window.ReactBootstrap object.
These bundles are available on jsDelivr, a content delivery network that hosts a wide range of open-source projects. This means you can easily link to these files in your application and start using the components.
Project Setup
To set up a React.js project, you'll need to create a new project using a tool like Create React App (CRA). This will give you a basic project structure and a way to run your app.
You can install Bootstrap 5 in a CRA project by running the command `npm install bootstrap` in your terminal. This will install the Bootstrap CSS and JavaScript files, which you can then import into your `src/index.js` file.
One of the key benefits of using a package like Bootstrap is that it provides a set of pre-built UI components that you can use in your app. To use these components, you'll need to import the individual components you want to use from the Bootstrap package.
A unique perspective: Why Use Tailwind Css
Here are some common ways to add Bootstrap to your React app:
- Using the Bootstrap CDN: This is the easiest way to add Bootstrap to your app, and it requires no extra installation or downloads. You just need to include a link to the CDN in the head section of your application entry file.
- Installing Bootstrap from npm: This method requires you to run the command `npm install bootstrap` in your terminal, which will install the Bootstrap CSS and JavaScript files.
- Using a package like React Bootstrap: This package provides pre-built React components that wrap up Bootstrap classes and deliver native support for Bootstrap JavaScript components.
Regardless of the method you choose, you'll need to import the Bootstrap CSS file into your app. This can be done by adding the following code to your `src/index.js` file:
```html
import 'bootstrap/dist/css/bootstrap.min.css';
```
By following these steps, you'll be able to set up a basic React project with Bootstrap installed and ready to use.
Frequently Asked Questions
How to use Bootstrap forms in React?
To update a React form with Bootstrap, set the changed field and value in the form object and remove any associated errors. This ensures seamless form updates and error handling.
Sources
- https://react-bootstrap.netlify.app/docs/getting-started/introduction/
- https://coreui.io/blog/bootstrap-react-tutorial-with-examples/
- https://www.techiediaries.com/react-bootstrap/
- https://blog.logrocket.com/using-bootstrap-react-tutorial-examples/
- https://www.geeksforgeeks.org/how-to-use-bootstrap-with-react/
Featured Images: pexels.com