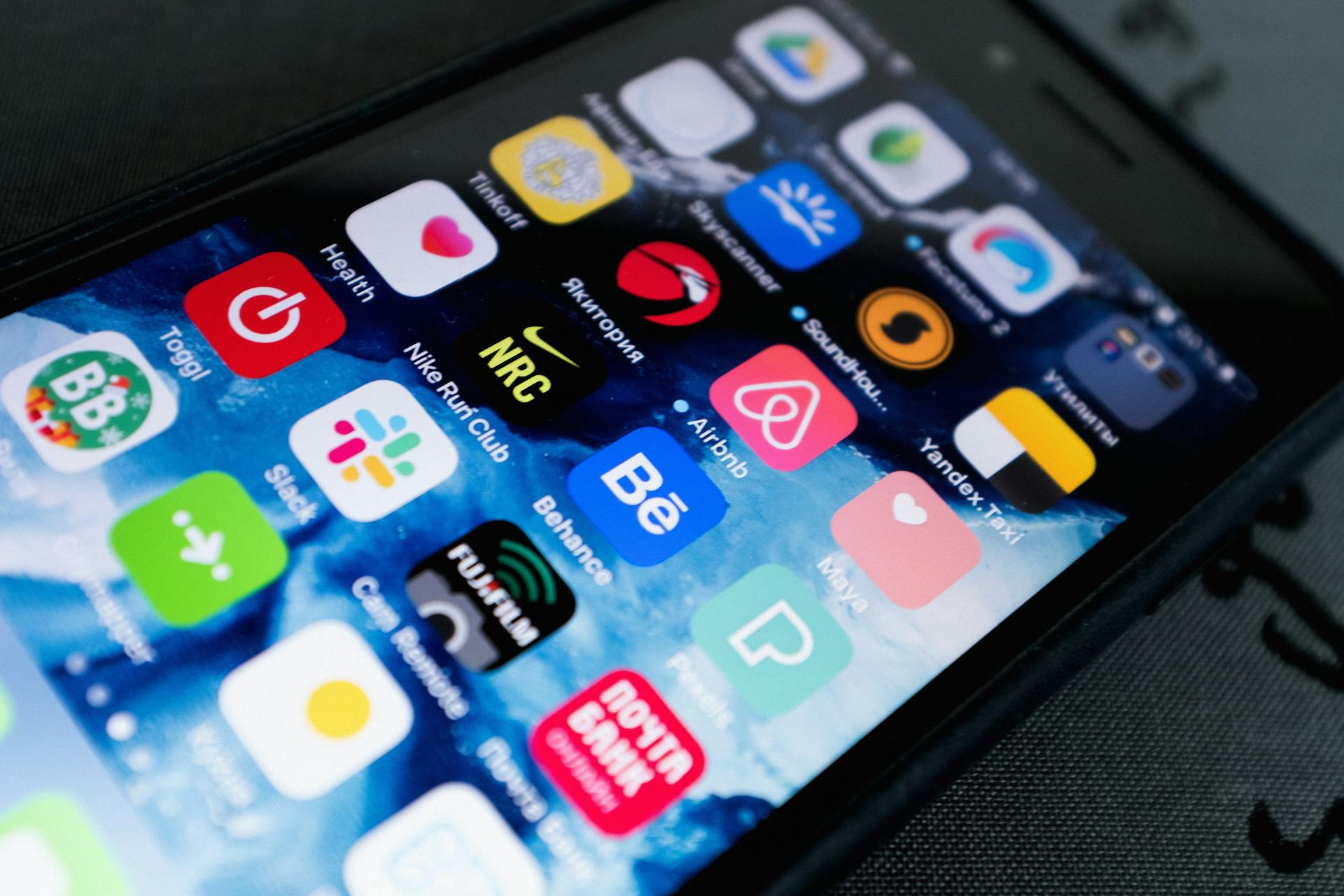
Bootstrap in JS is a popular front-end framework that simplifies the process of building responsive web applications. It provides a set of pre-designed HTML, CSS, and JS components that can be easily integrated into your project.
Bootstrap's grid system is based on a 12-column layout, which is divided into rows and columns. This allows for easy customization and responsiveness.
With Bootstrap, you can quickly create a responsive navigation bar using the navbar component. This includes features like dropdown menus and responsive design.
Bootstrap's JavaScript components, such as modals and tooltips, can be easily integrated into your project to enhance user interaction.
Getting Started
You can add Bootstrap to a Next.js application by installing Bootstrap and its dependencies. To do this, follow the steps outlined in the documentation.
If you're using the App Router, you'll need to import the Bootstrap CSS in your app/layout.js file instead of the usual method. This is a slight variation on the standard process, but still straightforward.
The Quick Version
To get started with Bootstrap in Next.js, you can install it and its dependencies.
You can install Bootstrap and its dependencies by following a simple process.
If you're using the App Router, you'll need to import the Bootstrap CSS in your app/layout.js file instead.
Now you can use Bootstrap classes in your Next.js components. That's it!
Next.js Best Practices
When building a Next.js application, it's essential to follow best practices to ensure a smooth development experience.
Use a consistent naming convention for your pages, such as using a prefix like "pages/" to distinguish them from other files.
Organize your pages into a directory structure that makes sense for your application, like grouping related pages together.
Use the `getStaticProps` method to pre-render pages at build time, which can improve performance and reduce the need for client-side rendering.
Make sure to handle errors properly by using a try-catch block to catch and display any errors that occur during page rendering.
Use internationalization (i18n) support to make your application accessible to users in different regions and languages.
Use a library like `react-intl` to manage translations and formatting for your application's UI.
Avoid using `dangerouslySetInnerHTML` to render HTML content, as it can lead to security issues and make your code harder to debug.
Use a consistent approach to handling API requests, such as using the `fetch` API or a library like Axios to make requests to your backend.
Importing and Customizing
Importing and Customizing Bootstrap in a Next.js Project is a breeze. Importing Bootstrap CSS in _app.js is the central hub where global stylesheets check-in, ensuring no corner of the application feels left out of the style party.
To customize _document.js for static resources, you can elegantly embed external links for Bootstrap CSS files, ensuring they load efficiently and reliably. This adjustment ensures that the Bootstrap style sheet is a fundamental part of the project’s architecture, woven into every corner of the application’s fabric.
Installing Bootstrap in a Next.js Project is like magic – suddenly, you have Bootstrap’s grid system, components, and styles at your fingertips, ready to distinguish your project from the rest.
Importing CSS in App.js
Importing CSS in App.js is a crucial step in getting your Next.js application looking its best. Adding Bootstrap CSS in _app.js ensures that no corner of your application feels left out of the style party.
Slide into the top of your _app.js file and add Bootstrap CSS like this. This tweak doesn't just dress up your code, it sets the stage for a stylish and cohesive design.
By importing Bootstrap CSS in _app.js, you're essentially checking in all global stylesheets at the central station of your application. This ensures a consistent look and feel throughout your project.
Imagine your _app.js file as the control center of your application, where all global styles and scripts check in. Adding Bootstrap CSS here is a great way to get started with a solid foundation.
Individual or Compiled
You can include Bootstrap plugins individually using the /js/dist/*.js files, which are UMD ready, making them compatible with bundlers like Webpack or Rollup.
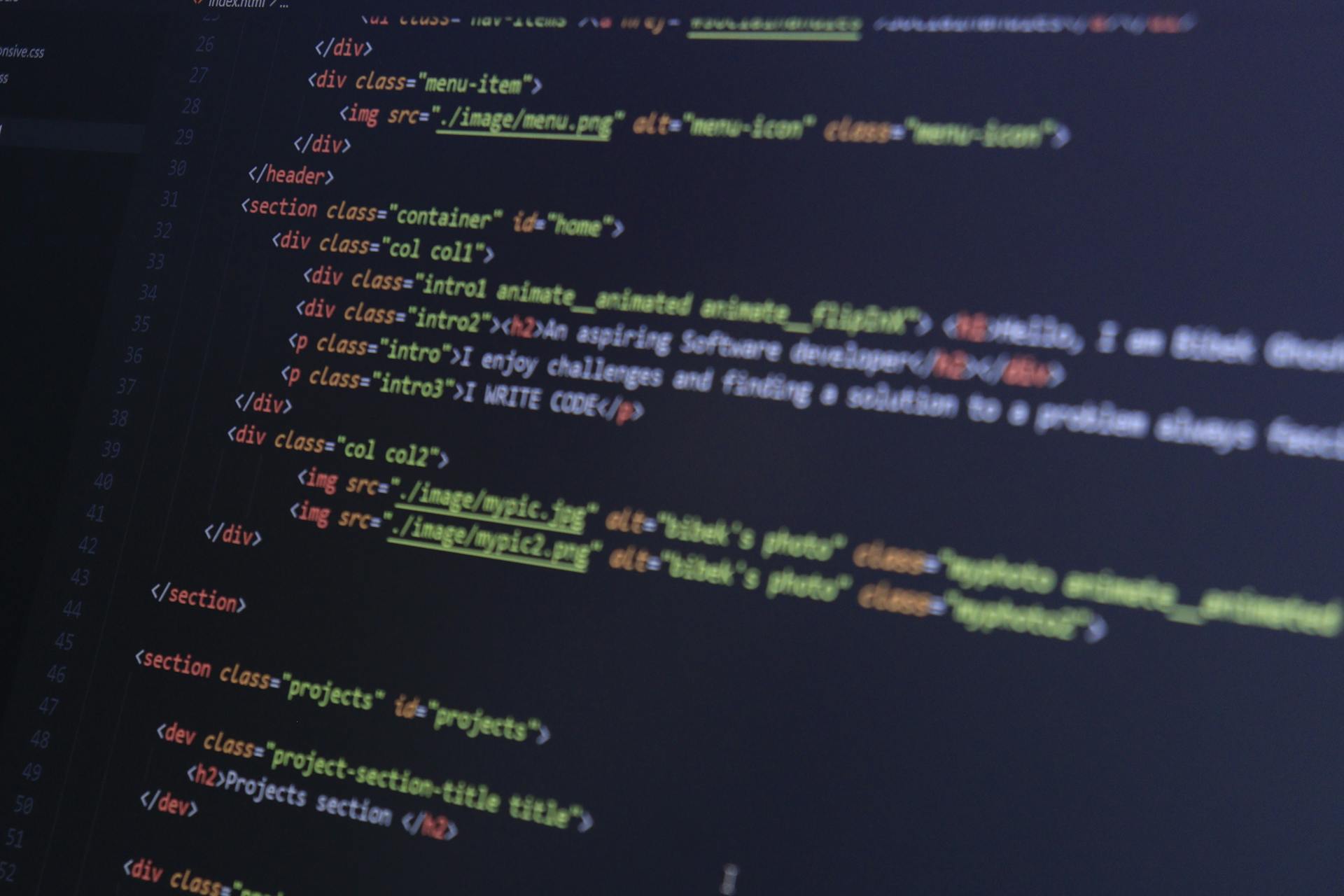
If you choose to use a bundler, you can use the individual /js/dist/*.js files, which are specifically designed to work with bundlers like Webpack or Rollup, and are UMD ready.
You can also include all Bootstrap plugins at once using bootstrap.js or the minified bootstrap.min.js, but be sure not to include both.
By including plugins individually, you have more control over which features you use, and can avoid loading unnecessary code.
Utilizing Bootstrap in JS
To utilize Bootstrap in your JavaScript application, you can import Bootstrap CSS in your _app.js file. This ensures that all parts of your application have access to Bootstrap's styles.
By adding Bootstrap's JavaScript bundle in _app.js, you can add dynamic behavior to your project, enabling features like popovers, modals, and dropdowns.
If you want to get a particular plugin instance, each plugin exposes a getInstance method. For example, to retrieve an instance directly from an element: `getInstance(element)`. This method will return null if an instance is not initiated over the requested element.
Here are the methods and static properties exposed by every Bootstrap plugin:
Enhancing App.js
To enhance _app.js, you can import Bootstrap CSS to give your application a consistent and stylish look. This involves adding the Bootstrap CSS to the top of your _app.js file.
Adding Bootstrap CSS ensures that all parts of your application have a unified style, making it easier to manage and maintain.
By importing Bootstrap's JavaScript bundle in _app.js, you can add dynamic behavior to your project, enabling interactive elements like popovers, modals, and dropdowns to respond to user interactions.
This tweak injects functionality and infuses life into your application, making it more engaging and user-friendly.
Utilizing JavaScript
Bootstrap's JavaScript bundle adds dynamic behavior to your project, enabling features like popovers, modals, and dropdowns.
You can import Bootstrap's JavaScript bundle in _app.js to add more dynamism to your project. This tweak infuses life into your application.
Bootstrap's Programmatic API allows you to get a particular plugin instance using the getInstance method. For example, to retrieve an instance directly from an element, you can use bootstrap.Popover.getInstance(myPopoverEl).
Every Bootstrap plugin exposes the following methods and static properties: dispose, getInstance, and getOrCreateInstance. The dispose method destroys an element's modal, while getInstance and getOrCreateInstance allow you to retrieve or create a modal instance associated with a DOM element.
If you want to use Bootstrap with a JavaScript framework like React, Vue, or Angular, you may encounter compatibility issues. A better alternative is to use a framework-specific package, such as React Bootstrap, BootstrapVue, or ng-bootstrap.
Bootstrap does not officially support third-party JavaScript libraries like Prototype or jQuery UI. However, you can use .noConflict on the plugin you wish to revert the value of in case of namespace collisions.
Asynchronous Functions and Transitions
All programmatic API methods in Bootstrap are asynchronous and return to the caller once the transition is started, but before it ends.
To execute an action once the transition is complete, you can listen to the corresponding event. This is a crucial aspect of working with transitions in Bootstrap.
A method call on a transitioning component will be ignored, so be sure to wait for the transition to finish before making another call.
Listening to the corresponding event is a simple yet effective way to ensure your code runs smoothly and predictably. It's a technique I've found to be particularly useful when working with complex UI components.
Frequently Asked Questions
How to install Bootstrap in js?
You can install Bootstrap in js using jsDelivr's CDN, Bower, npm, or Composer, depending on your project's requirements and setup. For a quick and easy installation, consider using jsDelivr's CDN or a package manager like Bower or npm.
Featured Images: pexels.com