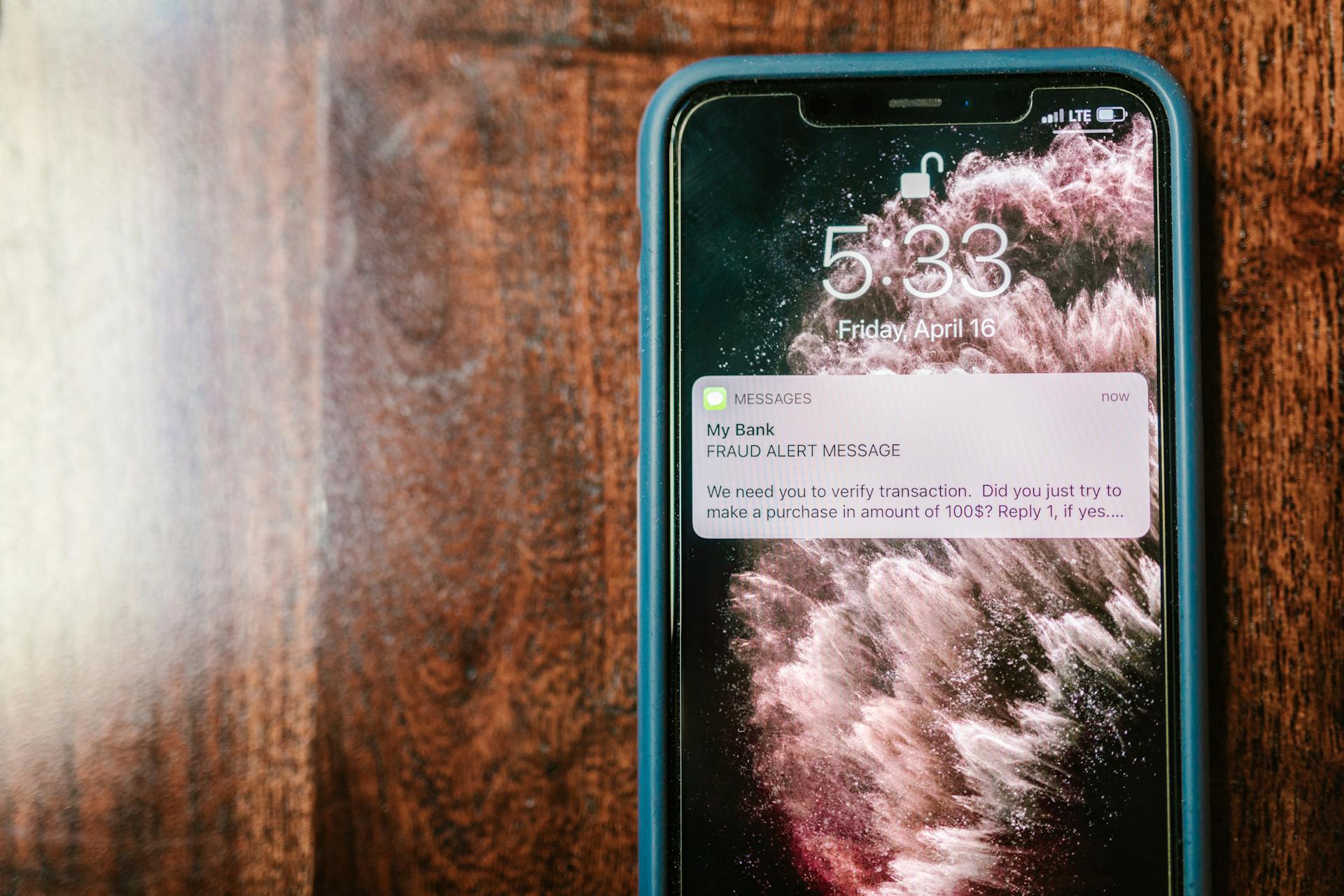
React JS Bootstrap Modal development can seem daunting at first, but with the right approach, you can create beautiful and functional modals that enhance your user experience.
To get started, you'll need to import the Bootstrap CSS and JS files into your React project. This is a crucial step, as it allows you to use Bootstrap's modal component.
One of the most important aspects of modal development is understanding how to trigger the modal to open and close. This is where the `onClick` event comes in, allowing you to attach a function to a button that opens the modal when clicked.
When working with modals, it's essential to consider accessibility features, such as keyboard navigation and screen reader support. This ensures that your modals are usable by all users, regardless of their abilities.
You might like: Twitter Bootstrap Modal Example
Modal Structure
A modal in React JS Bootstrap should have a modal header, which includes a dismiss action. This is a best practice to ensure users know how to close the modal.
The modal body is required for padding, meaning it's essential to include it to maintain a consistent layout.
Modal footers are optional, but can be useful for providing additional actions or information.
For your interest: Next Js Modal
Components
Modal components are a crucial part of a well-structured modal. They typically include a modal header, modal body, and modal footer.
The modal header is required for accessibility and should include a dismiss action whenever possible. This can be achieved by adding a close button or providing an explicit dismiss action.
A static modal example is one where its position and display have been overridden. This is a common use case for modal components.
The modal body is required for padding, which helps maintain a consistent layout.
Note that modal headers with dismiss actions are recommended, but not always necessary. In some cases, an explicit dismiss action may be sufficient.
The Modal component can be customized to change the direction of its animation by adding the 'animationDirection' prop with values 'top', 'bottom', or 'left'.
Here are the properties you can use to customize the Modal.Header:
- closeButton: Boolean(default true) - render a close button or not
- onClose: Function() - a Handle when the close button is clicked
Vertically Centered
To vertically center a modal, you can add the class "centered" to the MDBModalDialog. This will ensure the modal is centered vertically on the screen.
Adding a simple class can make a big difference in the overall user experience. It's surprising how often a small tweak like this can improve the flow of a website or application.
Vertically centered modals are a great way to draw attention to important information or to provide a clear call to action. By keeping the modal centered, you can prevent it from getting lost in the surrounding content.
In some cases, a vertically centered modal can be just what you need to keep your users engaged and focused on the task at hand.
Modal Behavior
The Modal component in React JS Bootstrap Modal offers a straightforward API, eliminating the need to deal with ModalTrigger or Overlay mixins.
This simplicity makes it easier to implement and manage modal behavior in your application.
Here are some key features that enable this streamlined behavior:
- Scoped focus, ensuring that the modal content receives focus when opened.
- Stackable, allowing you to use the modal in conjunction with other components that have constraints.
- Animated feedback is provided when trying to close a "static" Modal, enhancing the user experience.
Body
The body of a modal is a crucial part. It's a convenience component that holds the main content of the modal. This is where the actual content of the modal is placed, making it easily accessible to the user.
The main content of the modal is wrapped in a div with a class of 'modal-body'. This is the standard way to structure the body of a modal.
The content of the modal body can be anything, from text and images to forms and buttons. It's a flexible space that adapts to the needs of the user and the application.
By using a modal body, developers can create a clean and organized interface that guides the user through the modal's content.
On Exit
You can use the onExit callback to handle page interactions during the transition. This is especially useful when you want to display a message or change the page background when the user closes the modal.
The onExit callback is triggered when the modal is about to exit, and the onExited callback is triggered when the modal has finished exiting. You can use these callbacks to create a seamless user experience.
On a similar theme: Javascript Web Page Design
For example, you can show a 'goodbye' message when the user closes the modal by using the onExit and onExited callbacks, as shown in Example 3.
Here are some key points to keep in mind when using the onExit and onExited callbacks:
- The onExit callback is triggered when the modal is about to exit.
- The onExited callback is triggered when the modal has finished exiting.
By using these callbacks, you can create a smooth and intuitive user experience that complements your modal design.
Static Backdrop
The static backdrop is a key feature of modal behavior. It prevents the modal from closing when you click outside of it.
With a static backdrop, the modal remains open even if you click anywhere on the page. This can be useful for certain types of applications.
You can try this out by clicking the button below to see the static backdrop in action.
Scrolling Long Content
Scrolling long content can be a challenge, but there's a solution. You can create a scrollable modal that allows the user to scroll the modal body by adding .modal-dialog-scrollable to .modal-dialog.
To demonstrate this, try adding repeated line breaks to your content, just like the demo below. This will show how content can exceed the minimum inner height, resulting in inner scrolling.
If the content becomes too long, it will be cropped and scrollable within the modal. This is because the modal will automatically adjust its height to fit the content.
You can also use an inline style to set a minimum height for the modal, extending its length and demonstrating overflow scrolling. This is useful when you want to show how content will scroll when it exceeds the height of the viewport.
Fullscreen
Fullscreen modals are a great way to grab the user's attention. You can create a modal that covers the user's viewport by using modifier classes or props on the modal dialog.
There are several options for creating a fullscreen modal, depending on the screen size. For example, you can use the .modal-fullscreen class, which is always available.
Here's a breakdown of the different options:
Alternatively, you can use modifier props on the MDBModalDialog, such as size="fullscreen" or size="fullscreen-sm-down".
Change Animation
You can customize the animation of your modal by adjusting two variables: $modal-fade-transform and $modal-show-transform.
The $modal-fade-transform variable determines the transform state of .modal-dialog before the modal fade-in animation.
Setting $modal-fade-transform to scale(.8) can create a zoom-in animation effect.
The $modal-show-transform variable determines the transform of .modal-dialog at the end of the modal fade-in animation.
To achieve a zoom-in animation, set $modal-fade-transform to scale(.8) and let the animation do the rest.
On Entered
The onEntered event is a powerful tool for modal behavior. It fires once the modal has finished coming into view, making it ideal for asynchronously loading data or interacting with elements within the modal.
This event is particularly useful for updating the modal's title, as seen in an example where the title is updated from "Transitioning..." to "Modal Ready". The onEntered event can also be used to display the time it takes for the modal to open in milliseconds.
One practical application of the onEntered event is to start a timer and display the time it takes for the modal to open. In this example, the time is displayed in the modal's body, giving you a clear understanding of how long it takes for the modal to become visible.
The onEntered event can also be used to manually readjust the modal's position if the height of the modal changes while it is open, such as when a scrollbar appears. This ensures that the modal remains visible and accessible to the user.
GetOrCreateInstance
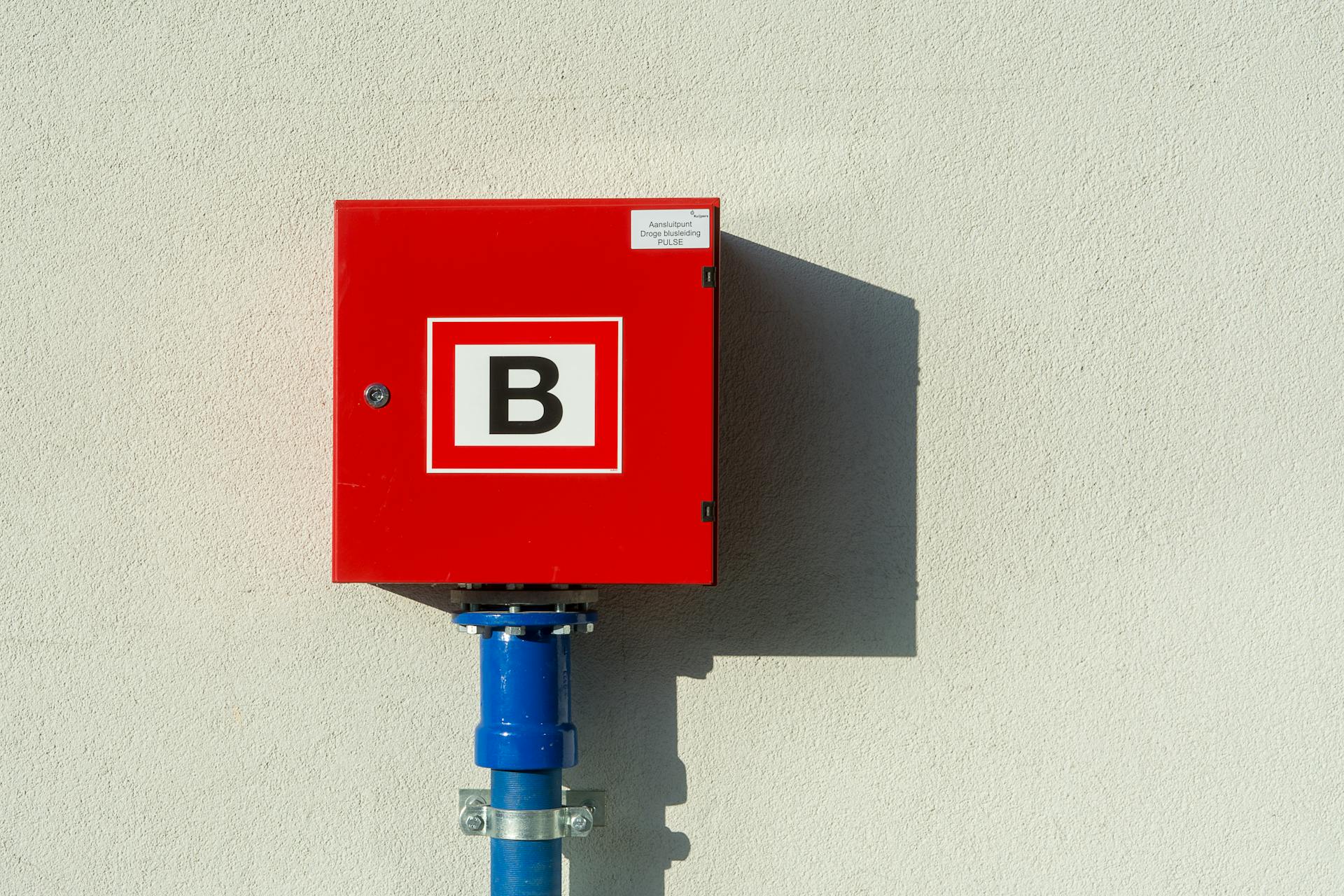
GetOrCreateInstance is a static method that allows you to get the modal instance associated with a DOM element, or create a new one in case it wasn’t initialised.
This method is super useful for handling modal behavior, especially when working with dynamic content or elements that are added to the DOM after the initial rendering.
To get started, you can simply call the method and pass in the DOM element as an argument, like so: getOrCreateInstance(element).
Frequently Asked Questions
How to use Bootstrap modals in React?
To use Bootstrap modals in React, combine the
How to center React-Bootstrap modal?
To center a React-Bootstrap modal, add the class "modal-dialog-centered" to the modal-dialog element. This will vertically align the modal in the center of the screen.
Featured Images: pexels.com