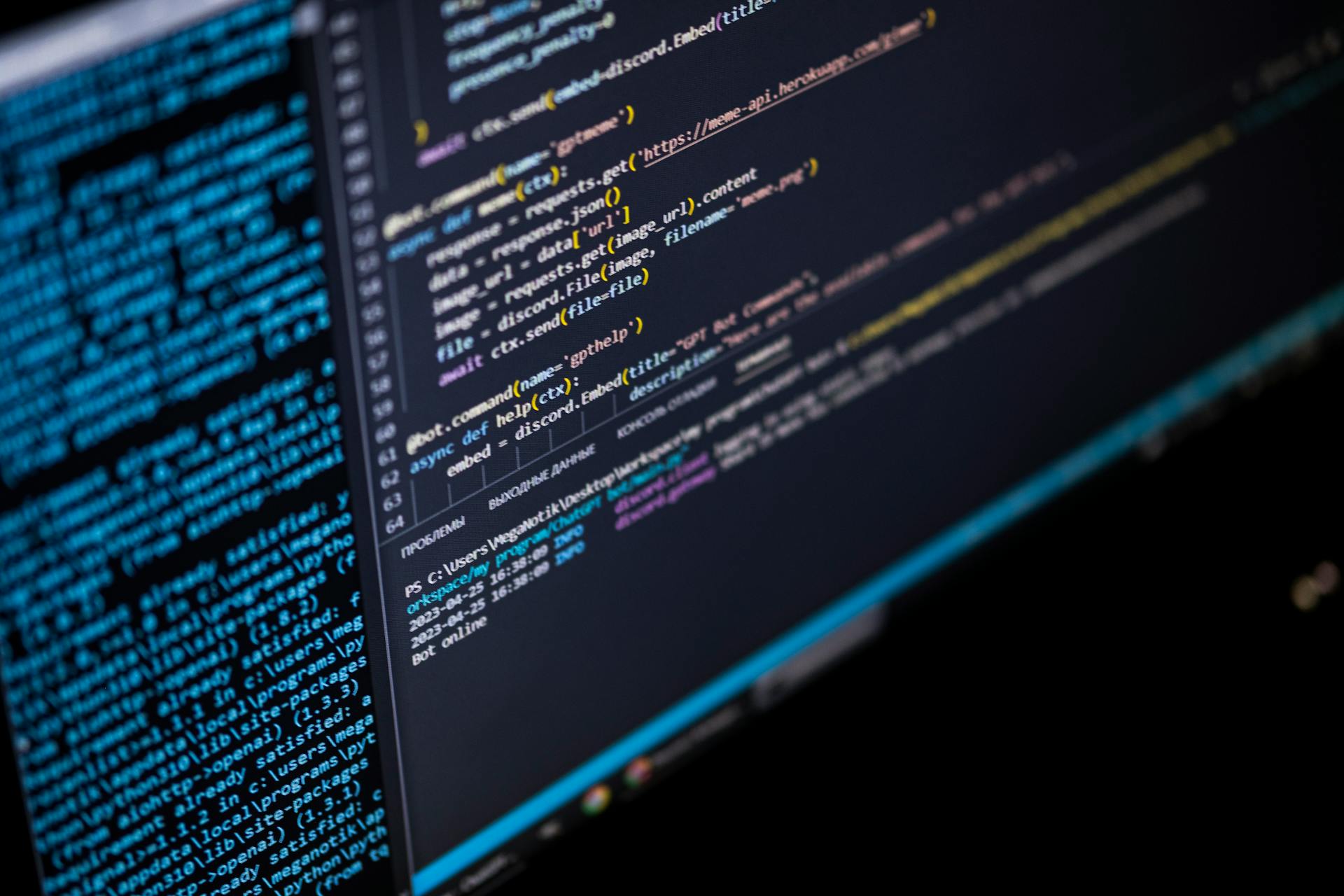
MUI Next JS and Next.js are two powerful tools that can revolutionize your web development workflow. By combining them, you can create fast, scalable, and maintainable applications.
MUI Next JS integrates Material-UI components with Next.js, allowing you to build responsive and accessible UI components with ease. This integration provides a wide range of pre-designed components, including buttons, forms, and navigation menus.
With Next.js, you can create server-side rendered (SSR) and statically generated (SG) websites, which can improve your website's performance and SEO. By leveraging MUI Next JS and Next.js, you can create a seamless user experience that is both fast and visually appealing.
Next.js provides a built-in support for internationalization (i18n) and localization (L10n), making it easy to translate and customize your application for different languages and regions. This feature is especially useful when building global applications with MUI Next JS.
Building Forms
Building forms with Material UI (MUI) and Next.js is a breeze. You can create stylish forms by importing the necessary packages, including React Hook Form and MUI.
To start, you'll need to install the packages in your project. This will give you access to the powerful useForm hook utility functions that simplify form management. React Hook Form provides a declarative way to create, manage, and validate forms.
One of the key benefits of using React Hook Form is its simplicity. You can create a form by adding the useForm hook to a new file, src/components/form.js. This hook streamlines the process of handling form state, input validation, and submission.
To create a visually appealing form, you can use MUI's UI components and styles. MUI provides a collection of ready-to-use UI components that you can customize by passing styling props. You can also use the styled method to style your UI elements with CSS properties.
Here are some key components to include in your form:
- A main container to hold the form
- A form component to handle form state and validation
- Input text fields to collect user data
- A submit button to trigger form submission
By using these components, you can create a functional and visually appealing form that makes it easy for users to interact with your application.
Here are some key props to include in your form components:
- `register` prop to register form input fields and track their values
- `validation rules` prop to specify validation rules for input fields
- `onSubmit` prop to trigger form submission
By following these steps, you can create a robust and user-friendly form that meets the needs of your application.
Here are some examples of how you can use these components and props in your form:
These are just a few examples of how you can build forms with Material UI and Next.js. With a little practice and experimentation, you can create custom forms that meet the unique needs of your application.
MUI Components
MUI Components are a game-changer for building visually appealing and functional UI elements in your Next.js application. They come with a variety of components that can be used to create a consistent and elegant design.
One of the most useful components in MUI is the CssBaseline component, which helps to establish a simple baseline for building upon. It's a great starting point for any project.
The Box component is another essential component in MUI, serving as a wrapper for most of the CSS utility needs. It packages all the style functions exposed in @mui/system.
Here are some common components in MUI:
- CssBaseline: Establishes a simple baseline for building upon
- Box: Wrapper component for most of the CSS utility needs
Box
The Box component is a wrapper component for most of the CSS utility needs, falling under the Layout Category of UI Components.
It packages all the style functions that are exposed in @mui/system, making it a great tool to have in your toolkit.
You can use the Box component to style your section and buttons in the forms, as I've done in my own Sass file.
To create your own styles, you can follow my example and create a Sass file to style your section and buttons accordingly.
The Box component is a versatile tool that can be used in a variety of ways, making it a great addition to your MUI Components arsenal.
Typography
Typography is a Data-Display Component that makes it easy to apply a default set of font weights and sizes in your application.
The Typography component uses the variantMapping prop to associate a UI variant with a semantic element, which is an important distinction to make.
This means the style of a typography component is independent of the semantic underlying element, giving you more flexibility in how you design your application.
The Typography component is a great way to simplify your application's typography, making it easier to manage and maintain.
Stepper Component
To create a Linear Stepper in Next.js (React) using the MUI library, you'll want to start by importing the necessary components, including Typography, Stepper, Step, StepLabel, and Button from Material UI.
The Stepper component is a crucial part of this process, and it's used to display the steps in a linear fashion. You can map the steps array to the Stepper to pass the elements of the steps as StepLabel.
To handle the navigation between steps, you'll need to create a function called generateStep, which returns the correct Form Component based on the activeStep value. This is typically done using a switch case statement.
You can use the handleBack and handleNext buttons to increment and decrement the activeStep value using the SetActiveStep function. This allows users to navigate through the steps in a linear fashion.
Here's a summary of the key components involved in creating a Linear Stepper in Next.js (React) using the MUI library:
The Stepper component is a powerful tool for creating linear step-by-step experiences in your Next.js (React) application. By using the MUI library and following these best practices, you can create a seamless and intuitive user experience for your users.
Next.js Integration
Combining Next.js and MUI can be a bit tricky, but it's worth it for the benefits of server-side rendering. Generally, it's not necessary to render CSS on the server side, but if you don't include styles in your server response, you'll risk FOUC, or flickering.
To avoid this issue, you need to correctly integrate MUI's server-side rendering functionality. This is exactly what this blog post aims to help you with.
You'll also need to handle routing carefully, as both Next.js and MUI provide their own Link component. The MUI Link component can be tied to a third-party framework like Next.js using the component prop.
Combining Next.js and MUI
Combining Next.js and MUI can be a bit tricky, but don't worry, I've got you covered. To combine both, you'll need to use the Link component from Next.js and the Link component from MUI together.
One point of confusion is that both Next.js and MUI provide their own Link component. The Link component from Next.js handles all the navigation, but the Link component from MUI is necessary for styling. Thankfully, the MUI Link component accepts a prop called component, which allows it to be tied to a third-party framework like Next.js.
Inside your page.js, you can tie your MUI Link with a Next.js Link by using the component prop. This is done by adding the component prop to the MUI Link, like this: component="next/link".
For any links that will leave your application, such as external or deep links, you don't need Next's Link component because you are leaving your app's router.
Calendar API
You'll often find yourself needing to access the underlying Calendar object for raw data and methods. This is especially useful for controlling the current date.
The initialDate prop is useful for setting the initial date of the calendar, but you'll need to use date navigation methods to change it later.
To change the date after the initial setup, you'll need to get ahold of the component's ref, also known as a reference. This is a crucial step in accessing the Calendar object.
Once you have the component's ref, you can call the getApi method of the "current" component instance. This is where the magic happens, and you can start manipulating the calendar data.
Faster Time-to-Market
In today's highly competitive tech landscape, time-to-market is a metric that organizations always seek to optimize.
A component library can give designers and developers a massive headstart with thoroughly tested UI elements ready to go. This can save a significant amount of time and effort that would otherwise be spent on designing and testing individual components from scratch.
Having a component library also means that teams can focus on more complex tasks and features, rather than getting bogged down in the details of UI design.
Here are some key benefits of using a component library:
- Massive headstart with thoroughly tested UI elements
- Significant time and effort saved on designing and testing individual components
Understanding & Implementing an Example
To create a Linear Stepper in Next.js using MUI, you can use the MUI library to make a Stepper that has Steps coming from an array of steps, with Step Content that gets shown as per the Active Step running.
You can pass different Forms as Child Components to the switch cases which are shown in the Active Step content.
In the Form Components, you can use the TextField Component of MUI to take the input and edit, and also use the register method of useForm to register the data.
For styling, you can use the sx prop, which allows you to customize the styling of a single component based on various situations.
The sx prop is especially useful when you need to adapt your styling based on a component's props, and you can't use the styled() utility to keep the Next.js server-side rendering benefit.
To implement dynamic customization, you can use the sx prop to style a specific component like a button based on various situations, such as success or failure.
Here's a quick rundown of the key components involved in creating a Linear Stepper:
By understanding how to use these components and props, you can create a Linear Stepper that meets your needs and provides a great user experience.
Frequently Asked Questions
What is the difference between MUI and NextUI?
MUI and NextUI differ in their design approaches, with MUI focusing on pre-built, accessible components and NextUI prioritizing a lightweight, customizable system. NextUI's flexibility makes it appealing to developers seeking tailored UI solutions.
Which UI framework is best for NextJS?
For Next.js projects, Tailwind CSS is a top choice due to its utility-first approach and extensive customization options. Other popular options like Chakra UI, Material-UI, and Shadcn UI also offer significant customization capabilities.
Featured Images: pexels.com