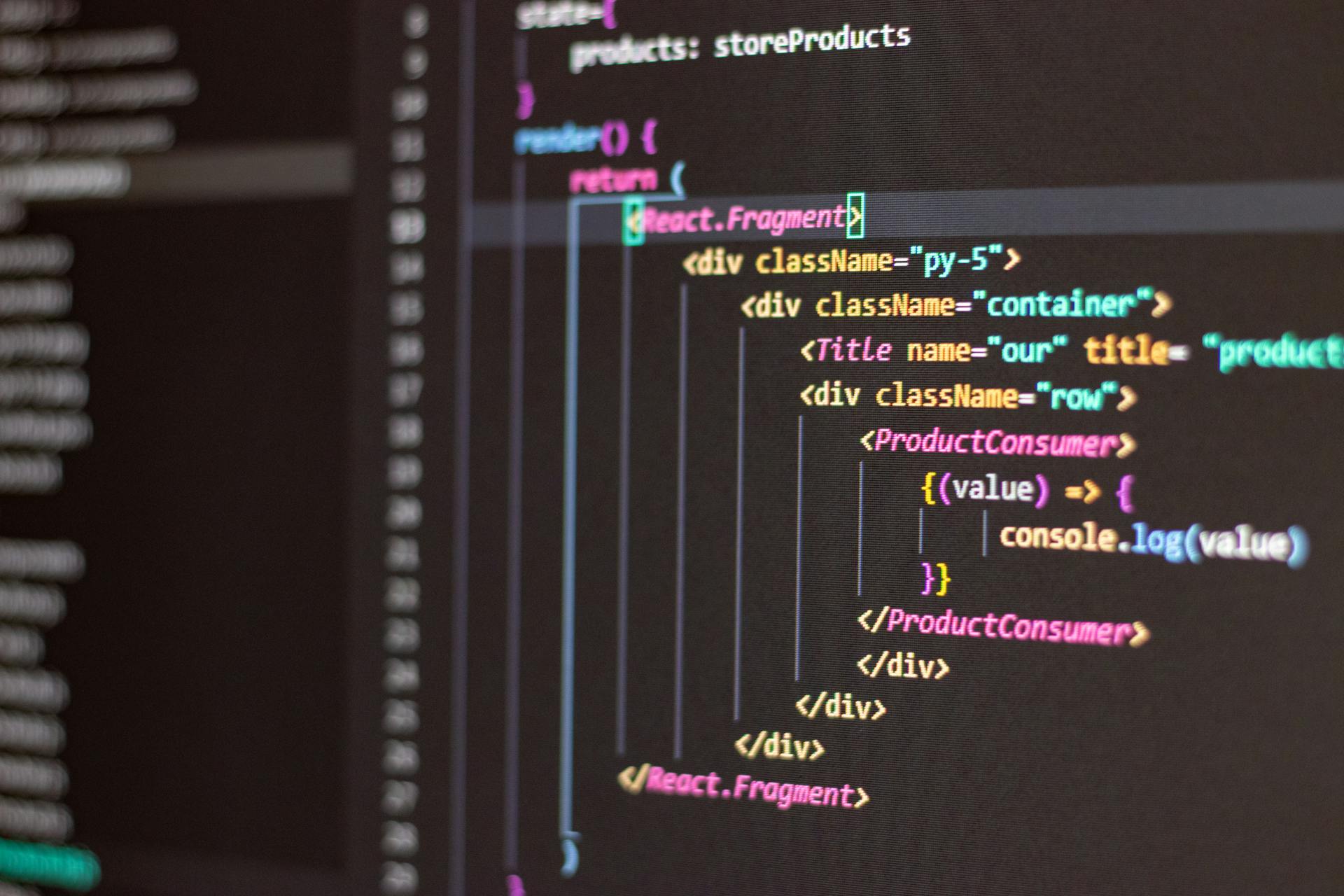
Let's dive into the world of Next.js examples and real-world use cases. Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
To get started with Next.js, you'll want to familiarize yourself with its core features, such as its built-in support for CSS-in-JS solutions like styled-components and CSS modules. This allows for efficient and scalable styling of your application.
Next.js also boasts a robust API routing system, which enables you to create RESTful APIs with ease. You can create API routes using the getServerSideProps method, as demonstrated in the example of creating a simple blog API.
In the example of building a simple blog, Next.js's getStaticProps method is used to pre-render pages at build time, resulting in faster page loads and improved SEO. This is a powerful feature that can significantly enhance the performance of your application.
Routing
Routing in Next.js is straightforward, thanks to its intuitive API. You can easily determine the current route using the `useRouter` hook, which returns an object containing the current route.
To catch all routes in Next.js, you can use the `catchAll` function, which allows you to define a catch-all route for your application. This is particularly useful for handling unknown routes.
Here's a breakdown of the different types of routes available in Next.js:
Optional catch-all routes can also be defined in Next.js, providing a flexible way to handle unknown routes.
Pages Router
With Next.js Pages Router, you can fetch data using server-side requests in two main ways.
getServerSideProps fetches data at runtime so that content is always fresh. This means that the data is updated every time the page is loaded.
getStaticProps pre-renders pages at build time for data that is static or changes infrequently. This approach is ideal for data that doesn't change often, as it allows for faster page loads.
Here's a quick rundown of the two methods:
Middleware
Middleware plays a crucial role in refreshing expired Auth tokens and storing them securely. You need middleware to accomplish this, especially since Server Components can't write cookies.
To refresh the Auth token, you call supabase.auth.getUser. This ensures the token is revalidated with the Supabase Auth server every time.
You should pass the refreshed Auth token to Server Components through request.cookies.set, so they don't attempt to refresh the same token themselves.
To replace the old token in the browser, you pass the refreshed Auth token to the browser using response.cookies.set.
You can add a matcher to the middleware to run only on routes that access Supabase. This is a good practice to follow.
Be careful when protecting pages, as the server gets the user session from the cookies, which can be spoofed by anyone. Always use supabase.auth.getUser() to protect pages and user data.
Here's a step-by-step guide to creating middleware for refreshing Auth tokens:
1. Create a middleware.js file at the project root.
2. Create another middleware.js file within the utils/supabase folder.
3. The utils/supabase file contains the logic for updating the session.
4. The middleware.js file uses the logic from utils/supabase to refresh the Auth token.
Never trust supabase.auth.getSession() inside server code such as middleware. It isn't guaranteed to revalidate the Auth token.
Revised Heading
Routing is a crucial aspect of Next.js, and one of the key features is the ability to fetch data on the server-side. Next.js getServerSideProps() allows you to fetch data on the server-side.
Next.js provides several ways to handle data fetching, including using the fetch API directly. This can be useful when you need more control over the fetching process.
Here are some key functions related to data fetching in Next.js:
- fetch: used to fetch data from an API
- getServerSideProps(): used to fetch data on the server-side
These functions are essential for building dynamic and data-driven routes in Next.js.
Features
Next.js offers several features that make it a powerful framework for building server-rendered and statically generated web applications. One of its key features is Global CSS Support.
Next.js also simplifies routing by automatically creating routes based on the file structure of your pages directory. This makes it easy to manage your application's URL structure.
Some of the key features of Next.js include Server-side Rendering (SSR), Static Site Generation (SSG), and Automatic Code Splitting. These features improve SEO and initial load performance by rendering pages on the server and pre-rendering pages at build time.
Here are some of the key features of Next.js in a list:
- Server-side Rendering (SSR)
- Static Site Generation (SSG)
- Automatic Code Splitting
- Data Fetching
- Routing
- Image Optimization
- Built-in CSS and JavaScript Bundling
- API Routes
Features of
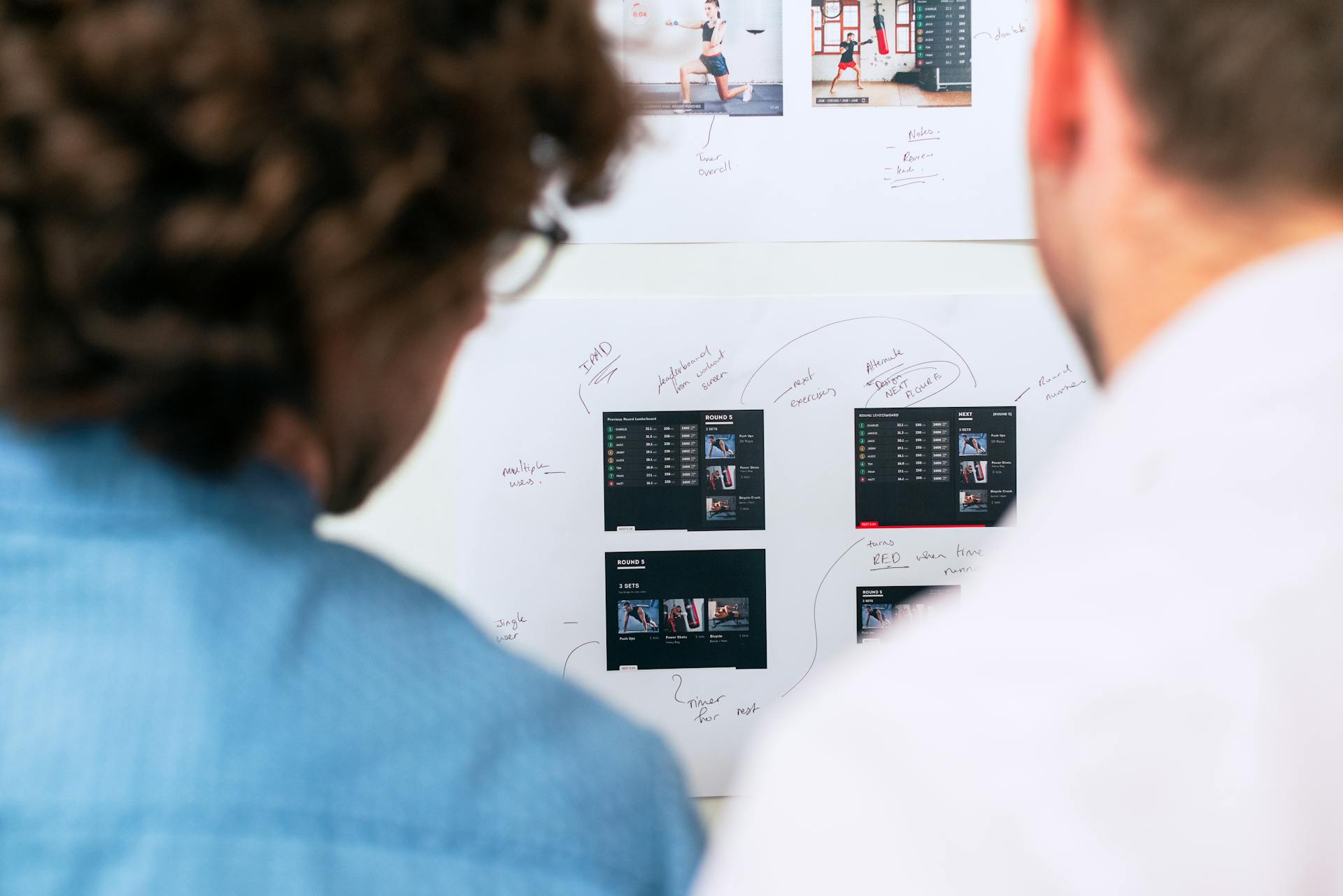
Next.js is a powerful React framework that offers a wide range of features to help you build fast and scalable web applications.
One of the key features of Next.js is its ability to support global CSS. This means you can write CSS that applies to your entire application, making it easier to manage your styles.
Next.js also allows you to serve static files directly from your pages directory. This is especially useful for static websites or blogs that don't require server-side rendering.
With Next.js, you can pre-render your pages at build time using its built-in static site generation feature. This can significantly improve the performance of your website by reducing the load time.
Next.js also offers several ways to fetch data, including getStaticProps for fetching data at build time and getServerSideProps for fetching data on each request.
Here are some of the key features of Next.js:
- Server-side Rendering (SSR): This improves SEO and initial load performance by rendering pages on the server.
- Static Site Generation (SSG): This pre-renders pages at build time, making them super fast to load.
- Automatic Code Splitting: This breaks down your application code into smaller bundles, improving load times by only loading the code needed for the current page.
- Data Fetching: Next.js offers several ways to fetch data, including getStaticProps for fetching data at build time and getServerSideProps for fetching data on each request.
- Routing: Routing is simplified in Next.js. It automatically creates routes based on the file structure of your pages directory.
- Image Optimization: Next.js automatically optimizes images, including resizing and compressing them.
Next.js also allows you to create serverless functions directly within your application using API routes. This lets you add backend functionality to your React application without needing a separate server.
Email Template
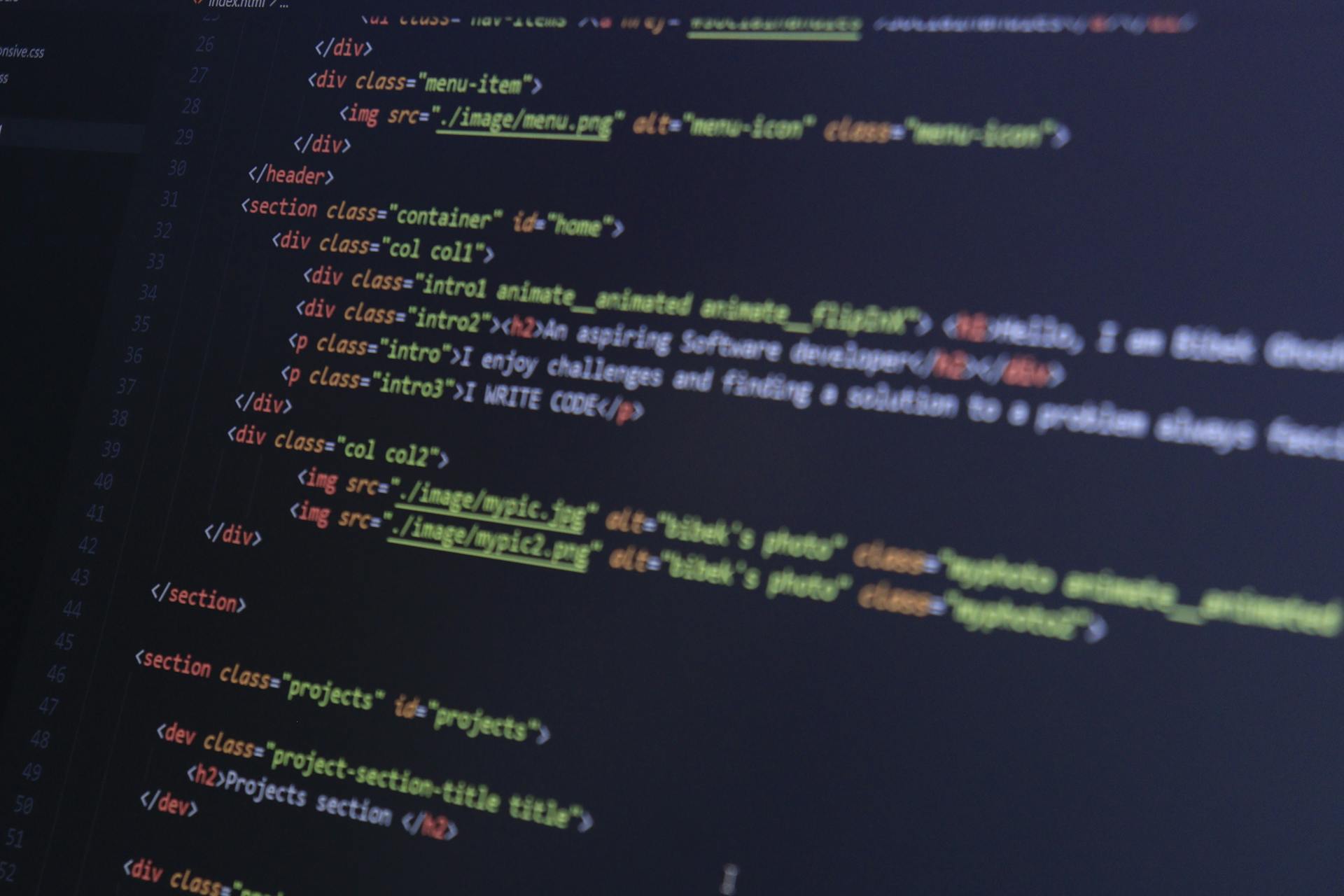
Email Template is a feature that lets you customize the look and content of emails sent to new users. You can do this by changing the template settings in your project.
To get started, go to the Auth templates page in your dashboard. From there, select the Confirm signup template. This template is where you can make changes to the email's content and query parameters.
One way to customize the email is to change the {{ .ConfirmationURL }} to {{ .SiteURL }}/auth/confirm?token_hash={{ .TokenHash }}&type=email. This will update the link in the email to point to the correct URL.
You can also check out the settings of your project to see what else you can customize.
Upload Widget
We can create an upload widget to allow users to upload their profile photos. This can be done by creating a new component, as shown in Example 1.
An avatar widget is a great way to enable users to upload a profile photo. It's a common feature in many applications.
To create an avatar widget, we can start by creating a new component. This is where the magic begins.
Featured Images: pexels.com