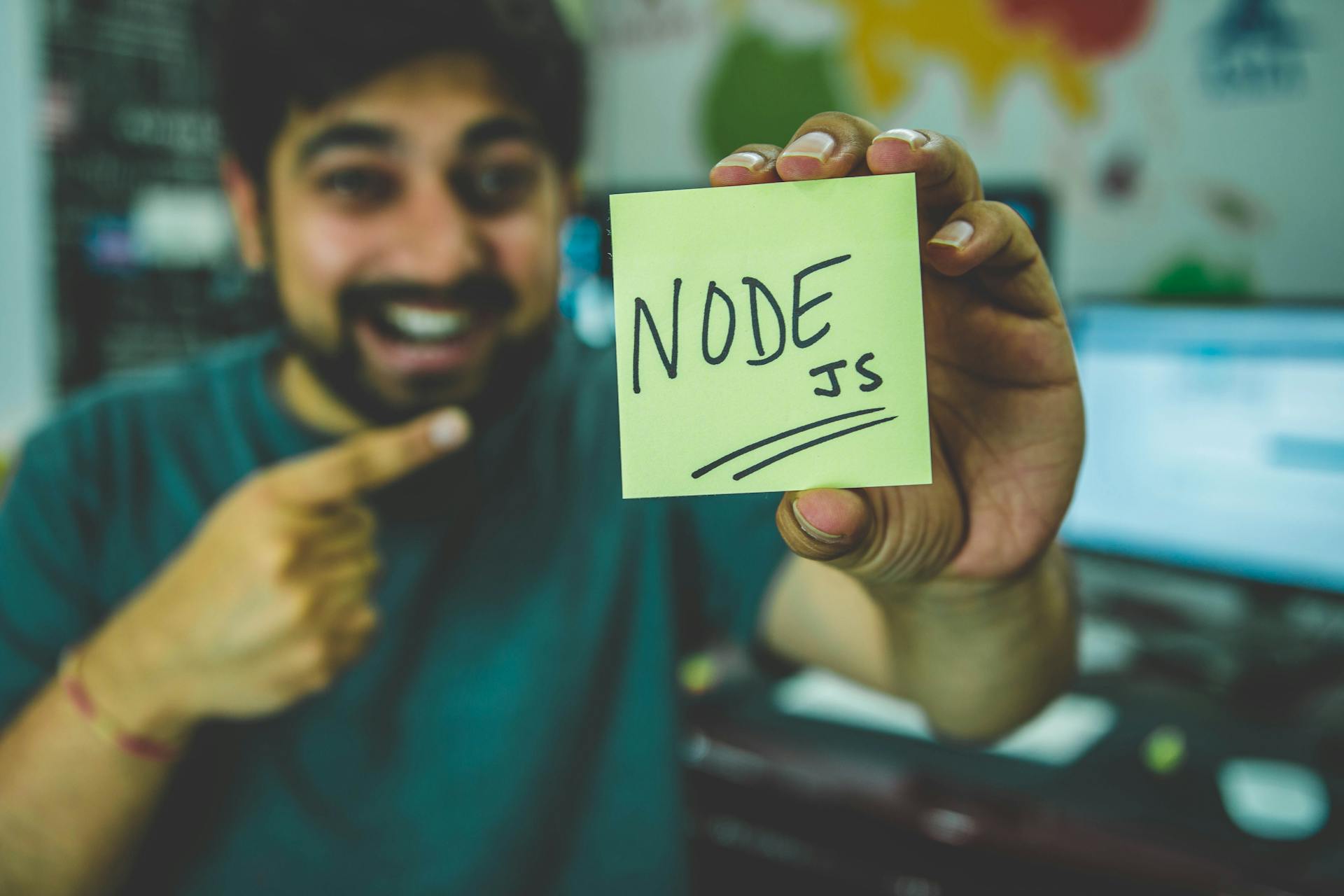
To set up Next JS Opentelemetry, you'll need to install the opentelemetry-api package using npm or yarn.
First, create a new Next JS project using the command npm create next-app my-app.
Next, install opentelemetry-api by running npm install opentelemetry-api or yarn add opentelemetry-api.
The opentelemetry-api package provides the necessary APIs for instrumenting your Next JS application.
Additional reading: Using State in Next Js
Introduction
OpenTelemetry is a powerful observability framework that allows you to collect traces, metrics, and logs from your application, providing a comprehensive view of its performance.
With OpenTelemetry, you can see the journey of a request through your application, which is represented by traces.
OpenTelemetry offers quantitative measurements of various aspects of your application through metrics.
Logs provide detailed information for debugging, helping you quickly identify and fix issues.
By using OpenTelemetry with Next.js, you can gain a deeper understanding of your application's performance and make data-driven decisions to improve it.
Instrumenting Applications
To instrument your Next.js application, you need to tell Next.js that you will be using an EXPERIMENTAL feature by adding lines to your next.config.js file.
First, you need to create an instrumentation.ts file in the same level as your pages folder, or in the src folder if it exists.
Instrumenting existing projects can be a bit tricky, but with the right tools, it's achievable.
Intriguing read: What Is .next Folder in Next Js
Install Packages
To get started with instrumenting your application, you'll need to install the necessary OpenTelemetry packages. These packages provide the core functionality needed to instrument your application.
You can install the OpenTelemetry packages using the command provided in the example. This will give you the foundation you need to start instrumenting your Next.js application.
Installing OpenTelemetry packages is a crucial step in the process, and it's essential to do it correctly to avoid any issues down the line.
If you want to instrument your project from scratch, you can use the command to create a new Next.js project using the OpenTelemetry example. This will give you a head start on the process.
Worth a look: Install Next Js
Instrumenting Existing Projects
Instrumenting existing projects can be a bit of a challenge, but it's a crucial step in getting OpenTelemetry up and running. To start, you need to tell Next.js that you'll be using an EXPERIMENTAL feature by adding a specific line to your next.config.js file.
You'll also need to create an instrumentation.ts file in the same level as your pages of app folder, or in your src folder if you have one. This file is where you'll write the code that instruments your application.
As you begin to instrument your project, remember that it's a process that requires patience and attention to detail. Take your time, and don't be afraid to ask for help if you need it.
Monitoring and Analysis
Monitoring resource consumption and error rates is a top priority for maintaining a healthy Next.js application. OpenTelemetry provides the tools to monitor these metrics effectively.
Resource consumption and error rates are critical metrics for maintaining the health of your application. You can optimize resource usage and minimize errors by using OpenTelemetry.
Analyzing performance metrics is a crucial step in understanding your application's behavior and performance. Run your Next.js application and analyze the collected performance metrics to gain insights.
Monitoring response times is crucial for ensuring a smooth user experience. OpenTelemetry allows you to track response times and other performance metrics efficiently.
Configuring and Exporting
Configuring and exporting OpenTelemetry for Next.js involves some careful setup, especially when working with the Vercel Edge Runtime. This runtime has limited support for standard Node APIs, which can make it tricky to get tracing working.
To export telemetry data, you'll need to configure the OpenTelemetry exporter, which sends the collected data to an external system for analysis. The exporter is a crucial part of the OpenTelemetry setup.
You can leverage the npm package @vercel/otel to connect your instrumentation hook for export, which bundles both @opentelemetry/webjs and @opentelemetry/nodejs depending on the Vercel runtime. This saves a lot of time and effort when getting tracing working across runtimes.
You might enjoy: Next Js Fetch Data save in Context and Next Route
Configuring Exporters
To export telemetry data to your observability tools, you need to configure the OpenTelemetry exporter. The exporter sends the collected telemetry data to an external system for analysis.
The OpenTelemetry exporter is a crucial part of the tracing setup, and it's essential to choose a compatible exporter for your environment. In the case of Vercel Edge Runtime, you can leverage the @vercel/otel package to connect your instrumentation hook for export.
Broaden your view: Next Js Build Collecting Page Data
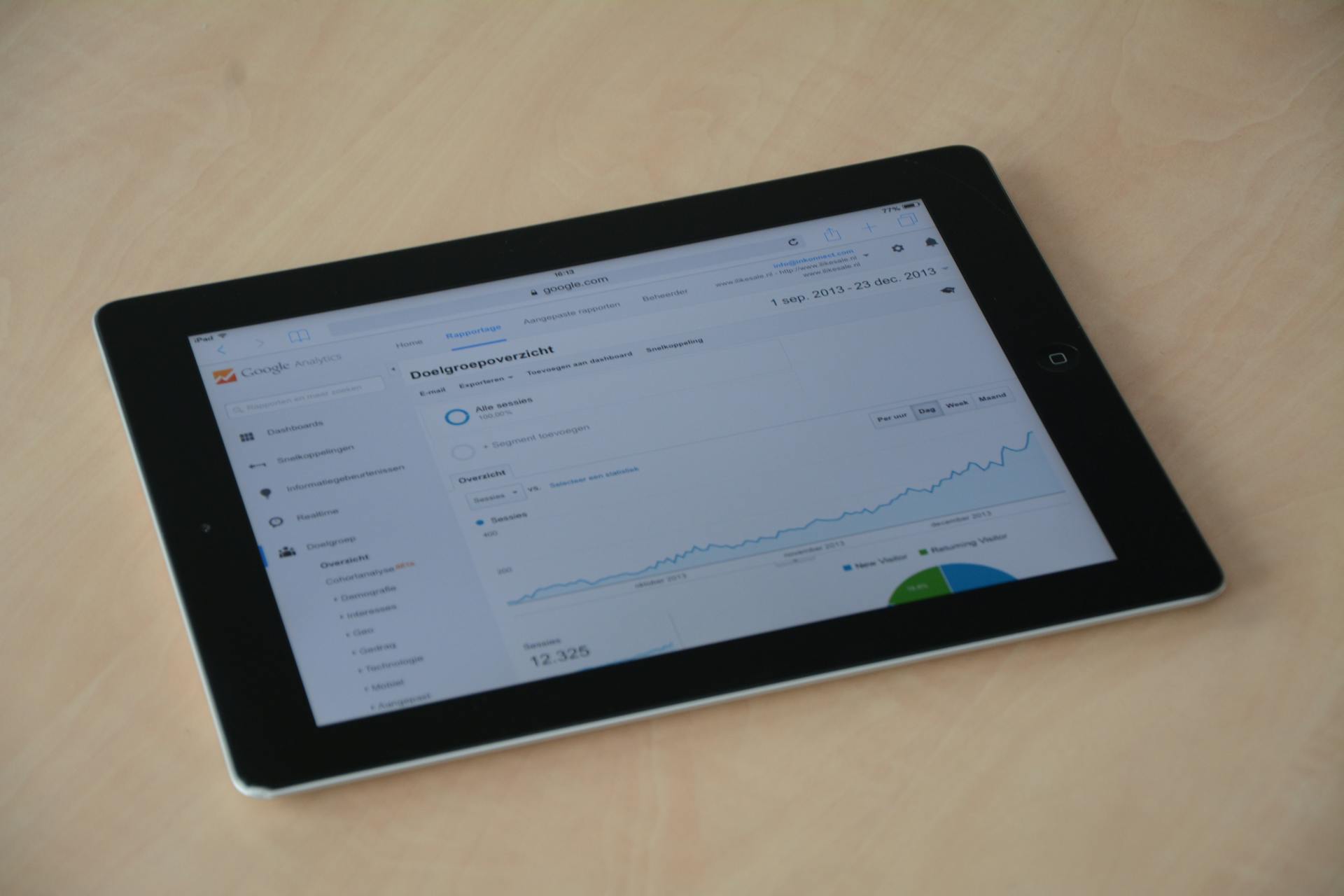
To get tracing working across runtimes, you can use the @vercel/otel package, which bundles both the edge runtime compatible @opentelemetry/webjs and @opentelemetry/nodejs depending on the Vercel runtime where the code is running. This saves a lot of time and effort when setting up tracing.
Don't forget to enable the instrumentation hook in the next.config.js file to complete the setup.
Exporting Traces from Vercel Edge Runtime
Exporting Traces from Vercel Edge Runtime is a crucial step in debugging and optimizing your application's performance. This process involves configuring the OpenTelemetry SDK to capture and send data to a telemetry backend.
The Vercel Edge Runtime has constraints with Node APIs, which means the setup usually involves initializing an OpenTelemetry tracer, configuring an exporter that is compatible with the Edge environment, and ensuring that traces are efficiently batched and sent without overwhelming the network.
Fortunately, Vercel's latest work in this space has made it easier to get tracing working across runtimes. You can leverage the npm package @vercel/otel to connect your instrumentation hook for export.
This package bundles both the edge runtime compatible @opentelemetry/webjs and @opentelemetry/nodejs depending on the Vercel runtime where the code is running, which saves a lot of time and effort. Don't forget to enable the instrumentation hook in the next.config.js file.
Additional reading: Nextjs Instrumentation
Best Practices and Tips
To get the most out of Next.js OpenTelemetry, follow these best practices and tips. Enable detailed logging for your OpenTelemetry setup to facilitate debugging. This will help you trace issues back to their source and adjust your configuration as needed.
By doing so, you'll be able to identify and fix problems more efficiently.
Telemetry Best Practices
Efficient telemetry data collection involves balancing the amount of data collected with the overhead introduced.
Sampling techniques can be a game-changer for optimizing performance. They help reduce the amount of data collected while still providing valuable insights.
Limiting the granularity of spans is another effective way to optimize performance. This means collecting data at a less detailed level, which can significantly reduce the overhead.
Balancing data collection with overhead is crucial for efficient telemetry. If you collect too much data, it can slow down your system.
Broaden your view: Next Js Reduce Unused Javascript
Effective Debugging Tips
Effective debugging is a crucial part of any development process. Enabling detailed logging for your OpenTelemetry setup is a game-changer for troubleshooting issues.
Using detailed logs, you can trace problems back to their source and adjust your configuration as needed. This makes it much easier to identify and fix errors.
By following these simple tips, you'll be well on your way to becoming a debugging master.
Frequently Asked Questions
What is OpenTelemetry?
OpenTelemetry is an open-source standard for collecting and exporting telemetry data from cloud-native applications and infrastructure. It simplifies the process of gathering metrics, traces, and logs from distributed systems.
Sources
- https://www.checklyhq.com/blog/in-depth-guide-to-monitoring-next.js-apps-with-opentelemetry/
- https://www.highlight.io/blog/lw5-vercel-otel-nextjs-tracing
- https://www.dhiwise.com/post/a-comprehensive-guide-to-implementing-nextjs-opentelemetry
- https://axiom.co/docs/guides/opentelemetry-nextjs
- https://medium.com/rescale/optimizing-next-js-with-opentelemetry-f80b8028b0e3
Featured Images: pexels.com