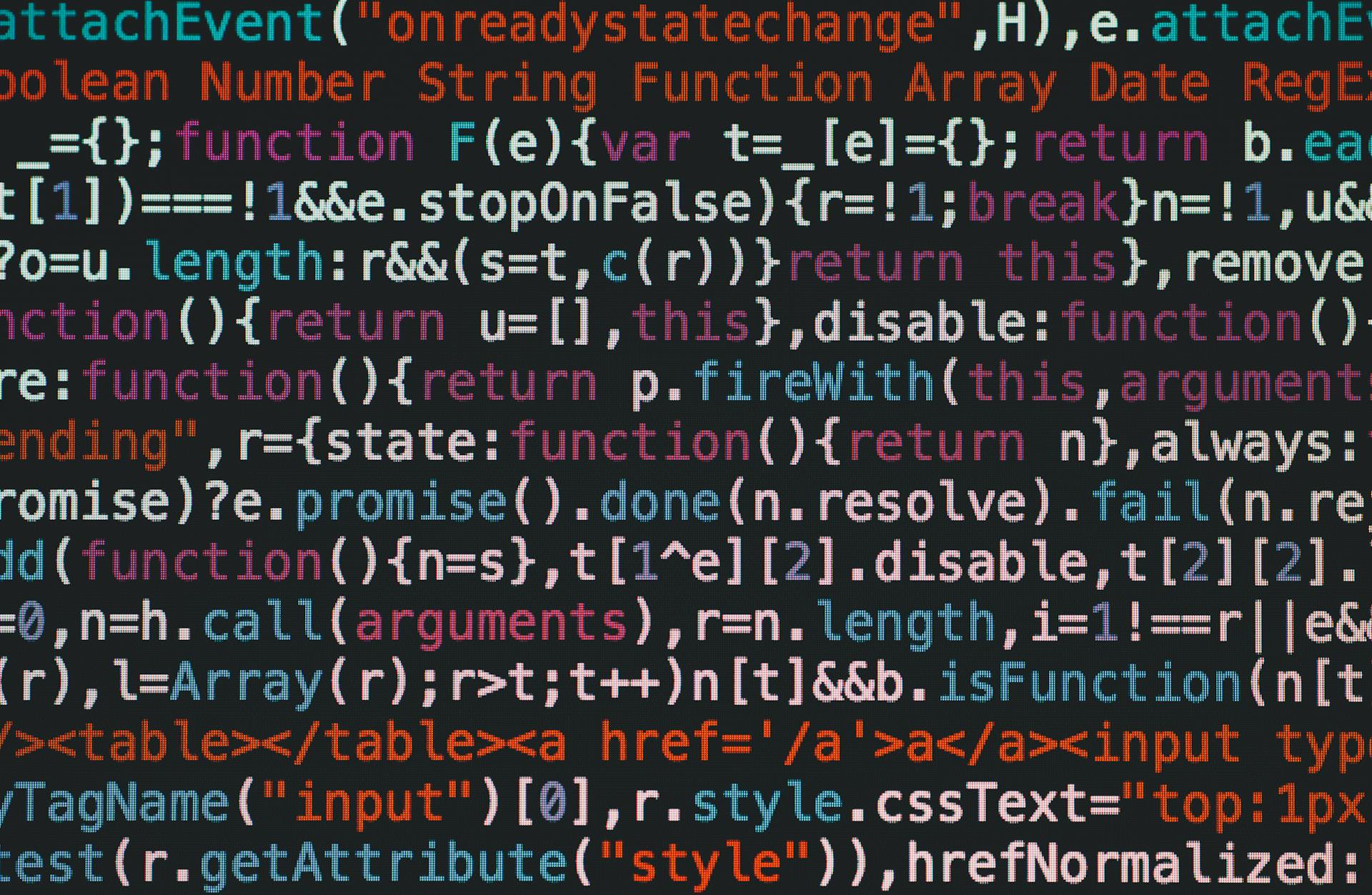
Reducing unused JavaScript in Next.js can be a game-changer for improved speed. By eliminating unnecessary code, you can significantly decrease page load times and enhance user experience.
Unused JavaScript can be a major culprit when it comes to slow page loads. As explained in the article, a large portion of unused code can be attributed to dynamic imports.
To tackle this issue, Next.js provides a built-in feature called code splitting. This allows you to split your code into smaller chunks, which are only loaded when needed. This approach is particularly effective for large applications with many features.
By leveraging code splitting, you can ensure that only the necessary code is loaded, resulting in faster page loads and improved performance.
Optimizing Next.js
Optimizing Next.js is crucial to reducing unused JavaScript. The MUI package exports a lot of components, making it unnecessary to import all packages.
Importing only the necessary components can significantly reduce the size of the JavaScript file. This is demonstrated by importing only the Badge component instead of the entire MUI package.
Running Lighthouse reports can help identify areas for improvement and measure the impact of changes. By running another Lighthouse report after making changes, you can prove that the size of the JavaScript file has been reduced.
Tree-shaking is a technique that can be used to remove unused code, but it's not recommended in this case. The example shows that importing only the necessary component can achieve the same result without using tree-shaking.
Analyzing and Fixing Issues
Analyzing and fixing issues is a crucial step in reducing unused JavaScript in Next.js. This step can have the biggest impact, but it can also take the longest due to various factors. Thanks to tools like the bundle-analyzer and source-map-explorer, we have a clear view of what's going on in the bundle and what could be changed.
To identify the main culprits, look for large imported files and a high number of small files. A good starting point is to examine libraries like validator, framer, mixpanel, fontawesome, and react-code-blocks, which can take up a lot of space without providing significant benefits.
By making smarter library selection choices, you can achieve impressive results. For instance, removing the Validator library and replacing it with lodash isEmpty reduced the bundle size by 28.054%. Similarly, removing the Mixpanel dependency and using other tools for data collection led to a 29.612% reduction in bundle size.
Here are some common suspects to look out for:
- Remove unneeded or trivial plugins.
- Remove dead code.
- Rewrite poorly coded scripts.
- Clean up your tag manager and restrict access.
- Remove unnecessary imports.
- Lazy load routes or components.
- Defer loading of non-critical scripts.
By addressing these issues, you can significantly reduce unused JavaScript and improve your website's performance.
Lighthouse Warning Definition
The 'reduce unused JavaScript' warning in Lighthouse flags scripts with more than 20kb of unused bytes. This warning directly impacts the Lighthouse score.
Unused JavaScript can slow down Core Web Vitals, especially the largest contentful paint (LCP) and the interaction to next paint (INP).
How to Fix
Analyzing and fixing issues can be a daunting task, but with the right approach, you can achieve significant improvements. You can start by identifying the root cause of the problem, just like analyzing a bundle to see what's causing the largest impact.
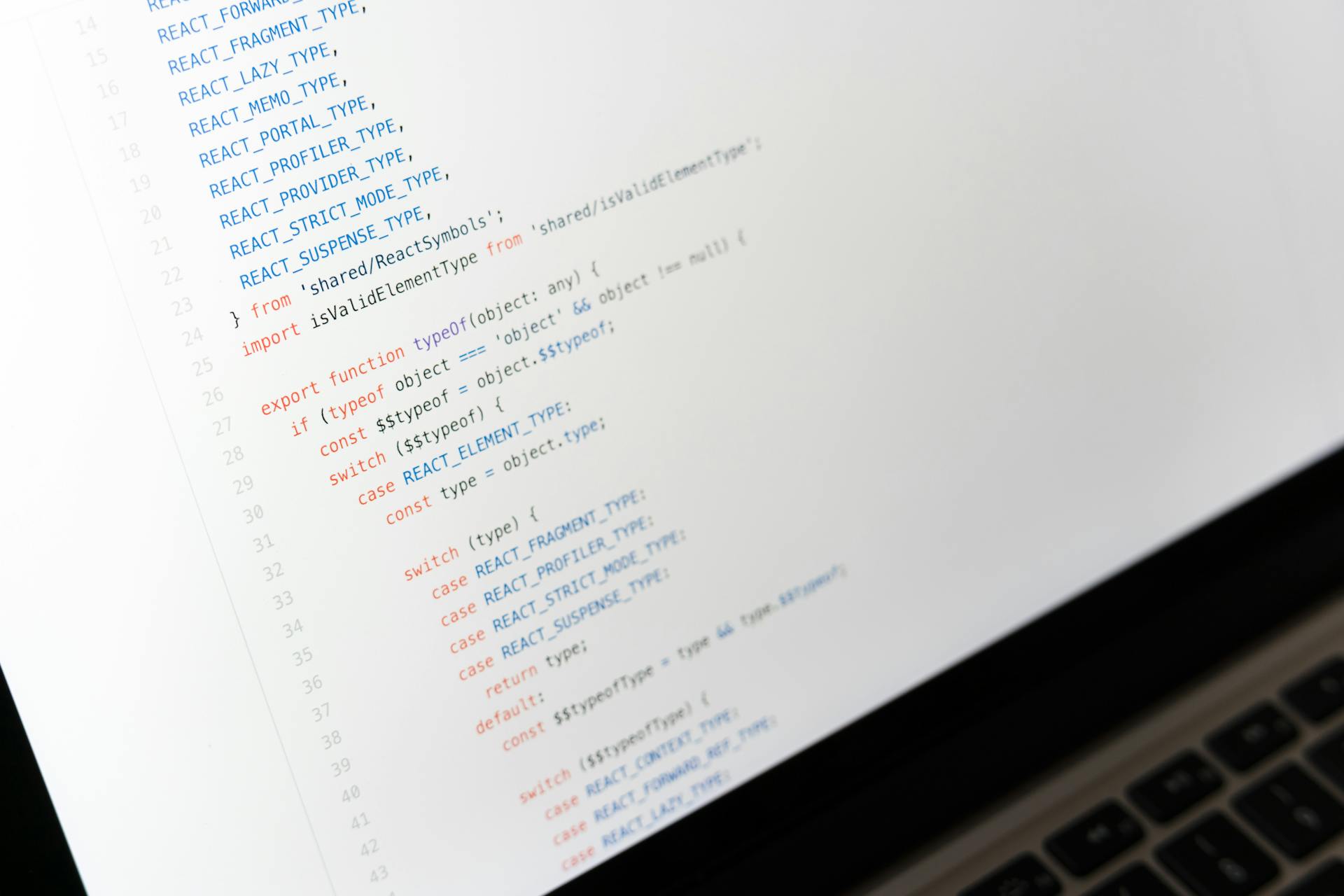
The bundle-analyzer and source-map-explorer can provide a clear view of what's going on in the bundle and what could be changed. This can help you identify large imported files and many small files, which can be optimized.
Removing unnecessary imports is a crucial step in fixing issues. In one case, the author removed the mixpanel library since the data was not used, resulting in significant gains. Similarly, removing unused code can also make a big difference.
You can also try rewriting poorly coded scripts, which can have a lot of unneeded checks and if/else statements. This can be a good opportunity to revisit older scripts and make them more efficient.
Here are the 5 usual suspects that can be causing unused JavaScript:
By following these steps, you can significantly improve the performance of your website and fix issues related to unused JavaScript. Remember to also consider lazy loading routes or components and deferring loading of non-critical scripts.
Improving Performance
Analyzing the bundle can have a significant impact on performance, with the potential to reduce bundle size by up to 30%. This can be achieved by identifying large imported files and unnecessary libraries, such as the Validator library, which took up too much space for its use.
By optimizing library selection, removing unused libraries, and replacing them with vanilla TypeScript, it's possible to achieve significant gains in performance. For example, the author of the article was able to reduce the bundle size from 1.59 MB to 1.45 MB, a reduction of 29.612%.
Here are some specific actions that can be taken to improve performance:
- Remove unused libraries and replace them with more efficient alternatives
- Optimize library selection to reduce bundle size
- Use tools like Next Bundle Analyzer to identify performance bottlenecks
Unused JavaScript can also have a significant impact on performance, competing for network resources and slowing down the browser. By removing unused JavaScript, it's possible to improve the largest contentful paint (LCP) and interaction to next paint (INP).
Analyze Chunks with Next Bundle
Analyzing chunks with Next Bundle is a crucial step in optimizing your application's performance. By using the @next/bundle-analyzer package, you can examine the chunks listed in the Lighthouse report and gain a deeper understanding of what's taking up space in your bundle.
To get started, install the @next/bundle-analyzer package as a dev dependency. This tool will only be used during build time, so it's essential to install it correctly. The package will help you visualize the chunks in your application and identify areas for improvement.
By running the bundle analyzer on the production build, you'll generate a file called client.html that opens up in your browser. You can ignore any other files that open, as they're not relevant to your analysis. The client.html file will present a pretty visualization of colored blocks, where the size of each block represents how much space it takes up.
You can clearly identify which packages a chunk contains by looking at the hashed chunk names and popover showing the size for each chunk. For example, chunk 609-52e1ddd0e27842fe.js contains the MUI package.
Here's a list of steps to follow:
- Install the @next/bundle-analyzer package as a dev dependency.
- Modify your project's next.config.js file to add support for the bundle analyzer.
- Run the bundle analyzer on the production build.
By following these steps, you'll be able to analyze your chunks and make data-driven decisions to improve your application's performance.
Affecting Page Speed
Unused JavaScript can really slow down your page speed. It makes rendering a webpage unnecessarily complicated, which can make it hard to get a high score on Lighthouse.
Unused JavaScript needs to be downloaded, which can compete for network resources and have a huge impact on the Largest Contentful Paint (LCP).
Unused JavaScript also needs to be executed by the browser, which can cause the browser to freeze and stop responding to user input. This can affect both the Largest Contentful Paint (LCP) and the Interaction to Next Paint (INP).
Here are the two problems with unused JavaScript:
- Unused JavaScript needs to be downloaded, competing for network resources and affecting the Largest Contentful Paint (LCP).
- Unused JavaScript needs to be executed by the browser, causing the browser to freeze and stop responding to user input, affecting both the Largest Contentful Paint (LCP) and the Interaction to Next Paint (INP).
Reduce
Reducing unused JavaScript in Next.js can be a daunting task, but it's essential for improving your website's performance. Make sure you only import code you need from packages, so tree-shaking can remove any unnecessary code. This can be achieved by importing specific functions or methods from a library, rather than importing the entire library.
Unused JavaScript can compete for network resources and block the main thread, slowing down your website's Core Web Vitals. To fix this issue, remove dead code and defer code that is not immediately needed until it is needed. This can be done by dynamically loading packages or code that is only required when a user interacts with your website.
Optimizing imports can have a significant impact on file sizes. For example, importing a specific method from Lodash can reduce the file size by 10 times. The recommended way to import methods from Lodash is by importing specific functions or methods, rather than importing the entire library.
Dynamic imports can also help reduce unused JavaScript. Next.js supports ES2020 dynamic imports, which allow you to dynamically import components that are not shown by default. This can be achieved by using the `dynamic` function to import components only when they are needed.
Tree-shaking is another technique that can help reduce unused JavaScript. By only importing the components or code that you need, you can avoid importing unnecessary code that can slow down your website. For example, if you only need to use a simple Badge component, you can import it separately, rather than importing the entire MUI package.
Before proceeding with any optimization, it's essential to start with an inventory. This will help you measure your efforts and justify the time spent on a task. You can use tools like Next-Compose-Plugin, next-bundle-analyzer, and source-map-explorer to analyze and visualize your bundle.
Here are some actionable steps to reduce unused JavaScript:
- Make sure you only import code you need from packages
- Dynamically load packages or code that is only required when a user interacts with your website
- Optimize imports by importing specific functions or methods from libraries
- Use tree-shaking to avoid importing unnecessary code
- Use dynamic imports to load components only when they are needed
By following these steps, you can significantly reduce unused JavaScript in your Next.js website and improve its performance. Remember to always start with an inventory and use tools to analyze and visualize your bundle.
Sources
- https://www.coteries.com/en/articles/reduce-size-nextjs-bundle
- https://www.charlievuong.com/fix-lighthouse-reduce-unused-javascript-using-next-js-bundle-analyzer
- https://www.corewebvitals.io/pagespeed/reduce-unused-javascript-lighthouse
- https://nextjs.org/docs/app/api-reference/config/eslint
- https://nextjs.org/docs/pages/api-reference/next-config-js/output
Featured Images: pexels.com