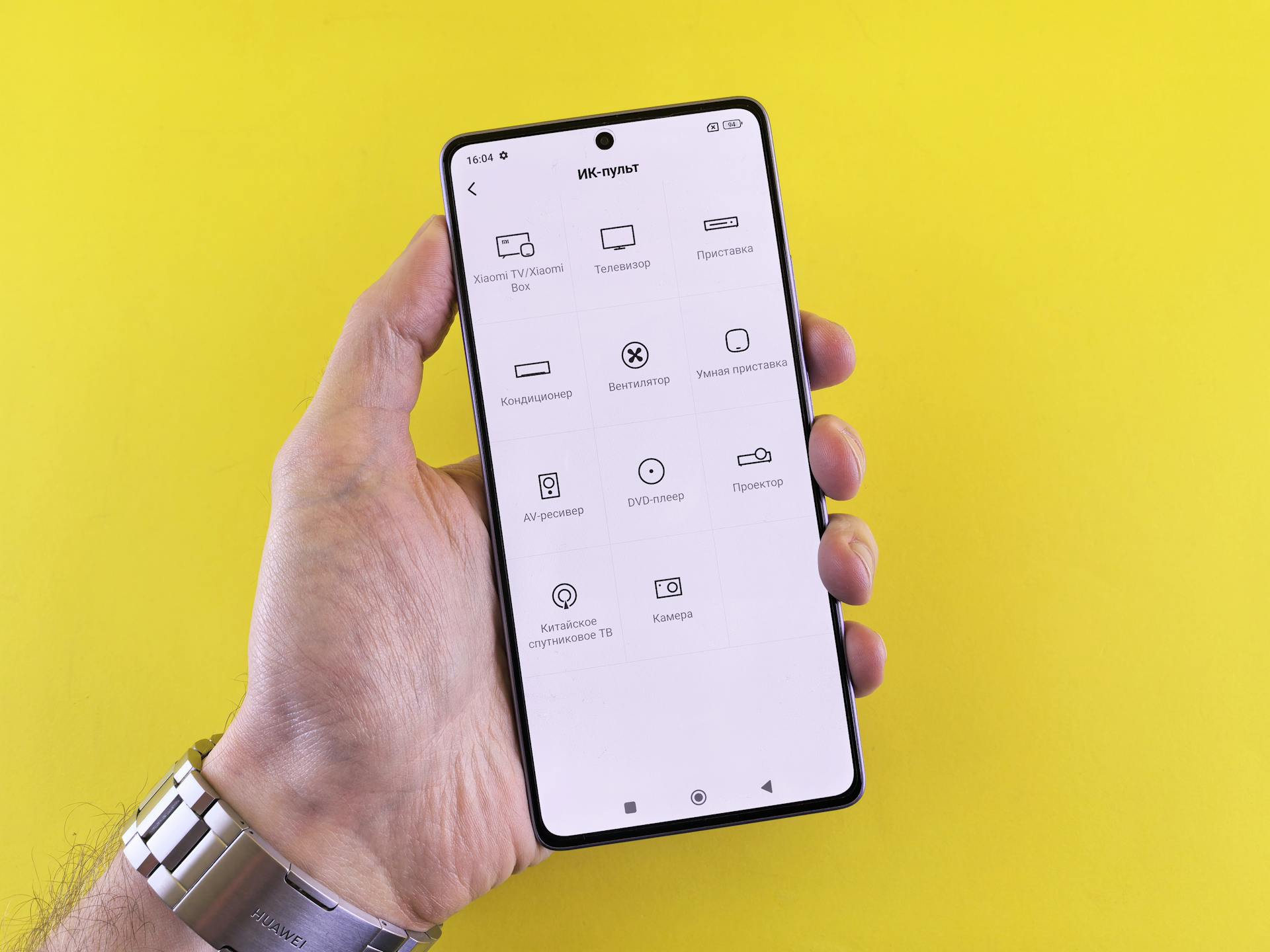
Getting started with Next.js instrumentation is a breeze, thanks to its intuitive configuration options. You can start by installing the `@next/directories` package, which is required for Next.js instrumentation.
Next.js provides a built-in `next.config.js` file that serves as the central configuration point for your application. This file is where you'll define your instrumentation settings.
To enable instrumentation, you'll need to add the `instrument` option to your `next.config.js` file. This option is set to `true` by default, but you can customize it to suit your needs.
Installation and Setup
To install and set up instrumentation for your Next.js application, start by installing the New Relic Node.js agent. Run the following command in your project to get started: `npx @newrelic/nr-agent install`. This will include the necessary dependencies in your package.json file.
As of version 12.0.0 of the New Relic Node.js agent, the @newrelic/next package is included by default, providing official instrumentation for monitoring Next.js applications.
For another approach, see: New Relic Nextjs
You can also set up the OpenTelemetry SDK for your Next.js app by creating an instrumentation.ts or instrumentation.js file in your project's root folder. This file will initialize the SDK and set up the necessary instrumentation.
To get started with OpenTelemetry, install the necessary packages by running the following command: `npm install @opentelemetry/api @opentelemetry/sdk-node @vercel/otel`. You can also use the @vercel/otel package for easier setup, which simplifies the configuration process.
Here are the required packages you'll need to install for OpenTelemetry:
- @opentelemetry/api: Provides the OpenTelemetry API for managing traces, metrics, and other telemetry data.
- @opentelemetry/sdk-node: The OpenTelemetry SDK for Node.js, which collects and exports telemetry data.
- @vercel/otel: A package from Vercel that simplifies OpenTelemetry setup and configuration for Next.js apps, especially when deploying on Vercel.
If you're using the @vercel/otel package, create an instrumentation.ts file in the root of your project with the following content: `import { registerOTel } from '@vercel/otel'; registerOTel('your-app-name');`. Don't forget to add the API_TOKEN and DATASET_NAME environment variables to your .env file.
Remember to check the prerequisites and initial setup steps for your specific Next.js project.
Configuration and Options
To effectively instrument a Next.js application, you need to modify the next.config.js file. This configuration ensures that the modules supported by New Relic are not mangled by webpack, and it externalizes those modules.
You'll need to add the following content to your next.config.js file. Additionally, you'll need to update your dev and start npm scripts by amending the scripts section of your package.json file. This will allow your application to run with Node's -r option, which will preload @newrelic/next middleware.
To set up Honeybadger, you'll need to create a configuration file for each Next.js runtime. This includes the honeybadger.server.config.js, honeybadger.client.config.js, and honeybadger.edge.config.js files. You can generate these files using a command that will add them to your project root.
The following files will be added to your project:
- honeybadger.server.config.js - Configuration file for Next.js server runtime
- honeybadger.client.config.js - Configuration file for Next.js client runtime
- honeybadger.edge.config.js - Configuration file for Next.js Edge runtime
- pages/_error.[js|tsx] - Next.js Pages Router custom error component - if pages folder exists
- app/error.[js|tsx] - Next.js App Router custom error component - if app folder exists
- app/global-error.[js|tsx] - Next.js App Router global error component - if app folder exists
Note that the honeybadger.edge.config.js file is necessary if you deploy your Next.js application to Vercel and use Vercel Edge Functions.
Imported Libraries
The OpenTelemetry SDK for Node.js provides a comprehensive set of tools for instrumenting Node.js apps, including automatic instrumentation for popular libraries and frameworks, as well as APIs for manual instrumentation.
You'll need to import this SDK to configure and initialize OpenTelemetry in your Next.js setup.
For more insights, see: Next Js Opentelemetry
@opentelemetry/semantic-conventions is a set of standard attributes and conventions for describing resources, spans, and metrics in OpenTelemetry, making your app's telemetry data more consistent and interoperable with other OpenTelemetry-compatible tools and systems.
This convention is essential for getting the most out of OpenTelemetry.
@vercel/otel is a package provided by Vercel that simplifies the setup and configuration of OpenTelemetry for Next.js apps deployed on the Vercel platform, abstracting away some boilerplate code and providing a more streamlined integration experience.
This package is particularly useful for Next.js apps deployed on Vercel.
Configuration
To set up your Next.js application with New Relic, you need to modify the next.config.js file.
You'll need to add the following content to the next.config.js file in your project root. This configuration ensures that the modules supported by New Relic are not mangled by webpack, and it externalizes those modules.
Modify your dev and start npm scripts by amending the scripts section of package.json file. This will allow your application to run with Node’s -r option, which will preload @newrelic/next middleware.
You'll also need to add the newrelic.jsAMP agent configuration file to the root directory of your project. This file should be named newrelic.jsAMP and should be placed in the root directory of your project.
To get started with Honeybadger, you'll need to create a configuration file for each Next.js runtime. You can do this by running a command that generates configuration files in your project root for each Next.js runtime.
Here are the configuration files that will be added to your project:
- honeybadger.server.config.js - Configuration file for Next.js server runtime
- honeybadger.client.config.js - Configuration file for Next.js client runtime
- honeybadger.edge.config.js - Configuration file for Next.js Edge runtime
- pages/_error.[js|tsx] - Next.js Pages Router custom error component - if pages folder exists
- app/error.[js|tsx] - Next.js App Router custom error component - if app folder exists
- app/global-error.[js|tsx] - Next.js App Router global error component - if app folder exists
If you deploy your Next.js application to Vercel and use Vercel Edge Functions, you'll need to keep the honeybadger.edge.config.js file. Otherwise, you can safely remove it.
To upload source maps to Honeybadger, ensure that disableSourceMapUpload is set to false and that apiKey and assetsUrl properties are set in webpackPluginOptions. The value of assetsUrl should be the URL to your domain suffixed with _next.
Custom
Custom instrumentation for server-side code is particularly useful for monitoring API routes and server-side rendering. You can achieve this by modifying your next.config.js file.
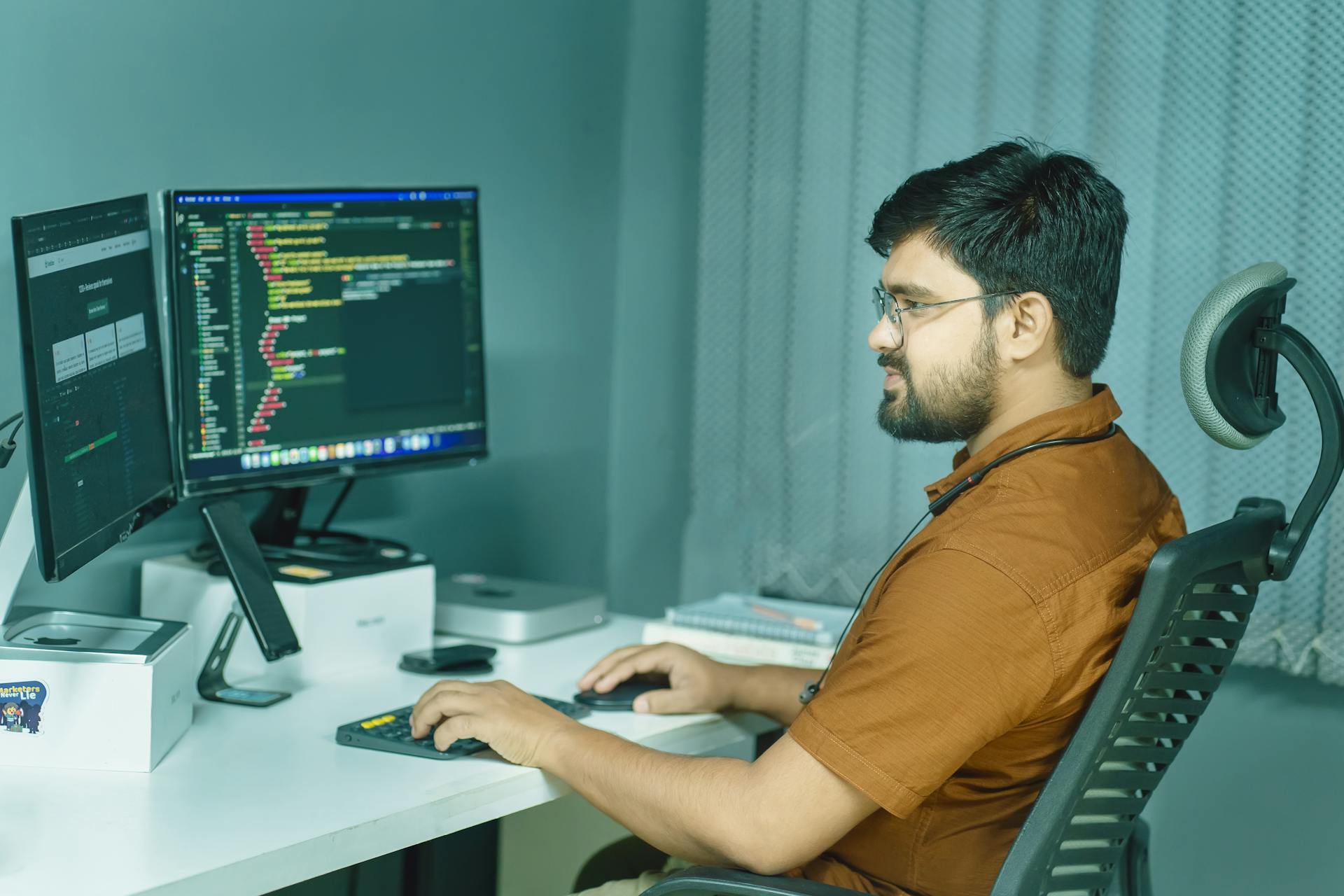
To effectively instrument a Next.js application, you need to modify the next.config.js file, as mentioned in the configuration section. This configuration ensures that the modules supported by New Relic are not mangled by webpack, and it externalizes those modules.
Instrumenting server-side code helps track performance and identify bottlenecks. You can do this by adding the newrelic.jsAMP agent configuration file to the root directory of your project.
Automatic instrumentation reduces the overhead of manually adding tracing code throughout your application. It ensures consistent and comprehensive data collection, helping you monitor various aspects of your application's performance, such as response times and error rates.
Related reading: Nextjs Performance
Error Handling and Reporting
Error handling and reporting is a crucial aspect of ensuring your Next.js application is reliable and stable. To capture detailed error information, you need to handle both client-side and server-side errors.
For client-side errors, you can use the error.ts(.js) file to capture and send error details to New Relic. This involves using the useEffect hook to call window.newrelic.noticeError whenever an error occurs.
The error.js(.ts) file defines an error UI boundary for a route segment, and to handle errors in the root layout, you should use global-error.js(.ts) and place it in the root app directory.
Server-side errors are handled automatically by the @newrelic/next module, so you don't need to add any additional code for server-side error tracking. This ensures that both client-side and server-side errors are effectively monitored and reported to New Relic.
Client-Side Errors
Client-side errors can be handled using the error.ts(.js) file to capture and send error details to New Relic. This is done by calling window.newrelic.noticeError whenever an error occurs.
The useEffect hook can be used to achieve this, making it a convenient and efficient solution. It's a powerful tool for capturing error details and sending them to New Relic for further analysis.
To handle errors in the root layout, you should use the global-error.js(.ts) file and place it in the root app directory. This will help ensure that errors are caught and reported correctly.
Server-Side Errors
Server-side errors can be tricky to handle, but fortunately, Next.js has got you covered. The @newrelic/next module automatically captures and reports server-side errors to New Relic.
To handle server-side errors, you don't need to add any additional code. This module will take care of it for you, ensuring that both client-side and server-side errors are effectively monitored and reported.
Server-side error tracking is crucial for identifying performance bottlenecks and improving your application's overall reliability. By leveraging the @newrelic/next module, you can gain valuable insights into your application's behavior and make data-driven decisions to optimize its performance.
Sources
- https://newrelic.com/blog/how-to-relic/how-to-monitor-app-based-router-nextjs-application
- https://staarter.dev/blog/opentelemetry-setup-for-nextjs-performance-monitoring
- https://docs.honeybadger.io/lib/javascript/integration/nextjs/
- https://www.dhiwise.com/post/a-comprehensive-guide-to-implementing-nextjs-opentelemetry
- https://axiom.co/docs/guides/opentelemetry-nextjs
Featured Images: pexels.com