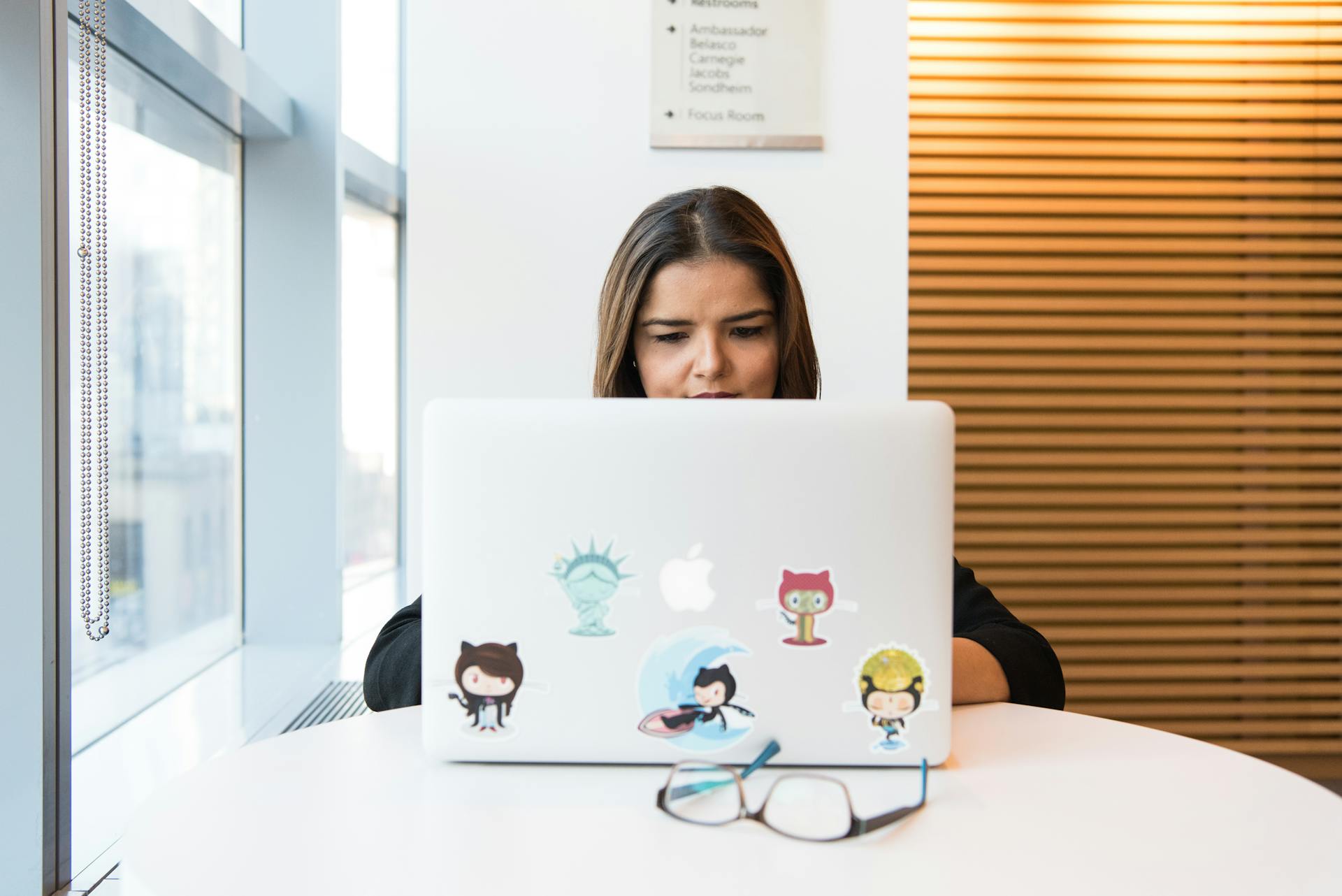
To set up New Relic for Next.js applications, you'll need to install the New Relic agent using npm or yarn. Simply run the command `npm install newrelic` or `yarn add newrelic` in your project directory.
New Relic's agent will automatically instrument your Next.js application, providing valuable insights into its performance and behavior. This includes metrics on page load times, server response times, and database query performance.
To enable monitoring, you'll need to configure the New Relic agent in your Next.js project. This typically involves adding a configuration file, such as `newrelic.config.js`, to your project root.
In this configuration file, you'll need to specify your New Relic API key and other settings. This will allow the New Relic agent to send data to your New Relic account and provide you with detailed performance metrics.
Worth a look: Newrelic Noticeerror
Installation and Setup
To install the New Relic Node.js agent for your Next.js application, you'll need to run a command in your project directory. This command will install the necessary dependencies, including the @newrelic/next package, which provides official instrumentation for monitoring Next.js applications.
Readers also liked: Next.js
The command to run is $ npm install newrelic, although as of version 12.0.0, the @newrelic/next package is included by default, eliminating the need for a separate installation.
You'll also need to create a newrelic.js config file at the root of your application, which you can use the newrelic.js example provided by newrelic-experimental to get started. Make sure to update the app_name and license_key with your own information.
Here's a quick rundown of the steps:
- Install the New Relic Node.js agent using npm
- Create a newrelic.js config file at the root of your application
- Update the app_name and license_key with your own information
Once you've completed these steps, you'll be well on your way to setting up New Relic for your Next.js application.
Install Your Packages
To install the necessary packages for New Relic, you'll need to run a command in your Next.js project. Specifically, you'll want to install the New Relic Node.js (APM) agent and New Relic middleware for Next.js using the following command: $ npm install newrelic.
This will install the dependencies, including the @newrelic/next package, which provides official instrumentation for monitoring Next.js applications. The integration with the New Relic Node.js agent offers all the agent's capabilities, enhancing performance monitoring and error tracking in Next.js applications.
If you're using Docker, you may need to install the library directly during build using the command RUN npm i @newrelic/next. This can help resolve issues with the module not being found.
To confirm that the dependencies have been installed, you should see them included in your package.json file.
If this caught your attention, see: Newrelic Nodejs
Local Dev Recap
You've successfully installed the New Relic Node agent and Next.js instrumentation. This is a crucial step in getting your application set up for performance monitoring.
To confirm everything is working correctly, run npm run dev and observe the New Relic logs populating your terminal. If everything boots up correctly, you can view data from your APM page.
Here are the key steps to review for a smooth local dev experience:
- Installed the New Relic Node agent and Next.js instrumentation
- Temporarily configured our dev script to bootstrap New Relic immediately
- Added a newrelic.js configuration file with the proper app_name and license_key
Once you've confirmed things are working locally, you can remove the NODE_OPTIONS='-r @newrelic/next' from the dev script.
Next.js Instrumentation
The New Relic Node.js agent provides instrumentation for Next.js, which offers telemetry for server-side rendering via getServerSideProps, middleware, and New Relic transaction naming for both page and server requests.
This instrumentation provides robust support for Next.js, with a minimum supported version of 12.0.9 for general Next.js usage and 12.2.0 for Next.js middleware.
To get started with Next.js instrumentation, you'll need to load the New Relic instrumentation and inject the browser agent.
Expand your knowledge: Nextjs Instrumentation
Here are the key steps to follow:
- Load the New Relic instrumentation in your Next.js application.
- Inject the New Relic browser agent to collect telemetry data on client-side actions.
By following these steps, you'll be able to leverage the power of New Relic to monitor and optimize your Next.js application's performance.
Here's a summary of the key benefits of Next.js instrumentation:
By implementing Next.js instrumentation, you'll be able to gain a deeper understanding of your application's performance and identify areas for improvement.
Troubleshooting
Troubleshooting can be a real challenge, especially when dealing with complex frameworks like New Relic Next.js. One common issue is a misconfigured Next.js environment, which can lead to errors like "Cannot find module 'next'".
To resolve this, make sure your Next.js version is compatible with New Relic. New Relic supports Next.js versions 10 and later. If you're using an earlier version, consider upgrading to a supported version.
Another issue that may arise is a missing or incorrect configuration in your `next.config.js` file. This can cause problems with data collection and performance monitoring. Double-check that you've correctly set up your New Relic configuration in this file.
Check this out: Hydration Issue Nextjs
If you're still experiencing issues, try checking your browser console for errors related to New Relic. This can help you identify the root cause of the problem. For example, if you see an error message indicating a missing script tag, it may be due to a configuration issue in your Next.js project.
Viewing and Sending Data
You can view your application's server-side data flowing into New Relic by going to the APM page. This will show you important performance metrics such as transaction time, Apdex score, throughput, and error rate.
To see client-side data, start your application and go to the browser monitoring page in New Relic. This will give you a visualization of important metrics such as core web vitals, user time on the page, load times, page views or throughput.
You can send detailed error information to New Relic by utilizing the _error.tsx page, which captures both backend and frontend errors and sends them to New Relic using the noticeError method. This method will pass the error along to New Relic as an argument.
Whether the code is run on the client or the server, the noticeError method will send the error information to New Relic. On the client side, since the New Relic browser agent attaches itself to the window object, the noticeError method is called on the window object.
Expand your knowledge: Next Js Build Collecting Page Data
Error Handling and Contextualization
Error handling is a crucial aspect of building a robust Next.js application. You can send detailed error information to New Relic by utilizing the _error.tsx page.
To capture both backend and frontend errors, update the getInitialProps prototype of the error function to send them to New Relic using the noticeError method. This method will pass the error along to New Relic as an argument, regardless of whether the code is running on the server or client side.
New Relic UI displays error details with stack traces and error attributes like error code and message. On the client side, the noticeError method is called on the window object, which is already attached to the New Relic browser agent.
For client-side errors, you can use the error.ts(.js) file to capture and send error details to New Relic. This is done by calling window.newrelic.noticeError whenever an error occurs using the useEffect hook.
Server-side errors are handled automatically by the @newrelic/next module, so you don't need to add any additional code for server-side error tracking. This ensures comprehensive error tracking for your Next.js application.
Related reading: Nextjs Server Only
To put your Next.js logs in context, you can use the Winston logging framework. Update your newrelic.js config file to forward all application logs and automatically link them to spans, traces, and other telemetry data.
You'll also need to enable distributed tracing in the agent configuration to integrate with the Winston logging framework. This allows you to gather information from subpages and use the logger in methods and files that are pre-rendered on the backend.
With Winston and log statements added to your application, you can run the application and see the results in New Relic under Logs. Automatic correlation between transactions and logs is also possible, giving you additional context to understand your application better.
Worth a look: Nextjs Logging
Agent and Middleware
Installing the New Relic Node.js agent and middleware for Next.js is a straightforward process. You can run the following command in your Next.js project to install the necessary packages.
The command is the same regardless of the version of the New Relic Node.js agent you're using, as of version 12.0.0 the @newrelic/next package is included by default, eliminating the need for a separate installation. This built-in support provides official instrumentation for monitoring Next.js applications, focusing on server-side rendering, middleware, and transaction naming for both page and server requests.
A different take: Nextjs Loading Indicator Server
You'll see the dependencies included in your package.json file after the command completes successfully. This integration offers all the agent's capabilities, enhancing performance monitoring and error tracking in Next.js applications.
The integration with the New Relic Node.js agent provides comprehensive observability of server-side activities, including server-side rendering, middleware, and transaction naming. This ensures you have a clear view of your application's performance.
While the New Relic Node.js agent covers server-side activities, it doesn't cover client-side actions. You can inject the New Relic browser agent for client-side telemetry, but that's a separate step.
Here are the steps to install the New Relic Node.js agent and middleware for Next.js:
- Run the following command in your Next.js project: `npm install newrelic` or use your favorite npm-based package manager.
- The command will add the dependencies to your package.json file.
- As of version 12.0.0, the @newrelic/next package is included by default, providing official instrumentation for monitoring Next.js applications.
Deploying and Environment
Having a newrelic.js file for each environment is the solution we landed on. This maintains our slightly modified Next.js 'with-docker' file and doesn't require any extra libraries.
We set specific newrelic.js environment files by having a docker-compose argument that uses a .env file with a file path variable for the newrelic.js file to copy.
Our Dockerfile is updated with a file path argument, keeping the flow fairly transparent.
Frequently Asked Questions
What is Newrelic for?
New Relic is a tool that helps you understand how your website performs and how users interact with it, identifying issues that slow it down or cause errors. It provides real-time insights into page load times, user behavior, and backend errors to optimize your web app's performance.
Sources
- https://newrelic.github.io/node-newrelic/
- https://wsfuller.medium.com/new-relic-next-js-backend-observability-with-docker-f6c61d87aea3
- https://newrelic.com/blog/how-to-relic/nextjs-monitor-application-data
- https://newrelic.com/blog/how-to-relic/how-to-monitor-app-based-router-nextjs-application
- https://dev.to/set808/how-to-monitor-app-router-nextjs-applications-with-new-relic-ghp
Featured Images: pexels.com