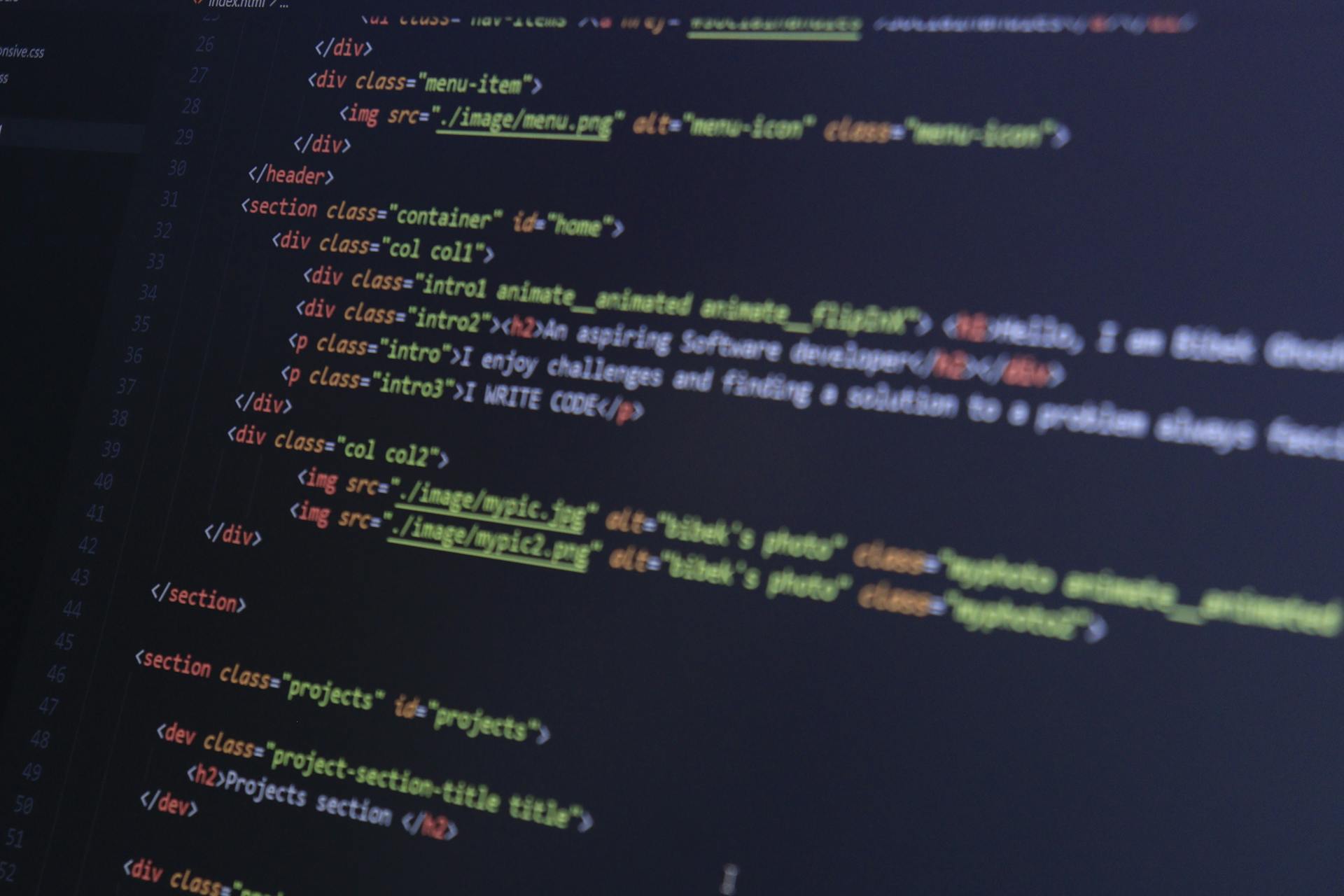
Building Nextjs AI apps with authentication and chat is a game-changer for developers. With Nextjs, you can create scalable and performant server-rendered applications that integrate AI capabilities seamlessly.
Nextjs provides a robust framework for building server-rendered applications, which is essential for AI-powered apps that require real-time data processing. By using Nextjs, you can create apps that render content on the server, reducing the load on the client-side and improving overall performance.
To add authentication to your Nextjs AI app, you can use popular libraries like NextAuth. NextAuth provides a simple and secure way to authenticate users, allowing you to focus on building your app's AI capabilities.
With NextAuth, you can easily integrate authentication into your Nextjs app, providing a seamless user experience for your users.
Related reading: With Ai Is Nextjs Relevant Anymore
Getting Started
You can create a full-stack AI-powered Journal app from scratch in Next.js, which will help you see how all the pieces of Next.js/React fit together.
This course is part of a larger collection available through the Frontend Masters video subscription.
To get started, you'll be building an AI-powered application that allows users to log in and manage journal entries with standard CRUD operations, and the final version can be found in the GitHub repo linked below.
The application will store data in a serverless Prisma DB on PlanetScale and integrate the OpenAI API and Langchain.
Broaden your view: Nextjs Spa
Course Description
To create a full-stack AI-powered Journal app, you'll start with a Next.js course that takes you from scratch. You'll learn how all the pieces of Next.js/React fit together, including client components, server components, static and dynamic routing, server actions, middleware, and layouts.
This course will help you understand the client-side and server-side aspects of Next.js, making you a more well-rounded developer.
You'll store data in a serverless Prisma DB on PlanetScale, which is a scalable and secure way to manage your app's data.
The course will guide you through integrating the OpenAI API and Langchain, allowing you to incorporate AI capabilities into your app.
By the end of the course, you'll have a fully functional AI-powered Journal app that you can deploy to the world.
Related reading: Next Js Courses
Introduction
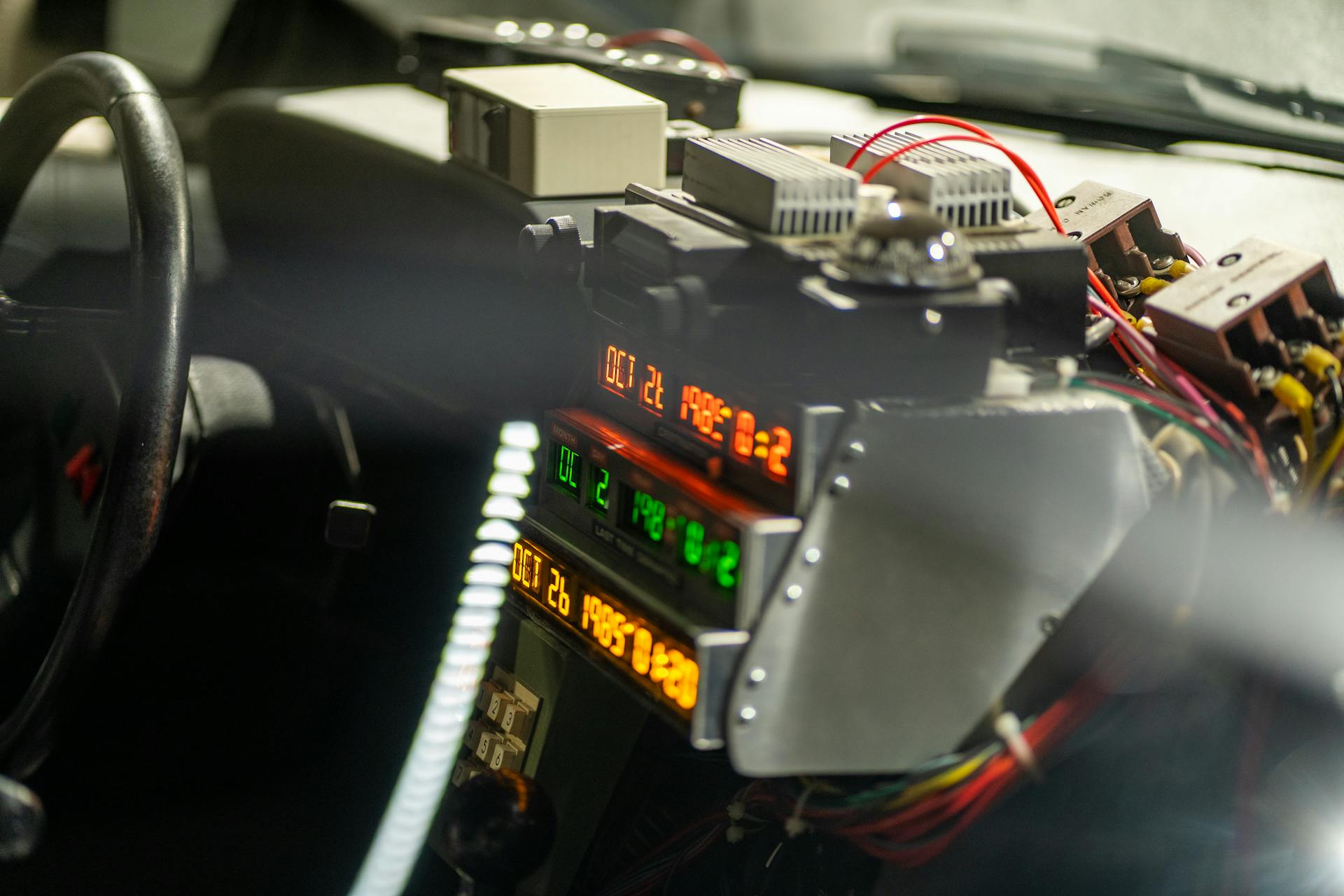
The course starts with a brief introduction where Scott Moss explains the project and demonstrates an AI-powered application built with Next.js.
The application will be built from scratch and allows users to log in and manage journal entries with standard CRUD operations.
The application also analyzes entries and generates AI-driven sentiment values, summaries, and colors.
You can find the final version of the application in the GitHub repo linked below.
Take a look at this: Why Is Artificial Intelligence Important
App Setup
To set up a Next.js app, start by using the `create-next-app` CLI tool, which will scaffold a new application. Next.js version 13.4.5 is recommended for this course.
The default application code is removed and a Prettier config is added, which will help keep your code organized and consistent. I've found that using a Prettier config can save a lot of time in the long run.
To get started, remove the default application code and add a Prettier config to your project. This will give you a solid foundation to build on.
Additional reading: Next Js Prettier
App Setup & Auth
Setting up your app's authentication is a crucial step in ensuring that only authorized users can access your application's features. This process involves integrating authentication tools like Clerk and Descope into your Next.js application.
Clerk is a powerful authentication provider that allows you to configure multiple auth providers. To get started, you'll need to install the Clerk library and add SignIn and SignUp components to their corresponding pages. This will enable users to sign in and sign up for your application.
To protect your routes and ensure only authenticated users can access certain features, you'll need to create a middleware function that checks for authentication. This involves configuring sign-in and sign-up redirection and testing the authentication flow.
Here's a breakdown of the steps involved in setting up app authentication:
- Install the Clerk library
- Add SignIn and SignUp components to their corresponding pages
- Create a middleware function to protect routes
- Configure sign-in and sign-up redirection
- Test the authentication flow
By following these steps, you can ensure that your application's authentication is secure and reliable.
Next.js and Vercel
Next.js is a great framework for building React apps because it's very easy to get started with and comes with a lot of features out of the box.
Next.js supports server-side rendering, which makes it super fast.
Next.js scales seamlessly from 1 user to 100s of 1000s of users.
Vercel's preview deployments are super useful for testing and reviewing changes before they go live.
Pages and Routing
In Next.js, pages are React components that get rendered on the server or on the client, and they're usually stored in the `pages` directory. This directory is automatically scanned for routes.
Each page in Next.js has a unique file name, which corresponds to a route in the application. For example, if you have a file named `[id].js` in the `pages` directory, it will match any route that ends with a number, like `/users/123`.
The `getStaticProps` method can be used to pre-render pages at build time, which is useful for pages that don't change often. This method returns a JSON object that gets passed to the page as props.
If this caught your attention, see: Nextjs Pages
Testing and Deployment
Testing and deployment are crucial steps in building a Next.js AI application.
Scott introduces Vitest and installs the testing dependencies.
Configuration files are added to the project, and a setupTests.ts file is created to import the jest-dom testing library. This file is essential for setting up the testing environment.
You might enjoy: Nextjs Server Actions File Upload
To avoid testing the functionality of third-party dependencies like Clerk, mocks are created for the library's methods. This approach ensures that the tests focus on the application's logic rather than external libraries.
Scott creates a test for the home page, which is a critical part of the application.
Here's a breakdown of the testing process:
- Test creation: Scott creates a test for the home page.
- Mocking third-party dependencies: Mocks are created for the library's methods to avoid testing external functionality.
Deploying the app to Vercel is the next step in the process. Scott pushes the project up to a GitHub repo and connects it to the Vercel deployment.
On a similar theme: Nextjs by Vercel Meaning
Tech Stack and Tools
Next.js is the framework used to build the platform, specifically leveraging the App Router for its architecture. We'll be exploring each piece in more depth.
The tech stack is comprised of several key technologies, including TypeScript, Tailwind CSS for styling, and shadcn/ui for component library.
Here's a breakdown of the main technologies used:
- Next.js App Router
- TypeScript
- Tailwind CSS
- shadcn/ui
- OpenAI's Assistants API with Streaming and File Search
- Descope for user authentication
- Vercel's AI SDK for chat UI
The platform's landing page is built with NextJS, Tailwind CSS, TypeScript, and shadcn/ui components.
Frequently Asked Questions
Which bundler does Next.js use?
Next.js uses Turbopack, an incremental bundler optimized for JavaScript and TypeScript. Built into Next.js for seamless performance and development.
What is Vercel AI?
Vercel AI is a collection of AI-powered tools and services, including the AI SDK, designed to help developers build intelligent applications. Learn how to integrate AI into your projects with our comprehensive toolkit.
What language does Next.js use?
Next.js is built on top of JavaScript, utilizing its capabilities to enhance web development. It leverages React, a popular JavaScript library, to provide additional features for building web applications.
How to use AI in NextJS?
To use AI in NextJS, you'll need to train an AI model using a suitable library like TensorFlow.js and convert it into a format loadable in JavaScript. This allows you to integrate AI capabilities into your NextJS application.
What is next JS best for?
Next.js is ideal for building fast, interactive, and dynamic web applications, such as Single Page Applications and Progressive Web Apps. Its optimized performance and React Server Components make it perfect for complex interfaces.
Featured Images: pexels.com