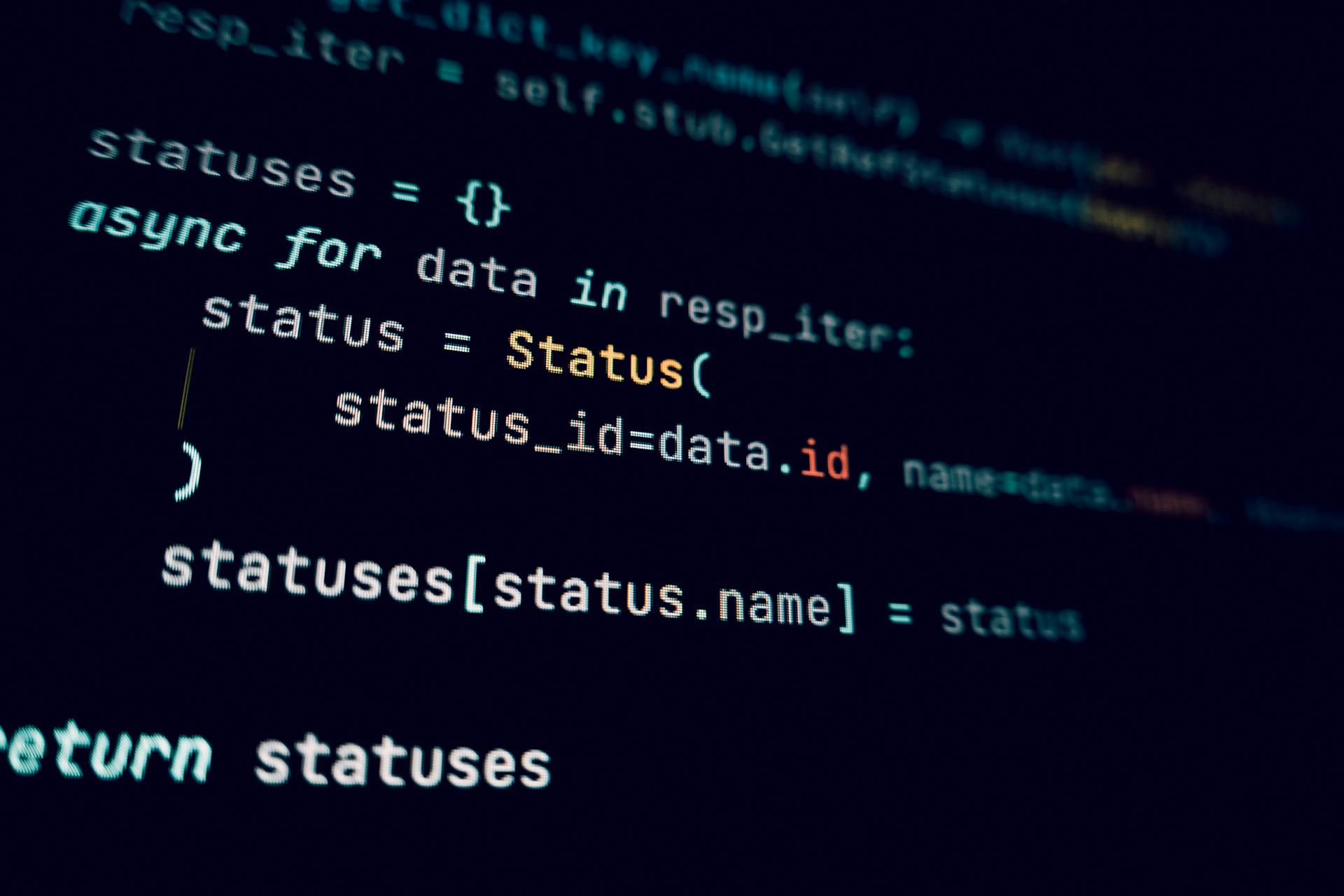
The .env.local file in Next.js is a crucial part of your project's configuration, storing sensitive information such as API keys and database credentials.
In the Next.js project structure, the .env.local file is located in the root directory, alongside the src folder. This file is not committed to version control, ensuring your sensitive data remains secure.
To access environment variables in your Next.js application, you can use the process.env object, which is a built-in Node.js object that allows you to access environment variables.
For example, if you have a variable named API_KEY set in your .env.local file, you can access it in your code using process.env.API_KEY.
Getting Started
To get started with using environment variables in Next.js, you'll need to add them to an env.local file in your project root directory. This file loads every variable into the Node.js process.
Environment variables in Next.js are only available for use on the server, so you can only use them in data fetching methods like getServerSideProps, getStaticProps, and getStaticPaths, or API routes.
You should always put the env.local file in your project root directory to avoid Next.js running into errors.
How to Use
To get started with using environment variables in Next.js, you'll need to create an env.local file in your project root directory. This is where you'll add your environment variables.
The env.local file will load every variable into the Node.js process, making them available for use on the server.
You can reference other variables in the same env.local file using a dollar sign. For example, process.env.PROTECTED_URL will evaluate to the value specified.
Next.js will automatically load the variables as process.env.API_URL and process.env.API_KEY, which you can then use in your code.
Remember to always put the env.local file in your project root directory to avoid Next.js running into errors.
Create a Project
To get started with Next.js, you'll first need to create a project. Run the command `npx create-next-app my-app` from your terminal to create a new Next.js application.
This will set up a basic Next.js project for you to work with. Now, go to the project's root folder and create a new file named `.env.local`. This is where you'll store your environment variables.
This file will serve as your first environment file, and you'll create others as you go forward.
Browser Integration
Browser integration is a crucial aspect of Next.js, and understanding how to expose environment variables to the browser is essential for a seamless user experience. You can make environment variables available in the browser by prefixing them with NEXT_PUBLIC_.
To expose a variable to the browser, you can simply prefix it with NEXT_PUBLIC_, like NEXT_PUBLIC_GOOGLE_ANALYTICS_ID. This allows you to use the variable in client-side code, such as in the _app.js file, which runs on both the client and server.
To add JavaScript code to configure Google Analytics, you can use the Script component from Next.js, which optimizes external scripts and improves browser performance. By using the lazyOnload strategy, you can tell the browser to generate the analytics when all other resources have been fetched and the browser is idle.
Here's a summary of the order in which Next.js looks for environment variables:
- process.env
- env.${NODE_ENV}.local
- env.local (env.local is not checked when NODE_ENV is test)
- env.${NODE_ENV}
- .env
Browser Integration
Browser Integration is a crucial aspect of Next.js development, allowing you to expose environment variables to the browser.
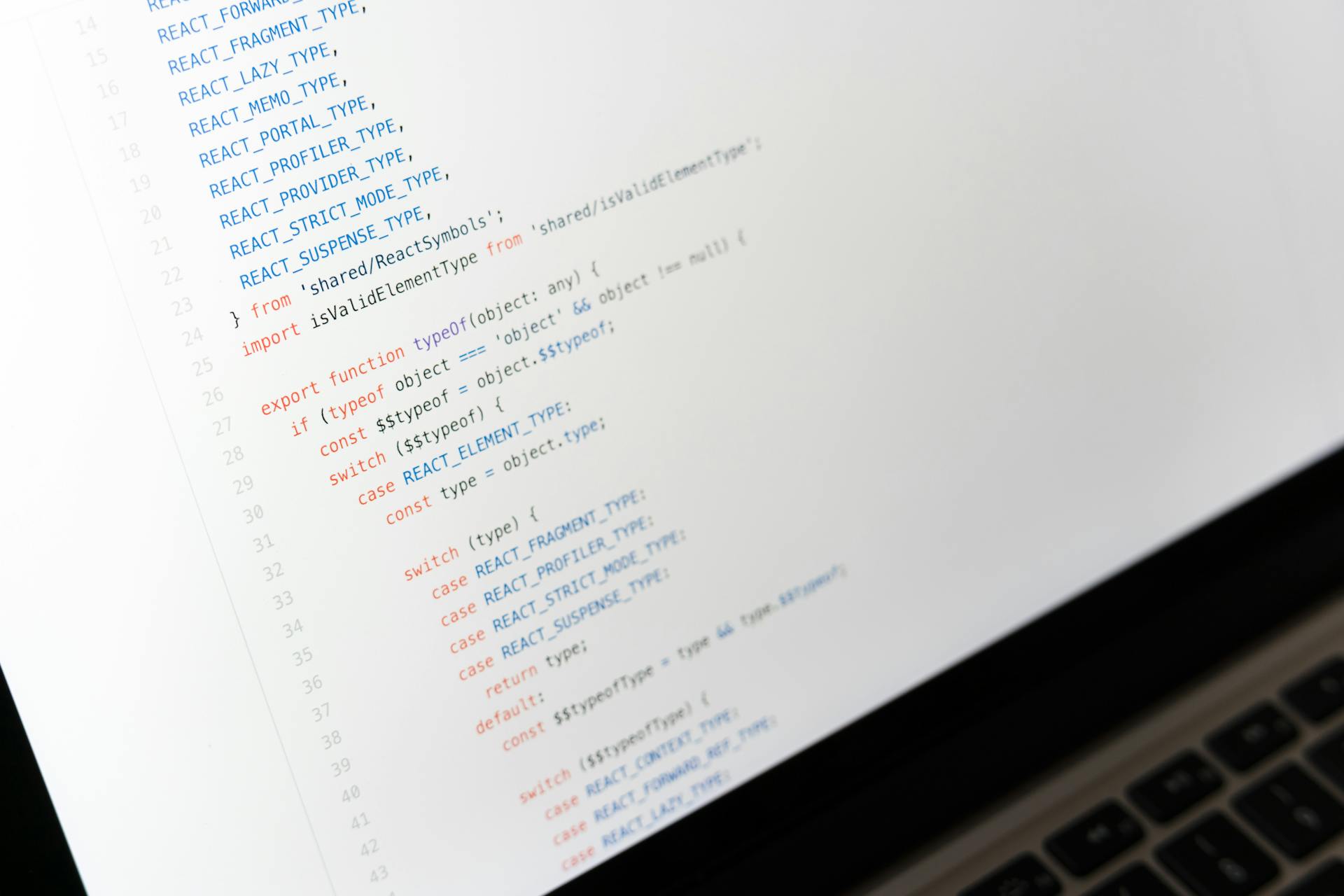
To make environment variables accessible in the browser, you need to prefix them with NEXT_PUBLIC_. This will automatically make them available for use in client-side code.
You can use this feature to add Google Analytics to your Next.js application. For example, you can define a NEXT_PUBLIC_GOOGLE_ANALYTICS_ID variable in env.local, and then use it in your code like process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID.
The NEXT_PUBLIC_ prefix is only necessary for variables you want to expose to the browser. If you have a variable that should only be accessible from the server, you can define it without the prefix.
Here's a summary of the order in which Next.js looks for environment variables:
- process.env
- env.${NODE_ENV}.local
- env.local (env.local is not checked when NODE_ENV is test)
- env.${NODE_ENV}
- .env
Keep in mind that if you declare the same environment variable in multiple files, the one in the lowest file in the list will win.
Web Development Importance
Web development importance is a crucial aspect of browser integration. Environment variables play a vital role in web development by allowing developers to configure their applications for different environments.
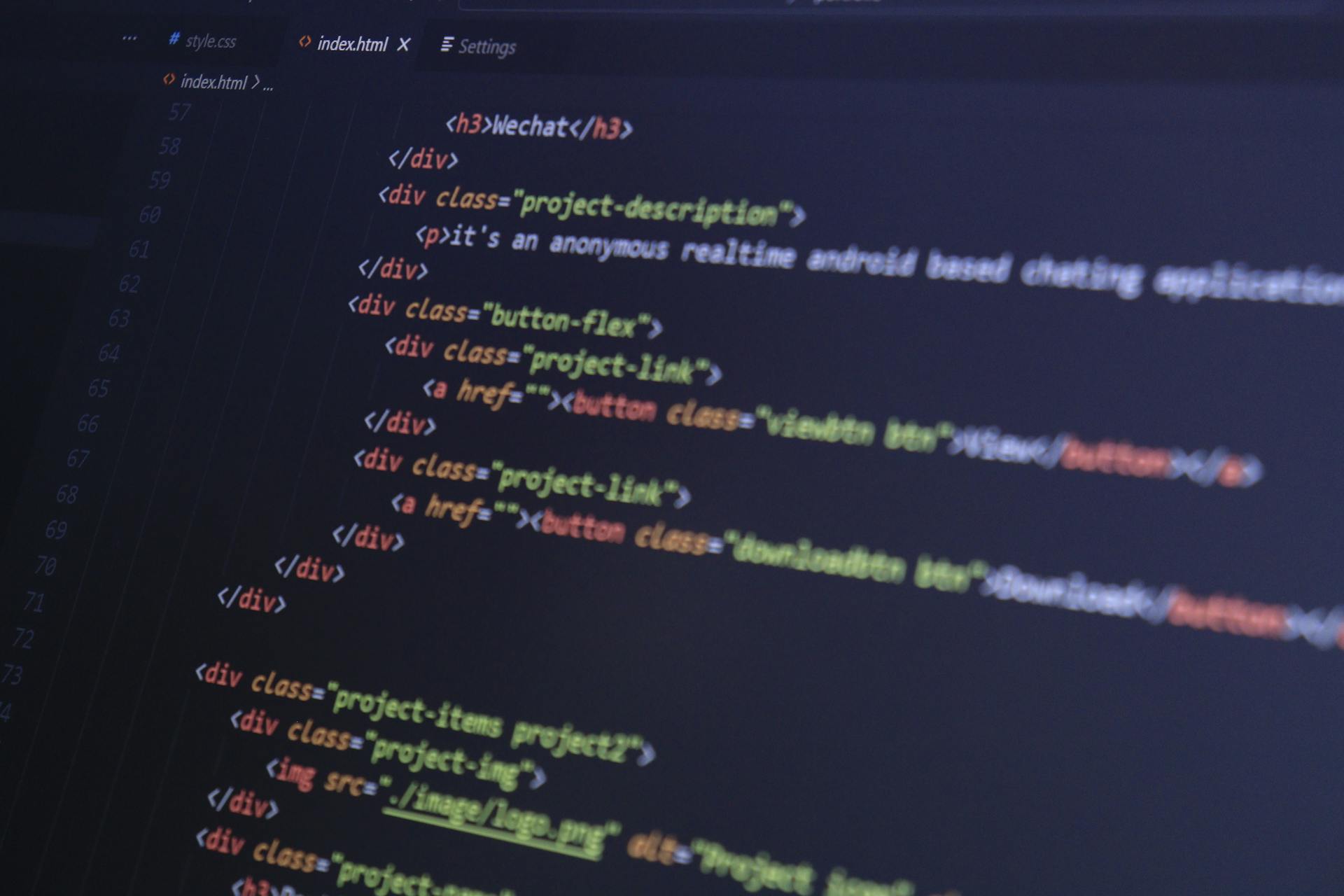
This approach improves the security and flexibility of Next.js applications. By separating configuration settings from the application code, developers can manage settings such as API keys, database URLs, and other environment-specific details more effectively.
Environment variables make adapting an application to different environments easy without modifying the codebase. This is a significant advantage, especially when working with Next.js serverless applications.
Here are some key benefits of using environment variables in web applications:
- Enhanced Security: By storing sensitive information in environment variables, developers can prevent exposing critical data in their source code repositories.
- Flexibility: Environment variables make adapting an application to different environments easy without modifying the codebase.
- Maintainability: Separating configuration details from the codebase simplifies maintenance for Next.js serverless applications.
Understanding
Environment variables are external values defined outside of the application code, used to influence the behavior of an application. They often store sensitive data like API keys.
You can use environment variables to store different configuration settings for various environments, such as development, testing, and production. This allows you to keep the codebase short, clean, and organized.
In a development environment, tools for code formatting, linting, and testing are essential, but not necessary in production. The value of the NODE_ENV environment variable can be set to production to install dependencies required for production.
Modifying an environment variable usually doesn't require rebuilding or redeploying the application, but in some frameworks like Next.js, some environment variables are hardcoded in the codebase at build time, so you may need to build and redeploy after modifying an environment variable.
There are two types of environment variables: private and public. Private variables, like SOME_SECRET, are only accessible from the server environment, while public variables, like NEXT_PUBLIC_BASE_URL, are exposed to both the browser and Node.js environments.
Built-in Support and Features
Next.js has built-in support for environment variables, which can be declared in the .env.local file at the root of your project directory. This file loads environment variables into the process.env object in the Node.js environment.
You can store constants and default values in .env, env.development, and env.production environment files, which are not meant for storing secrets but for environment-specific configurations and settings. These files are useful for separating configuration details from the codebase.
Here are the different types of environment files and their purposes:
By using environment variables, you can enhance security, flexibility, and maintainability in your Next.js application.
Understanding Variable Types
In Next.js, environment variables are divided into two types. Some variables are exposed to the browser, while others are not.
To store a secret that's not needed in the front-end, use a variable without the NEXT_PUBLIC_ prefix. This will make it inaccessible from the browser.
Variables without the NEXT_PUBLIC_ prefix can be accessed in getStaticProps, but will return undefined if tried to be accessed inside the browser.
To expose a variable to the browser, prefix it with NEXT_PUBLIC_. This allows you to access the variable inside your component.
Variables prefixed with NEXT_PUBLIC_ can be accessed like any other variable inside your component, and will print the value properly.
Built-in Support
Next.js has built-in support for environment variables, making it easy to configure your application for different environments.
To start using environment variables, you can declare a .env.local file at the root of your project directory. Next.js will load the environment variables into the process.env object in the Node.js environment.
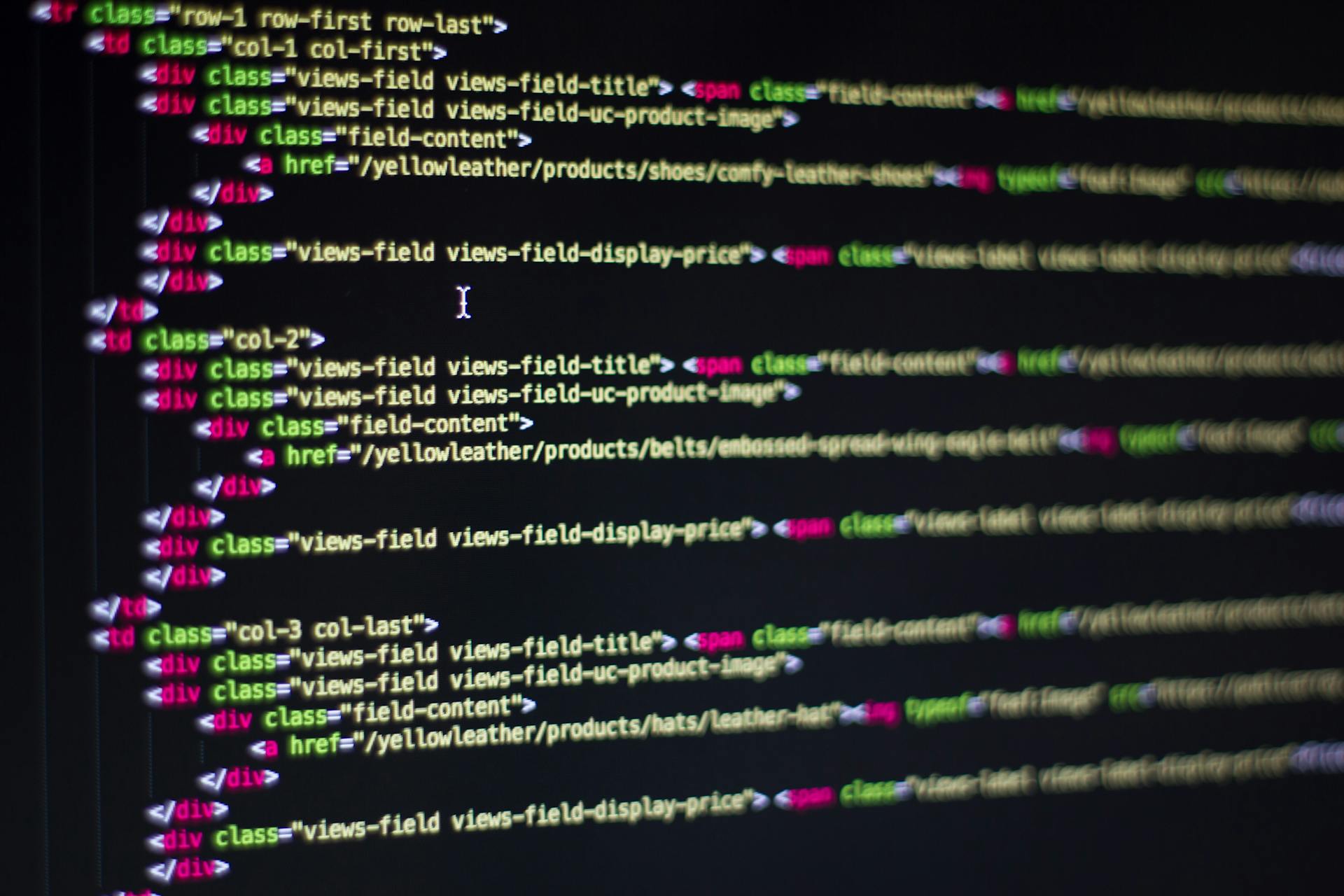
Public environment variables are available in both the browser and Node.js environments, while private environment variables are only accessible in the Node.js environment. To make a private environment variable public, prefix it with NEXT_PUBLIC_.
Here's a breakdown of how to use environment variables in Next.js:
You can store constants and default values in .env, env.development, and env.production environment files, but not secrets. These files are meant for environment-specific configurations and settings.
Next.js automatically loads environment variables from the .env.local file into the Node.js process, making them available for use in data fetching methods or API routes. You can also reference other variables in the same env.local file using a dollar sign ($).
Security and Best Practices
Security is a top priority when working with sensitive information in your Next.js project. Avoid hardcoding values directly into your code, as this can lead to security breaches.
Always use environment variables to store sensitive information, such as API keys. This ensures that your code remains secure, even if your repository is compromised.
Forgetting to update variables can also lead to security issues. Ensure that when you update sensitive information like API keys, you also update the corresponding environment variables.
Public and Private
Public environment variables in Next.js are mostly used for storing constants or default values that you don’t want to import or declare in multiple files.
You can declare public environment variables in a .env* file by prefixing their name with NEXT_PUBLIC_.
Public environment variables are available both in the browser and in Node.js, making them a convenient option for storing common settings.
To make an environment variable public, you simply need to prefix its name with NEXT_PUBLIC_.
At build time, Next.js will replace references to public environment variables with their actual values in the codebase bundled for the browser environment.
This means that process.env.NEXT_PUBLIC_ENV_VARIABLE will be replaced with the actual value of the environment variable at build time.
Public environment variables are private by default, so you need to explicitly make them public by prefixing their name with NEXT_PUBLIC_.
Common Pitfalls and How to Avoid Them
Hardcoding sensitive information directly into code is a major security risk. Always use environment variables instead.
Forgetting to update variables is a common mistake that can put your application at risk. Make sure to update corresponding environment variables when updating sensitive information like API keys.
Here are some common pitfalls to watch out for:
Sources
- https://create-react-app.dev/docs/adding-custom-environment-variables/
- https://refine.dev/blog/next-js-environment-variables/
- https://blog.logrocket.com/customizing-environment-variables-next-js-13/
- https://www.saffrontech.net/blog/understanding-next-js-environment-variables
- https://www.mdfaisal.com/blog/how-to-handle-different-environments-in-a-nextjs-application
Featured Images: pexels.com