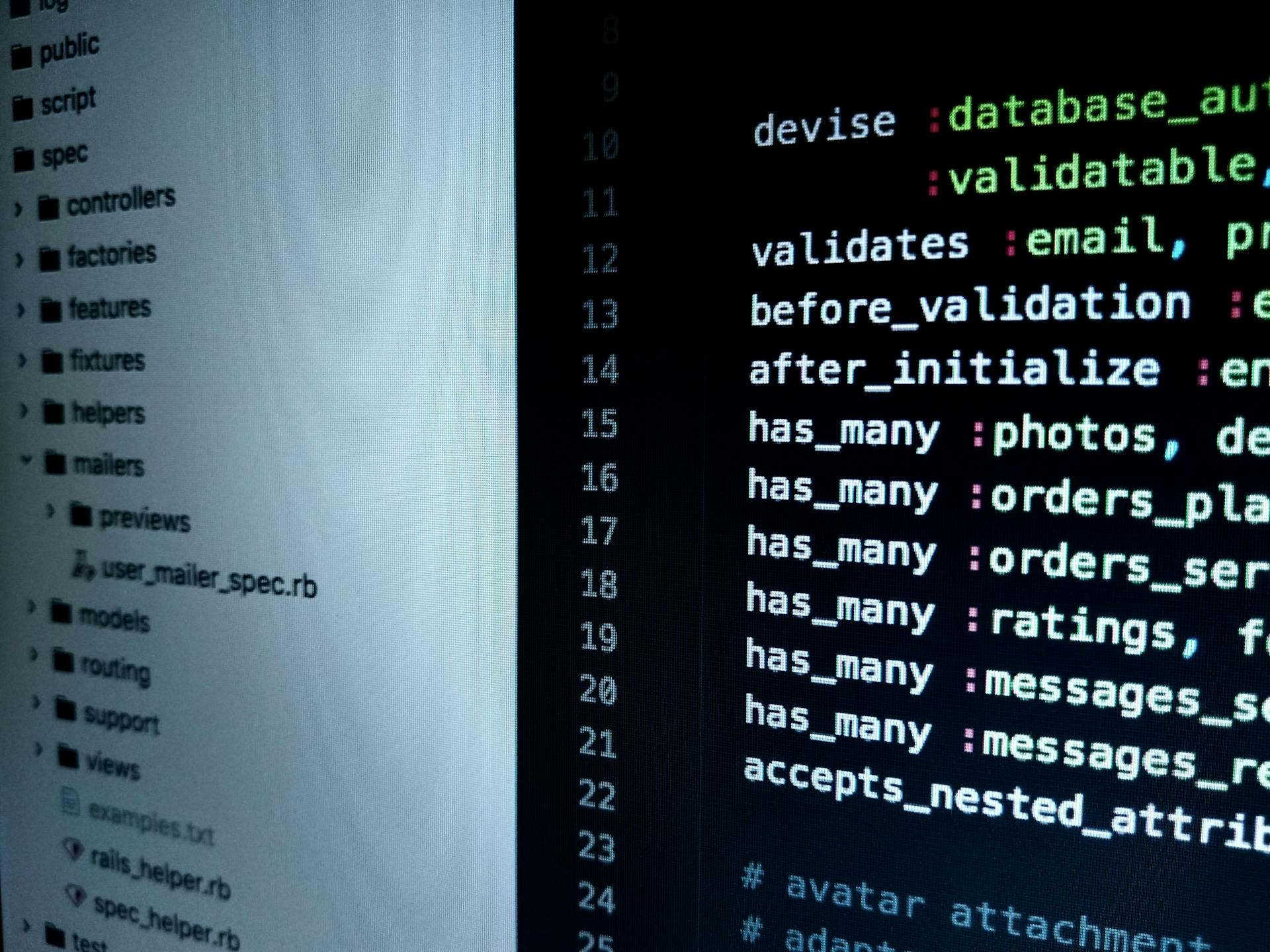
Implementing rate limiting in Next.js is crucial to prevent abuse and ensure a smooth user experience.
To achieve this, you can use middleware to limit the number of requests from a specific IP address or user agent.
Middleware can also be used to implement algorithms like the Leaky Bucket algorithm, which allows a certain number of requests within a time window before blocking further requests.
For instance, you can use the Leaky Bucket algorithm to allow 100 requests per minute from a specific IP address before blocking further requests.
Next.js Middleware
Next.js Middleware is a powerful tool that allows developers to customize the handling of each request on the server. This functionality enables developers to enhance application performance and security.
Middleware in Next.js refers to functions that execute during the server-side rendering phase, dealing with the incoming requests before they reach your React components. This placement allows middleware to act on the request, modifying headers, query parameters, the request path, and other aspects of the request or to decide on the control flow.
To integrate middleware into a Next.js project, you would typically modify or create a middleware.js file in the root of the project. A simple example of how a middleware might look is by intercepting every API request and allowing the request to be rejected or redirected.
Middleware in Next.js operates at an essential layer within the framework's architecture, configured to run after the HTTP server has parsed the incoming request but before it reaches the React application logic. This unique capability means developers can use middleware to handle concerns relevant both to UI rendering and backend API services within the same framework seamlessly.
Next.js middleware can preprocess requests heading to API routes to ensure they’re properly authenticated or to log API usage metrics. This adaptability is crucial for developing high-performance, secure, and scalable web applications.
Rate Limiting Algorithm
The sliding window algorithm is a great choice for rate limiting, especially when dealing with a surge in requests. It's unaffected by such surges, making it a reliable option.
One of the main advantages of this algorithm is that it allows for easy limiting of each IP address to a certain number of requests per time period, such as 5 requests per 24 hours. However, this comes at the cost of writing a record to the database for each request.
Using the sliding window algorithm, you can also trigger the calculation of how many requests occurred in the previous 24 hours with each request. This can add an extra layer of complexity to your code, but it's a necessary trade-off for the benefits it provides.
Sliding Window Algorithm
The sliding window algorithm is a great choice for rate limiting because it's easy to implement and limits each IP address to 5 requests per 24 hour period.
This algorithm is particularly useful because it's unaffected by a surge in requests, which can be a major advantage in high-traffic situations.
One of the main advantages of the sliding window algorithm is that it's easy to implement, allowing you to limit each IP address to a specific number of requests within a time frame.
However, one of the disadvantages is that a record must be written to the database for each request, which can impact performance.
This algorithm requires a record to be written to the database for each request, which can be a drawback.
Each request will trigger the calculation of how many requests occurred in the previous 24 hours, which can be computationally expensive.
Overall, the sliding window algorithm is a good choice for rate limiting because it's flexible and can handle high traffic, but it does require some additional overhead.
The sliding window algorithm uses a record for each request, which can be a drawback in terms of database performance.
Selecting the Algorithm
The token bucket algorithm is a popular choice for rate limiting, and it's what we'll be using in this example. It works by assigning each client a "token" that expires after a certain amount of time.
This approach can be adjusted to handle traffic originating from specific geographical regions. By assigning tokens to clients based on their geographic location, you can restrict the number of requests coming from a particular country or region.
Assigning fewer tokens to clients in a specific location can help to evenly distribute the load on your server. This ensures that all clients can access your application from different places.
The token bucket algorithm has its advantages and drawbacks, which we'll discuss later.
Database and Storage
Next.js provides a built-in API Routes feature that allows you to create server-side routes for your application, which can be used to store and manage data.
You can use a database like MongoDB or PostgreSQL to store data, and Next.js provides support for these databases through its API Routes feature.
For example, you can use a database to store user data, such as their username, email, and password.
Next.js also provides a built-in storage system called `next/image` that allows you to store and manage images in your application.
This feature is especially useful for storing large amounts of data, such as images and videos.
By using a database or storage system, you can ensure that your application can handle a large number of requests and scale accordingly.
For instance, if you're building an e-commerce application, you can use a database to store product information and customer data.
Next.js also provides support for caching, which can help improve the performance of your application by reducing the number of requests made to the database or storage system.
Implementation and Setup
To implement rate limiting in a Next.js project, you need to set up the rate limiter. This is done by creating a new file called 'rate-limiter.js' in the '/lib' directory.
You'll use the '@upstash/ratelimit' package, which provides a simple interface for implementing rate limiting using Redis. The package is imported along with the Redis instance created earlier.
A new rate limiter instance is created using a sliding window algorithm with a limit of 1 request per 40 seconds. This is just for testing purposes and should be adjusted according to your project's needs.
To implement the rate limiter to the API, you'll create a new file in the API folder, such as 'pages/api/redis-limit.ts'. This file will contain the Redis client connection with the rate limit config.
The rate limiter is used to handle API requests by importing the 'rateLimiter' instance from './lib/rate-limiter.js'. This instance is then used to limit the number of requests.
By following these steps, you can easily add a rate limiter to your Next.js API and protect it from overload. The 'rate-limiter.js' file is a crucial part of this process, as it sets up the rate limiter instance.
Middleware in Next.js
Middleware in Next.js is a powerful tool that allows developers to customize the handling of each request on the server. This functionality enables developers to enhance application performance and security.
Middleware in Next.js refers to functions that execute during the server-side rendering phase, dealing with the incoming requests before they reach your React components. This placement allows middleware to act on the request, modifying headers, query parameters, the request path, and other aspects of the request or to decide on the control flow.
To integrate middleware into a Next.js project, you would typically modify or create a middleware.js file in the root of the project. This file can contain a simple example of how a middleware might look.
Middleware in Next.js operates at an essential layer within the framework's architecture, configured to run after the HTTP server has parsed the incoming request but before it reaches the React application logic. This unique capability means developers can use middleware to handle concerns relevant both to UI rendering and backend API services within the same framework seamlessly.
Ultimately, Next.js middleware offers a robust solution to extend the functionality of web applications dynamically and conditionally based on the request’s characteristics. This adaptability is crucial for developing high-performance, secure, and scalable web applications.
Sources
- https://www.lucasamos.dev/articles/ratelimit
- https://wyattweb.dev/blog/posts/how-to-add-a-rate-limiter-for-your-api-using-next-js-13
- https://loadforge.com/guides/nextjs-middleware-key-to-faster-web-applications
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
- https://blog.logrocket.com/set-up-rate-limiting-next-js-redis/
Featured Images: pexels.com