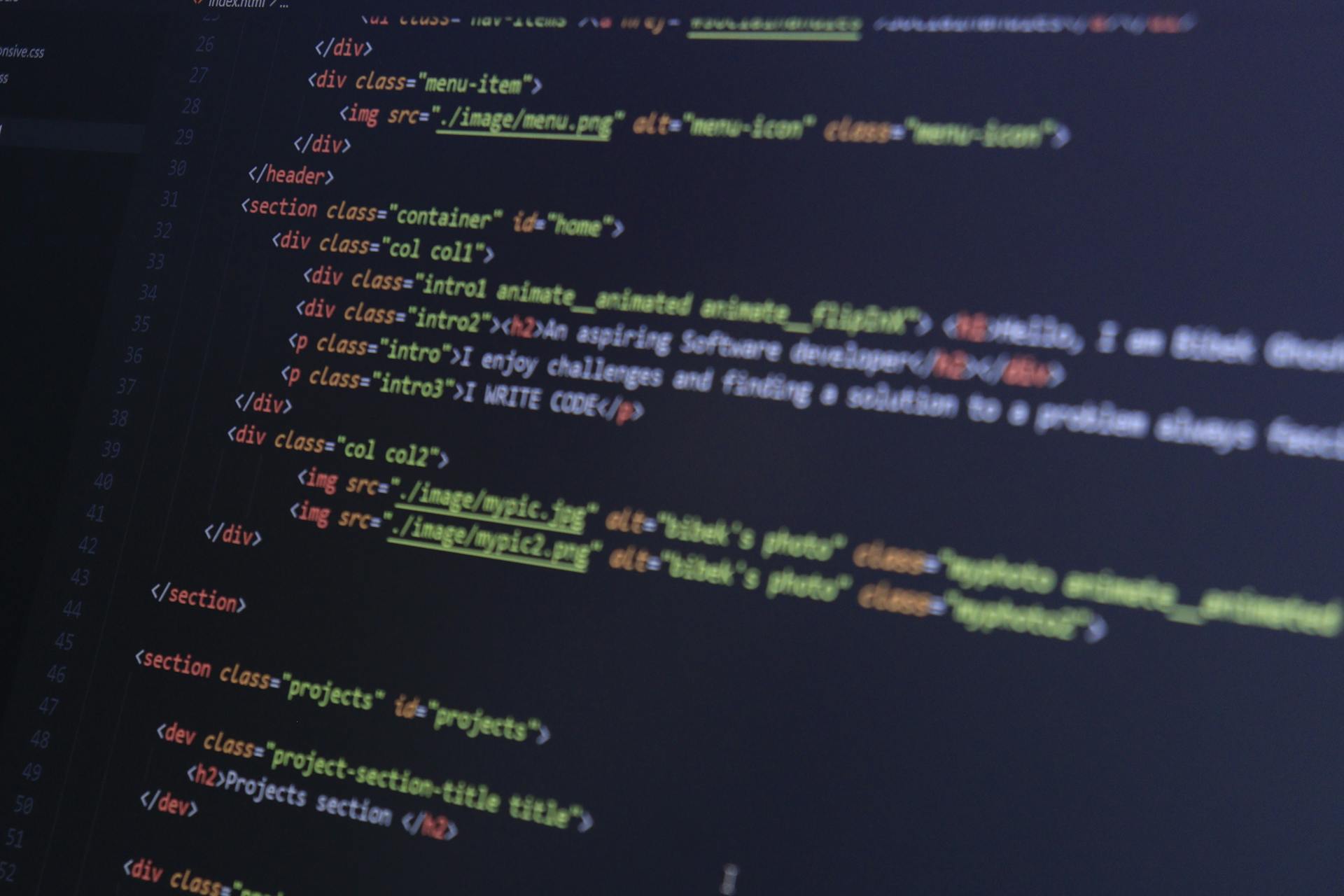
React DevTools is a game-changer for any React developer, allowing you to inspect and debug your components with ease. It's a must-have tool for anyone working with React.
The React DevTools Panel is where the magic happens, providing a comprehensive view of your component tree. You can navigate through the component hierarchy, inspect props, state, and context, and even see the component's lifecycle methods.
By using the React DevTools Panel, you can quickly identify issues in your components and make necessary adjustments.
Installation
You can install React DevTools as a development tool globally with a simple command. This is often the most convenient way to get started.
To install it globally, run `npm install -g react-devtools@^4` in your terminal. This command will install the necessary tools for you to use React DevTools.
Alternatively, you can install React DevTools as a project dependency using Yarn or NPM. With Yarn, you can run `yarn add react-devtools` to add it to your project.
If you prefer not to install it globally, you can use NPX with NPM by running `npx react-devtools`.
If you're using a browser other than Chrome or Firefox, you can install the DevTools as a standalone app using the `npm install -g react-devtools@^4` command.
Intriguing read: Can I Use React Query with Nextjs
Debugging and Troubleshooting
To confidently debug your React application, you'll need to use the standalone version of React DevTools, which can be used to debug a React application running in Safari.
Even experienced developers can face challenges with React DevTools, making troubleshooting an essential skill. Knowing how to troubleshoot these issues can save you a lot of time and frustration.
Connection issues can often be resolved by ensuring that the React app is running in development mode and that the React DevTools core is properly integrated. Simply re-enabling the extension or restarting the browser can sometimes fix the connection problems.
Debugging in Chrome
Debugging in Chrome is a breeze with the right tools. The React Developer Tools extension is a must-have for inspecting component props, state, hooks, setting breakpoints, and viewing stack traces for errors.
You can open Chrome devtools on your React app and select the Components tab in the React Developer Tools to inspect React elements by hovering over components in the tree. This is especially helpful when you need to see what's going on with a specific component.
Even the most experienced developers can face challenges with React DevTools, but knowing how to troubleshoot these issues is an essential skill. Connection issues can often be resolved by ensuring that the React app is running in development mode and that the React DevTools core is properly integrated.
Sometimes, simply re-enabling the extension or restarting the browser can fix the connection problems. If you're stuck, don't forget to leverage the React community for assistance – forums, GitHub, and Stack Overflow are all great resources for seeking help with issues like timeout errors or compatibility concerns.
Inspecting and Understanding
You can use React DevTools to inspect the props and state of each component in the application tree. This helps you identify rendering issues and data flow problems by providing a visual representation of the components.
The application tree can be viewed in the React DevTools by clicking the Components tab. It displays the root React components and their subcomponents, allowing you to navigate through the component tree.
To inspect a component, select it in the Components tab and view its props and state in the right pane. You can also click on a component to view its props and state in the console.
Here are some key features of React DevTools that help with inspection and understanding:
- Verifying a component’s props and state
- Addressing performance issues
- Inspecting the context values
These features make it easier to identify and fix bugs, optimize performance, and understand the behavior of your React applications.
Components Tab
The Components tab in React DevTools is a powerful tool for inspecting and understanding your React application. It provides a visual representation of the component hierarchy, allowing you to quickly identify rendering issues and data flow.
You can access the Components tab by opening React DevTools and selecting it from the tabs. This will display the application tree, showing the components in your application and their relationships.
The application tree can help you identify issues with component rendering, data flow, and other problems. You can also use the tree to inspect the props and state of each component.
To inspect the props and state of a component, click on it in the tree. This will display the props and state in the right pane. You can also use the Components tab to inspect the child components and parents of a selected component.
Here are some key features of the Components tab:
- Component Hierarchy: The application tree shows the components in your application and their relationships.
- Props and State: You can inspect the props and state of each component by clicking on it in the tree.
- Child Components: You can inspect the child components of a selected component.
- Parent Components: You can inspect the parent components of a selected component.
By using the Components tab, you can gain a better understanding of your React application and identify issues more quickly.
Differences and Tooling
React DevTools works similarly for both React DOM and React Native, but there are differences in the tooling due to the platforms' distinct environments and rendering engines.
React DOM and React Native have different tooling because of their unique environments and rendering engines.
The React DevTools interface is adapted to each platform's specific needs, making it easier to inspect and understand the differences between React DOM and React Native.
This means developers need to be aware of the specific tooling differences when working with React DOM versus React Native.
For another approach, see: Tailwind Css React Native
Performance and Optimization
The React Profiler Tab is a powerful feature for analyzing the performance of React applications, helping developers identify and address performance bottlenecks.
By recording performance information, developers can analyze the rendering time of components and determine which ones are causing slowdowns.
To record performance information, navigate to the Profiler tab in React DevTools and start a profiling session. The tool will track the re-render cycles and provide a visual representation of the performance data.
The Profiler tab allows you to collect and evaluate data about your application's performance. With this feature, you can analyze each UI element, viewing changes in the component's React tree over time.
Follow these steps to use the Profiler:
- Open a website that's built with the profiling build of ReactDOM.
- Start recording a session by clicking the red circle.
- Perform some operations in the application.
- Finally, press the circle again to end the session.
Enabling a highlight when a component is re-rendered will offer you a visual representation of how the components react to data changes. This is a handy method to understand how components interact.
Advanced Features and Tools
The React Developer Tools offer advanced features that can supercharge your development workflow.
The Components tab allows you to view the component tree structure, which is a game-changer for debugging complex React applications.
You can also use the Profiler tab to identify slow components, which is essential for optimizing performance.
The Profiler tab's Flame chart shows relative render times, giving you a clear picture of where your app's performance is bottlenecked.
Here's a comparison of the key features of the Components and Profiler tabs:
With these advanced features, you can optimize your React development workflow and create faster, more efficient apps.
Best Practices and Workflow
To maximize the benefits of React Developer Tools, developers should adopt best practices that streamline their debugging and inspection processes. Key features like inspecting state changes, component re-renders, mocking props, catching errors, and integrating with Redux DevTools can help debug React apps faster.
Inspecting state changes is a crucial feature of React Developer Tools. This allows developers to understand how state changes affect the application's behavior.
Component inspection is invaluable for understanding the page's React tree. The UI element inspection in the Components tab enables developers to filter components, edit state and props, and manage performance bottlenecks.
Debugging with purpose-built tools like React Devtools is a crucial discipline in web development today. Debugging can no longer only rely on console logging and breakpoints.
Tight integration with frameworks gives developers precise control over app execution and infrastructure. Powerful debugging improves developers' ability to write high-quality code efficiently.
Redux and Additional Extensions
React DevTools can be extended with additional extensions to provide a more comprehensive debugging experience. One such extension is Redux DevTools, which offers state management debugging features for apps using Redux.
Integrating Redux DevTools with React DevTools can be a game-changer for developers, allowing them to trace state changes alongside component updates. This integration provides a more detailed understanding of how the application state is being managed.
Other browser extensions can also be used in conjunction with React DevTools to enhance debugging capabilities. For example, extensions for network monitoring or performance analysis can be particularly useful for identifying and resolving issues.
For another approach, see: Redux Devtools Extension
Native Apps
React DevTools is a powerful tool for debugging React Native apps. It can connect to React Native apps, allowing developers to inspect and debug components in a similar fashion to web applications.
The standalone version of React DevTools is particularly useful for debugging React Native applications because it is platform-independent and can connect to apps running on iOS or Android devices. This makes it a versatile option for developers who need to debug their React Native apps in different contexts.
React DevTools can be used to determine why a specific component of your React Native app isn’t rendering correctly on an iOS device. You can use it to inspect the component tree, check component props and state, and identify issues with styling or logic that may be causing the rendering problem.
React Native developers can also benefit from React DevTools, as it supports debugging for mobile applications built with React Native.
Check this out: React Native Devtools
Understanding and Learning
React Devtools are an invaluable asset for React developers, making it easier to inspect and understand component-based UIs. With over 4 million installations, it's clear that many developers have found them to be a game-changer.
The React DevTools browser extension is like an x-ray vision into your React app's component structure, state, props, and other key internals. You can install it as a browser extension for Chrome or Firefox, although it's not currently available for Safari.
To get the most out of React Devtools, you can take advantage of its features, including verifying a component's props and state, addressing performance issues, and inspecting context values. The Components tab is a great place to start, where you can view the app's hierarchy tree and see how components are structured.
Some of the key features of React Devtools include:
- Verifying a component's props and state
- Addressing performance issues
- Inspecting context values
Understanding
React DevTools is a game-changer for React developers, with over 4 million installations and a wide range of features to make your workflow more efficient.
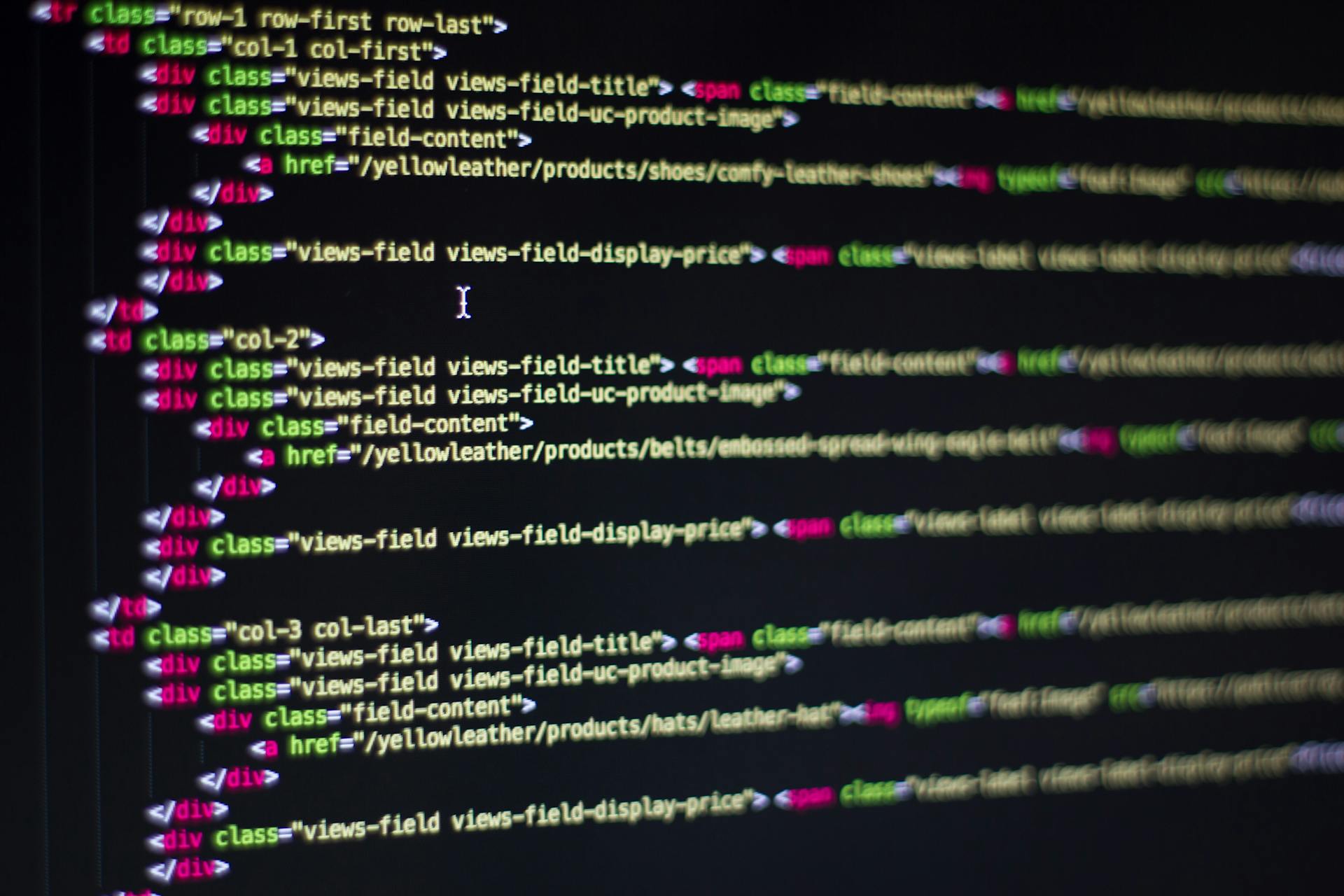
The tool is available as a browser extension for Chrome and Firefox, and can be used to debug and inspect your React applications in real-time. It's like having an x-ray vision into your app's component structure, state, props, and other key internals.
React DevTools allows you to verify a component's props and state, address performance issues, and inspect the context values used in nested components.
Some key features of React DevTools include:
- Verifying a component’s props and state
- Addressing performance issues
- Inspecting the context values
By using React DevTools, you can pinpoint performance bottlenecks in the UI, test state changes and prop values directly, and debug React Hooks and other React features.
The tool also allows you to inspect the component hierarchy and view component properties in the Component tab, and use the search feature to quickly locate components by name.
Community Knowledge for Issues
The React community is an invaluable resource for solving common problems. You can seek assistance for issues like timeout errors or compatibility concerns through forums, GitHub, or Stack Overflow.
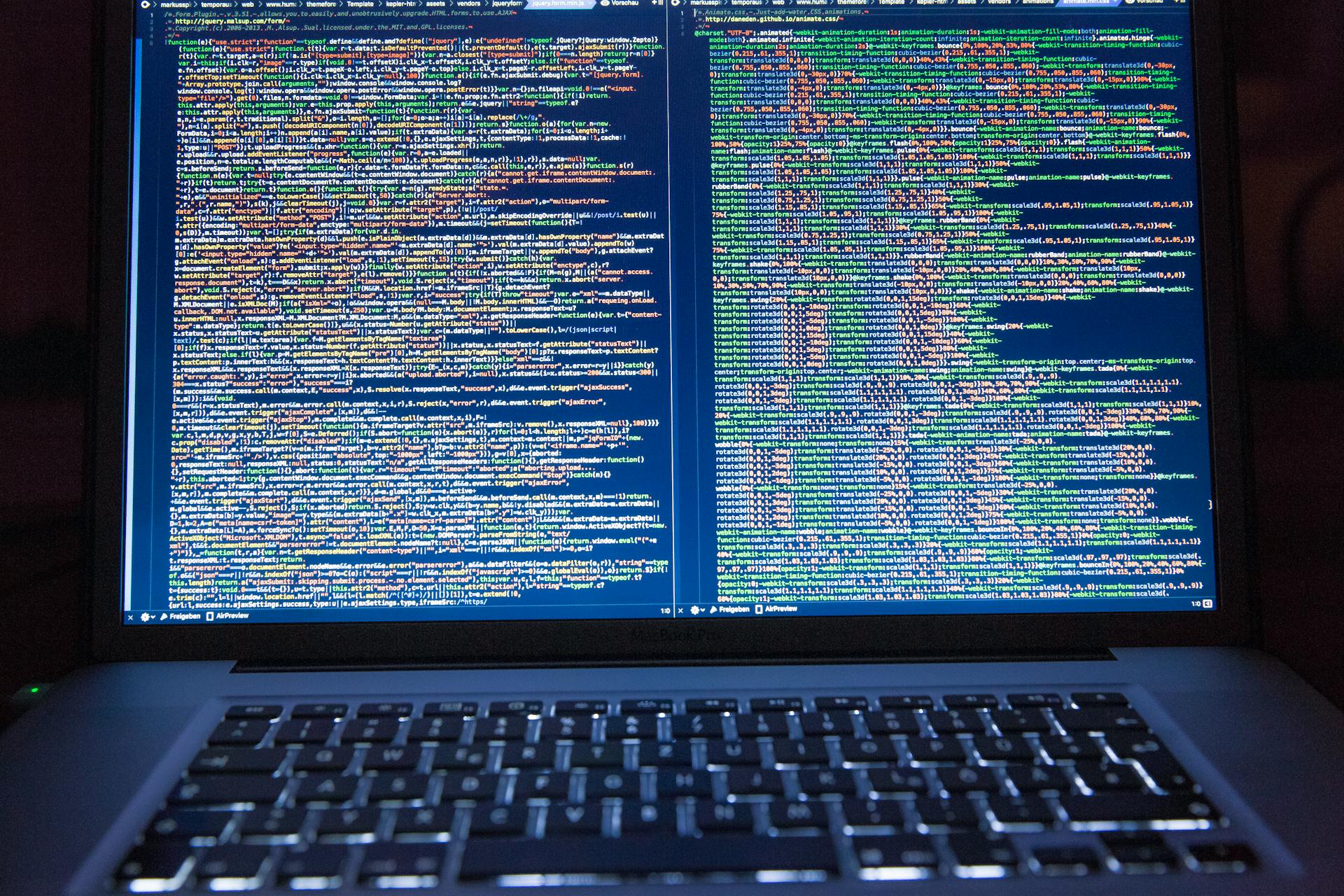
Even the most experienced developers can face challenges with React DevTools. Knowing how to troubleshoot these issues is an essential skill.
The community's collective knowledge and experience can help you overcome obstacles and find solutions. This is especially true when you're stuck on a specific problem and need a fresh perspective.
Developers can leverage community knowledge to solve common problems and improve their development workflow. This can lead to increased productivity and a better overall experience.
Frequently Asked Questions
How to connect React Dev Tools?
To connect React Dev Tools, install the package with npm install --save-dev react-devtools and run npm run react-devtools from your project folder. This will open the DevTools for debugging and optimizing your React application.
Sources
- https://draft.dev/learn/how-to-use-react-devtools-in-safari
- https://www.npmjs.com/package/react-devtools
- https://blogs.purecode.ai/blogs/react-developer-tools/
- https://www.dhiwise.com/post/how-to-fix-the-error-react-dev-tools-timed-out
- https://www.syncfusion.com/blogs/post/debugging-react-applications-with-react-devtools
Featured Images: pexels.com