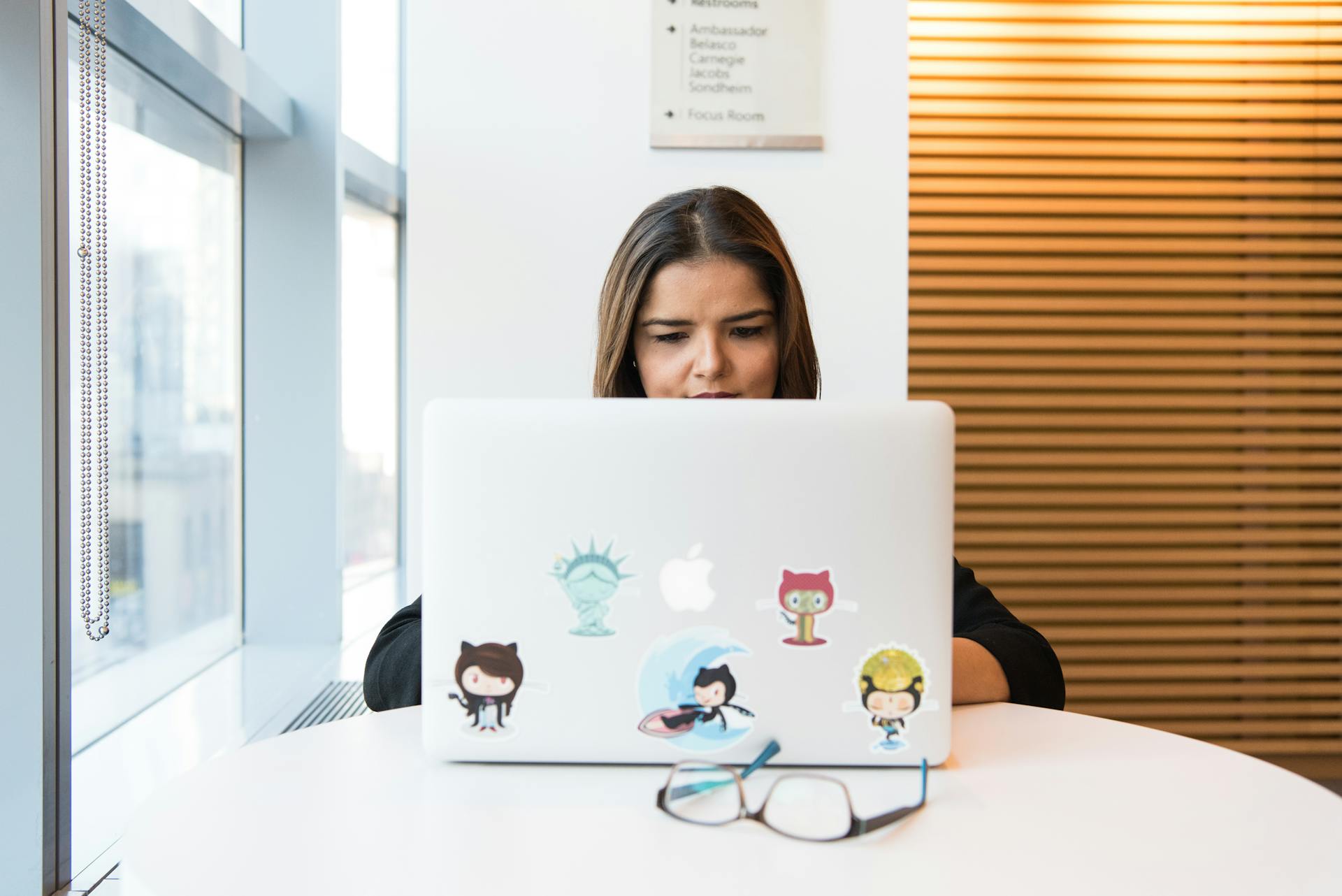
In this guide, we'll walk you through setting up Swagger API documentation for a Next.js project on GitHub. This involves creating a Swagger file, configuring it in Next.js, and pushing the changes to GitHub.
To start, you'll need to create a Swagger file, which is essentially a JSON file that describes your API. This file will contain information such as API endpoints, request and response formats, and authentication methods.
You can create a Swagger file using the OpenAPI Specification, which is a widely adopted standard for describing APIs. In a typical Swagger file, you'll define the API's structure, including the paths, methods, and parameters.
The Swagger file will serve as the foundation for your API documentation, making it easier for developers to understand and interact with your API.
A unique perspective: Nextjs Github Pages
Getting Started
API documentation is an integral part of developing software. It's an instruction manual that explains how to use an API and its services.
To get started with Swagger API documentation, you'll need to use a tool called Swagger. Swagger allows you to describe the structure of your APIs so that machines can read them.
First, you'll need to install Swagger in your Node.js project. This will enable you to create a YAML or JSON file that contains a detailed description of your entire API.
This file is essentially a resource listing of your API which adheres to OpenAPI Specifications.
Here's a quick rundown of the basic steps to get started with Swagger:
- Install Swagger in your Node.js project.
- Use Swagger to describe the structure of your API.
- Generate a YAML or JSON file that contains a detailed description of your API.
By following these steps, you'll be well on your way to creating beautiful and interactive API documentation using Swagger.
API Documentation
API documentation is crucial for any API, and Swagger is a great tool to help you create it. You can use Swagger Editor to create an API documentation by adding a simple header and routes or endpoints. The Swagger Editor is easily accessible online at editor.swagger.io.
There are two ways to build Swagger documentation for your API: manually writing the specs inside the router files or using existing developer's tools or packages to generate the documentation automatically. In this case, we will use the manual approach to ensure accuracy in our definitions and specifications.
To create an API documentation, you can add the following information: API endpoint, request type, request body, parameters, content type, schema, and response. You can also use Swagger UI and configurations to integrate your API documentation into your app. To do this, you need to install the "swagger-jsdoc" and "swagger-ui-express" packages and create a new file called swagger.js in the root directory of your application.
A fresh viewpoint: Next Js Use Context
Adding Configurations
You can add configurations to your API documentation in two ways. First, you can manually write the specs inside the router files or a dedicated JSON or YAML file. This approach ensures accuracy in your definitions and specifications.
To use the manual approach, you'll need to install two packages called "swagger-jsdoc" and "swagger-ui-express" as dependencies. You can do this using the command mentioned in the tutorial.
After installation, create a new file called swagger.js in the root directory of your application and paste the code into it. This code defines the Open API Specification (OAS) that you'll be using for your documentation.
You can also use existing developer's tools or packages to generate the documentation automatically. One such tool is "next-swagger-doc", which allows you to generate your OpenAPI Specification in various formats.
Here are the two ways to generate OpenAPI Specification:
To use "next-swagger-doc", you can follow the implementation guide on the npm package page. This will give you a range of options for generating your OpenAPI Specification, including a .json file via the CLI.
API Documentation
API Documentation is a crucial part of any API, allowing developers to understand how to interact with it. It's a standard, language-agnostic interface that humans and computers can use to understand your API service with minimal implementation context.
You can create API documentation using Swagger, a tool that allows you to generate visual displays of your API service and integrate testing tools. Swagger Editor is a great resource for creating your API documentation, and it supports both YAML and JSON formats.
To create an API documentation, you can follow these steps: first, define the OpenAPI Specification (OAS) for your API, including information such as API name, description, version, and base URL. Then, add the routes or endpoints for your API, including request types, request bodies, parameters, and responses.
Here's an example of what the OpenAPI Specification might look like:
- API name: My API
- Description: This is a sample API
- Version: 1.0
- Base URL: https://api.example.com
- License: MIT
- Important Links: https://github.com/my-api
You can also use next-swagger-doc to generate your OpenAPI Specification from your Next.js routes' comments. This method is useful if you want to automatically generate your API documentation from your code.
Once you have your OpenAPI Specification, you can use it to generate visual displays of your API service, such as Swagger UI. You can also use it to integrate testing tools, such as Postman.
API documentation can be served on a /docs route, and it can be exported as a JSON file. You can also use it to generate static files of the Swagger API pages into a docs directory, as oppose to having your server serve it.
Worth a look: Nextjs 13 Docs
Here are some benefits of documenting your API routes with OpenAPI Specifications:
- OAS is a standard, language-agnostic interface that humans and computers can use to understand your API service with minimal implementation context.
- One of the best ways to integrate your API routes to Swagger is to have developers define an OpenAPI Specification when they create an API route.
By following these steps and using the right tools, you can create high-quality API documentation that will make it easier for developers to understand and interact with your API.
OpenAPI Specifications
OpenAPI Specifications are a standard, language-agnostic interface that humans and computers can use to understand your API service with minimal implementation context. This means that OpenAPI Specifications can be useful for generating visual displays of your API service, generating client- and server-side code to interact with your API, or integrating testing tools.
You can create your OpenAPI spec with next-swagger-doc dependency, which allows you to natively create your Swagger documentation, generate your OpenAPI specification to a page, or generate a .json file via the CLI. This can be done by following the implementation guide on the npmjs.com package page.
The benefits of documenting your Next.js API routes with OpenAPI Specifications include UI-driven API testing, non-technical people can see the benefits and purpose of your API at a high-level, and documentation can help acclimate new team members to your solution.
Intriguing read: Nextjs 404 Page
Benefits of OpenAPI Specifications
OpenAPI Specifications are a powerful tool for documenting and leveraging your API routes. They provide a standard, language-agnostic interface that humans and computers can understand.
One of the key benefits of OpenAPI Specifications is that they enable the generation of visual displays of your API service, making it easier for non-technical people to understand the benefits and purpose of your API at a high-level.
Having an OpenAPI Specification can also help acclimate new team members to your solution by providing a clear and concise overview of your API. This can save a lot of time and effort in onboarding new team members.
Here are some of the benefits of documenting your Next.js API routes with OpenAPI Specifications:
- OAS is a standard, language-agnostic interface that humans and computers can use to understand your API service with minimal implementation context.
- One of the best ways to integrate your API routes to Swagger is to have developers define an OpenAPI Specification when they create an API route.
- An OpenAPI Specification can be useful for generating visual displays of your API service, generating client- and server-side code to interact with your API, or integrating testing tools.
By leveraging your OpenAPI Spec using Swagger, you can enjoy benefits such as UI-driven API testing, which makes it easier to identify and fix issues with your API. This can save a lot of time and effort in the long run.
OpenAPI Comment Syntax
OpenAPI Comment Syntax is a powerful tool that helps you generate your OpenAPI specification from your Next.js routes' comments. This syntax is used by the next-swagger-doc method #3, which will generate your OpenAPI spec from your Next.js routes' comments.
To get started, you'll need to add comments to your routes using a specific format. This will allow next-swagger-doc to extract the necessary information and generate your OpenAPI specification.
Here's an example of what the comments might look like:
This documentation clearly describes everything we need to know about this route. It has no parameters, returns a JSON array with the properties name and description for each hero.
By using this syntax, you can easily generate your OpenAPI specification and make it available for others to use.
Intriguing read: Next.js
Sources
- https://dev.to/desmondsanctity/documenting-nodejs-api-using-swagger-4klp
- https://github.com/vercel/next.js/discussions/14589
- https://blogs.perficient.com/2022/09/13/nextjs-swagger-openapi/
- https://github.com/jellydn/next-swagger-doc/releases
- https://lo-victoria.com/build-interactive-api-documentations-easily-with-swagger
Featured Images: pexels.com