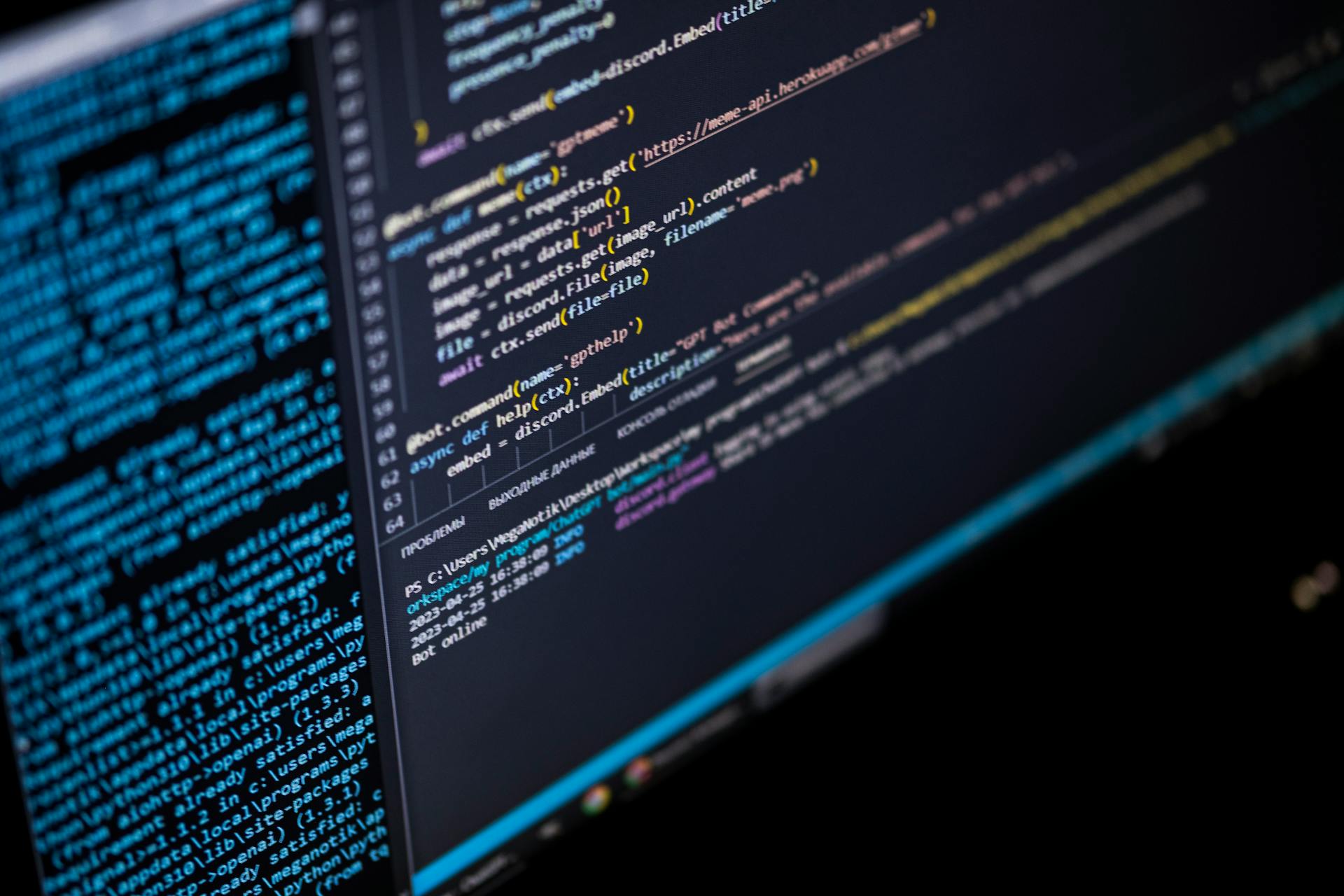
Next.js 13 introduces a new architecture that enables building fast, secure, and scalable applications. This new architecture is built on top of the Server Components API.
The Server Components API allows for server-side rendering of components, which improves performance and reduces the amount of data transferred to the client. This results in faster page loads and a better user experience.
By using the Server Components API, developers can also improve security by reducing the attack surface of their application. This is achieved by rendering sensitive data on the server-side, rather than on the client-side.
With Next.js 13, developers can now build applications that scale more easily, thanks to the improved architecture and new features.
For more insights, see: Nextjs Pathname Server Component
Getting Started
To get started with Next.js 13, you'll want to create a new application using the `create-next-app` tool, which sets up everything automatically for you.
Run the following command and follow the wizard to create a project. You'll be up and running in no time.
Enter the project directory and start the development server by executing a single command.
Quick Start
To get started with Next.js, create a new application using create-next-app, which sets up everything automatically for you.
This tool is a great way to quickly set up a Next.js project without having to do a lot of manual configuration.
Run the command to create a project and follow the wizard to set up your application.
The command is straightforward and easy to use, even for those new to Next.js.
Once the project is set up, enter the project directory and start the development server.
Discover more: Nextjs 14 New Features
Setting Up Markdoc
To set up Markdoc, you'll want to start by creating a separate workspace for it. In this workspace, create a top-level directory called "content" to hold all your markdown files, which will serve as the site's content.
Markdoc is designed to work seamlessly with Next.js, so you'll want to make sure you have both installed in your project. Next.js has a built-in way to collect markdown files and render them as web pages using a dynamic catch-all route.
See what others are reading: Next Js Markdown
The dynamic catch-all route relies on two built-in functions: getStaticPaths and getStaticProps. These functions work together to convert markdown files into URL slugs that render the markdown content as HTML.
The collected data is then returned as page props to be used in the page component. This allows you to manipulate the file content as needed, and access Node.js features server-side.
Here's an interesting read: Nextjs 404 Page
Client-Side Setup
When working with client-side setup, it's essential to remember that values need to start with NEXT_PUBLIC_ to be accessible on the client-side.
These values are crucial for making data accessible to the client, allowing for a smoother user experience.
Remember, the NEXT_PUBLIC_ prefix is a key identifier for client-side values, so make sure to include it when setting up your project.
You might like: Next Js Client Portal
Routing and Navigation
Next.js 13 introduces a new app directory that allows us to colocate files, making it easier to organize our code.
We can define various types of components in the app directory using a specific filename convention: pages are defined as page.tsx, layouts as layout.tsx, templates as template.tsx, and so on.
Discover more: Next Js Stripe
This approach is a big improvement over the current routing system, which required a specific directory structure for pages, API handlers, and other components.
We can place our components for a specific page right in the folder where it's defined, making it easier to manage our code and reduce clutter.
Here's a quick reference guide to the file naming conventions:
- pages: page.tsx
- layouts: layout.tsx
- templates: template.tsx
- errors: error.tsx
- loading states: loading.tsx
- not found pages: not-found.tsx
With the new app directory, we can also use route groups to group pages under a common path segment or layout, making it easier to manage complex routing scenarios.
Layouts
Layouts are foundational components that wrap pages, useful for displaying a common UI across pages, reusing data-fetching and logic. This is made possible by Next.js Layouts, a new functionality introduced in the App Router.
Layouts can be defined using the convention layout.tsx in the app directory, and Next.js will automatically wrap all pages within the folder where the layout is defined. For example, if we have a layout defined in app/(site)/layout.tsx, Next.js will wrap all pages in the app/(site) directory with this layout.
Additional reading: Next Js Chat App
The SiteLayout component will be used to display a common UI across all pages in the app/(site) directory. This is a great way to reuse data-fetching and logic across multiple pages.
With the new App Router, we can fetch data in parallel and pass it to the page component, solving the waterfall problem. This is a substantial performance improvement over the current routing system.
Layout components can be extremely useful in case you need to load some data needed in all pages of a directory. For example, we could load the user's profile in the layout component and pass it to the page components.
Here are the two options for rendering content in Next.js:
- Static Rendering: Your components can be pre-rendered at build time then stored for reuse on subsequent requests
- Dynamic rendering: Your Components are rendered on the server at request time and no result cache is done
Actions
Actions are a powerful tool in our development toolkit, allowing us to execute functions on the server instead of wiring them through an API handler.
Server Actions, still in alpha, enable us to send emails, update databases, and even redirect users to a new page.
Executing server-side logic with Server Actions eliminates the need for complex client-side state management due to data mutation.
Additional reading: Next Js Server Side Api Call
Enhanced Router
The Enhanced Router is a game-changer for Next.js developers. With conventional filenames, you can add various types of components in the app directory.
You can define pages as page.tsx, layouts as layout.tsx, templates as template.tsx, errors as error.tsx, loading states as loading.tsx, and not found pages as not-found.tsx. This is a big improvement over the current routing system.
This means you can organize your code in a more flexible and intuitive way. No more worrying about specific directory structures.
Here's a quick rundown of the new filename conventions:
- pages: page.tsx
- layouts: layout.tsx
- templates: template.tsx
- errors: error.tsx
- loading states: loading.tsx
- not found pages: not-found.tsx
With this new system, you'll be able to create a more maintainable and scalable codebase.
Client Components
Client Components are a fundamental part of building Next.js applications, and understanding how they work is crucial for efficient routing and navigation.
To define Client components, you need to specify the use client pragma at the top of the file. This allows you to use React hooks, Context, and browser-only APIs.
Recommended read: How to Use Reducer Api in Next Js 14
Client components cannot import server components, but you can pass a Server Component as a child or prop of a Client Component. This is a key difference between the two.
Here are the key benefits of using Client components:
- Use React hooks, Context, and browser-only APIs
- Pass Server Components as children or props
With Client components, you can create dynamic and interactive user interfaces that respond to user input and events.
Co-Locating Files in Routing
In Next.js 13, we can colocate our files, which means we can define any type of component in the app directory without it becoming a page.
This is a big improvement over the older pages router, where we had to use a specific directory structure to define pages, API handlers, and so on.
We can place our components for a specific page right in the folder where it's defined, making it easy to organize our code and reduce clutter.
For example, we could place our components for a specific page in the site directory, which becomes "pathless" when we use parentheses, like this: (site).
Recommended read: Next Js Useeffect
This means we can create new layouts, loading files, and pages in the site directory without adding a new path segment to the routing.
Here are the different types of components we can define in the app directory using a specific filename convention:
- pages are defined as page.tsx
- layouts are defined as layout.tsx
- templates are defined as template.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
- not found pages are defined as not-found.tsx
By colocating files in the app directory, we can place files close to where they are used, making it easier to maintain and update our code.
Redirecting from Layouts
Redirecting from layouts is a powerful feature in Next.js. You can redirect users to a different page from within a layout component.
For example, if you want to redirect users to the login page if they are not authenticated, you can do it in the layout component. This approach solves the problem of having to repeat the same logic across multiple pages.
Layouts can also redirect users using the redirect function imported from next/navigation. This is useful for common UI elements that need to be displayed across pages.
In the new Next.js App Router, you can fetch data in parallel and pass it to the page component, which is a substantial performance improvement over the current routing system. This means that redirects from layouts can be more efficient than ever before.
For another approach, see: Next Js 14 Redirect to Another Page Loading Indicator
Pages
Pages are defined in the new app directory using a special convention. This convention is a big improvement over the current system, which required a specific directory structure.
To define a page, you need to name the file `page.tsx`. This is a specific filename convention used by Next.js.
If you want to define the home page of your website, you can place the page in the `app/(site)` directory and name it `page.tsx`. This is a straightforward way to create a new page.
Here are the different types of pages you can create using the `page.tsx` convention:
- pages are defined as `page.tsx`
- not found pages are defined as `not-found.tsx`
This convention makes it easy to organize your code and create new pages. Just remember to use the correct filename and directory structure.
Frequently Asked Questions
What is the difference between Nextjs 12 and 13?
Nextjs 13 introduces server components, which render on the server without sending JavaScript to the browser, reducing bundle size and improving performance. This key change sets Nextjs 13 apart from its predecessor, Nextjs 12.
Is Nextjs 13 backwards compatible?
Next.js 13 is mostly backward compatible, but some breaking changes require updates to your source code. Check the documentation for a list of changes to ensure a smooth transition.
Is NextJS 13 stable?
Next.js 13.4 marks the stability of the App Router, but other features like Turbopack and Server Actions are still in beta and alpha stages respectively. The App Router is now considered stable, but other features are still in development.
Featured Images: pexels.com