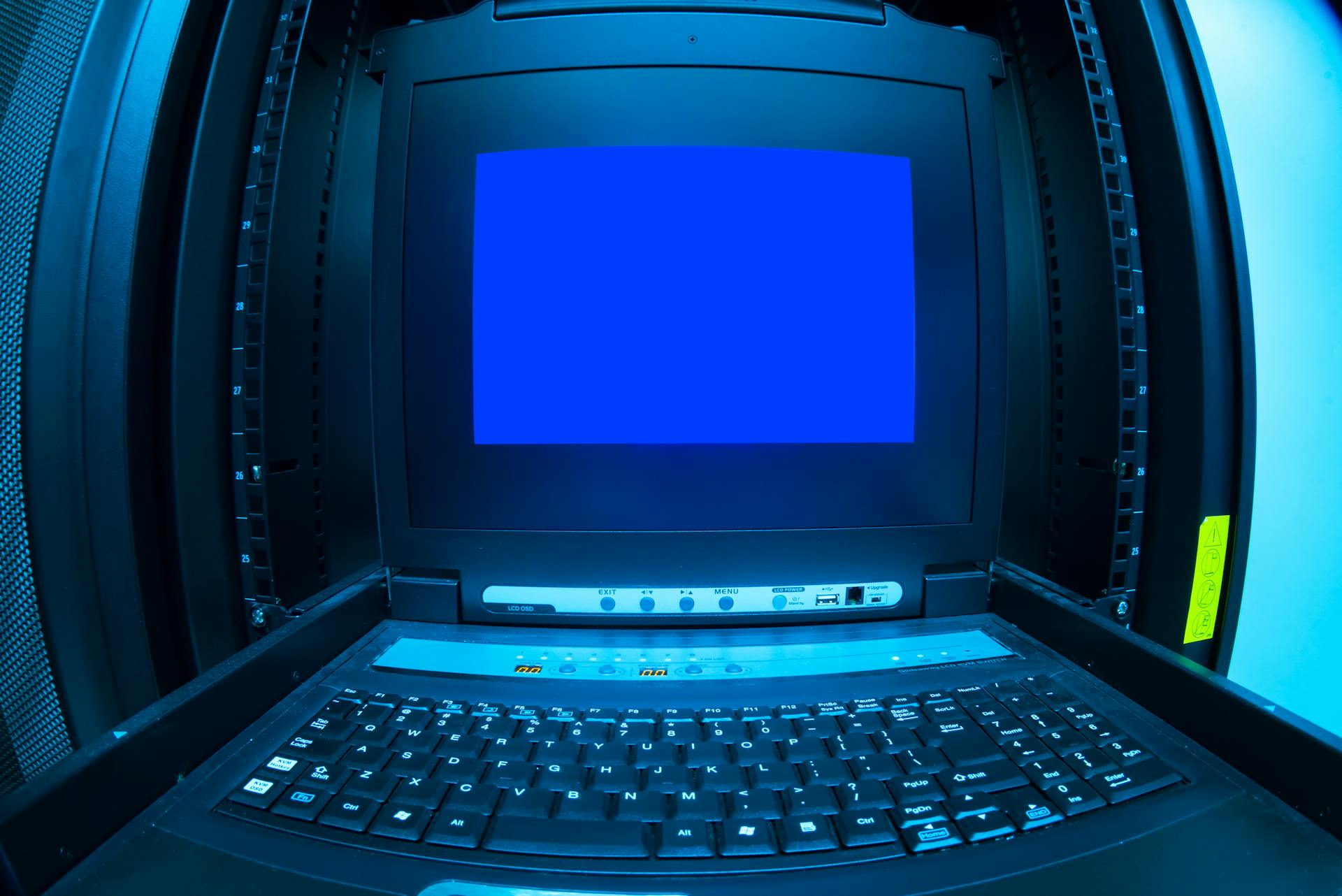
Configuring and managing webhooks in Azure involves creating a webhook service that can receive and process HTTP requests.
To create a webhook service, you need to create a new Azure Function.
This service will act as the endpoint that receives HTTP requests from other services.
You can then configure the webhook to trigger a specific Azure Function when an HTTP request is received.
The trigger is set up by specifying the HTTP method and the path of the request that should trigger the function.
This allows you to automate tasks and workflows by having other services send HTTP requests to your webhook service.
Create a Webhook
To create a webhook in Azure, you can start by creating a PowerShell runbook with the necessary code. This code will allow you to capture and process the webhook data. For example, you can use the following code in your runbook:
```
param (
[Parameter(Mandatory=$false)]
[object] $WebhookData
)
write-output "start"
write-output ("object type: {0}" -f $WebhookData.gettype())
write-output $WebhookData
write-output "`n`n"
write-output $WebhookData.WebhookName
write-output $WebhookData.RequestBody
write-output $WebhookData.RequestHeader
write-output "end"
if ($WebhookData.RequestBody) {
$names = (ConvertFrom-Json -InputObject $WebhookData.RequestBody)
foreach ($x in $names)
{
$name = $x.Name
Write-Output "Hello $name"
}
}
else {
Write-Output "Hello World!"
}
```
You can then create a webhook using the Azure portal, PowerShell, or the REST API. To do this, you'll need to publish the runbook and use the PUT command to create the webhook. For example, you can use the following PowerShell cmdlet to send the PUT request:
```
Invoke-RestMethod -Uri "https://management.azure.com/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Automation/automationAccounts/{automationAccountName}/webhooks/{webhookName}?api-version=2020-01-13-preview" -Method Put -Body $body
```
Alternatively, you can use an Azure Resource Manager (ARM) template to create a webhook. This will allow you to create a webhook as part of a larger deployment. For example, you can create a file named `webhook_deploy.json` with the following code:
```
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"automationAccountName": {
"type": "String",
"metadata": {
"description": "Automation account name"
}
},
"webhookName": {
"type": "String",
"metadata": {
"description": "Webhook Name"
}
},
"runbookName": {
"type": "String",
"metadata": {
"description": "Runbook Name for which webhook will be created"
}
},
"WebhookExpiryTime": {
"type": "String",
"metadata": {
"description": "Webhook Expiry time"
}
},
"_artifactsLocation": {
"defaultValue": "https://raw.githubusercontent.com/Azure/azure-quickstart-templates/master/quickstarts/microsoft.automation/101-automation/",
"type": "String",
"metadata": {
"description": "URI to artifacts location"
}
}
},
"resources": [
{
"type": "Microsoft.Automation/automationAccounts",
"apiVersion": "2020-01-13-preview",
"name": "[parameters('automationAccountName')]",
"location": "[resourceGroup().location]",
"properties": {
"sku": {
"name": "Free"
}
},
"resources": [
{
"type": "runbooks",
"apiVersion": "2018-06-30",
"name": "[parameters('runbookName')]",
"location": "[resourceGroup().location]",
"dependsOn": [
"[parameters('automationAccountName')]"
],
"properties": {
"runbookType": "Python2",
"logProgress": "false",
"logVerbose": "false",
"description": "Sample Runbook",
"publishContentLink": {
"uri": "[uri(parameters('_artifactsLocation'), 'scripts/AzureAutomationTutorialPython2.py')]",
"version": "1.0.0.0"
}
}
},
{
"type": "webhooks",
"apiVersion": "2018-06-30",
"name": "[parameters('webhookName')]",
"dependsOn": [
"[parameters('automationAccountName')]",
"[parameters('runbookName')]"
],
"properties": {
"isEnabled": true,
"expiryTime": "[parameters('WebhookExpiryTime')]",
"runbook": {
"name": "[parameters('runbookName')]"
}
}
}
]
}
],
"outputs": {
"webhookUri": {
"type": "String",
"value": "[reference(parameters('webhookName')).uri]"
}
}
}
Configuring Webhook Properties
Configuring Webhook Properties is a crucial step in setting up your Azure webhooks. The properties you must configure include Name, URL, Expiration date, and Enabled.
The Name property is simply a label you give to your webhook, used for identification purposes only.
You can't specify a custom URL for your webhook, it's automatically generated when you create it. This URL contains a security token that allows a third-party system to invoke the runbook with no further authentication.
Make sure to note the URL in a secure location for future use. You can only view the URL in the Azure portal when creating the webhook.
The Expiration date property determines when your webhook can no longer be used. You can modify this date after the webhook is created, as long as it hasn't expired.
If you set the Enabled property to Disabled, no client can use the webhook. You can set this property when you create the webhook or any other time after its creation.
The URL property is automatically generated when you create the webhook, and it's used to invoke the runbook with no further authentication.
Here are the Webhook Properties in a summary table:
Registering and Managing Webhooks
Registering and managing webhooks is a crucial step in setting up your Azure Function App. To register a Webhook in the Plugin Registration Tool, follow these steps:
You can select the Authentication type as WebhookKey. To get the key, go to the Function App in portal and look for the Get function URL link.
In the Plugin Registration Tool, register a new Webhook and enter Webhook Details. You can then copy the URL part from the Get function URL link and paste it in the Endpoint URL field.
Paste the URL part in the Endpoint URL and key in the Value field. Click Save to register the Webhook.
You can now add a Step to the Webhook, such as the update of Account's Account Name field.
Security and Authentication
Webhook security relies on the privacy of its URL, which contains a security token that allows the webhook to be invoked.
Azure Automation doesn't perform any authentication on a request as long as it's made to the correct URL, so clients shouldn't use webhooks for runbooks that perform highly sensitive operations without using an alternate means of validating the request.
To add an extra layer of security, you can include logic within a runbook to determine if it's called by a webhook. This can be done by checking the WebhookName property of the WebhookData parameter.
Here are some strategies to consider:
- Validate the request by looking for particular information in the RequestHeader and RequestBody properties.
- Have the runbook perform some validation of an external condition when it receives a webhook request, such as connecting to GitHub to validate a new commit.
- Use Azure virtual network service tags, specifically GuestAndHybridManagement, to define network access controls on network security groups or Azure Firewall.
Security
Security is a top priority when it comes to webhooks. Azure Automation doesn't perform any authentication on a request as long as it's made to the correct URL, which contains a security token that allows the webhook to be invoked.
This means that your clients shouldn't use webhooks for runbooks that perform highly sensitive operations without using an alternate means of validating the request.
You can include logic within a runbook to determine if it's called by a webhook by checking the WebhookName property of the WebhookData parameter. The runbook can perform further validation by looking for particular information in the RequestHeader and RequestBody properties.
Having the runbook perform some validation of an external condition when it receives a webhook request is another strategy. For example, consider a runbook that is called by GitHub whenever there's a new commit to a GitHub repository. The runbook might connect to GitHub to validate that a new commit has actually just occurred before continuing.
Here are some strategies to secure your webhooks:
- Include logic within a runbook to determine if it's called by a webhook.
- Have the runbook perform some validation of an external condition when it receives a webhook request.
- Use Azure virtual network service tags, specifically GuestAndHybridManagement, to define network access controls on network security groups or Azure Firewall.
By implementing these strategies, you can ensure that your webhooks are secure and only invoked by authorized sources.
Azure Automation
Azure Automation is a powerful tool that allows you to automate tasks and workflows in Azure. You can create runbooks that can be started manually or automatically through various triggers, including webhooks.
A webhook is a way to start a runbook in Azure Automation through a single HTTP request. This allows external services such as Visual Studio Team Services, GitHub, or custom applications to start runbooks without implementing a full solution using the Azure Automation API.
You can create a webhook using the Azure portal, PowerShell, or the REST API. A webhook requires a published runbook, and you can create a runbook using Azure Automation's built-in runbook editor.
To create a webhook, you'll need to specify the runbook you want to start and any parameters you want to pass to the runbook. You can also specify a value for the WebhookData parameter, which defines an object containing data that the client includes in a POST request.
The WebhookData parameter has the following properties:
- WebhookName: The name of the webhook.
- RequestBody: The body of the incoming POST request, which can be in JSON, XML, or form-encoded format.
- RequestHeader: A PSCustomObject containing the headers of the incoming POST request.
These properties can be accessed in the runbook using the WebhookData object, and you can use the data to perform actions or make decisions in your runbook.
Azure Automation logs the values of all input parameters with the runbook job, so you can see the values of any parameters passed through the webhook in the job's logs. This can be useful for debugging or troubleshooting issues with your webhook or runbook.
You can also use Azure Automation webhooks to react to Azure alerts. Resources in Azure can be monitored by collecting statistics like performance, availability, and usage with the help of Azure alerts. When the value of a specified metric exceeds the threshold assigned or if the configured event is triggered, a notification is sent to the service admin or co-admins to resolve the alert.
Webhook-enabled runbooks can be used to react to these alerts by executing a runbook in response to the alert. This allows you to automate tasks or workflows in response to specific events or conditions in Azure.
In summary, Azure Automation webhooks provide a powerful way to automate tasks and workflows in Azure by allowing external services to start runbooks through a single HTTP request. By specifying the runbook and any parameters you want to pass to the runbook, you can create a webhook that can start a runbook in response to various triggers, including Azure alerts.
Using and Managing Runbooks
A webhook can define values for runbook parameters that are used when the runbook starts. This allows you to customize the runbook's behavior and make it more flexible.
To start a runbook with a webhook, you need to provide values for any mandatory runbook parameters and can include values for optional parameters. You can't override these parameter values when starting the runbook using a webhook.
Azure Automation logs the values of all input parameters with the runbook job, so be cautious about including sensitive information in webhook calls.
The WebhookData parameter has properties such as WebhookName, RequestHeader, and RequestBody, which contain data from the client's POST request. You can access these properties in your runbook to process the data.
Here are some common return codes from a POST request to a webhook:
Using a Runbook
To start a runbook, you can use a webhook, which is a unique URL that a client can call with an HTTP POST to trigger the runbook. The webhook URL contains a security token that allows the runbook to be invoked by a third party system with no further authentication.
You can prepare values to pass to the runbook as the body for the webhook call using PowerShell. For example, you can script the values as follows: $Names = @(@{ Name="Hawaii"}, @{ Name="Seattle"}, @{ Name="Florida"}) and then convert them to JSON using ConvertTo-Json -InputObject $Names.
The webhook response will contain the job ID in JSON format, which you can extract using the following PowerShell command: $jobid = (ConvertFrom-Json ($response.Content)).jobids[0].
Once the runbook is triggered, you can use the Get-AzAutomationJobOutput cmdlet to get the output. This cmdlet will return the output of the runbook, which you can then use to take further action.
Here is a list of the possible return codes you can receive from the POST request:
You can also use Azure Resource Manager (ARM) templates to create a runbook and a webhook. This can be done by creating a file named webhook_deploy.json and deploying it using the New-AzResourceGroupDeployment cmdlet.
The webhook has a validity time period of 10 years, after which it automatically expires. Once a webhook has expired, you can't reactivate it, but you can only remove and then recreate it.
Modifying Code for Dynamics 365 Call
To modify code for a Dynamics 365 call, you'll want to read the context, which can be done by simply reading the request into a string and logging it, just like we did in our example.
This allows you to see the request in the logs of your Azure Function app, making it easier to troubleshoot and debug.
You can also use the RemoteExecutionContext class to get all the contextual information into your Function app, which provides more flexibility and options for further processing.
Once you're ready with your modified code, don't forget to publish it to make it live and functional.
Clean Up Resources
Cleaning up resources is an essential part of managing runbooks. You can delete a webhook using PowerShell with the Remove-AzAutomationWebhook cmdlet.
To use this cmdlet, you'll need to specify the resource group, automation account name, and webhook name. Here's an example of how to do it: Remove-AzAutomationWebhook -ResourceGroup $resourceGroup -AutomationAccountName $automationAccount -Name $psWebhook.
Alternatively, you can use the REST Webhook - Delete API to delete a webhook. This involves sending a DELETE request to the specified URI with the correct headers.
To check if the deletion was successful, look for a status code of 200. This indicates that the deletion was completed successfully.
Frequently Asked Questions
What are webhooks used for?
Webhooks automate communication between applications by sending data via HTTP when specific events occur, enabling seamless workflows and integration. They're ideal for triggering actions in response to events, such as updates or changes, in various environments.
Sources
- https://community.dynamics.com/blogs/post/
- https://learn.microsoft.com/en-us/azure/automation/automation-webhooks
- https://samcogan.com/triggering-azure-automation-runbooks/
- https://knowledgebase.autorabit.com/product-guides/arm/arm-features/webhooks/configure-a-webhook-in-microsoft-azure
- https://github.com/Huachao/azure-content/blob/master/articles/automation/automation-webhooks.md
Featured Images: pexels.com