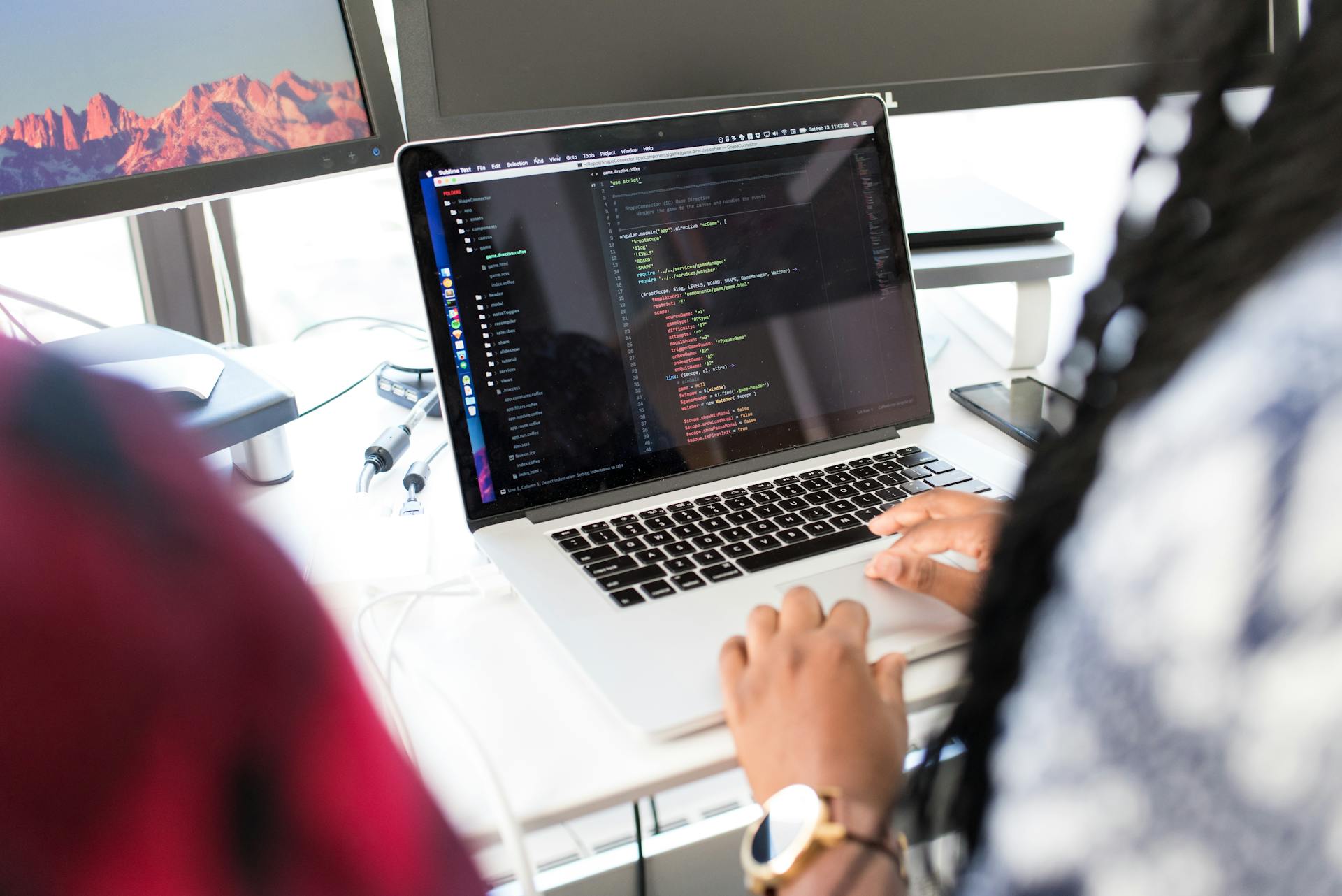
Azure DevOps Version Control and Git are two popular version control systems used by developers worldwide. Azure DevOps offers a built-in version control system that integrates well with its other services.
One key difference between the two is that Azure DevOps is a centralized system, whereas Git is a distributed system. This means that with Azure DevOps, all your code is stored in a single location, whereas with Git, each developer has a local copy of the code.
Azure DevOps also offers a more streamlined workflow, with features like pull requests and code reviews, which can help catch errors and improve code quality.
Prerequisites
To get started with Azure DevOps version control, you'll need to meet some prerequisites. You'll need to have Visual Studio Code with the C# extension installed, as well as Git for Windows 2.21.0 or later.
To access and contribute to code in Azure DevOps projects, you'll need to meet certain access requirements. You must be a member of an Azure DevOps project with Basic access level or higher to view code in private projects.
Here's a breakdown of the access levels you'll need:
- Basic access level or higher for private projects
- Contributors security group or corresponding permissions for private projects
- Public projects can be accessed by anyone, but you must be a member of the Contributors security group or have the corresponding permissions to clone or contribute to code
Additionally, you'll need to ensure that Repos is enabled in your Azure DevOps project settings. If you don't see the Repos hub and associated pages, you can reenable it by following the instructions in the Azure DevOps documentation.
Configuring and Using VS Code
To configure Visual Studio Code, start by opening the application. This will allow you to access the terminal and configure a Git credential helper to securely store your Git credentials used to communicate with Azure DevOps.
From the main menu, select Terminal | New Terminal to open a terminal window. This will give you a command prompt where you can execute Git commands.
To configure a credential helper, execute the command `git config --global credential.helper wincred`. This will securely store your Git credentials.
To configure your user name and email for Git commits, replace the parameters in the following commands with your preferred user name and email and execute them: `git config --global user.name "John Doe"` and `git config --global user.email [email protected]`.
Here are the steps to configure Visual Studio Code in a concise list:
- Open Visual Studio Code.
- Open a terminal window from the main menu.
- Configure a credential helper with the command `git config --global credential.helper wincred`.
- Configure your user name and email with the commands `git config --global user.name "John Doe"` and `git config --global user.email [email protected]`.
Version Control Basics
Version control is a fundamental aspect of Azure DevOps and Git. Commits are the building blocks of version control, and they consist of the changed files, a reference to the parent commit(s), and a descriptive message.
You can think of a commit as a snapshot of your code at a particular point in time. Git keeps the contents of all file changes in your repository in the commits, which keeps it fast and allows for intelligent merging. This makes it easy to track changes and collaborate with others.
To get started with version control, you can clone a remote Git repository to create a local copy of it. This creates both a copy of the source code and version control information so Git can manage the source code. You can also create a new local repository without cloning, but cloning is a great way to start.
Here are the basic components of a commit:
- The file(s) changed in the commit.
- A reference to the parent commit(s).
- A message describing a commit.
Cloning an Existing Repository
Cloning an existing repository is a fundamental concept in version control. It allows you to create a local copy of a remote Git repository.
You can clone a repository from the Azure DevOps web portal or using Visual Studio. To do this, navigate to your team project on Azure DevOps and click on the Repos hub.
In the Repos hub, click Clone and copy the repo clone URL to your clipboard. You can then plug this URL into any Git-compatible tool to get a copy of the codebase.
Visual Studio Code also provides a convenient way to clone a repository. Press Ctrl+Shift+P to show the Command Palette and execute the Git: Clone command.
To complete the cloning process, paste the repo clone URL into the Command Palette and select a local path to clone the repo to. You will then be prompted to log in to your Azure DevOps account.
Once the cloning has completed, you can click Open to open the cloned repository. Keep in mind that the solution may not be in a buildable state, but that's okay since we're going to focus on working with Git.
Here are the general steps to clone a repository:
- Navigate to your team project on Azure DevOps.
- Click on the Repos hub and click Clone.
- Copy the repo clone URL to your clipboard.
- Plug the URL into any Git-compatible tool to get a copy of the codebase.
- Paste the repo clone URL into the Command Palette in Visual Studio Code.
- Select a local path to clone the repo to.
- Log in to your Azure DevOps account.
- Click Open to open the cloned repository.
Saving Work with Commits
Saving work with commits is a crucial aspect of version control. You make changes to your files, and Git records those changes in your local repository.
Commits are made against your local Git repository, so you don't have to worry about the commit being perfect or ready to share with others. You can make more commits as you continue to work and push the changes to others when they are ready to be shared.
A commit consists of the file(s) changed in the commit, a reference to the parent commit(s), and a message describing the commit. This keeps it fast and allows intelligent merging.
Here's a breakdown of what's in a commit:
- The file(s) changed in the commit. Git keeps the contents of all file changes in your repo in the commits.
- A reference to the parent commit(s). Git manages your code history using these references.
- A message describing a commit. You give this message to Git when you create the commit.
To commit changes, you enter a commit message, like "My commit", and press Ctrl+Enter to commit it locally. You can also select the changes you want to commit by staging them, which allows you to selectively add certain files to a commit while passing over the changes made in other files.
Managing Branches
Managing branches in Azure DevOps is a breeze. You can manage your work in the Azure DevOps Git repo from the Branches view on the web, where you can customize the view to track the branches you care most about.
With branches, you can commit changes without affecting other branches, making it easy to share branches with others. You can also create new branches to isolate changes for a feature or bug fix from your master branch and other work.
To create a new branch, simply navigate to Repos | Branches in the Azure DevOps portal, click New branch, and enter a name for the new branch. You can also use the Work items to link dropdown to select one or more work items to link to this new branch.
Here's a step-by-step guide to creating a new branch:
- Switch to the Azure DevOps browser tab.
- Navigate to Repos | Branches. Click New branch.
- Enter a name of “release” for the new branch. Use the Work items to link dropdown to select one or more work items to link to this new branch. Click Create branch to create it.
- After the branch has been created, it will be available in the list.
- Return to Visual Studio Code.
- Press Ctrl+Shift+P to open the Command Palette.
- Start typing “Git: Fetch” and select Git: Fetch when it becomes visible. This command will update the origin branches in the local snapshot.
- Click the master branch.
- Select origin/release. This will create a new local branch called “release” and check it out.
You can also delete a branch in Azure DevOps by clicking the Delete branch from the more actions drop down. If you want to restore a deleted branch, you can search for an exact branch name and select Restore branch.
Locking a branch is a great way to prevent new changes that might conflict with an important merge or to place a branch into a read-only state. To lock a branch, simply select Lock from the master context menu, and to unlock it, select Unlock from the same menu.
Release Management
Release management is a crucial aspect of Azure DevOps version control. You can tag releases to mark specific versions of your site.
To tag a release, navigate to the Tags tab and click Create Tag. Enter a name and description for the tag, such as "v1.1" and "Great release!". You can also edit and delete tags as needed.
Azure DevOps offers flexibility in managing tags, including managing permissions. This means you have control over who can view and edit your tags.
Here are the steps to tag a release in Azure DevOps:
- Click Create Tag.
- Enter a name and description for the tag.
- Click Create.
By following these steps, you can effectively manage your releases and keep track of different versions of your site.
Managing Repositories
Managing repositories is an essential part of Azure DevOps version control. You can create a new repository from the Azure DevOps portal by selecting New repository from the project Add dropdown.
To create a new repository, you can set the Repository name to "New Repo" and optionally create a README.md file. You can also preconfigure the repo with a .gitignore file to specify which files to ignore from source control.
Azure DevOps offers a range of templates for the .gitignore file, including common patterns and paths to ignore based on the project type. This can save you time and ensure that you're ignoring the right files.
Once you've created a new repository, you can clone it using Visual Studio or other Git-compatible tools. To clone a repository, navigate to the Repos hub, click Clone, and copy the repo clone URL.
You can then open an instance of Visual Studio Code, press Ctrl+Shift+P to show the Command Palette, and execute the Git: Clone command. Paste in the URL to your repo and select a local path to clone the repo to.
Alternatively, you can manage your repository branches from the Azure DevOps portal or from Visual Studio Code. This allows you to easily switch between branches and collaborate with your team.
Here are the steps to clone a repository:
- Navigate to the Repos hub and click Clone.
- Copy the repo clone URL.
- Open Visual Studio Code and press Ctrl+Shift+P to show the Command Palette.
- Execute the Git: Clone command.
- Paste in the URL to your repo and select a local path to clone the repo to.
Collaboration and Sharing
Collaboration and Sharing is a breeze with Azure DevOps and GitHub, both of which offer robust tools for developer teams. Azure DevOps is a better option for closed-source projects, while GitHub excels for open-source projects, making their projects more accessible to the open-source community.
You can integrate Azure DevOps with GitHub using official DevOps features, allowing teams to adopt both solutions and leverage their strengths.
To keep your local branches in sync with remote counterparts, use the Fetch, Pull, Push, and Sync buttons in the Git Changes window, or select Fetch, Pull, Push, and Sync from the Git menu. This will download remote commits, merge them into your local branch, upload unpushed commits, and synchronize your branch with its remote counterpart.
Here's a summary of the Sync buttons:
By using these tools, you can collaborate and share your code with ease, whether you're working on an open-source or closed-source project.
Sync with Others
Syncing with others is a crucial part of collaboration, and Git makes it easy. You can keep your local branches in sync with their remote counterparts by pulling commits created by others.
To do this, you can use the Fetch, Pull, Push, and Sync buttons in the Git Changes window. Fetch downloads remote commits that aren't in your local branch, but doesn't merge them. Pull performs a fetch and then merges the downloaded commits into your local branch.
Here are the steps to sync with others:
- Fetch downloads remote commits that aren't in your local branch, but doesn't merge them.
- Pull performs a fetch and then merges the downloaded commits into your local branch.
- Push uploads your unpushed commits to the remote repository, which adds them to the corresponding remote branch.
- Sync performs a Pull then a Push.
You can also use the Git menu to select Fetch, Pull, Push, and Sync. In Team Explorer, select Home and choose Sync to open Synchronization. You can download the latest changes to your branch using the Pull link.
If you want to manually pull changes made by others, you can switch to the branch where you want to download the changes others have made, and then use the command `git pull`. Git will download the changes and merge them with your own changes into your local branch.
Choosing Between GitHub
If you're considering GitHub as an option for your team's collaboration needs, you should know that it's a powerful platform that offers DevOps solutions for software development teams.
GitHub is not identical to Azure DevOps, and it has some significant differences that may affect your choice.
GitHub provides a free plan for open-source projects, which can be a great option for teams that want to collaborate on projects without incurring costs.
However, if you're working on commercial projects, you may need to consider the paid plans offered by GitHub.
GitHub offers a wide range of integrations with other tools and services, which can be a major advantage for teams that want to streamline their workflow.
The free plan offered by GitHub has limitations, such as limited storage and collaboration features, which may not be suitable for large teams or complex projects.
Ultimately, the choice between GitHub and Azure DevOps will depend on your team's specific needs and requirements.
Flexibility and Customization
Flexibility and customization are crucial for effective collaboration and sharing.
GitHub is a flexible and customizable platform, allowing advanced users and large teams to tailor it to their needs.
You can use GitHub Enterprise, a self-hosted version of GitHub, to run it on your own servers or cloud.
Azure DevOps, on the other hand, is less flexible or customizable, with some features or integrations not available elsewhere.
You can use Azure DevOps Server to run some or all of the services on-premises, but it may not have all the features or updates of the cloud version.
If you're looking for a flexible and customizable solution, GitHub might be the better choice.
If you prefer a less flexible or customizable solution that follows a more standardized approach, Azure DevOps could be the way to go.
Key Concepts and Comparison
Azure DevOps and GitHub are both robust platforms that offer DevOps solutions, but they have significant differences.
Azure DevOps provides a comprehensive and integrated solution for all DevOps needs, though it may be complex for beginners and could prove expensive for large teams or projects.
GitHub, on the other hand, is popular for its simplicity and ease of use, especially for beginners and small teams.
The choice between Azure DevOps and GitHub depends on factors such as team size, project complexity, budget, preferences, and requirements.
Both platforms offer free plans with different limitations and paid plans based on different pricing models, which should be considered when choosing between Azure DevOps and GitHub.
To help you decide, here are the key differences between Azure DevOps and GitHub:
It's worth noting that both platforms have their strengths and weaknesses, and it's possible to use both together to enhance DevOps capabilities.
GitHub Integration
GitHub Integration allows you to leverage the best of both worlds, combining the strengths of Azure DevOps and GitHub.
You can connect your Azure Boards project to your GitHub repositories and link your work items to your GitHub commits, pull requests, and issues. This way, you can use GitHub for software development while using Azure Boards to plan and track your work.
Azure Pipelines can automatically build, test, package, release, and deploy your GitHub repository code. You can use predefined templates or create your own pipelines using YAML or a graphical editor.
By creating a GitHub Release from Azure Pipelines, you can publish your artifacts to GitHub Packages. This way, you can use Azure Pipelines for CI/CD while using GitHub for package management and distribution.
GitHub Actions can be used to trigger workflows based on events in Azure DevOps. This allows for seamless integration between the two platforms.
Here are some examples of how you can integrate Azure DevOps and GitHub:
- You can add status badges of Azure Boards to your GitHub repository README file.
- You can view the linked work items in the Release summary page of Azure DevOps.
- You can sign in to Azure DevOps using your GitHub credentials.
- You can invite your GitHub collaborators to your Azure DevOps project.
Users and Use Cases
GitHub is beloved in the open-source community, where many projects have a GitHub repository to make the project accessible to the community.
Developer teams working on an open-source project can use a GitHub repository to make their project accessible to the open-source community.
Both Azure DevOps and GitHub integrate with popular tools, platforms, and services, including Microsoft Azure.
Developers can use either GitHub or Azure DevOps with Azure to take advantage of its security offerings, made possible through partnerships with cybersecurity firms like Coalfire.
CI/CD and Project Management
Both Azure DevOps and GitHub offer robust CI/CD and project management tools to help developers streamline their workflows.
Azure DevOps has its own automation and CI/CD tool, Azure Pipelines, which supports hosted agents for public and private projects.
GitHub Actions is another popular CI/CD tool, but it lacks some features, such as scale set agents, that Azure Pipelines offers.
Azure Pipelines offers more flexibility and scalability than GitHub Actions, making it a better choice for large-scale projects.
Azure DevOps also offers a wide range of project management features, including agile tools that support developer teams.
GitHub Projects, on the other hand, is primarily designed for project management of code hosted on GitHub.
If you need to manage projects that involve code from multiple sources, Azure DevOps is a better choice due to its wider compatibility.
In contrast, GitHub Projects is ideal for projects that keep their code exclusively on GitHub.
Frequently Asked Questions
Can Azure DevOps be used for version control?
Yes, Azure DevOps offers a robust version control platform with seamless integration capabilities. Discover how it can streamline your development process and elevate your team's productivity.
Is Azure DevOps Git or TFVC?
Azure DevOps uses standard Git for version control, not Team Foundation Version Control (TFVC). Learn more about using Git with Azure DevOps.
Featured Images: pexels.com