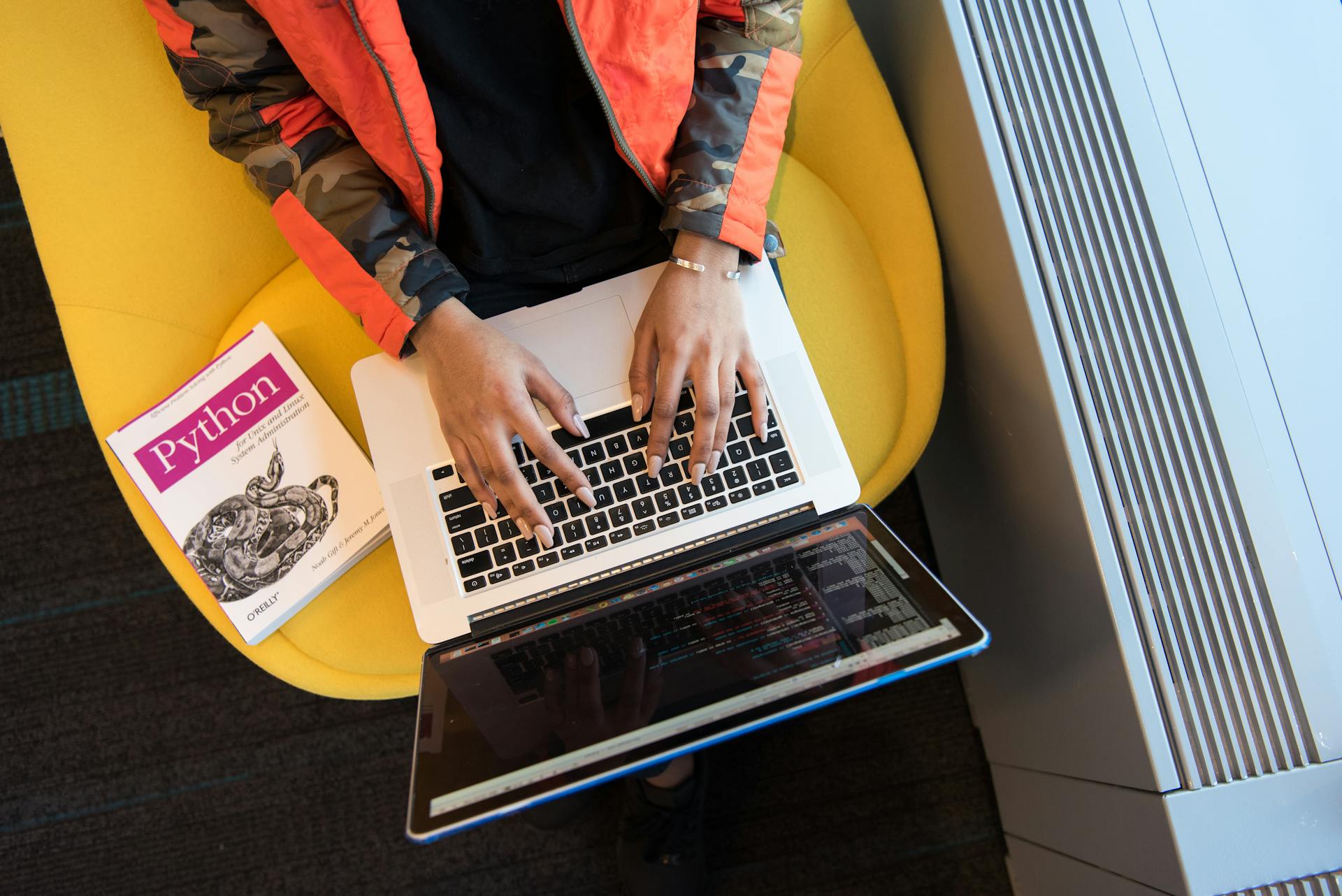
As a developer, you're likely familiar with Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design. Django is a popular choice for building complex and scalable web applications.
One of the key features of Django is its Model-View-Controller (MVC) architecture, which separates the application logic into three interconnected components. This allows for a clean and maintainable codebase, making it easier to debug and extend the application.
Django's ORM (Object-Relational Mapping) system provides a high-level interface for interacting with the database, abstracting away the underlying SQL syntax. This makes it easier to work with databases and reduces the risk of SQL injection attacks.
To work with Django, you'll need to have a solid understanding of Python and its syntax.
Project Setup
To set up a Django project, you can use the command `django-admin startproject project_name` in your terminal. This will create a basic directory structure for your project.
The project will have a basic directory structure, including a manage.py file and a project_name directory, which contains the basic project files. You can then navigate into the project directory and start working on your project.
By using this command, you can quickly set up a new Django project and start building your application.
Project Directory Structure
A Django project directory structure is made up of several important files. The most important file is setting.py, which holds all the configuration values that your web app needs to work.
The init.py file is an empty Python file that tells the Python interpreter that this directory is a package. This file is called when the package or one of its modules is imported.
The manage.py file is used to interact with your project from the command line utility. It allows you to manage several commands.
Here's a list of some of the key files in a Django project directory structure:
- init.py: an empty Python file that makes the directory a package
- manage.py: used to interact with your project from the command line utility
- setting.py: holds all the configuration values for your web app
- views.py: shows the user the model's data
- urls.py: contains all the endpoints for your project
- models.py: represents the models of your web application
- wsgi.py: used for deploying the project in WSGI
- admin.py: used to create a superuser and register models
- app.py: includes the application configuration for your app
Virtual Environment Setup
A virtual environment setup is crucial for Django projects. It allows you to establish separate dependencies for each project, creating an isolated environment that isn't related to each other.
Using a virtual environment is not strictly necessary, but it's ideal practice. This is because it eliminates dependencies and conflicts, making your projects more manageable.
With a virtual environment, you can quickly enable and deactivate it when you're done working on a project. This is a huge time-saver and helps prevent clutter.
It's worth noting that you can still work with Django projects without a virtual environment, but it's not the most efficient way to do things.
Starting Development Server
To start our development server, we can use the command provided in the documentation. This command is essential for testing and debugging our project.
The command to start the development server is straightforward and easy to execute. We just need to type it into our terminal or command prompt.
By starting the development server, we can see our project in action and make any necessary changes. This is a crucial step in the development process.
Development
In Django development, you'll encounter various concepts that require a solid understanding of the framework. To evaluate a developer's skills, ask them intermediate Django interview questions that assess their grasp of complex concepts and ability to apply them in real-world scenarios.
Handling file uploads in Django is a crucial task, and developers should be able to explain how to do it efficiently. They should also be able to implement pagination in a Django application, which involves dividing a large dataset into smaller, more manageable chunks.
Developers should also be familiar with managing different environments, such as development, staging, and production, in a Django project. This involves setting up separate configurations for each environment to ensure smooth deployment and testing.
Here are some key concepts to cover in the "Development" section:
- Handling file uploads
- Implementing pagination
- Managing different environments
These concepts are essential for a mid-tier Django developer, and asking the right questions during an interview will help you determine whether they have the necessary skills to handle complex development tasks.
MVC vs MVT Design Patterns
In MVC, Model and View are both driven by the controller, whereas Views in MVT are used to receive HTTP requests and return HTTP responses.
MVC is highly coupled, whereas MVT is loosely coupled, making it easier to modify in MVT.
MVC is suitable for large applications, but MVT is suitable for both small and large applications.
We must write all of the control-specific code in MVC, whereas we must write all of the control-specific code in the View and Template components in MVT.
Here's a comparison of MVC and MVT design patterns in a table:
The flow of MVT is sometimes harder to understand, whereas MVC has a clear and easy-to-understand flow.
Lifecycle
The lifecycle of a Django Response is a crucial part of the development process. It's responsible for data interchange between clients and servers.
As a request is made by the client to the server, information is passed through the system using request and response objects. These objects are transmitted over the web.
The request object contains metadata such as images, HTML, CSS, and JavaScript. This data is then loaded and presented to the user by Django.
The view method receives the HttpRequest as its first argument. It's the responsibility of each view to return a HttpResponse object.
Differences from Flask
Django is ideal for large projects, whereas Flask is more suited to smaller projects.
One of the key differences between Django and Flask is the presence of an in-depth administration panel, which Django offers but Flask does not.
Flask, on the other hand, doesn't provide visual debugging support, unlike Django.
Project Configuration
The project configuration in Django is crucial for setting up your web application. The settings.py file is the core configuration file, holding all the necessary values for your project to function.
This file includes database settings, logging configuration, static file URLs, API keys, and more. It's essentially a dictionary or list containing all the project's settings.
To create a Django project, you'll need to use the command, which will set up the basic project structure, including the settings.py file.
Here's a breakdown of the basic project directory structure:
- init.py: An empty Python file that tells the interpreter this directory is a package.
- manage.py: A file used for interacting with your project from the command line.
- settings.py: The most important file, containing all the project's configuration values.
- views.py: Where you define how data is presented to the user.
- urls.py: The universal resource locator containing all project endpoints.
- models.py: Where you define the models of your web application.
- wsgi.py: Used for deploying the project and communicating with the web server.
- admin.py: Used for creating a superuser, registering models, and logging in.
- app.py: Helps include application configuration.
Admin Interface
The admin interface is a vital part of any Django project, allowing you to create, read, update, and delete model objects with ease.
Django provides a default admin interface that's very helpful for administrative tasks, reading a set of data to create a quick interface where you can alter the application's contents.
This in-built module is a huge time-saver, allowing you to manage your project's data without having to write a lot of custom code.
To customize the admin interface functionality, you can use various methods, such as overriding templates or adding custom actions.
This flexibility makes it easy to tailor the admin interface to your specific needs, whether you're working on a small project or a large-scale application.
One of the best ways to view every item in the model is by using the Django admin interface, which provides a quick and easy way to manage your project's data.
In contrast, permanent redirection has some drawbacks, such as affecting search engine rankings and user experience.
To avoid these issues, it's essential to carefully consider your approach to redirection and use the most effective method for your project's needs.
What Does settings.py Do?
The settings.py file is a core file in Django projects, holding all the configuration values that your web app needs to work. This includes database settings, logging configuration, and where to find static files.
It's essentially the project's configuration hub, storing everything from databases and middlewares to backend engines, templating engines, and installed applications. This file is executed first when Django files are started, making it crucial for the project's functionality.
The settings.py file is a dictionary or list, containing various settings such as databases, middlewares, and security keys. This file is used to store API keys if you work with external APIs.
Here are some of the key settings stored in the settings.py file:
- Database settings
- Logging configuration
- Static file URLs
- Main URL configurations
- Authorized hosts
- Servers
- Security keys
This file is essential for the project's configuration, and any changes made to it can impact the project's functionality.
Configuring Static Files
Configuring static files is a crucial step in setting up a Django project. It involves adding a specific app to your project's INSTALLED_APPS list.
To do this, you need to make sure you've added 'Django.contrib.staticfiles' to your INSTALLED_APPS. This is a required step to enable static file configuration.
Next, you need to define the STATIC_URL in your settings.py file. This is where you specify the URL that will be used to serve static files.
Once you've configured STATICFILES_STORAGE, you can use the {% static %} template tag in your Django templates to establish the appropriate URL for the path that is given.
Finally, you need to add and store the static files in your project's static subfolder. This is where you'll keep all your CSS, JavaScript, pictures, and other types of static files.
Here are the four required steps to configure static files in Django:
- Making certain that you've added 'Django.contrib.staticfiles' to INSTALLED_APPS
- Establishing the definition in STATIC_URL
- Configuring STATICFILES_STORAGE
- Adding and storing the static files
Advantageous Features of
When building a project, it's essential to consider the configuration of your framework. One of the key advantages of Django is its ability to split HTML from business logic, making it easier to manage and maintain your code.
This feature is particularly useful when working on complex projects, as it allows developers to focus on different aspects of the codebase without getting tangled up in each other's work.
Django also offers the option to split the modules of code into groups, making it easier to organize and reuse code.
This modular approach can significantly reduce development time and improve collaboration among team members.
Some of the advantageous features of Django include:
- The option to split HTML from business logic
- The option to split the modules of code into groups
- The option to choose from exceptional libraries
By leveraging these features, developers can build robust and scalable applications that meet the needs of their users.
Security and Authentication
Django's security features are robust and comprehensive, protecting against common threats like cross-site scripting (XSS), SQL injection, and cross-site request forgery (CSRF).
Django's security features include cross-site scripting (XSS) protection, SQL injection protection, and cross-site request forgery (CSRF) protection, among others.
To ensure security in a Django application, you should use Django's authentication system and permission checks, implement CSRF protection, and enable HTTPS.
Here are some key security features of Django's authentication system:
- Users
- Permissions
- Groups
- Password Hashing System
- Forms Validation
- A pluggable backend system
What Is Superuser?
The superuser is the most powerful user with permission to create, read, delete, and update on the admin page.
To create a superuser, you must first have access to the Admin Panel, which is available to users of Django.
Before using this feature, you must migrate your project, otherwise, the superuser database will not be created.
To start, reach the same directory and run the command to create a superuser.
Ensuring Application Security
Django provides adequate security against common threats such as cross-site scripting (XSS), SQL injection, and cross-site request forgery (CSRF). Django's security features include XSS protection, SQL injection protection, CSRF protection, enforcing SSL/HTTPS, session security, clickjacking protection, and host header validation.
To prevent malicious attacks, Django provides a token per cent tag {% csrf_token %} that is implemented within the form. This token is generated on the server and cross-checked against any requests coming back in.
Security is a critical aspect of web development, and Django's built-in security features should be used to ensure secure applications. This includes using Django's authentication system and permission checks, implementing CSRF protection, enabling HTTPS, and properly handling user input.
Key security measures in Django include:
- Using Django's authentication system and permission checks
- Implementing CSRF protection
- Enabling HTTPS
- Properly handling user input and using parameterized queries to prevent SQL injection
Regular security audits and keeping Django and all dependencies up to date are also essential for maintaining a secure application.
Advanced Topics
Django supports six databases, including MySQL, PostgreSQL, and SQLite. Middleware is used to modify the request and response objects, and it's crucial for settings.py to be properly configured.
In Django, the `render` function is used to render a template with a given context. The `render` function is a shortcut that takes a template name, a dictionary of context variables, and an optional status code as arguments.
Django's mixin is a class that can be used to mix in functionality from multiple classes. You can use the `get_object_or_404` method to obtain one specific item in the model. This method raises a 404 error if the object doesn't exist in the database.
Here are a few ways to customize the admin interface functionality: Overriding the `get_queryset` method to change the data displayedAdding custom fields to the admin interfaceChanging the display of the admin interface
Django differs from Flask in several key areas, including its ORM, admin interface, and built-in support for internationalization and templating. Django's ORM provides a high-level interface for interacting with the database, while Flask relies on the underlying database driver.
What Are 'Signals'?
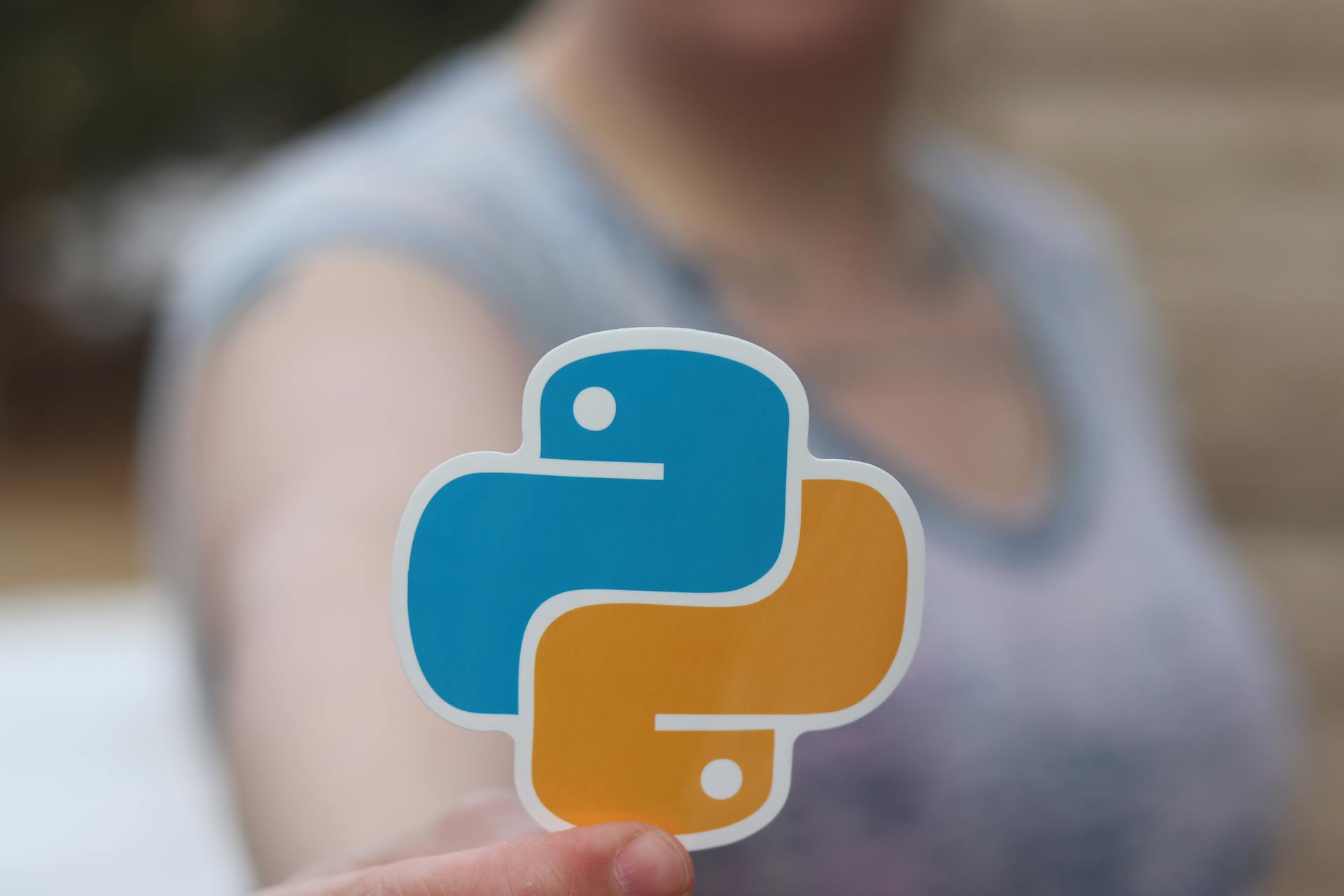
Signals are used to take action in response to the modification or creation of a database entry. They help connect events with their corresponding actions.
There are three types of signals, which are triggered at different stages of a model's lifecycle. These include pre_delete/post_delete, pre_init/post_init, and pre_save/post_save signals.
The pre_delete and post_delete signals are thrown before and after the remove() method is used to delete a model's instance. This allows you to perform any necessary actions before or after the deletion.
The pre_init and post_init signals are thrown before and after instantiating a model using the __init__() method. This can be useful for setting up or cleaning up model instances.
The pre_save and post_save signals work before and after the save() method is used to save a model instance. This can be used to perform any necessary actions before or after saving the data.
Here are the three types of signals, summarized in a table:
Understanding DRF and One Advantage
DRF refers directly to the Django REST framework, which makes building RESTful APIs easier.
The Django REST framework is a powerful tool for software engineers, and one of its key benefits is that it doesn't use much bandwidth when building RESTful APIs.
This means you can create robust and efficient APIs without worrying about consuming too much bandwidth, which is essential for any web application that needs to handle a large volume of requests.
Understanding of Architecture
Django's architecture is built around the Model-View-Template (MVT) pattern, which is a software design pattern for developing web applications.
In Django, the Model represents the data structure and handles database operations, making it an essential component of the MVT architecture.
The View is the user interface that renders a website page in your browser, using HTML/CSS/Javascript and Jinja files to represent it.
A template in Django is made up of both static sections of the desired HTML output and specific syntax that describes how dynamic content will be included.
Here's a breakdown of the MVT components and their roles:
The Django request/response cycle is a critical aspect of the architecture, involving the journey of a request from URL resolution through middleware to the view, and finally, the rendering of the response.
Database and Storage
Django's database support is quite impressive, with built-in support for SQLite, Oracle, PostgreSQL, and MySQL. It also uses third-party packages for Microsoft SQL Server, IBM DB2, SAP SQL Anywhere, and Firebird.
To connect your Django project to a database, you'll need to configure your database in the settings.py file, replacing the default SQLite setting with the one you prefer, such as Postgres, MongoDB, or MySQL.
Django's ORM (Object-Relational Mapping) system provides a high-level interface for interacting with databases, but for complex queries, raw SQL can be a better option. To optimize database queries, use techniques like select_related() and prefetch_related() to reduce the number of database queries, and implement database indexing on frequently queried fields.
Here are some key databases supported by Django:
- SQLite (inbuilt)
- Oracle
- PostgreSQL
- MySQL
- Microsoft SQL Server (via third-party packages)
- IBM DB2 (via third-party packages)
- SAP SQL Anywhere (via third-party packages)
- Firebird (via third-party packages)
What Are the Models?
Django models are a built-in feature that contain a definitive source of information about the data we're storing.
Each model maps to a single database table in Django.
To use Django Models, you'll need a project and an app to work with.
Django makes use of SQL to access the database.
Exception Classes Present
Exception classes are an essential part of Django's error handling mechanism, and they can be triggered by various scenarios. Django has its own set of exception classes to handle these situations, in addition to supporting all fundamental Python exceptions.
The MultipleObjectsReturned error is thrown by a query when it returns more objects than expected. This can happen when trying to retrieve a single item but getting multiple objects instead.
Django's ViewDoesNotExist exception is raised when a requested view does not exist. This can occur when a URL is not properly mapped to a view.
PermissionDenied is triggered when a user lacks the necessary permissions to perform a requested action. This is an important exception to handle, as it can prevent users from accessing certain features or data.
The SuspiciousOperation error is similar to MultipleObjectsReturned, as it's also thrown by a query when it returns more objects than expected. However, it's not as specific as MultipleObjectsReturned, as it's triggered in a broader range of situations.
Here's a list of some of the key exception classes in Django:
These exception classes can help you handle various errors and edge cases in your Django application.
What Is NoSQL?
NoSQL is a type of database that stores data in a non-tabular form, optimized for specific requirements of the data being stored.
It's a departure from traditional relational databases, which organize data into tables with defined relationships. NoSQL databases, on the other hand, use a storage model that's more flexible and adaptable to different types of data.
There are several types of NoSQL databases, including pure document databases, graph databases, wide column databases, and key-value stores. These variations are designed to handle specific use cases and data structures.
Django, a popular web framework, does not officially support NoSQL databases like CouchDB, Redis, Neo4j, and MongoDB.
Model Inheritance Styles
Model inheritance styles in Django allow you to create complex database relationships. Django supports three types of inheritance: Abstract base classes, Multi-table Inheritance, and Proxy models.
Abstract base classes are a type of inheritance in Django that allows you to define an abstract base class that cannot be instantiated on its own. This is useful for defining a common interface or set of methods that can be shared by multiple models.
In Django, you can define multiple models that inherit from a single abstract base class. This is useful for creating a hierarchy of models where some models inherit behavior from a parent model.
Here are the three types of model inheritance styles in Django:
- Abstract base classes
- Multi-table Inheritance
- Proxy models
Connecting to the Database
Django supports a wide range of databases, including SQLite, Postgres, MySQL, and more.
To connect your Django project to a database, you'll need to configure your database in the settings.py file. By default, SQLite is mentioned there, and you'll need to change this setting accordingly.
You can choose from several databases, including MySQL, Oracle, and PostgreSQL. Django also uses some third-party packages to handle databases like Microsoft SQL Server and IBM DB2.
Some of the databases supported by Django include:
- MySQL
- Oracle
- PostgreSQL
- Microsoft SQL Server
- Firebird
- IBM Db2
Note that while Django supports these databases, it's worth mentioning that NoSQL databases are not officially supported.
Filtering Model Items
Filtering Model Items is a crucial aspect of managing data in our database. We use a QuerySet to filter items present in our database.
A QuerySet is a database collection of data that is built up as a list of objects. We can use it to filter and organize the data.
The filter() method allows us to return only the rows that match the search word. This makes it easier to retrieve the information we need.
QuerySet makes our work easy by allowing us to filter and organize the data. It's also easier to retrieve the information that we need with its help.
We can use the filter() method to filter our data with the help of QuerySet.
What is CRUD?
CRUD is a simple yet powerful concept that helps software engineers build models for application programming interfaces. It stands for create, read, update, delete.
The CRUD operations are the fundamental actions that can be performed on data in a database. CRUD helps developers design and implement robust and efficient database systems.
CRUD operations are essential for managing data in a database. They enable users to interact with the data, whether it's creating new records, reading existing ones, updating them, or deleting them altogether.
The CRUD acronym is a helpful mnemonic device that reminds developers of the four core operations. It's a useful tool for anyone working with databases, from beginners to experienced professionals.
Optimization and Performance
Optimizing database queries is crucial for Django developer performance. Candidates should mention techniques like using select_related() and prefetch_related() to reduce the number of database queries.
Implementing database indexing on frequently queried fields can also have a significant impact. This technique helps the database retrieve data more efficiently, reducing query time and improving overall performance.
Here are some key techniques to look for in a candidate's answer:
- Using select_related() and prefetch_related() to reduce the number of database queries
- Implementing database indexing on frequently queried fields
- Utilizing QuerySet methods like values() or values_list() when full model objects aren't needed
- Employing caching strategies to store frequently accessed data
Monitoring query performance using Django Debug Toolbar or similar tools is also essential. This helps identify areas for improvement and ensures that the application is running efficiently.
Optimizing Database Queries
Optimizing Database Queries is crucial for Django developer performance.
Using select_related() and prefetch_related() can reduce the number of database queries, making your application faster.
Implementing database indexing on frequently queried fields can significantly speed up query performance.
Utilizing QuerySet methods like values() or values_list() is a good idea when you don't need full model objects.
Employing caching strategies to store frequently accessed data can also improve performance.
Here are some key techniques to optimize database queries in a Django application:
- Using select_related() and prefetch_related() to reduce the number of database queries
- Implementing database indexing on frequently queried fields
- Utilizing QuerySet methods like values() or values_list() when full model objects aren't needed
- Employing caching strategies to store frequently accessed data
What is Stability?
Stability is the backbone of any successful project, and it's essential to understand what it means in the context of optimization and performance. Django's stability is a game-changer, allowing engineers to code with confidence that their work will continue to function with future releases.
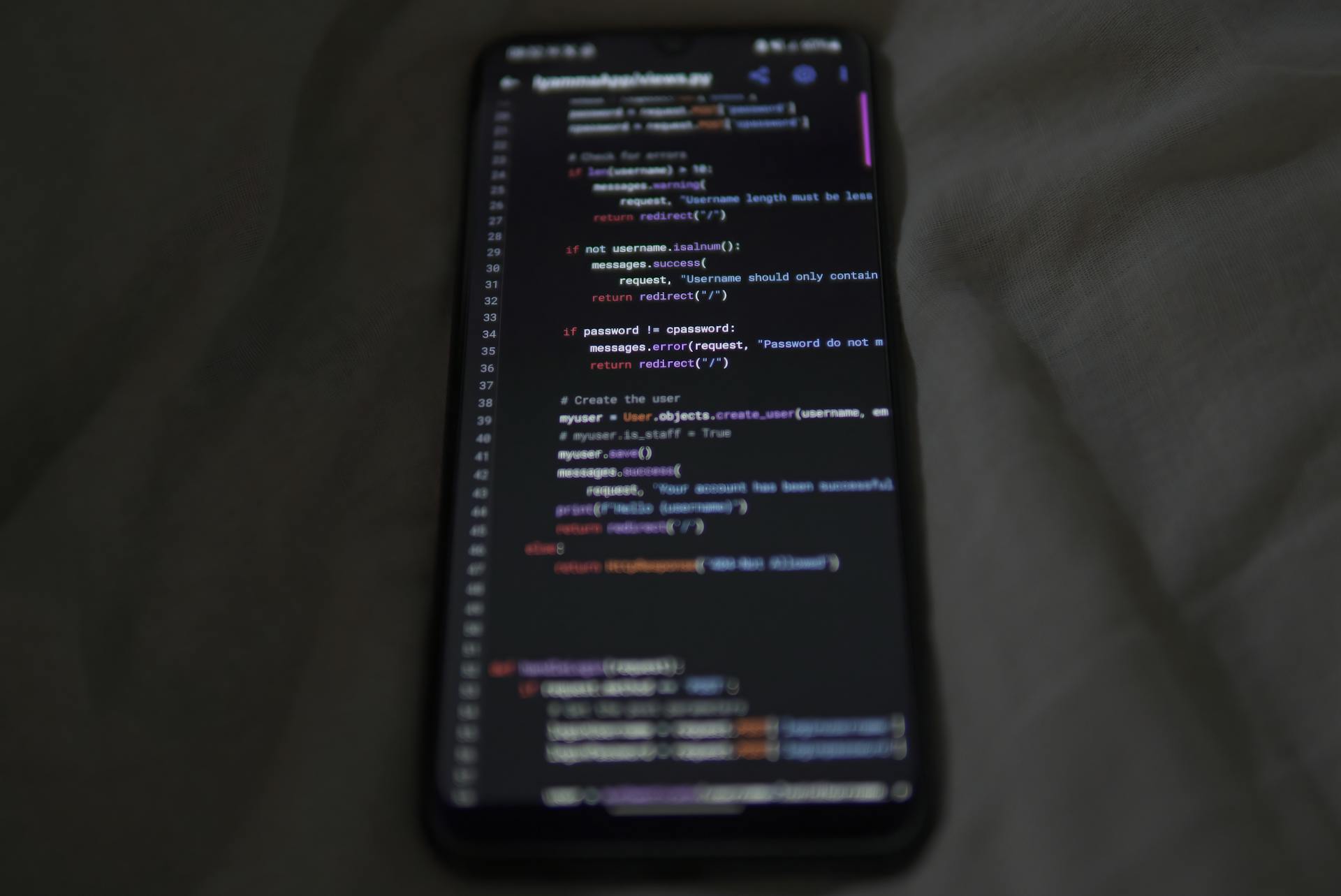
Engineers should be aware that Django's stability is ideal, ensuring compatibility for future releases. This means that code developed with Django will continue to work as expected, even with updates and patches.
Stability is not just about avoiding bugs, but also about ensuring that code is compatible with future versions of a framework or library. Django's stability makes it an excellent choice for projects that require long-term maintenance and updates.
Engineers can benefit from Django's stability by developing code that is less prone to errors and easier to maintain. This, in turn, can save time and resources in the long run.
Forms and Validation
Forms and validation are crucial aspects of building robust and secure web applications with Django. Django provides built-in form validation through its Forms API.
Django forms are Python classes that automatically generate HTML, handle server-side validation, and provide easy data processing. Unlike plain HTML forms, Django forms offer several advantages, including automatic HTML generation, server-side validation, and built-in CSRF protection.
To handle form validation in Django, candidates should mention using Django's Form class to define form fields and validation rules, implementing clean() methods for custom validation logic, utilizing built-in validators or creating custom validators, and handling form errors in views and templates.
Here are some key differences between Django forms and HTML forms:
- Automatic HTML generation
- Server-side validation
- Easy handling of form data in views
- Built-in CSRF protection
- Integration with Django's ORM for model-based forms
CharField vs TextField
CharField is a string field used for storing small strings like first names and last names. It's generally used for storing tiny text.
The main difference between CharField and TextField in Django is the amount of text they can store. TextField is used for big amounts of text, while CharField is used for small strings.
Here's a quick summary of the two fields:
In practice, I've found that using CharField for small text fields like names and addresses makes sense. It's a good rule of thumb to use TextField for larger text fields like paragraphs or descriptions.
Form Validation
Form validation is an essential part of working with forms in Django. It's a built-in feature that helps ensure user input is accurate and secure.
To handle form validation, you can use Django's Form class to define form fields and validation rules. This is a key part of the process.
Django forms provide automatic HTML generation, server-side validation, and easy handling of form data in views. This makes it easier to work with user input in a secure and efficient manner.
You can implement custom validation logic using the clean() method, which is a powerful tool for validating form data. This method is often used in conjunction with built-in validators.
Here are some key points to consider when handling form errors in views and templates:
- Use Django's Form class to define form fields and validation rules
- Implement custom validation logic using the clean() method
- Utilize built-in validators or create custom validators
- Handle form errors in views and templates
By following these best practices, you can ensure your forms are secure, efficient, and user-friendly.
Frequently Asked Questions
How to prepare for a Django interview?
To prepare for a Django interview, familiarize yourself with Django's core components, including views, models, templates, URLs, and forms, as well as key concepts like ORM, middleware, authentication, and Django Admin. Mastering these fundamentals will give you a solid foundation for tackling interview questions and real-world projects.
Sources
- https://www.geeksforgeeks.org/django-interview-questions/
- https://codeinterview.io/interview-questions/django-questions-answers
- https://www.adaface.com/blog/top-django-interview-questions-with-answers/
- https://www.testgorilla.com/blog/django-interview-questions/
- https://www.geeksforgeeks.org/django-interview-questions-answers-with-practical-tips-for-junior-developers/
Featured Images: pexels.com