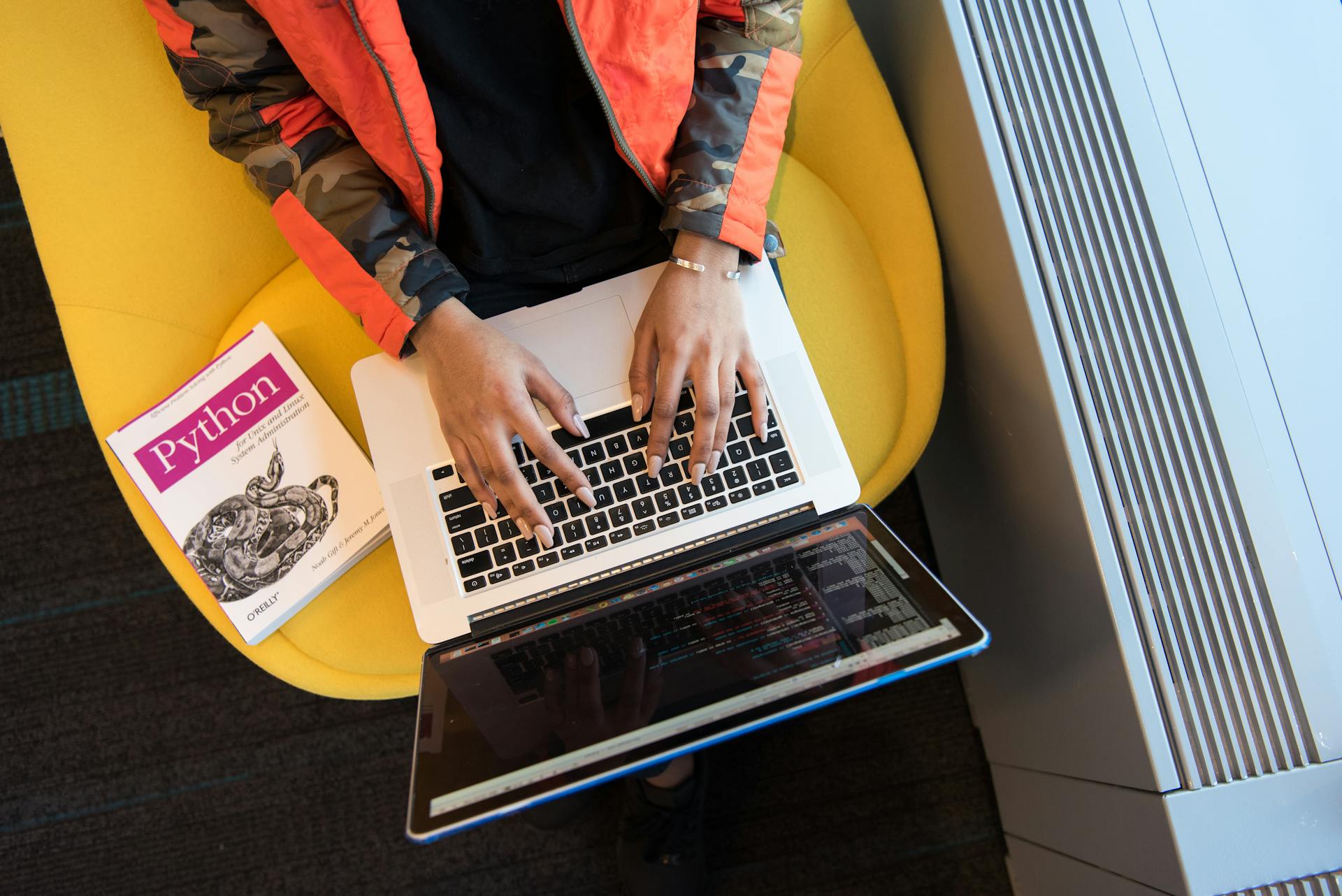
For large-scale Django applications, a well-structured project layout is crucial for maintainability and scalability.
Separate apps for different functionalities, like a separate app for the admin interface, can help keep the project organized.
This approach also makes it easier to reuse code across different projects.
A good example of this is the Django project structure for the Django REST framework, which separates the API into its own app.
In a large Django project, it's essential to keep the project root clean and focused on high-level configuration.
See what others are reading: Next Js App Router Project Structure
Django Project Structure
The architecture of a Django project is the invisible force that propels your development journey. It encompasses a well-thought-out plan that orchestrates the harmony between different components.
Django's project structure embodies the essence of the Model-View-Template (MVT) architectural pattern, segregating data, user interface, and control logic into distinct layers.
This framework-driven architecture inherently separates concerns, making code maintainable and development collaborative. Developers can easily navigate each other's work and contribute effectively.
For another approach, see: Frontend Development Projects with Vue.js 3
The project structure is not just about knowing where to find things, but also how to extend functionalities seamlessly. Understanding this structure empowers developers to create robust applications.
A template/project structure for developing django-based applications is available, using django-rest-framework along with django. This template is meant to be easily clone-able and used as the starter template for the next big thing.
The default Django project structure is what you'll see when you create a new project. Understanding the functions of the files in this structure will give you a context of what a project folder consists of.
The project structure is a folder structure only, not "best practices". This means you should use it as a starting point and adapt it to your needs.
Directory and File Structure
The main directory of a Django project is where everything starts. It's the root directory, containing settings, routing, and other high-level configurations.
manage.py is the gateway to various Django management commands, initiating the development server, creating applications, running migrations, and more. manage.py is the conductor's baton, guiding your project's activities.
The main project directory also contains several pivotal files, each with its significance in orchestrating the symphony of your development journey. Here's a breakdown of the common components you might find in a well-structured Django project:
The backend directory is the root directory of your Django project, containing settings, routing, and other high-level configurations. The app directory is where you create Django apps, each with a specific purpose, and reusable components that can be integrated into other projects.
Organizing Applications
Organizing applications in a Django project is crucial for maintainability and scalability. A well-structured project should have a clear distinction between projects and applications.
Developers can create independent pieces of functionality with reusable applications, promoting modularity and code reusability. This allows for easy integration into different projects, enhancing efficiency and maintainability.
Here's a breakdown of how to organize apps and modules effectively:
- Models: Define database models in models.py.
- Views: Handle requests and responses in views.py.
- Forms: Define forms in forms.py to handle user input.
- URLs: Define app-specific routes in urls.py.
- Admin: Customize Django's admin interface in admin.py.
- Migrations: Store database migrations in the migrations/ directory.
- Templates and Static Files: Optionally, include app-specific templates in a templates/ directory and static files in a static/ directory.
Organizing Applications Within
Organizing applications within a Django project is crucial for maintainability and scalability.
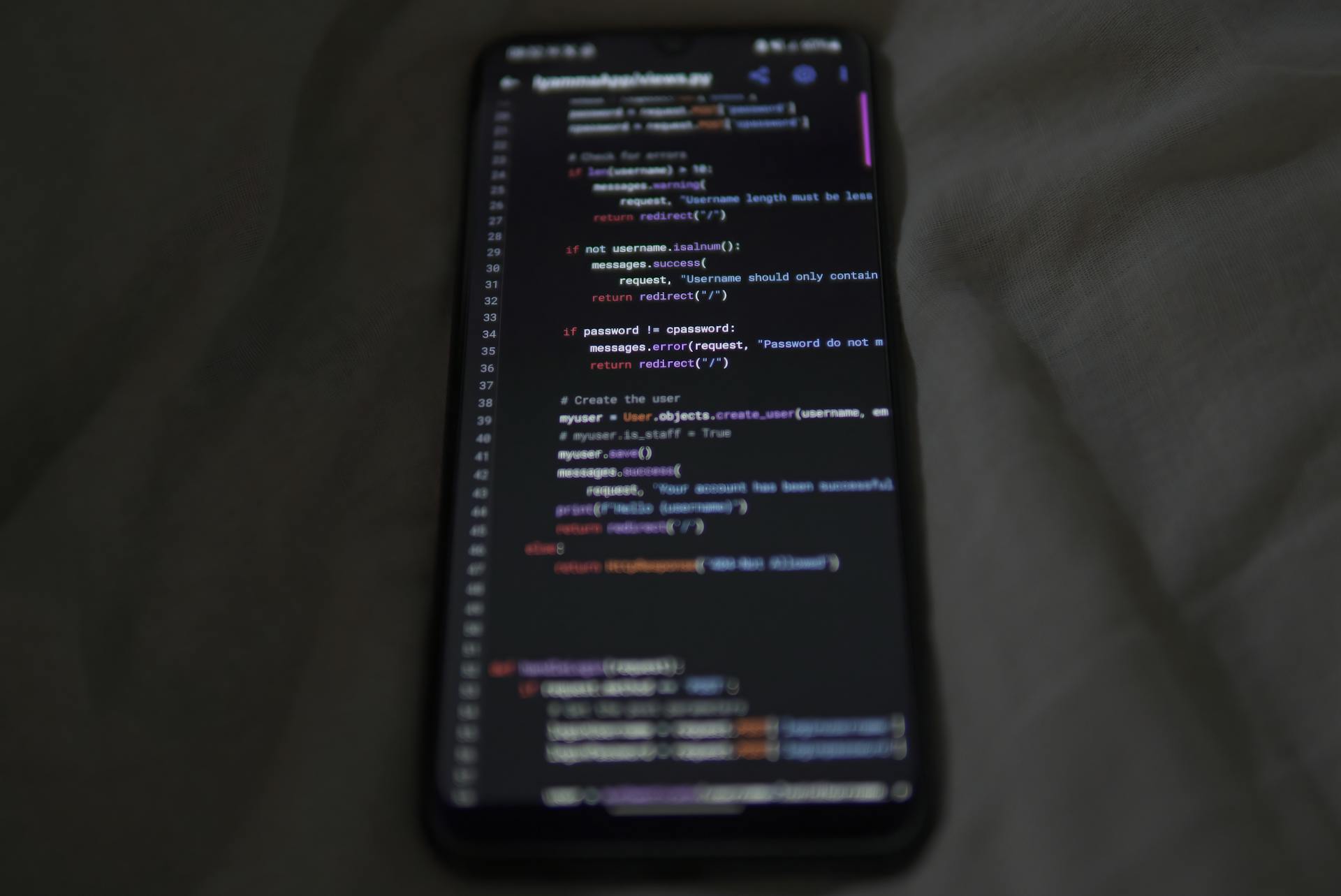
A fundamental principle in Django is the concept of "reusable applications", which promotes modularity and code reusability. This allows developers to build independent pieces of functionality that can be easily integrated into different projects.
To create a reusable application, you can use the `python manage.py startapp` command, which generates a skeletal framework with crucial configurations and settings.
Each application's directory is populated with files that collectively define its behavior and functionality. This includes models, views, forms, URLs, admin, migrations, templates, and static files.
Here's a breakdown of the common components you might find in a well-structured Django project:
By organizing applications within a Django project, you can foster well-defined roles, smooth collaboration, and maintainable code. This distinction between projects and applications paves the way for an organized development journey.
Roles
In a well-organized application, each file has a specific role to play. The models.py file defines the data structures using Django's ORM, forming the foundation of your application's data management.
A unique perspective: Google Data Structure and Algorithm
The views.py file encapsulates the logic that defines how your application interacts with users' requests, handling data processing and rendering templates. This file transforms user interactions into tangible responses.
Test-driven development gains momentum through the tests.py file, where you write unit tests to ensure your application's components function as expected. These tests bolster the reliability and stability of your codebase.
The admin.py file configures how your application's models are presented in Django's admin interface, allowing administrators to manage data seamlessly. This file is not just for administrators.
The migrations directory is a blueprint of all changes in your application models. It's a crucial component in ensuring data integrity and consistency.
Here's a breakdown of the key roles of each generated file:
- models.py: Defines data structures using Django's ORM
- views.py: Handles data processing and rendering templates
- tests.py: Ensures application components function as expected
- admin.py: Configures model presentation in Django's admin interface
- migrations: Tracks changes in application models
Understanding these roles helps developers create applications that are cohesive, maintainable, and feature-rich. Each file contributes to a specific aspect of your application's functionality, paving the way for an efficient and well-structured project.
Create Modular Apps
Creating modular apps is a great way to structure your Django project, making it easier to manage and maintain. This approach involves dividing your project into multiple apps, each focused on a specific task.
To create modular apps, start by dividing your project into multiple apps based on functionality, each handling a specific feature. This makes it easier to manage and reuse code. For example, you can separate apps for blog, users, and payments.
Here's a list of the key components to keep in mind when creating modular apps:
- Apps: Divide your project into multiple apps based on functionality.
- Views: Keep your views concise and focused on handling HTTP requests.
- Models: Organize models in a way that reflects your project's data structure.
- Templates: Use template inheritance to avoid code duplication.
- Utils: Create a utils module within your app for utility functions or classes.
By following these guidelines, you can create modular apps that are easy to understand, test, and maintain. This approach also makes it easier to collaborate with team members and scale your project without introducing unnecessary complexity.
Frequently Asked Questions
What is the root of a Django project?
The root of a Django project is the directory named after the project, which serves as the central hub for configuration and connection to Django. This directory contains the project's settings, split across multiple files within a Python module.
Sources
- https://medium.com/django-unleashed/django-project-structure-a-comprehensive-guide-4b2ddbf2b6b8
- https://medium.com/@akshatgadodia/a-comprehensive-guide-to-structuring-django-projects-best-practices-and-example-afb77d8497d5
- https://github.com/saqibur/django-project-structure
- https://www.askpython.com/django/django-app-structure-project-structure
- https://www.geeksforgeeks.org/best-practice-for-django-project-working-directory-structure/
Featured Images: pexels.com