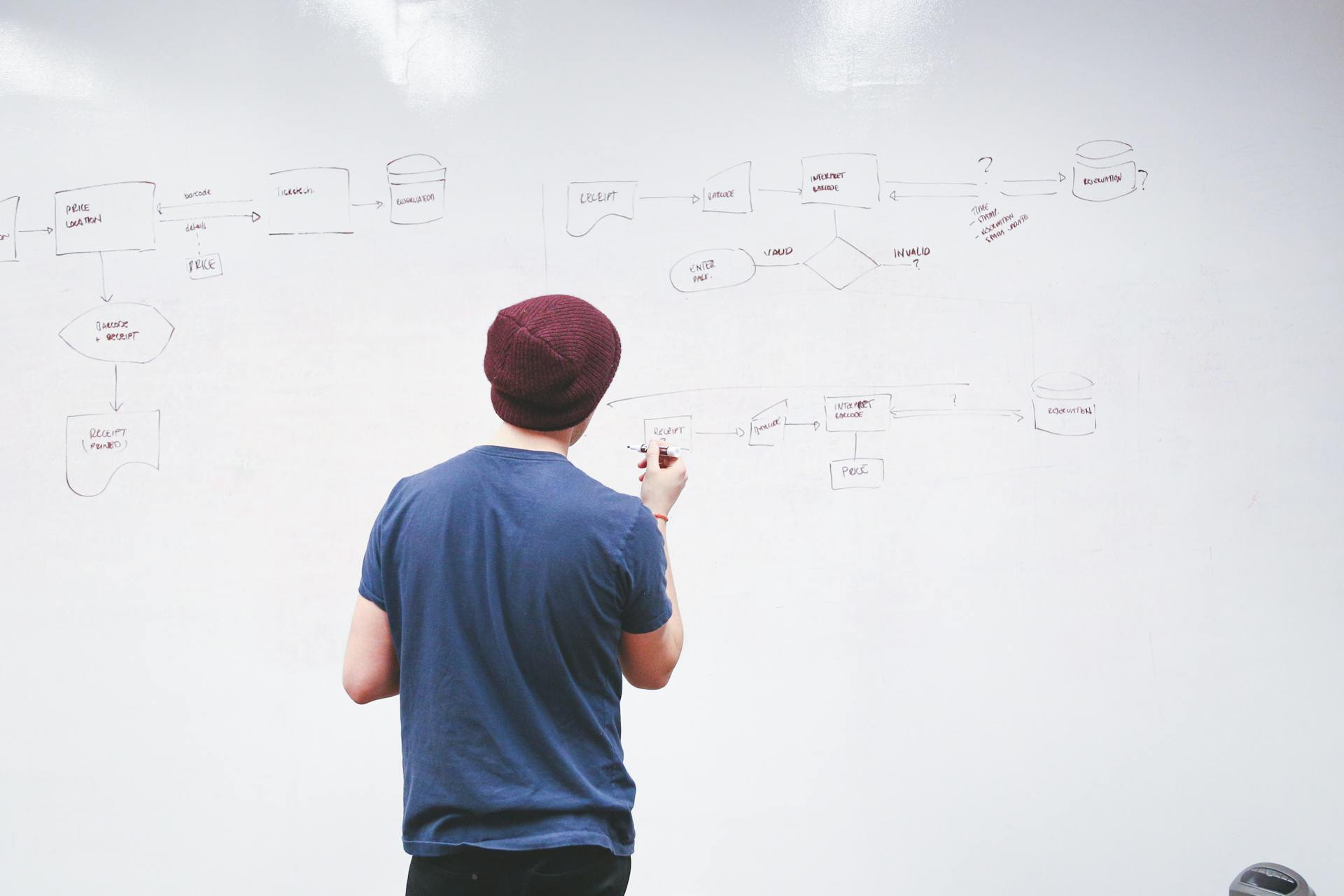
Django is a high-level Python web framework that follows the Model-View-Controller (MVC) architectural pattern.
The MVC pattern separates an application into three interconnected components: the model, view, and controller. This structure allows for a clean and maintainable codebase.
A model represents the data and its structure, while the view handles the presentation layer, rendering the data in a user-friendly format. The controller acts as an intermediary between the model and view, handling user input and updating the model accordingly.
This separation of concerns makes it easier to modify and extend the application without affecting other parts of the codebase.
Worth a look: Django Project Layout
What Is Django MVC?
Django MVC is an architectural/design pattern that separates an application into three main logical components: Model, View, and Controller. Each component is built to handle specific development aspects of an application.
The Model-View-Controller (MVC) framework is an industry-standard web development framework used to create scalable and extensible projects. It isolates the business logic and presentation layer from each other.
MVC was created by Trygve Reenskaug, with the main goal of solving the problem of users controlling a large and complex data set by splitting a large application into specific sections with their own purpose.
Intriguing read: Yii Framework Development Company
What Is?
Django MVC is a framework that separates an application into three main logical components: Model, View, and Controller.
The Model-View-Controller (MVC) framework is an architectural/design pattern that isolates the business logic and presentation layer from each other.
This design pattern was traditionally used for desktop graphical user interfaces (GUIs) but is now widely used in web development, including for creating scalable and extensible projects.
MVC was created by Trygve Reenskaug, who aimed to solve the problem of users controlling a large and complex data set by splitting a large application into specific sections that all have their own purpose.
In Django MVC, you define routes, which are URL patterns associated with different pages, and the application tries to match the entered URL to one of the predefined routes behind the scenes.
Controllers, models, views, and routes are the four major components at play in a web app built using Django MVC.
Consider reading: Twitter Bootstrap Components
Model-View-Controller Pattern in Python Web Development
The Model-View-Controller (MVC) pattern is a fundamental concept in web development, and it's the backbone of Django's architecture.
The MVC pattern breaks an application into three main components: the Model, which handles data, the View, which is what users see, and the Controller, which connects the two. This makes it easier to work on each part separately, so you can update or fix things without messing up the whole app.
The MVC pattern was created by Trygve Reenskaug, and its main goal was to solve the problem of users controlling a large and complex data set by splitting a large application into specific sections that all have their own purpose.
The MVC pattern is widely used in web development, including for designing mobile apps, and it's one of the most frequently used industry-standard web development frameworks to create scalable and extensible projects.
In Python web development, the MVC pattern is particularly useful because it allows developers to define routes, which are URL patterns associated with different pages. This way, when someone enters a URL, the application tries to match that URL to one of the predefined routes behind the scenes.
Related reading: Zend Framework Development
The MVC pattern also helps developers add new features smoothly, makes testing simpler, and allows for better user interfaces. It helps keep everything organized and improves the quality of the software.
The use of a web framework like Django provides out-of-the-box support to perform common operations in the process of web development, such as connecting your application to databases and handling tasks like session management.
Design Pattern Basics
The MVC design pattern is a fundamental concept in software development that separates an application into three distinct components: the Model, View, and Controller. This separation makes the application easier to maintain and extend.
The MVC pattern breaks an application into three parts: the Model (which handles data), the View (which is what users see), and the Controller (which connects the two). This separation allows developers to update or fix things without messing up the whole app.
Here are the key components of the MVC design pattern:
- The Model: handles data
- The View: what users see
- The Controller: connects the Model and View
What Is a Design Pattern?
A design pattern is a reusable solution to a common problem in software design. It's a tried-and-true approach that's been proven to work in various situations.
Design patterns help developers create more maintainable, flexible, and scalable code by separating concerns into distinct components. This separation of concerns makes it easier to modify one part of the code without affecting the rest.
A good design pattern should be easy to understand, implement, and reuse. It should also be flexible enough to adapt to changing requirements.
The MVC design pattern, for example, separates an application into three distinct components: data model, presentation information, and control information. This separation makes the application easier to maintain and extend.
Here's a breakdown of the MVC pattern's components:
- Data model: responsible for managing application data
- Presentation information: handles user interface and display
- Control information: manages application logic and user input
By using design patterns like MVC, developers can create more robust and maintainable software systems.
Design Pattern Purpose
The MVC Design Pattern is a fundamental concept in software development, and understanding its purpose is essential for any aspiring developer. The MVC pattern separates an application into three distinct components: the Model, View, and Controller.
This separation of concerns makes it easier to maintain and extend an application, as changes to one component do not require changes to the other components. The MVC pattern helps developers add new features smoothly, makes testing simpler, and allows for better user interfaces.
The main purpose of the MVC pattern is to keep everything organized and improve the quality of the software. It achieves this by breaking an application into three parts: the Model (which handles data), the View (which is what users see), and the Controller (which connects the two).
The MVC pattern helps developers adhere to several design principles, including dividing and conquering, increasing cohesion, reducing coupling, increasing reuse, and designing for flexibility.
Here are the five design principles that the MVC pattern helps developers adhere to:
- Divide and conquer: The three components can be somewhat independently designed.
- Increase cohesion: The components have stronger layer cohesion than if the view and controller were together in a single UI layer.
- Reduce coupling: The communication channels between the three components are minimal and easy to find.
- Increase reuse: The view and controller normally make extensive use of reusable components for various kinds of UI controls.
- Design for flexibility: It is usually quite easy to change the UI by changing the view, the controller, or both.
Django MVC Components
A Django application consists of the following components: URL dispatcher, View, Model, and Template.
The Model component is responsible for managing the application's data, processing business rules, and responding to requests for information from other components.
The Model interacts with the database and gives the required data back to the controller. It manages data through CRUD (Create, Read, Update, Delete) operations, enforces business rules, and notifies the View and Controller of state changes.
The Model is connected to the database, responds to controller requests, and talks to the database back and forth to give the needed data to the controller.
The different types of Lego blocks are the models. You have different sizes and shapes, and you grab the ones that you need to build the spaceship. In a web app, models help the controller retrieve all of the information needed from the database to make the products.
The Model layer is responsible for data definitions, its processing logic, and interaction with the backend database.
The View is the presentation layer of the application. It takes care of the placement and formatting of the result and sends it to the controller, which in turn, redirects it to the client as the application's response.
Data representation is done by the view component. It actually generates the UI or user interface for the user.
The View uses the data from the client as well as the model and renders its response in the form of a template.
Recommended read: Azure Data Factory Framework
The Controller acts as an intermediary between the Model and the View. It handles user input and updates the Model accordingly and updates the View to reflect changes in the Model.
The Controller doesn't have to worry about handling data logic, it just tells the model what to do. It processes all the business logic and incoming requests, manipulates data using the Model component, and interacts with the View to render the final output.
Here is a summary of the Django MVC components:
Frequently Asked Questions
Is Django based on MVT?
Yes, Django is based on the Model-View-Template (MVT) architecture, which separates application logic into three distinct components. This design pattern is a key feature of the Django framework.
What framework does Django use?
Django is built on top of Python, a high-level programming language. It's a Python web framework that simplifies web development.
Featured Images: pexels.com