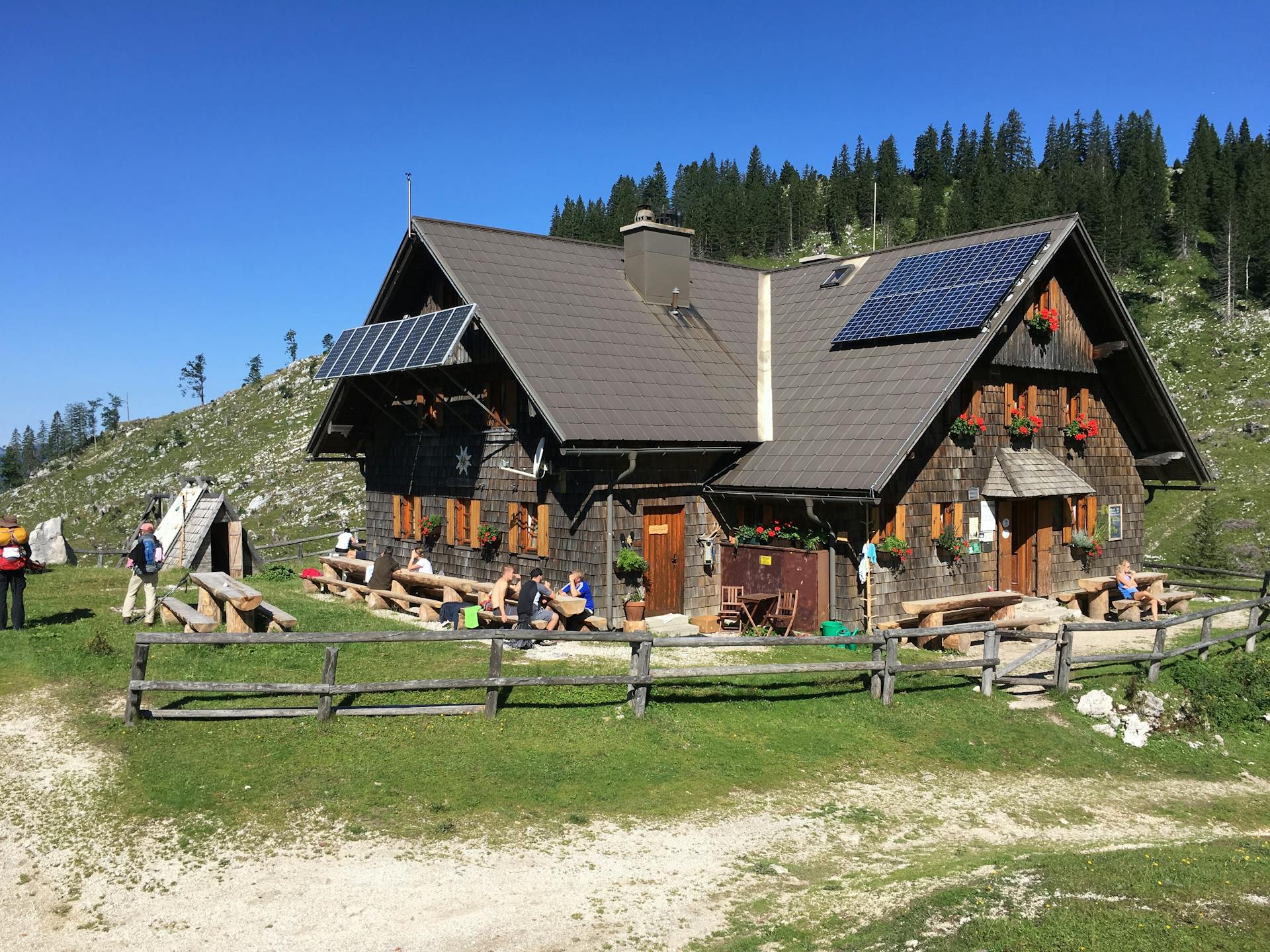
Go web programming is a great way to build scalable and concurrent systems.
Go's concurrency model is based on goroutines and channels, which allow for efficient and safe concurrent execution of code.
Go's simplicity and ease of use make it a great language for beginners to learn.
With Go, you can build fast and reliable web applications with a small codebase.
Go's standard library is comprehensive and includes many useful packages for web development, such as net/http and encoding/json.
You might like: Go Programming Language Web Framework
Getting Started
To get started with Go web programming, you'll need a machine running FreeBSD, Linux, macOS, or Windows. You can install Go on any of these operating systems.
To begin, make a new directory for your project inside your GOPATH and change into it. This is where you'll write your application code.
You'll need to import the fmt and os packages from the Go standard library to start building your web application.
Fast Development
Fast development is a key advantage of using Go frameworks.
You can start building your project quickly because packages are already available and can be imported easily, eliminating the need to write redundant code.
Managing dependencies in a large Go application can be done without additional tools, as long as the project isn't too big.
This means you can focus on writing code and building your project without getting bogged down in dependency management.
As long as the project isn't too big, managing dependencies is relatively simple, and you can try to build dependencies in Builders or Factories.
Explore further: Project Web Page Design
Start Simple
Starting with a simple project is a great way to get familiar with Go. You can try to build dependencies in Builders or Factories without needing additional tools. This approach is relatively simple for smaller projects.
The Go application can be managed without additional tools, as long as the project isn't too big. This is a great way to start, especially for beginners.
To get started, you need to have Go installed on your computer. You should also be vaguely familiar with its syntax and constructs.
For another approach, see: Rapid Web Application Development Tools
Go Web Programming Basics
Go web programming is a great field to get into, and it's relatively simple to manage dependencies in smaller projects. As long as the project isn't too big, you can try to build dependencies in Builders or Factories without needing additional tools.
To get started, you'll need to import the required packages, such as net/http, which provides a set of functions for building HTTP servers. The main function is where you'll initialize http using a handler function to handle incoming HTTP requests.
Here are the basic packages you'll need to import for Go web programming:
- net/http
- html/template
- regexp
These packages will help you build web applications, process HTML templates, and validate user input. With these basics in place, you'll be well on your way to creating your own Go web applications.
Consider reading: Developing Web Applications
Built-in Concurrency
Go's built-in concurrency features make it a breeze to write concurrent code. Goroutines are lightweight threads that allow you to run multiple tasks simultaneously.
Goroutines provide language-level support for concurrency. This means you don't need to worry about the low-level details of threads and synchronization.
Strict rules for avoiding mutation help prevent race conditions. By disallowing mutation, you can write concurrent code with confidence.
Overall, Go's concurrency features are incredibly simple to use. This simplicity is a major advantage when building concurrent systems.
Getting Started
To get started with Go web programming, you'll need a machine with FreeBSD, Linux, macOS, or Windows. You can install Go on your machine by following the installation instructions.
You'll need to create a new directory for your project inside your GOPATH and navigate to it using the command prompt. To do this, you'll need to create a new directory and navigate to it using the "cd" command.
You can import the fmt and os packages from the Go standard library to start building your project. Later, you'll add more packages to your import declaration as you implement additional functionality.
You can download the starter files for your project and view the contents of the repository using the "tree" command. The main files you'll need to work with are main.go, index.html, and assets/styles.css.
To manage dependencies in your project, you can use a DI container or build dependencies in Builders or Factories. For smaller projects, managing dependencies without additional tools is relatively simple.
Here are the basic requirements for getting started with Go web programming:
- A machine with FreeBSD, Linux, macOS, or Windows
- Go installed on your machine
- A new directory created for your project inside your GOPATH
- The fmt and os packages imported from the Go standard library
- The main files for your project downloaded and viewed using the "tree" command
By following these steps and meeting the basic requirements, you'll be well on your way to starting your Go web programming project.
Error Handling and Management
Error handling is crucial in Go web programming. Gin offers convenient error management, documenting errors as they occur during HTTP requests.
Ignoring errors is a bad practice, leading to unintended behavior when an error does occur. A better solution is to handle errors and return an error message to the user.
The http.Error function sends a specified HTTP response code and error message. This can be seen in the decision to handle errors in the renderTemplate function.
Any errors that occur during p.save() will be reported to the user. This is a key aspect of error handling in Go web programming.
Gin's simplicity and ease of use make it an ideal framework for Go web development, especially for those just starting out. Its minimal and straightforward approach helps developers focus on error handling and management.
Handling errors in a separate function can pay off in the long run. This approach allows for more efficient error reporting and user notification.
Creating and Serving Content
To create and serve content in Go web programming, you need to understand how to use the net/http package. This package is used to create a basic server that sends a "Hello World!" text to the browser when a GET request is made to the server root.
Discover more: C Programming Web Server
You can create a basic server by importing the net/http and os packages, and then using the http.NewServeMux() method to create an HTTP request multiplexer. This multiplexer matches the URL of incoming requests against a list of registered patterns, and calls the associated handler for the pattern whenever a match is found.
To serve static files, you need to instantiate a file server object and tell your router to use this file server object for all paths beginning with the /assets/ prefix. This is done using the http.FileServer() method, which returns an http.Handler type instead of a HandlerFunc. To use this handler, you need to use the Handle method instead of HandleFunc.
Broaden your view: Css in Html File
Serving Wiki Pages with Net/http
To serve wiki pages with net/http, you need to import the net/http package. Let's create a handler, viewHandler, that will allow users to view a wiki page. It will handle URLs prefixed with "/view/".
The viewHandler function extracts the page title from r.URL.Path, the path component of the request URL, by re-slicing it with [len("/view/"):]. This drops the leading "/view/" component of the request path.
To use this handler, we rewrite our main function to initialize http using the viewHandler to handle any requests under the path /view/. We also need to create some page data (as test.txt) and compile our code.
The http.HandleFunc method registers the handler, viewHandler, to handle requests to the path /view/. This allows us to serve wiki pages to users.
Paginating the Results
Paginating the results is a crucial feature for any search functionality. This allows users to navigate to the next or previous page of results at any time.
Since we're only displaying 20 results at a time, we need a way to handle pagination. To determine if the last page of results has been reached, we can create a method called IsLastPage.
This method checks if the NextPage field is greater than the TotalPages field on a Search instance. To make this work, we need to increment NextPage every time a new page of results is received. We can modify the searchHandler function to achieve this.
As users navigate through the results, we can add a button that allows them to go to the next page. This button should be rendered at the bottom of the results list, as long as the last page for that query has not been reached.
The href of the anchor tag for the Next button points to the /search route and retains the current search query in the q parameter while using the value of NextPage in the page parameter.
We can also add a Previous button that is only rendered if the current page is greater than 1. This is achieved by creating a new CurrentPage() method on Search, which simply returns NextPage - 1 unless NextPage is 1.
To get the previous page, we can subtract 1 from the current page. This method does just that, making it easy to render the Previous button only if the current page is greater than 1.
Intriguing read: Next Js Drizzle Supabase
Total Results
Displaying the total number of results is an essential feature of any search app.
You can show the total number of results at the top of the page by adding code as a child of .container in your index.html file.
The gt function is used to check if the TotalResults field of the Results struct is greater than zero.
This is a comparison function that helps determine whether to display the total number of results or a "No results found" message.
To try this out, type some gibberish into the search input so that no news items are found for your query.
You should see the "No results found" message on the screen, thanks to the comparison function.
Go ahead and make another search query on a popular topic, and the number of results will be outputted at the top of the page.
This is shown below: the total number of results will be printed at the top of the page.
Templating and Rendering
Templating and rendering are crucial aspects of Go web programming. To improve efficiency, you can use template caching by calling `ParseFiles` once at program initialization, parsing all templates into a single *Template.
You can create a global variable named `templates` and initialize it with `ParseFiles`. The `template.Must` function is a convenience wrapper that panics when passed a non-nil error value, and otherwise returns the *Template unaltered.
A better approach to rendering templates is to use the `ExecuteTemplate` method to render a specific template, passing the name of the template as an argument. This approach is more efficient than calling `ParseFiles` every time a page is rendered.
For another approach, see: Tailwind Css Templates Free
Editing Pages
Editing pages is a crucial part of any wiki, and Go makes it easy to implement.
To display an 'edit page' form, you'll need to create a new handler, which we've named editHandler. This function loads the page, or creates an empty Page struct if it doesn't exist, and then displays the HTML form.
All that hard-coded HTML is ugly, so there's a better way. You can use Go's templating features to create a template for the edit page form and use it across all pages of the site without duplicating the code.
In our example, we've added the editHandler to the main function and used it to load the page and display the HTML form. However, we'll need to create a template for the edit page form to make it look better.
For more insights, see: How to Use Inspect Element to Find Answers
Templating Basics
Templating Basics is the foundation of rendering dynamic HTML pages in Go.
You can use the text/template or html/template packages in Go to create templates. The html/template package is safer against code injection.
To get started, import the html/template package in your main.go file and use it to parse and execute templates.
A template provides an easy way to customize the output of your web application without duplicating code. You can create a template for the navigation bar and use it across all pages of the site.
See what others are reading: Building Web Templates
The template.Must function is used to wrap the template.ParseFiles function to panic if an error is obtained while parsing the template file. This is because a web app with a broken template is not much of a web app.
You can execute a template by providing two arguments: where you want to write the output to, and the data you want to pass to the template.
The dot operator is used to access a struct inside a template. This operator refers to the entire struct object, and you can access field names by specifying the field name after the dot.
A range block allows you to iterate over a slice in Go and output some HTML for each item in the slice. The dot inside the range block refers to each item in the slice, and you can access fields like Title, Description, etc.
To render a template, you can use the template.ParseFiles function to parse the template file, and then use the tmpl.Execute function to render the template and write the result to the http.ResponseWriter interface.
A template engine separates the HTML code from the Go code, making it easier to maintain and update your web pages. Go has a built-in template engine in the html/template package.
You can use the html/template package to render dynamic HTML pages by parsing the template file and executing the template with the provided data.
Here's an interesting read: Data Text Html Charset Utf 8 Base64
API and News Client
To create an API client for the News API, you'll need to create a news folder at the root of your project directory and a news.go file within it. The Client struct represents the client for working with the News API, with fields for the HTTP client, API key, and page size.
The Client struct has a NewClient() function that creates and returns a new Client instance for making requests to the News API. You can retrieve the News API key from the environment and create a new Client instance in your main.go file. To access the newsapi client from the searchHandler, you can either make it a package-level variable or use a closure to access it.
The News API provides two main endpoints for retrieving news items, and you can make requests to the /everything endpoint to get a JSON response. To work with this data in Go, you'll need to generate a struct that mirrors the JSON data, with fields for each property and tags to specify the JSON property.
Suggestion: Web Programming News
News API Client
To create a News API client, you'll first need to create a news folder at the root of your project directory. This will serve as the base for your API client.
You'll then create a news.go file within the news directory, where you'll define the Client struct that represents the client for working with the News API.
The Client struct has three fields: httpClient, apiKey, and PageSize. The httpClient field points to the HTTP client that should be used to make requests, while the apiKey field holds the API key and the PageSize field holds the number of results to return per page.
You'll also create a NewClient() function that creates and returns a new Client instance for making requests to the News API. The API key should be retrieved from the environment and used to create a new Client instance in your main.go file.
To make requests to the News API, you'll need to create a new method on the Client struct called FetchEverything(). This method will accept two arguments: the search query and the page number. The search query will be URL encoded using the QueryEscape() method.
The FetchEverything() method will make an HTTP request to the News API using the custom HTTP client, which has been set to timeout after 10 seconds. The response from the News API will be checked to ensure it's 200 OK. If not, a generic error will be returned.
For more insights, see: Html File
Check the Popularity
Checking the popularity of a tool can be a great way to narrow down your options. It's a good indicator of how actively maintained and supported a project is.
Looking at the number of stars on Github can give you an idea of a tool's popularity. For example, the most popular Golang framework has 32k stars. This can be a good benchmark for what constitutes a popular tool.
If a tool has fewer than 6k stars, it may not be very popular, which can make it harder to find a team of programmers or community support.
Let's take a look at the most popular Go frameworks. Here are the top 4 tools with more than 6k stars:
These tools have a significant following and are likely to have active communities and good support.
Frequently Asked Questions
Is Golang frontend or backend?
Golang is primarily used for backend development, where it excels due to its efficient compilation and execution. It's the top choice for building scalable and high-performance backend applications.
Is Go language easier than Python?
Go is generally considered a simpler language than Python, due to its more specialized purpose and fewer complexities. However, both languages are still relatively easy to learn for beginners.
Featured Images: pexels.com