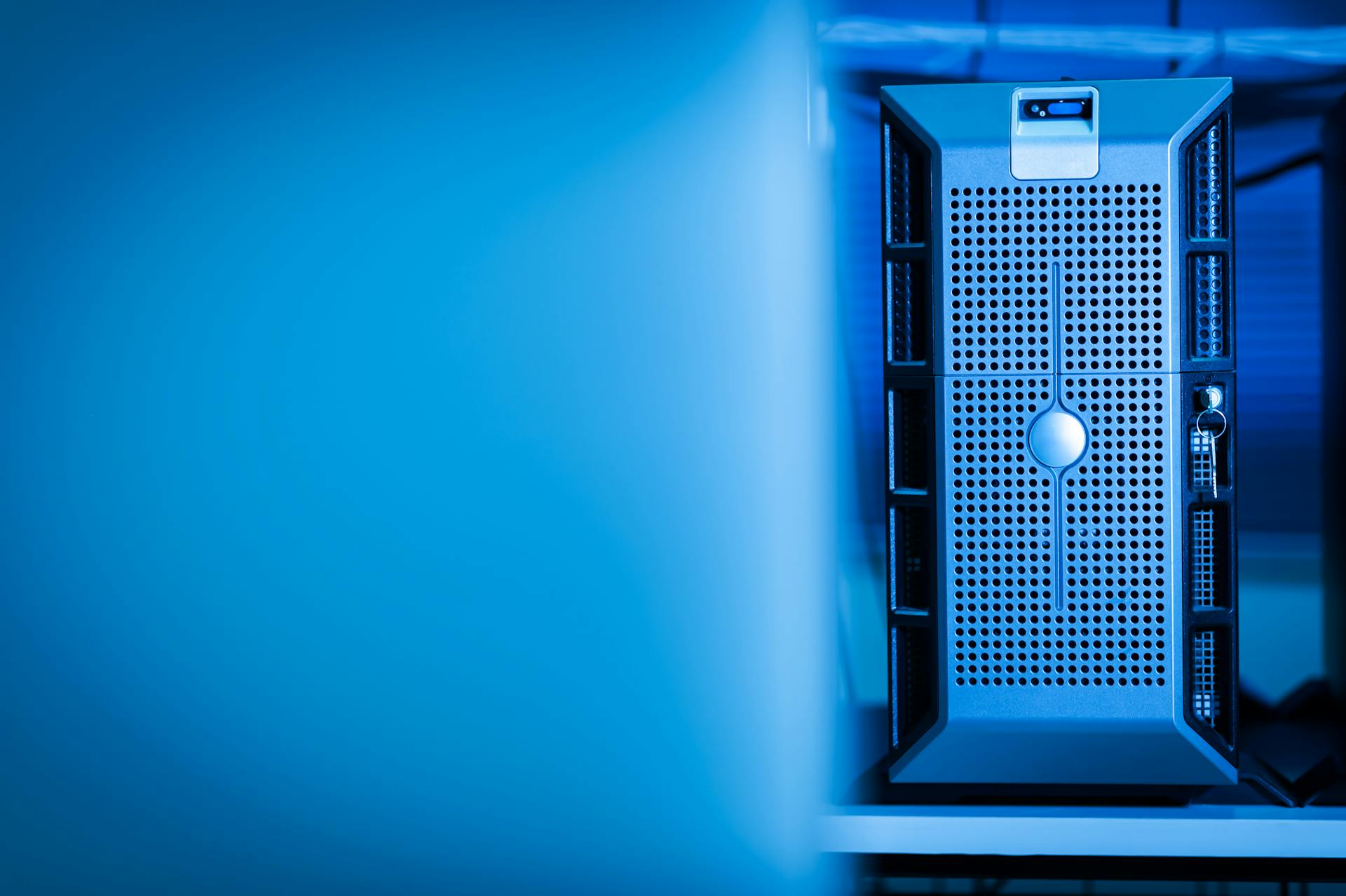
Integrating Google Cloud Storage with your Golang application is a game-changer for data storage and management.
You can use the Google Cloud Storage client library for Go to interact with Cloud Storage buckets, enabling you to upload, download, and manage files with ease.
The client library provides a simple and intuitive API that makes it easy to integrate Cloud Storage into your Golang application.
Authentication and Authorization
Authentication and authorization are crucial steps in using Google Cloud Platform with Golang. Google Application Default Credentials (ADC) is the recommended way to authorize and authenticate clients, which can be obtained from https://cloud.google.com/docs/authentication/production.
You can use Google Application Default Credentials without passing extra information to the client libraries if your environment is configured correctly. This is a convenient way to get started with authentication and authorization.
In some cases, you might want to use a JSON key file for a Service Account instead of ADC. Service Account keys can be created and downloaded from https://console.cloud.google.com/iam-admin/serviceaccounts.
You can also create credentials from in-memory JSON and use the WithCredentials option if you don't want to store secrets on disk. This is a useful option when you need more control over authorization.
By default, each API will use Google Application Default Credentials for authorization credentials used in calling the API endpoints. This allows your application to run in many environments without requiring explicit configuration.
You can exert more control over authorization by using the credentials package to create an auth.Credentials. Then pass option.WithAuthCredentials to the NewClient function for more control.
Client Configuration
To create a Google Cloud client, you need to authenticate using a service account key. This key is downloaded as a JSON file from the Google Cloud console, and you'll need to export the path to this file as an environment variable named GOOGLE_APPLICATION_CREDENTIALS.
In the terminal, you'll run a command like export GOOGLE_APPLICATION_CREDENTIALS=/path/to/sa-json to set the environment variable. The storage library will automatically read the key file if it exists, or throw an error if it doesn't.
Declaring Dependencies
To declare dependencies for your API, you'll need to initialize a mod.go file in your project directory with go mod init. This will set up your project to use go modules for defining application dependencies.
You'll then use go get to fetch the dependencies your API needs. This command will download the necessary libraries and store them in your project directory.
Cloud Functions uses go modules to define application dependencies, and it's essential to note that upon deployment, Cloud Functions uploads your application code to a build server, which then fetches dependencies remotely. This means you should not rely on any local dependencies that won't be accessible by the Cloud Functions build server.
New Client
Creating a new client is a crucial step in working with the Google Cloud Client Libraries for Go. You can create a new client using the `NewClient` function, which creates a new storage client based on gRPC.
The returned client must be closed when it's done being used to clean up its underlying connections. This is important to avoid any potential issues.
To create a new client, you'll need to follow the instructions provided by the library. Specifically, you'll need to use the `NewClient` function, which is described in the documentation.
Here's a quick rundown of the `NewClient` function:
By following these steps and using the `NewClient` function, you'll be able to create a new client that you can use to interact with the Cloud Storage API. Just remember to close the client when you're done using it to avoid any issues.
API Endpoints
API Endpoints are a crucial part of Google Cloud Platform's Golang implementation. You can configure the endpoint to specify the URL to which requests are sent, which is useful for services that support regional endpoints or for testing against fake servers.
Google Cloud services respect system parameters that can be used to augment request and/or response behavior, but they are not needed when using one of the enclosed client libraries. These parameters are made available via the callctx package.
To implement an API, you can create a handler function that takes a request, performs the necessary actions, and returns a JSON-encoded response. For example, the Sunset/Sunrise API implementation takes a request with query parameters, performs a sunrise/sunset lookup, and returns a JSON-encoded response.
Supported APIs
Our API endpoints are designed to be flexible and adaptable, and we've made sure to support the latest versions of the Go programming language.
For an updated list of all our released APIs, be sure to check our reference docs.
We follow the same policy as the Go programming language, supporting the two most-recent major Go releases as of January 1, 2025.
Our libraries are compatible with at least the three most recent, major Go releases.
Here's a breakdown of the specific Go versions we currently support:
- Go 1.23
- Go 1.22
- Go 1.21
Createbucket
Creating a bucket is a straightforward process, and we have two endpoints to help us achieve this: DeleteBucket and UpdateBucket. The DeleteBucket endpoint can be used to delete an empty bucket, but it's worth noting that it permanently deletes the bucket.
You can use the UpdateBucket endpoint to update a bucket, and this is equivalent to using the storage.buckets.patch method from the JSON API. This endpoint is available since version inv1.19.0, which is a significant update that brings new features and improvements.
GetBucket
The GetBucket API endpoint is a simple yet powerful tool for retrieving metadata about a specific bucket. It was added in version 1.19.0.
To use GetBucket, you'll need to call the function on a Client object, passing in the name of the bucket you're interested in. This will return metadata for the specified bucket.
GetBucket is a basic but essential endpoint for working with buckets, and it's a great place to start when building your app.
GetIamPolicy
The GetIamPolicy endpoint is a crucial tool for managing access to your buckets. It allows you to get the IAM policy for a specified bucket.
The resource field in the request should be projects/_/buckets/{bucket}. This is a specific requirement for the endpoint to function correctly.
To use the GetIamPolicy endpoint, you need to specify the bucket you're interested in. This can be done by including the bucket name in the resource field of the request.
The GetIamPolicy endpoint was added in version 1.19.0. This indicates that it's a relatively new feature, but one that's already quite useful.
You can use the GetIamPolicy endpoint to retrieve the IAM policy for a bucket, which can help you understand who has access to your data and what permissions they have.
Lock Bucket Retention Policy
Locking the retention policy on a bucket is a key feature of the API. It's available since inv1.19.0.
You can use the LockBucketRetentionPolicy function to achieve this, which is part of the Client API. It's a simple yet powerful tool for managing your buckets.
The LockBucketRetentionPolicy function locks the retention policy on a bucket, ensuring that it cannot be changed accidentally or maliciously. This adds an extra layer of security to your data.
This feature is a must-have for any serious API user, especially those working with sensitive data.
RestoreObject
The RestoreObject API endpoint allows you to restore a deleted object from the database.
To use this endpoint, you'll need to provide the ID of the deleted object, which is a unique identifier assigned to each object in the system.
This endpoint is particularly useful for recovering accidentally deleted data, and it's a lifesaver when you need to retrieve a deleted object quickly.
The RestoreObject endpoint returns the restored object in its original state, with all its attributes and relationships intact.
In the event of a restore failure, the endpoint returns a detailed error message indicating the cause of the failure.
WriteObject
WriteObject is a method that stores a new object and its metadata. It can be used in two ways: writing in a single message stream or in a resumable sequence of message streams.
To write using a single stream, the client must include a WriteObjectSpec in the first message describing the destination bucket, object, and any preconditions. The final message must set 'finish_write' to true.
If the stream is closed before finishing the upload, the client should attach the returned upload_id to the first message of each following call to WriteObject. The service will not view the object as complete until the client has sent a WriteObjectRequest with finish_write set to true.
Sending any requests on a stream after sending a request with finish_write set to true will cause an error. The client should check the response it receives to determine how much data the service was able to commit and whether the service views the object as complete.
Attempting to resume an already finalized object will result in an OK status, with a WriteObjectResponse containing the finalized object’s metadata.
Headers
Headers are set in the same way for all transports using `callctx.SetHeaders`.
You can set headers in a generic way, but be aware that some headers are reserved for internal use.
Headers like `x-goog-api-client` and `x-goog-request-params` should not be overwritten unless instructed to do so.
Check the individual package documentation for service-specific reserved headers.
Storage, for example, supports a specific auditing header mentioned in its documentation.
API Operations
API operations on Google Cloud Platform can be easily implemented using Go. You can write a function to take a request, perform a sunrise/sunset lookup, and return a JSON-encoded response using the http.Request object.
To test permissions, you can use the TestIamPermissions function, which tests a set of permissions on a given resource, such as a bucket or object, to see which permissions are held by the caller.
The NewClient function creates a new storage client based on gRPC, which must be closed when it's done being used to clean up its underlying connections.
Endpoint Override
Endpoint Override is a crucial aspect of API operations. It allows you to specify the URL to which requests are sent, which is particularly useful for services that support or require regional endpoints.
You can configure the endpoint to the location with the features you want that is closest to your physical location or the location of your users. This is recommended for services like Vertex AI, which has no global endpoint.
Google Cloud services respect system parameters that can be used to augment request and/or response behavior. However, they are not needed when using one of the enclosed client libraries.
DeleteObject
DeleteObject is a permanent action when versioning is disabled or the generation parameter is used. Deletions are irreversible in these cases.
However, if soft delete is enabled for the bucket, deleted objects can be restored using RestoreObject until the soft delete retention period has passed. This gives you a chance to recover deleted files before they're gone for good.
The generation parameter is crucial in ensuring permanent deletions. By including it in your request, you can guarantee that the object and its metadata will be deleted without the possibility of recovery.
Soft delete retention period limits the time you have to restore deleted objects. Once this period has passed, the objects are permanently deleted and cannot be recovered.
GetObject
GetObject is a fundamental operation in API design, allowing clients to retrieve specific resources from the server. It's a crucial part of the CRUD (Create, Read, Update, Delete) operations.
A GetObject request typically involves specifying the resource ID or key, which uniquely identifies the resource on the server. This ID is usually a string or a numeric value.
For example, if you're using a RESTful API, the GetObject request might look like this: GET /users/123, where 123 is the unique ID of the user resource. The server would then respond with the user data in a standard format, such as JSON.
In a real-world scenario, a client might make a GetObject request to retrieve a user's profile information, including their name, email address, and other relevant details. This would be a simple way to fetch the required data without having to send a full GET request for all users.
ListObjects
The ListObjects method is a convenient way to retrieve a list of objects from Cloud Storage. It's available in the inv1.19.0 version of the client.
To use ListObjects, you need to have a client set up, which can be created from Google Cloud's Storage library. This involves authenticating with a service account that has editor permissions on your GCS bucket.
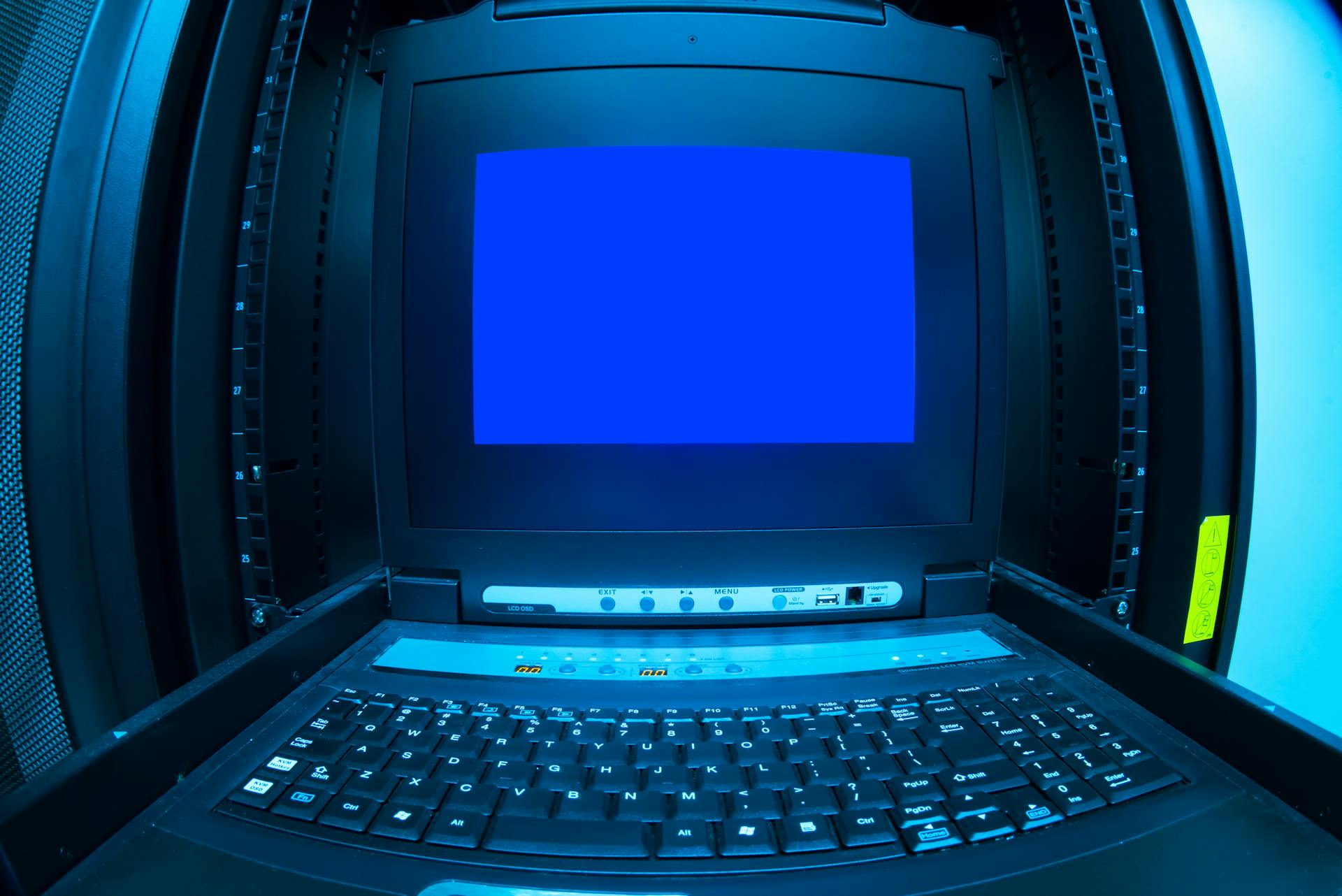
The ListObjects method can be called concurrently with other methods, but you should avoid modifying fields while making method calls. This is a safety precaution to prevent data inconsistencies.
You can authenticate with a service account by creating one in Google Cloud and downloading a JSON key file. Then, you can export the key file's path as an environment variable, which the storage library will automatically read.
RewriteObject
The RewriteObject operation is a powerful tool for modifying existing objects in your system. It allows you to rewrite a source object to a destination object, optionally overriding metadata.
This operation is particularly useful when you need to update an object's metadata without altering its underlying data. With RewriteObject, you can specify new metadata and the service will update the object accordingly.
RewriteObject is available in version 1.19.0 and later, making it a relatively new addition to the API.
StartResumableWrite
The StartResumableWrite operation is a crucial part of API operations, allowing you to start a resumable write.
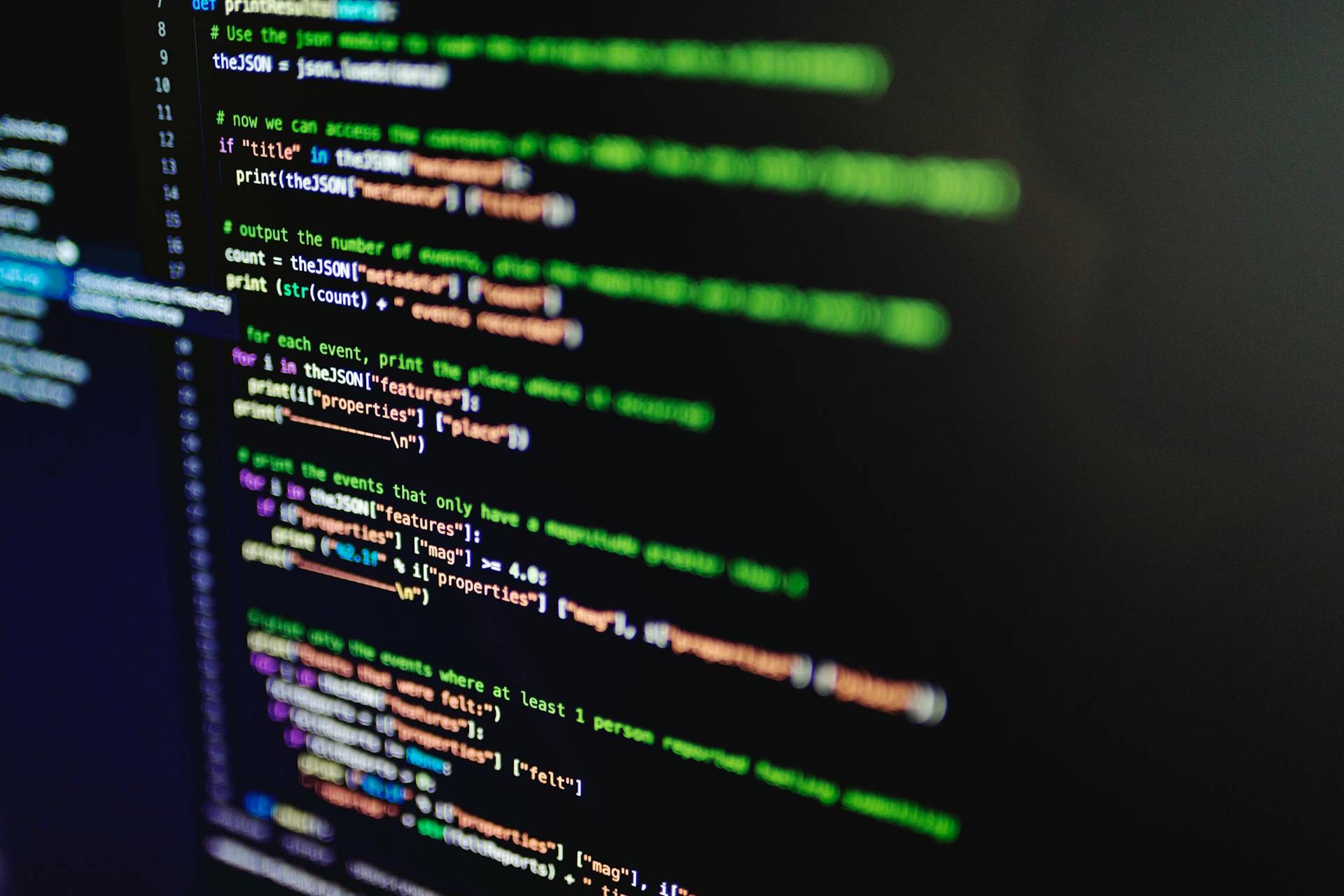
This operation is service-dependent, meaning its behavior varies depending on the specific service you're using.
The duration for which the write operation remains valid is also service-dependent, so be sure to check your service's documentation for more information.
You'll need to check the service's documentation to understand what happens when the write operation becomes invalid.
The StartResumableWrite operation is a powerful tool for managing API operations, but it requires careful consideration of the service's specific requirements.
TestIamPermissions
TestIamPermissions is a crucial API operation that allows you to test a set of permissions on a specific resource.
The TestIamPermissions method is added in version inv1.19.0, which means it's a relatively new feature that you can take advantage of.
To use TestIamPermissions, you need to specify the resource field in the request, which depends on the type of resource you're testing. For a bucket, it's projects/_/buckets/{bucket}.
UpdateObject
The UpdateObject method is a powerful tool in the Cloud Storage API. It updates an object's metadata, which is equivalent to using the JSON API's storage.objects.patch method.
This method is particularly useful for making quick changes to your object's metadata without having to rewrite the entire object. It's also a good option when you need to update multiple objects at once.
The UpdateObject method can be called concurrently with other methods, but you should avoid modifying fields concurrently with method calls to avoid any potential issues.
In terms of when to use UpdateObject, it's a good choice when you need to update metadata only. If you need to make more extensive changes, you may want to consider using the RewriteObject method instead.
Non-HTTP Triggers
API Operations don't have to be limited to HTTP triggers. Cloud Functions can be called in response to other events in the cloud.
One such event is Cloud Storage triggers, which can watch a storage bucket for new video files. This can be useful for re-encoding new files into a separate bucket.
Cloud Pub/Sub Triggers are another type of non-HTTP trigger. You can publish data to a Cloud Pub/Sub topic and have a Cloud Function do some transformation on that data.
Here are some examples of non-HTTP triggers:
- Cloud Storage triggers: For example, re-encoding new video files into a separate bucket.
- Cloud Pub/Sub Triggers: For example, creating a data ingest pipeline by publishing data to a Cloud Pub/Sub topic and having a Cloud Function do some transformation on that data.
Call Options
Call Options are a crucial part of API Operations, and they're used to specify the retry settings for each method of a Client.
The CallOptions contain the retry settings, which are essential for handling failed requests and ensuring the stability of your API operations.
In particular, CallOptions are used to determine how many times a method should be retried before giving up, and what the delay should be between retries.
This helps to prevent a single failed request from causing a cascade of errors and ensures that your API remains responsive and reliable.
By specifying the retry settings, you can customize the behavior of your API to suit your specific needs and requirements.
ComposeObject
ComposeObject is a powerful API operation that allows you to concatenate a list of existing objects into a new object in the same bucket.
It was added in version inv1.19.0, which means you can use this feature if your API is updated to this version or later.
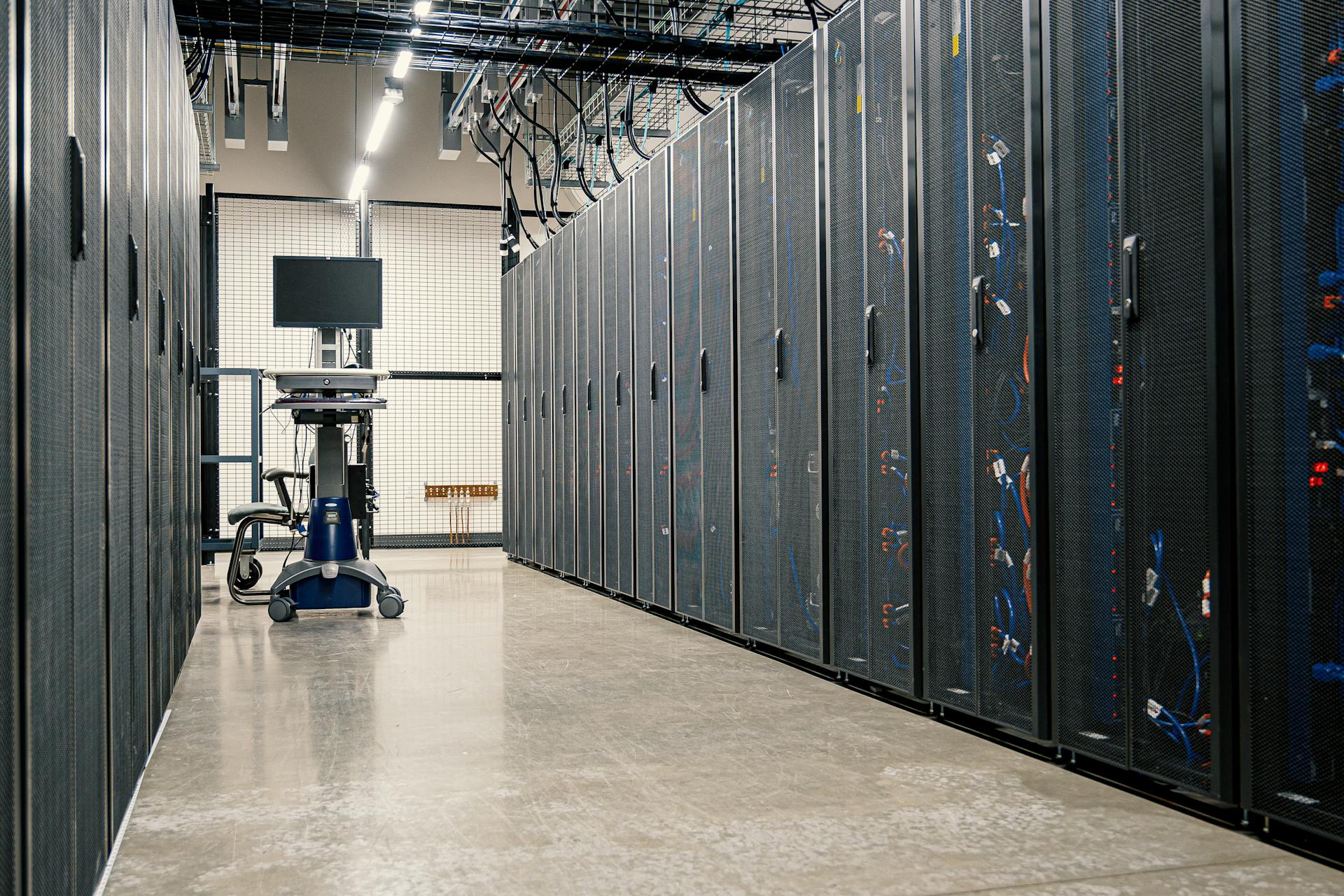
This operation is useful when you need to combine multiple objects into a single object, making it a great tool for data aggregation and processing.
As an example, you can use ComposeObject to merge multiple images into a single image, or to combine multiple text files into a single document.
Connection Deprecated
The Connection Deprecated method is a thing of the past. It's been replaced by a more efficient pooled connection system.
This method used to return a connection to the API service, but that's no longer the case. Connections are now pooled, which means they're not always the same resource.
As a result, you can no longer rely on getting the same connection every time you use the Connection method. This change might require some adjustments to your code.
Client QueryWriteStatus
The Client QueryWriteStatus method is a powerful tool in the API operations toolkit. It determines the persisted_size for an object that is being written, which can then be used as the write_offset for the next Write() call.
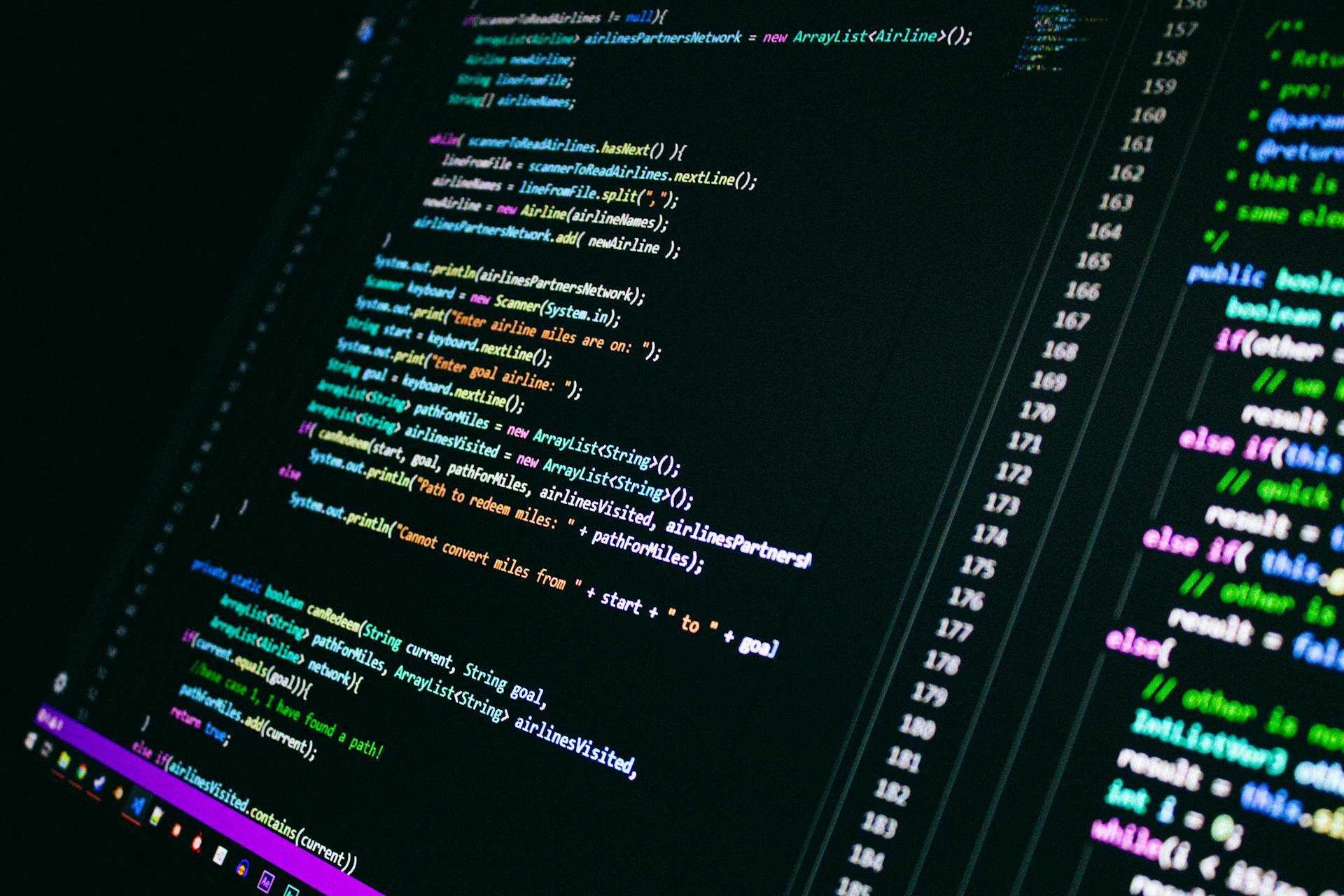
This method is particularly useful if you're buffering data and need to know which data can be safely evicted. For instance, if you're writing a large file in chunks, you can use QueryWriteStatus() to see how much data has been processed so far.
The client may call QueryWriteStatus() at any time to determine the persisted_size for an object. This information can be used to optimize data processing and reduce the risk of data loss.
If the object does not exist, QueryWriteStatus() returns the error NOT_FOUND. This can happen if the object has been deleted or the first Write() has not yet reached the service.
The sequence of returned persisted_size values will always be non-decreasing for any sequence of QueryWriteStatus() calls for a given object name. This means you can rely on the information provided by QueryWriteStatus() to make informed decisions about your data processing.
Sources
- https://pkg.go.dev/cloud.google.com/go
- https://maddevs.io/writeups/free-go-project-hosting-with-google-cloud/
- https://benjamincongdon.me/blog/2019/01/21/Getting-Started-with-Golang-Google-Cloud-Functions/
- https://j18e.medium.com/using-google-cloud-storage-with-go-b5baaa5ca142
- https://pkg.go.dev/cloud.google.com/go/storage/internal/apiv2
Featured Images: pexels.com