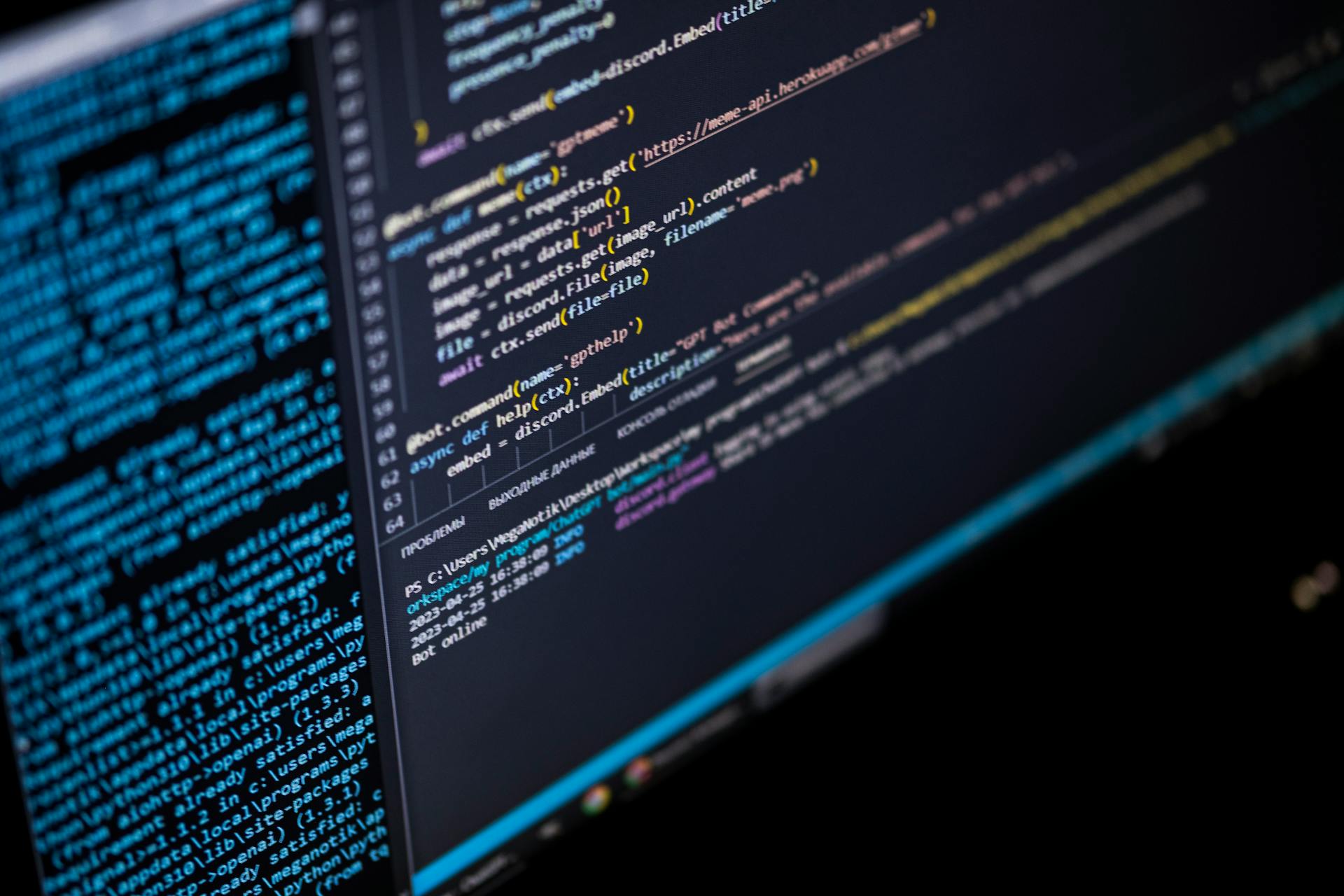
If you're working on a Next.js project and encounter the error "Module not found: Can't resolve 'fs'", don't worry, it's a common issue.
The 'fs' module is a built-in Node.js module that provides a way to interact with the file system. However, in a Next.js project, this module is not included by default.
This is because Next.js uses a custom build process to optimize the code, which can sometimes cause issues with built-in modules.
To fix this issue, you need to make sure that the 'fs' module is properly installed and configured in your project.
Worth a look: Next Js Localstorage Is Not Defined
Error in Nextjs
The "Module not found: Can't resolve 'fs'" error in Nextjs is a common issue that occurs when trying to use Node.js modules that are not available in the browser.
This error usually means that the code is trying to use the client-side Node.js fs module, which is not accessible through browsers.
The fs module is unique to Node.js environments and poses an issue when used in browser code.
Readers also liked: Next Js Document Is Not Defined
Nextjs makes a distinction between code on the client and server sides, and the "use client" directive shows that the code must run in the browser.
The syntax of the error is as follows: "Module not found: Can't resolve 'fs'".
To fix this error, you can check import locations and make sure fs and other Node.js modules are only present in server-side code.
Verify usage by ensuring fs and other Node.js modules are only used in server-side code like getServerSideProps, getStaticProps, or API routes.
Avoid using Node.js modules in React components that run on the client-side.
You can use dynamic imports or conditional logic to ensure Node.js modules are only imported when running server-side.
Nextjs data fetching methods like getServerSideProps or getStaticProps can be used to handle server-side operations.
It's essential to check third-party libraries and ensure they are compatible with both server and client environments.
Some libraries may have specific instructions for client-side usage.
You can move fs operations to API routes, where they are executed server-side, and then fetch data from these routes in client-side components.
Custom Webpack settings should be adjusted to exclude Node.js modules for client-side builds.
Browser-compatible alternatives or libraries can be used for some file operations.
Broaden your view: Can Nextjs Be Used on Traditional Web Application
Possible Causes
The "module not found can't resolve 'fs'" error in Next.js can be frustrating, but it's often caused by something simple.
The fs module is a Node.js core module used for file system operations and is not available in the browser environment.
This issue can arise from importing fs directly or indirectly in components or libraries intended to run in the browser.
If you're using Next.js, you might be trying to import fs in a component meant to run client-side, which can cause this error.
API routes can also be a culprit if they're using fs but client-side is calling them directly rather than server-side.
Custom Webpack configurations in Next.js can fail to exclude Node.js modules for client-side builds, resulting in this error.
Conditional imports can also be a problem, especially if you're trying to use fs in a way that's not compatible with the browser environment.
See what others are reading: React Next Js Err_require_esm
Error Fix
The "Module not found: Can't resolve 'fs'" error in Next.js is a common issue that can be fixed by understanding the distinction between server-side and client-side code.
Make sure to only use Node.js modules like fs in server-side code, such as getServerSideProps, getStaticProps, or API routes, where they are executed server-side.
You can safely use fs within getStaticProps or getServerSideProps in modern versions of Next.js (9.4+) without extra configuration, thanks to a custom Babel plugin that tree-shakes away unused dependencies.
You might enjoy: Nextjs Can Layouts Be Server Components
Install Missing Dependencies
If you're seeing an error message about missing dependencies, it's likely because a required library or module is not installed.
First, check the error message for specific dependency names, as seen in the "Error Messages" section. These names will guide you in installing the right dependencies.
To install missing dependencies, you'll need to use a package manager like pip, which is used for Python packages, or npm for Node.js packages, as mentioned in the "Package Managers" section.
Start by opening a terminal or command prompt and navigating to the project directory, as described in the "Project Navigation" section.
Next, run the installation command, such as `pip install requests` or `npm install express`, using the specific dependency name from the error message.
Update Module Imports
In Nextjs apps, fs and other Node.js modules should only be present in server-side code like getServerSideProps, getStaticProps, or API routes.
To avoid using Node.js modules in client-side components, you can use dynamic imports or conditional logic to ensure they're only imported when running server-side.
Nextjs data fetching methods like getServerSideProps or getStaticProps can handle server-side operations.
Make sure any third-party libraries used are compatible with both server and client environments or have specific instructions for client-side usage.
If you have custom Webpack configurations, ensure Node.js modules are excluded for client-side builds.
For some file operations, consider using browser-compatible alternatives or libraries that don't rely on Node.js-specific APIs.
Things to Remember
To avoid issues with "Module not found: Can't resolve 'fs'", it's essential to understand the difference between server-side and client-side code in Next.js. Server-side code can include Node.js modules like fs, but client-side code should not.
Ensure that file system operations (fs) are only executed on the server-side using Next.js features like getServerSideProps, getStaticProps, or API routes. This will prevent errors from occurring.
Use environment checks to conditionally load Node.js modules only on the server. This ensures server-only modules are not bundled for the client, avoiding errors.
Use Next.js data-fetching methods to perform server-side operations and pass data as props to components. This keeps the server-side logic isolated and separate from UI components.
API routes are the best place to move any fs operations or similar server-side tasks. These can be called from client-side components, ensuring server-side code runs in the correct environment.
When using third-party libraries, ensure they are compatible with both server-side and client-side environments. Some libraries are specific for Node.js and will not work on the client-side.
Solving the Error
The Module not found: Can't resolve 'fs' error occurs when you try to import a module that's available on the server-side but not in the browser.
This error usually happens when you're trying to use the fs module, which is unique to Node.js environments, in a file or component meant for use in a browser.
The good news is that you can safely use fs within getStaticProps or getServerSideProps in modern versions of Next.js (9.4+), without any extra configuration required.
These functions are specifically designed for static generation and server-side rendering, and Next.js' custom Babel plugin correctly removes unused dependencies from the bundle.
Tree shaking eliminates unused functions from across the bundle, and in the case of Next.js, it's extended to remove dependencies and functions used in getStaticProps or getServerSideProps files.
You can refer to a server-side module in these functions, and Next.js will take care of the rest.
Make sure to verify usage and avoid client-side components to prevent the Module not found: Can't resolve 'fs' error from occurring.
Dynamic imports or conditional logic can also help ensure that Node.js modules are only imported when running server-side.
The Problem
You'll get an error if you run this code and access an existing "/example-route" route. This is because Next.js middleware only supports the Edge Runtime currently.
Curious to learn more? Check out: Nextjs Route
The Edge Runtime is based on standard Web APIs, it does not support native Node.js APIs like the file system API. You can see a list of available APIs in the Vercel Edge Runtime API docs.
You'll need to use the Node.js runtime to use the Node.js file system module, but this means you won't be able to access it in a middleware function.
A fresh viewpoint: Can Amazon S3 Take in Nextjs File
Third Party
The 'fs' module is a built-in Node.js module, but in some cases, Next.js might not be able to resolve it due to third-party dependencies.
If you're using a third-party package that imports 'fs', it could cause issues with Next.js. For example, the 'sharp' package imports 'fs', which can lead to the "module not found" error.
To resolve this, you can try using the 'fs-extra' package instead, which is a drop-in replacement for the built-in 'fs' module.
Common Pitfalls
One of the biggest mistakes people make when working with third-party companies is not doing their due diligence on the vendor's security practices. This can leave sensitive data vulnerable to breaches.
Not having a clear contract or agreement in place can lead to misunderstandings and disputes down the line.
Third-party vendors can have varying levels of access to your system, which can be a major security risk if not managed properly. For example, a vendor may have access to sensitive customer data.
Failing to regularly review and update contracts can leave you locked into outdated agreements that don't meet your current needs. This can be costly and time-consuming to rectify.
Not having a plan in place for vendor termination can leave you stuck with a vendor that's no longer meeting your needs. This can be especially true if the vendor has access to sensitive data or systems.
Here's an interesting read: Google Drive Can't Access Large Files
External Libraries
External libraries are a crucial part of any third-party integration, and they can greatly impact the success of your project.
Many developers are familiar with popular libraries like jQuery, which can simplify tasks such as DOM manipulation and event handling.
However, it's essential to note that not all libraries are created equal, and some may have compatibility issues or security concerns.
Some libraries, like Lodash, offer a wide range of utility functions that can save you a significant amount of time and effort.
When choosing an external library, consider factors such as performance, documentation, and community support.
Package Managers
Package managers play a crucial role in managing dependencies for third-party software. They help keep track of the required libraries and tools, making it easier to install, update, and remove them.
The most popular package managers include npm for JavaScript, pip for Python, and Maven for Java. They provide a standardized way to manage dependencies, which is essential for maintaining a consistent and reliable environment.
A good package manager can save you a lot of time and effort in the long run. It helps prevent dependency conflicts and ensures that your software is up-to-date with the latest security patches.
Some package managers, like npm, have a large community of developers who contribute to and maintain the packages. This leads to a vast collection of packages available for use, making it easier to find the right one for your project.
However, package managers can also introduce security risks if not used properly. For example, if a package is not properly vetted, it can contain malicious code that can compromise your system.
Sources
- https://bobcares.com/blog/module-not-found-cant-resolve-fs-nextjs/
- https://maikelveen.com/blog/how-to-solve-module-not-found-cant-resolve-fs-in-nextjs
- https://blog.logrocket.com/common-next-js-errors/
- https://sentry.io/answers/next-js-middleware-module-not-found-can-t-resolve-fs/
- https://www.npmjs.com/package/fs-extra
Featured Images: pexels.com