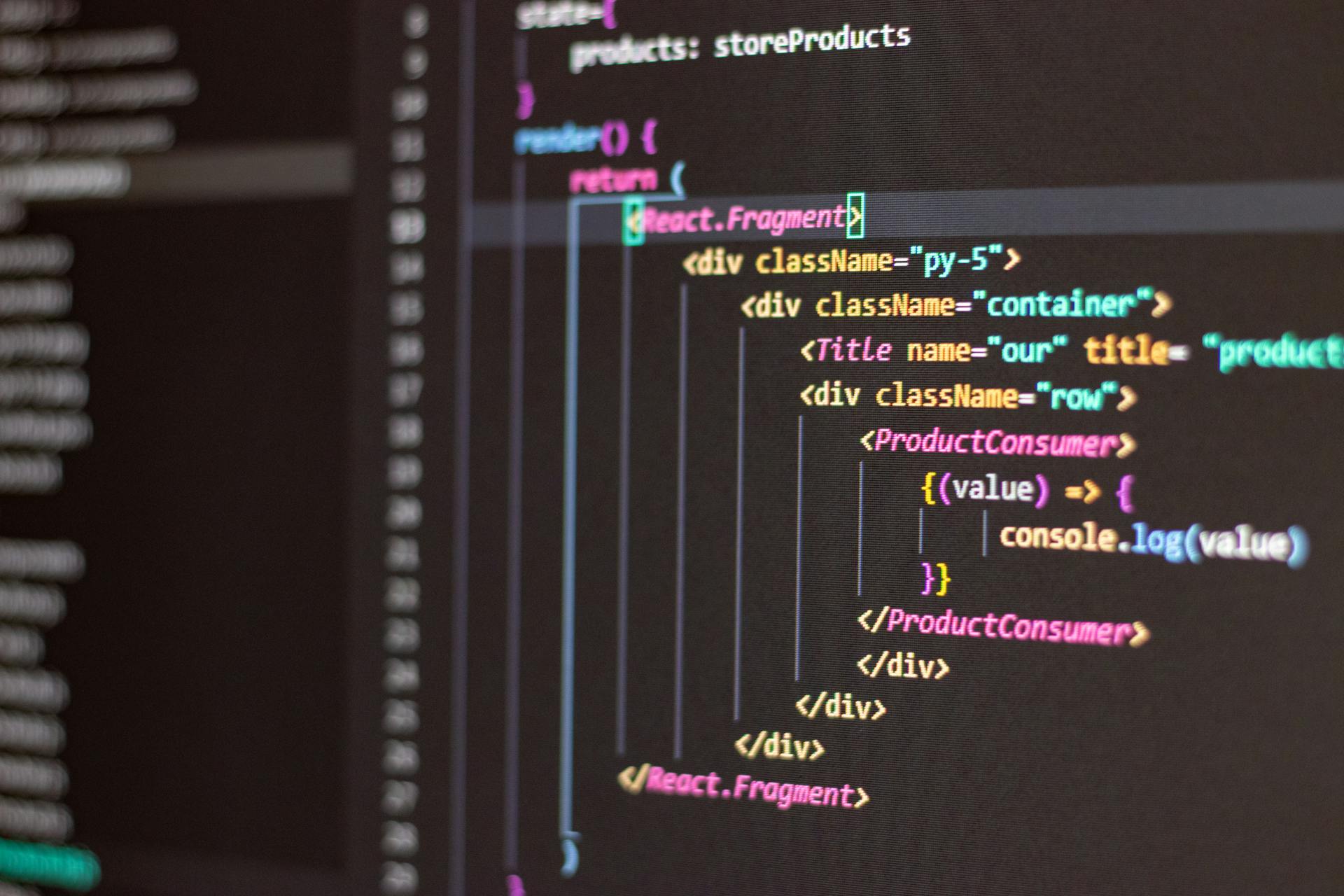
Next Js Open Graph Setup and Optimization is a crucial step in getting your website's content shared seamlessly across social media platforms. The Open Graph protocol allows you to control how your website's content is displayed when shared.
To start, you'll need to add the necessary meta tags to your Next Js pages. This includes the og:title, og:description, and og:image tags, which will be used to display your content's title, description, and image on social media platforms.
A well-configured og:image tag is essential, as it determines the image that will be displayed when your content is shared. For example, if you're using Next Js, you can use the Image component from the Next Js library to optimize your images and ensure they meet the required dimensions.
By following these steps, you can ensure that your website's content is displayed correctly on social media platforms and drives more engagement.
Setting Up Open Graph
Open Graph support is a must-have for any website that wants to be shared on social media. The Open Graph protocol controls what content is displayed when sharing links on social media platforms.
To get started, you need to use Open Graph tags in your web pages. This will enable them to become rich objects in a social graph. Platforms like Twitter, LinkedIn, and Facebook recognize Open Graph tags.
Next SEO supports the following OG properties: Audio, Video, Article, Profile, and Book. This means you can customize the content displayed on social media platforms for each type of content.
If you share a link without OG tags, social media platforms will choose a random title, image, and description. To avoid this, make sure to include OG tags in your web pages.
Here are the OG properties supported by Next SEO:
- Audio
- Video
- Article
- Profile
- Book
To implement dynamic Open Graph in Next.js, you can use a code snippet that generates dynamic Open Graph images based on a post title. This will ensure that your social media posts look great and are consistent with your brand.
A unique perspective: Nextjs Dynamic
Generating OG Images
Generating OG images is a crucial step in making your Next.js app social media-friendly. You'll want to create a separate app or add the functionality to your existing app to generate the images and display them.
A different take: Best Seo Practices for Images on Wordpress
To make the image dynamic, you can use URLSearchParams to get the values from the URL query. This will allow you to style your image like you want, as long as it has the right dimensions. Most sites recommend a width of 1200 and a height of 630.
You can generate the OG image by using a function that checks if the image already exists, and if not, it opens the browser with the correct query parameters, takes a screenshot of the page, and stores the screenshot where Next.js can use it.
The function can be called inside the getStaticProps function of a page to generate the OG image. This is what my getStaticProps in the [slug].js file inside the pages folder looks like:
You can also use the @vercel/og library to generate OG images. This library uses satori to create an image from HTML and CSS code. With @vercel/og, you can generate an image from React code using the ImageResponse type.
However, keep in mind that there are some constraints when using @vercel/og, such as not being able to use script or link elements, and only being able to use flex layout. But you can still do a lot with it, and it's a great way to generate OG images efficiently.
Recommended read: Next Js Performance Analyzer Library Npm
Here are some key differences between using serverless functions and Edge functions:
To generate an OG image with @vercel/og, you can create a new /pages/api/og.tsx file and add the dependency to your project with yarn add @vercel/og. Then, you can generate a basic image containing the text “Hello World!”.
You can also use TailwindCSS classes natively with @vercel/og by using the tw prop on elements. And you can use the classic style prop to specify CSS properties.
To add the OG image to your pages, you can use a custom component, such as SeoHeaders, to define the meta tags. You can also pass the URL of the OG image to the SeoHeaders component.
Note that you need to specify the other tags, such as og:type and twitter:card, for the OG image to be used in social media.
Expand your knowledge: How to Add Sitemap to Django Project
Implementing Open Graph
Open Graph support is crucial for sharing links on social media, and Next.js makes it easy to implement. The Open Graph protocol controls what content is displayed when sharing links on social media platforms.
Using Open Graph tags in web pages enables them to become rich objects in a social graph. If you share without OG tags, social media platforms will choose a random title, image, and description.
Next SEO supports the following OG properties: Audio, Video, Article, Profile, and Book.
To implement dynamic Open Graph, you can use Next.js. Here's how to generate dynamic Open Graph images based on a post title: Implement the Dynamic Open Graph RouteImplement the Dynamic Open Graph Meta TagsGenerate the OG images during the build
You can use a serverless function to generate OG images. Let's create a new /pages/api/og.tsx file and update this function to return an image using @vercel/og.
The constraints of using JSX in @vercel/og are: Not all CSS properties are supportedOnly flex layout is supported, and each element with multiple children must explicitely use (display: flex)You can’t use script or link elements, etc.
You can use TailwindCSS classes natively, using the tw prop on elements (instead of className). If you prefer, you can use the classic style prop to specify CSS properties.
To add OG image to your pages, you can use a custom component or add the meta tags by yourself. You can build the OG image URL:
Then, you can pass this URL to the SeoHeaders component:
Or, if you add the meta tags by yourself:
You might enjoy: Next Js Pages
Advanced Open Graph Topics
Open Graph support is a crucial aspect of making your Next.js website shareable on social media.
The Open Graph protocol controls what content is displayed when sharing links on social media, and using Open Graph tags in web pages enables them to become rich objects in a social graph.
Platforms like Twitter, LinkedIn, and Facebook recognize Open Graph tags, but Twitter also has meta tags called Twitter Cards, which it will use when there's no need to use Open Graph tags.
Next SEO supports the following Open Graph properties: AudioVideoArticleProfileBook
If you don't use Open Graph tags, social media platforms will choose a random title, image, and description when sharing your links.
Recommended read: How to Use Loading in Next Js
Implementing Open Graph with Next.js
Implementing Open Graph with Next.js is a straightforward process. Next SEO supports the Open Graph protocol, which allows you to control what content is displayed when sharing links on social media platforms.
To get started, you'll need to add the necessary Open Graph tags to your web pages. You can use the following OG properties: Audio, Video, Article, Profile, and Book. These properties will help social media platforms understand the content of your page.
Here's a list of the supported OG properties:
- Audio
- Video
- Article
- Profile
- Book
By implementing Open Graph with Next.js, you can ensure that your content is displayed correctly on social media platforms, increasing engagement and driving more traffic to your site.
Audio Example
Implementing Open Graph with Next.js can be a bit tricky, but don't worry, I've got you covered.
To enable audio Open Graph support with multiple audio files, you can use a specific configuration. This configuration allows you to add multiple audio files to your Open Graph metadata.
You can take a look at other examples for other OG types to learn more.
Vercel and Edge Functions
Vercel's @vercel/og library makes it easy to generate OG images from HTML and CSS code using JSX.
This library relies on satori, another Vercel package that can generate images efficiently on the client or in serverless functions.
Edge functions are a special kind of serverless function that are free from classic Node.js runtime features.
You can only use basic JavaScript features from the V8 runtime in Edge functions, but they are extremely fast and cheaper to execute.
To use Edge functions in Next.js, you need to export a config object and return a response of type NextResponse.
In Edge functions, you use req.url to get query parameters, and parse it using the URL class.
Here are the key differences between serverless functions in Next.js and Edge functions:
- The exported config object is required.
- req parameter has type NextRequest instead of NextApiRequest.
- You return a response of type NextResponse.
Sources
- https://phiilu.com/generate-open-graph-images-for-your-static-next-js-site
- https://scastiel.dev/create-og-images-for-your-blog-with-nextjs
- https://cruip.com/generate-dynamic-open-graph-and-twitter-images-in-next-js/
- https://blog.logrocket.com/manage-seo-next-js-with-next-seo/
- https://wiscaksono.com/articles/generate-dynamic-open-graph-with-nextjs
Featured Images: pexels.com