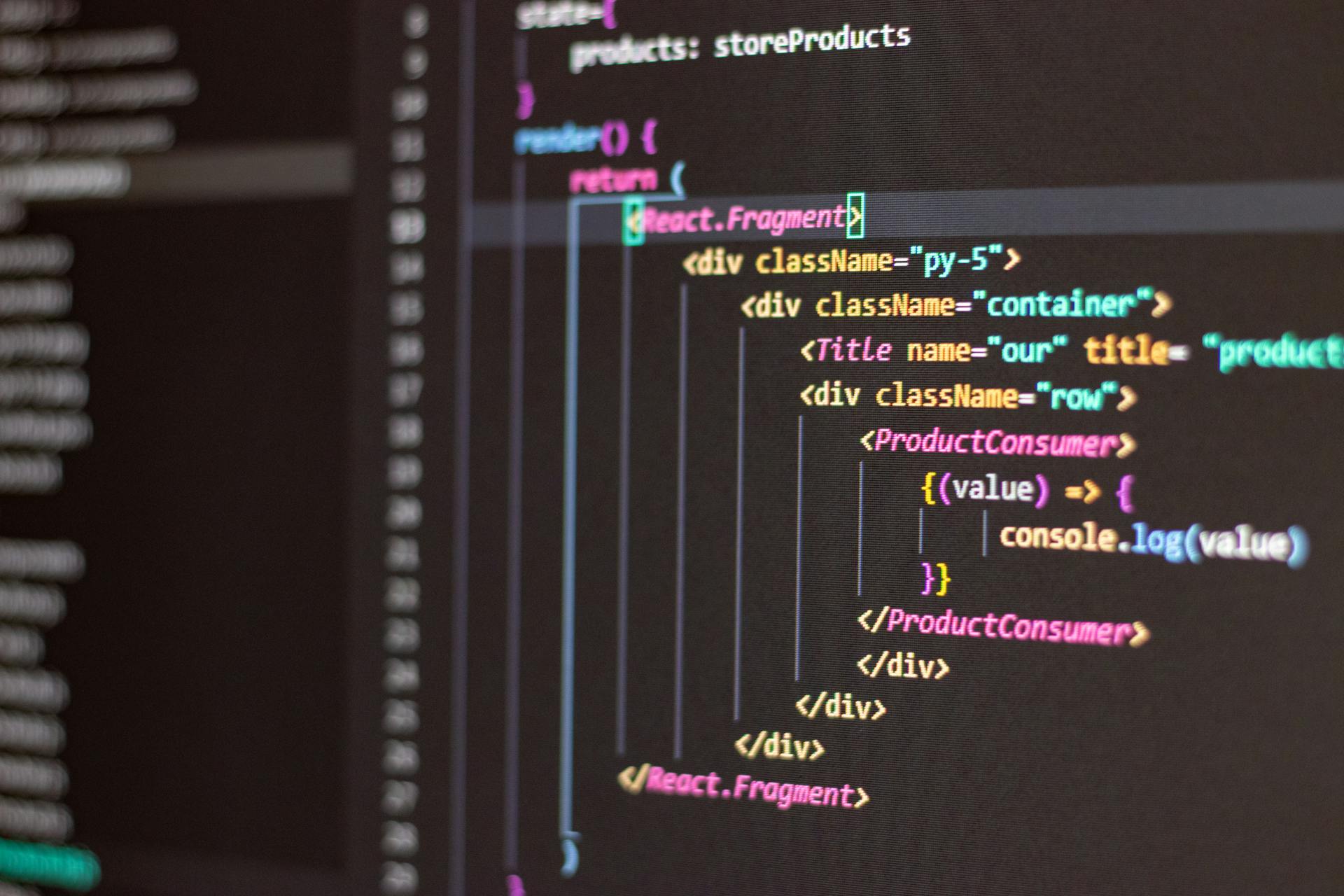
Real-time web development is a game-changer, and Next Js Socket Io is a powerful tool to achieve it.
By using Socket Io with Next Js, you can create real-time web applications that update automatically without requiring a full page reload.
This means a better user experience and reduced latency.
With Socket Io and Next Js, you can also handle a large number of concurrent connections, making it perfect for real-time applications.
Real-time updates can be achieved through the use of WebSockets, which establish a persistent connection between the client and server.
This allows for bi-directional communication, enabling the server to push updates to the client in real-time.
Socket Io simplifies the process of setting up WebSockets, making it easier to implement real-time functionality in your Next Js application.
Real-Time Communication
Real-time communication is a crucial aspect of Next.js applications, and Socket.io makes it easy to achieve. You can send and receive messages between clients and the server using custom events and handlers.
To send a message from the client to the server and vice versa, you can define custom events and handlers. For example, you can emit a "chatMessage" event from the client to the server and listen for a "serverMessage" event from the server to the client.
Here are some real-world use cases where real-time communication can greatly enhance user experiences:
- Live updates: Display live updates to users, such as scores, stock prices, or news.
- Chat applications: Create real-time chat applications where users can send and receive messages instantly.
- Collaborative tools: Develop collaborative tools, such as text editors or whiteboards, where multiple users can work together in real-time.
Sending Messages
Sending messages is a crucial aspect of real-time communication. To send messages between clients and the server, you can use Socket.io, which establishes a socket connection.
You can emit messages to the server using the `socket.emit()` function, specifying a unique event name and the message itself. For example, in the `onChangeHandler`, you can set the input state to a new value and emit this change to the server as an event, like this: `socket.emit('input-change', this.state.inputValue)`.
The server must handle this event by listening for the specific `input-change` event. This can be done by registering a connection listener and subscribing to the `input-change` event within the callback function.
To send messages to all connected clients except the one that emitted the `input-change` event, you can use `socket.broadcast.emit()`. This will send the message to all other clients, allowing them to receive the updated input value in real-time.
Here are some key methods for sending messages:
- `socket.emit()`: Emits a message to the server
- `socket.broadcast.emit()`: Sends a message to all connected clients except the one that emitted the event
- `socket.on()`: Subscribes to an event and executes a callback function when the event is received
By using these methods, you can establish real-time communication between clients and the server, enabling features like collaborative editing, live updates, and more.
Real-World Use Cases
Real-time communication can greatly enhance user experiences in various scenarios. Real-world use cases demonstrate the power of this technology.
Live updates are essential for sports fans, and WebSockets can provide them with instant scores and game stats. This can be particularly useful during high-stakes games where every second counts.
Real-time communication can also be beneficial for online shopping, allowing customers to see the status of their orders in real-time. This can help build trust and increase customer satisfaction.
Instant messaging and live chat features can also be powered by WebSockets, enabling users to engage with businesses and each other in real-time. This can be particularly useful for customer support and live events.
Real-time communication can also enhance the user experience in online gaming, allowing players to interact with each other in real-time. This can create a more immersive and engaging experience for players.
Client Setup
To set up a client for real-time communication in a Next.js application, you'll need to create a component that establishes a socket connection to the server. This component will be responsible for emitting changes to the server, which will then broadcast the updates to all connected clients.
In Next.js, you can create a RealtimeView component in the components/RealtimeView.tsx file. This component will import the necessary modules, including useEffect and useState, to manage side effects and state.
The RealtimeView component will use the socket object to establish a connection to the server and listen for the "count" event. When the component mounts, it will establish a connection to the socket server and update the component state with the count received from the server.
To create the RealtimeView component, you'll need to import the necessary modules, including socket.io-client, and create a socket object using io from. The RealtimeView component will also use the state hook (useState) to manage the view's state, representing the count of connected users.
Here's a summary of the necessary imports and setup for the RealtimeView component:
- Import socket.io-client
- Create a socket object using io from
- Use the state hook (useState) to manage the view's state
- Establish a connection to the socket server
- Listen for the "count" event and update the component state with the count received from the server
By following these steps, you'll be able to create a RealtimeView component that establishes a socket connection to the server and updates the component state in real-time.
Session Management
Session management is crucial in a real-time collaboration application like our Next.js Socket.IO project.
To manage sessions, we need to keep track of which users are currently connected and emit events to all clients when a user connects or disconnects. We can do this by adding code to our session backend that listens for new connections and emits events accordingly.
The session backend will be our source of truth for the document state, so let's start by adding some code to it. In our session-backend/index.ts file, we're already importing socket.io and starting a WebSocket server on port 8080. Now, we need to add some code to keep track of connected users and emit events when they connect or disconnect.
To connect to the session backend, we'll use the @jamsocket/server helper functions to spawn a new backend when someone opens the whiteboard. We'll also update our client-side logic to connect to the session backend and listen for user-entered and user-exited WebSocket events.
Here's a summary of the steps we need to take:
- Spawn a new session backend using the @jamsocket/server helper functions
- Update our client-side logic to connect to the session backend and listen for user-entered and user-exited events
- Keep track of connected users and emit events when they connect or disconnect
By following these steps, we'll be able to implement a robust session management system that allows multiple users to collaborate in real-time.
Presence and State
You can track the cursor position for each user by subscribing to a cursor-position event and updating your list of users with the user passed to it. This allows you to display the cursor position on top of the whiteboard.
To implement this, you'll need to send a cursor-position event to the session backend as the cursor moves over the whiteboard. This can be done using the useSend hook to create a sendEvent function.
The session backend code should subscribe to the cursor-position event and emit a cursor-position event to all connected clients. You can use volatile.broadcast here because it's okay if you drop a couple cursor-position events.
Moving your cursor over one canvas should show a moving cursor on the other client, but the shapes you create in one window don't appear in the other. To fix this, you'll need to implement state sharing across clients.
State sharing allows you to share shapes across clients, so when one user draws a shape, it appears on all connected clients. To implement state sharing, you'll need to create an array to store all the shapes on the session backend.
When a new user connects, you'll need to send them a snapshot of all the shapes. You'll also need to listen for two new events: create-shape and update-shape, which will update your list of shapes accordingly.
Here's a summary of the events you'll need to implement for state sharing:
- snapshot: sends a snapshot of all shapes to new users
- create-shape: updates the list of shapes when a new shape is created
- update-shape: updates the list of shapes when an existing shape is updated
Backend Setup
The backend setup is a crucial part of building a real-time application with Next.js and Socket.IO. We start by adding presence to our application, which involves keeping track of which users are currently connected and emitting events to all clients when a user connects or disconnects.
To do this, we add code to the session backend in `src/session-backend/index.ts` to keep track of connected users and emit events to clients.
We then need to update our client-side logic to connect to the session backend and listen for user-entered and user-exited WebSocket events.
Here are the steps to update our client-side logic:
- Connect to the session backend using the `socket.io-client` library.
- Listen for the "count" event to update the component state with the count of connected users.
To deploy our session backend code to Jamsocket, we need to build and push our session backend to Jamsocket. This involves creating an API token on the Jamsocket settings page and updating the `Jamsocket` constructor in `src/app/page.tsx` to pass in the account, service, and token.
Here are the steps to deploy to Jamsocket:
- Create an API token on the Jamsocket settings page.
- Update the `Jamsocket` constructor in `src/app/page.tsx` to pass in the account, service, and token.
By following these steps, we can set up our backend to handle real-time communication between clients using Socket.IO.
Testing and Deployment
Testing your Next.js application with WebSocket support is a breeze. Simply start your server by running `node server.js` and navigate to `http://localhost:3000` in multiple browser tabs or windows.
If your WebSocket connection is set up correctly, actions in one tab should be reflected in real-time across other tabs. You can use WebSocket testing tools like Postman or WebSocket King to verify the real-time capabilities of your server.
To deploy your session backend code to Jamsocket, you'll need to create an API token on the Jamsocket settings page.
Here are the steps to deploy your session backend code to Jamsocket:
- Build and push your session backend to Jamsocket.
- Create an API token on the Jamsocket settings page.
- Change the new Jamsocket() call in `src/app/page.tsx` by passing in account, service, and token.
Now, when you run your NextJS app locally, it'll spawn your session backend on Jamsocket. You can see which session backends have been spawned by visiting your new whiteboard-demo service in the Jamsocket Dashboard or by running.
Choosing and Using
To use a WebSocket server in Next.js, you need to set up an API route in the pages/api directory that initializes the Socket.io connection. This is similar to the socket.js file discussed earlier.
You can then connect to the WebSocket server using the Socket.io client in your Next.js pages. For example, in pages/index.js, you can use the io() function from 'socket.io-client' to establish a connection.
Here are some compelling reasons to use WebSockets in Next.js:
- Real-Time Updates: Keep your users informed with instant updates to content or notifications.
- Live Chat: Create engaging chat applications with minimal latency.
- Interactive Gaming: Enable multiplayer gaming experiences with real-time data synchronization.
- Collaborative Tools: Build collaborative applications where multiple users can interact simultaneously.
To choose between Socket.io and the WebSocket API, consider your specific requirements and the advantages of each method.
Choosing Between API
Choosing the right API for your project depends on your specific requirements.
Both Socket.io and the WebSocket API have their advantages and use cases.
For real-time applications, Socket.io is often preferred due to its ability to handle connection loss and reconnection.
The WebSocket API, on the other hand, is a more lightweight option that's well-suited for applications with a large number of connections.
Why Use?
Using WebSockets in Next.js can greatly enhance the real-time capabilities of your applications. You can keep your users informed with instant updates to content or notifications, which is especially useful for applications that require up-to-date information.
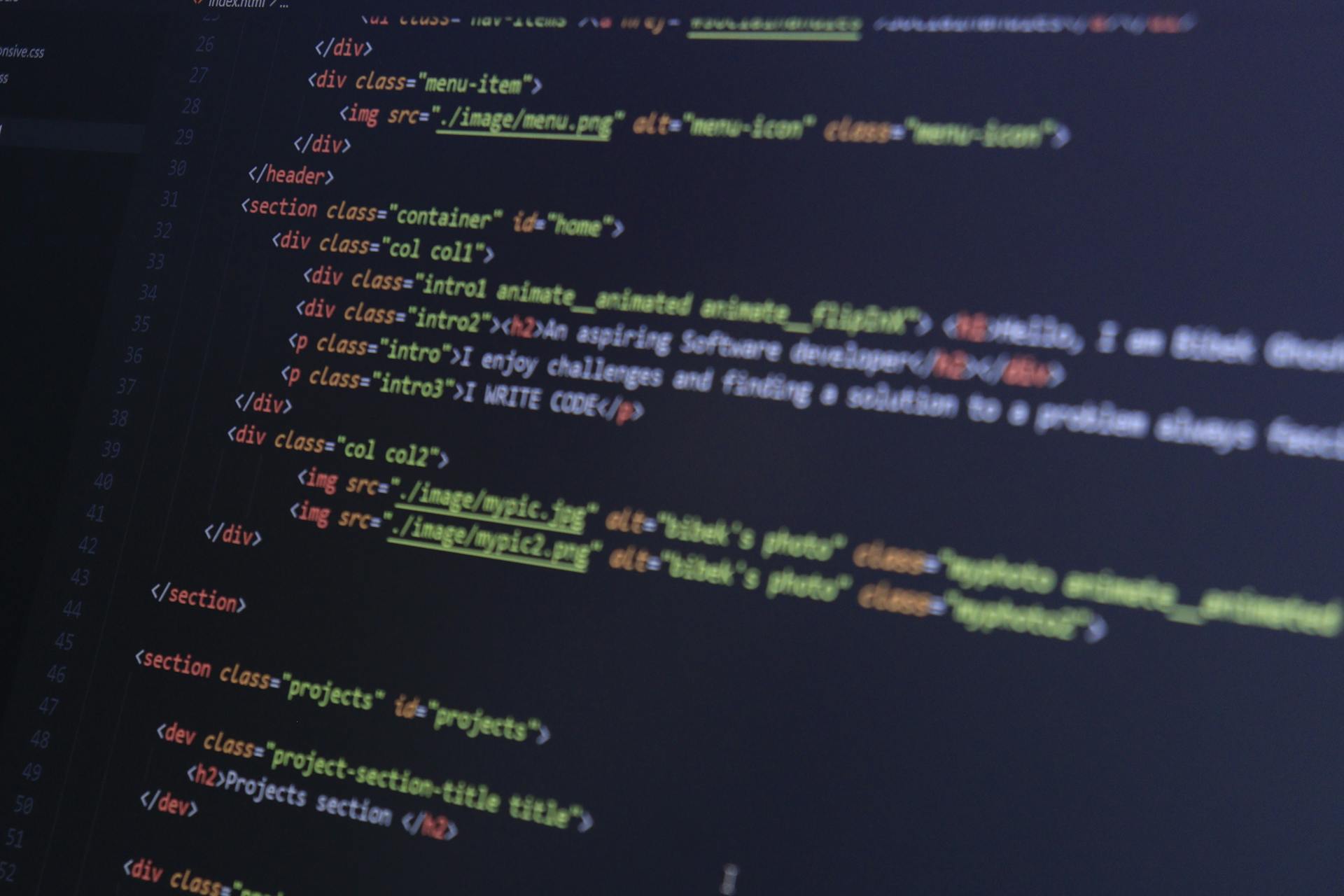
One of the most compelling reasons to use WebSockets is for real-time updates. This can be achieved through instant updates to content or notifications, making it perfect for applications that require users to stay informed.
Live chat applications can be created with minimal latency, allowing for more engaging user experiences. This is especially useful for applications that require users to interact with each other in real-time.
Interactive gaming experiences can be enabled with real-time data synchronization, making it possible to build multiplayer gaming experiences. Collaborative tools can also be built where multiple users can interact simultaneously, making it perfect for applications that require teamwork.
Here are some examples of how WebSockets can be used in Next.js:
- Real-Time Updates: Keep your users informed with instant updates to content or notifications.
- Live Chat: Create engaging chat applications with minimal latency.
- Interactive Gaming: Enable multiplayer gaming experiences with real-time data synchronization.
- Collaborative Tools: Build collaborative applications where multiple users can interact simultaneously.
Sources
- https://codedamn.com/news/nextjs/how-to-use-socket-io
- https://blog.logrocket.com/implementing-websocket-communication-next-js/
- https://docs.jamsocket.com/tutorials/nextjs-socketio
- https://clouddevs.com/next/socketio-and-websocket-api/
- https://codewithmarish.com/post/realtime-views-component-nextjs-socketio
Featured Images: pexels.com