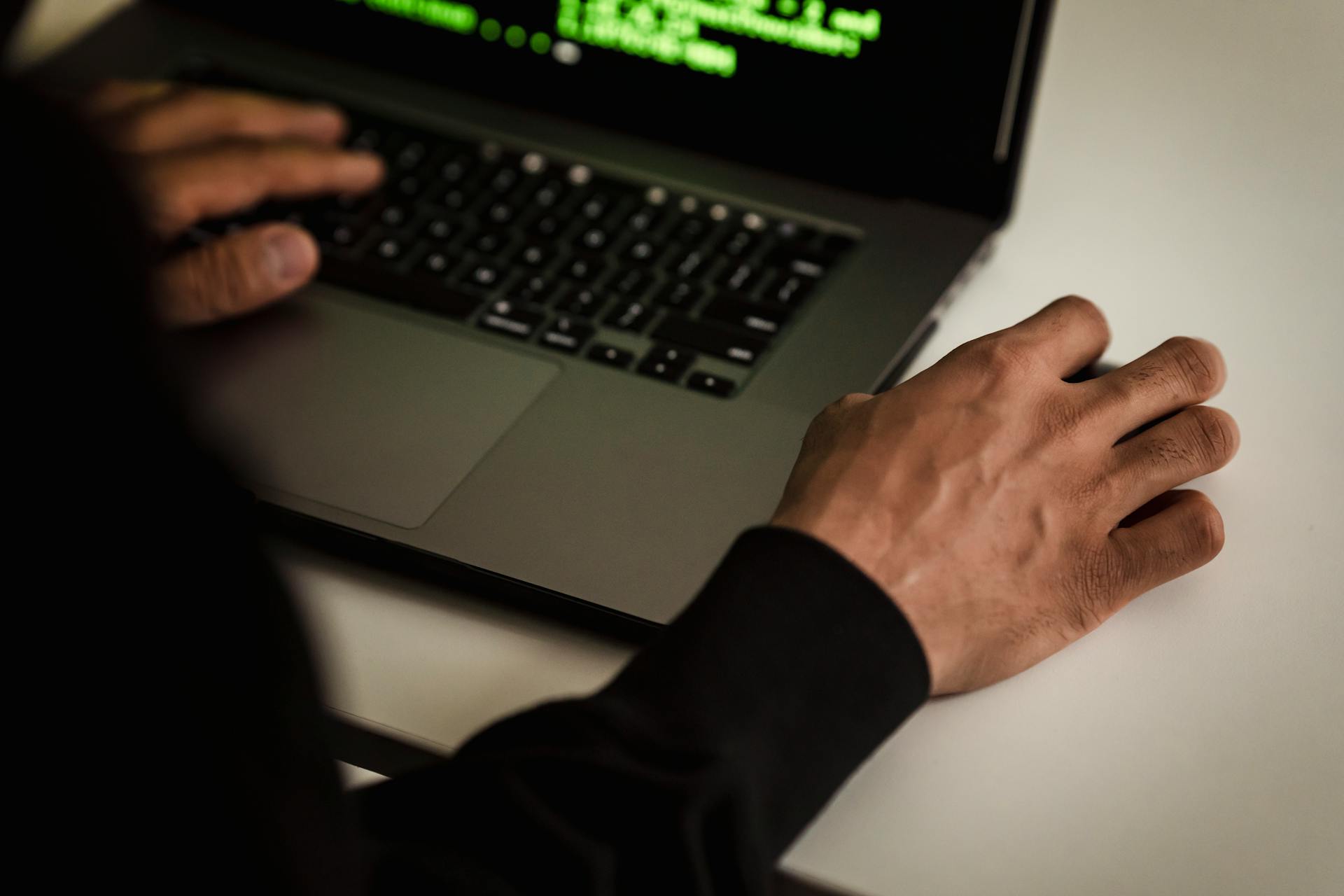
Next.js Proxy is a powerful tool that allows you to optimize your API by setting up a reverse proxy server. This server acts as an intermediary between your client-side application and your server, enabling features like caching, authentication, and rate limiting.
By using Next.js Proxy, you can improve the performance and security of your API, making it more efficient and scalable. Next.js Proxy provides a simple and intuitive way to manage API requests and responses.
One of the key benefits of Next.js Proxy is its ability to cache frequently accessed data, reducing the load on your server and improving response times. This is especially useful for APIs that handle a high volume of requests.
With Next.js Proxy, you can also implement authentication and rate limiting to protect your API from unauthorized access and abuse. This helps to ensure the security and reliability of your API.
For another approach, see: Nextjs Proxy Api Route
What is Next.js Proxy
In Next.js, a proxy is a crucial component that helps manage proxy requests, making it easier to integrate with multiple APIs.
Expand your knowledge: Azure Proxy
Next.js applications often need to interact with multiple APIs, and http-proxy-middleware plays a key role in routing API requests to the appropriate backend services without exposing complexity to the client.
By using http-proxy-middleware, you can easily route API requests, making it a seamless integration.
This middleware hides the details of your backend servers from the client, adding a layer of security by ensuring that clients interact with the proxy, not the backend servers directly.
http-proxy-middleware allows for sophisticated request routing and modification, making it easier to integrate with different APIs and services.
Some of the benefits of using http-proxy-middleware include:
To use http-proxy-middleware in Next.js, you need to import it in your next.config.js file and configure it to use the proxy middleware.
By setting up http-proxy-middleware, you can forward requests to a specific URL, such as https://example.com, using a Next.js API route.
Take a look at this: Why Use Next Js
Benefits and Importance
Using a proxy in Next.js can simplify API requests management, making it easier to handle complex request routing.
By acting as a reverse proxy, it hides your backend server details from the client, adding an extra layer of security. This is especially important for Next.js applications that need to interact with multiple APIs.
Proxies can cache responses and handle SSL termination, improving the overall performance of your Next.js application.
Here are some key benefits of using a proxy in Next.js:
- Simplified API Requests Management: A Next.js proxy helps manage proxy requests to different backend servers or APIs.
- Enhanced Security: By acting as a reverse proxy, it hides your backend server details from the client.
- Improved Performance: Proxies can cache responses and handle SSL termination.
- Flexibility in API Path Management: With HTTP proxy middleware, you can easily rewrite API path and incoming request path.
- Environment-specific Configurations: Proxies can be configured differently for development and production environments.
Next.js applications often need to interact with multiple APIs, and using http-proxy-middleware makes this process seamless.
Configuring and Using
To configure and use a Next.js proxy, you'll need to install the necessary packages, including `http-proxy-middleware` and `next-http-proxy-middleware`. Create a new file named `middleware.js` in the root of your project, where you'll configure the proxy settings.
In this file, import the required modules and set up the proxy middleware. You can then import the middleware in your `next.config.js` file and configure it to use the proxy. To create a proxy, define a middleware function that uses `http-proxy-middleware`, specifying the target server and any necessary options like path rewriting.
Here's a step-by-step guide to configuring and using a Next.js proxy:
1. Install the necessary packages: `http-proxy-middleware` and `next-http-proxy-middleware`.
2. Create a new file named `middleware.js` and configure the proxy settings.
3. Import the middleware in your `next.config.js` file and configure it to use the proxy.
4. Define a middleware function that uses `http-proxy-middleware` and specifies the target server and any necessary options.
5. Integrate the proxy with your Next.js API routes.
By following these steps, you can create a Next.js proxy that simplifies API requests management, enhances security, improves performance, and provides flexibility in API path management.
Expand your knowledge: Next Js Api
Using External Resolver and Bindable Events
You can use an external resolver to resolve the target dynamically based on the request. This is useful when the target server might change depending on the request parameters.
An external resolver can be used to dynamically resolve the target server for each request. This is a powerful feature that allows for more flexibility in your proxy configurations.
Check this out: Nextjs Redirect from Server Component
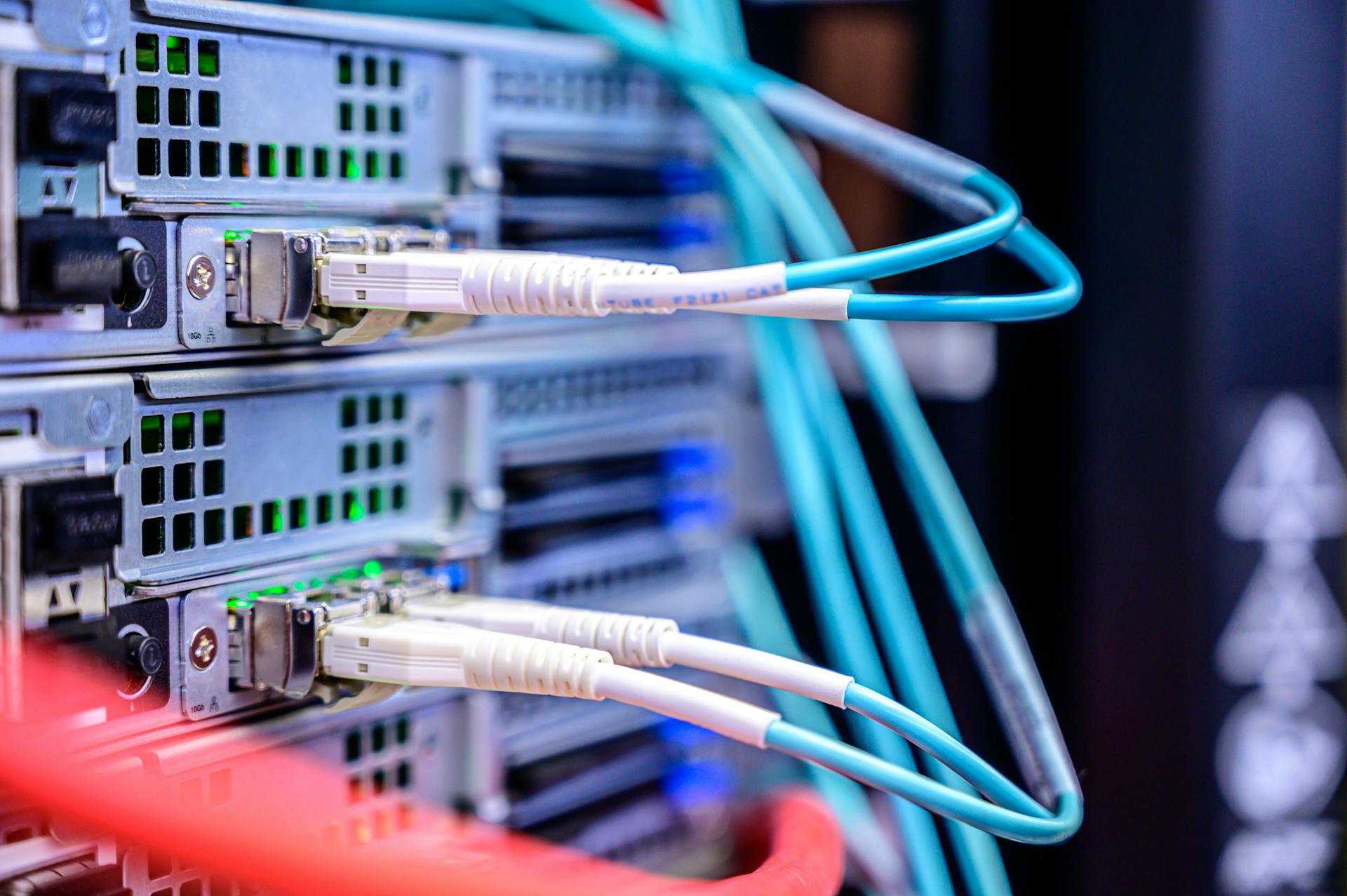
Here are some examples of bindable events that you can use with http-proxy-middleware:
- proxyReq: This event is triggered before the proxy sends the request to the target server.
- proxyRes: This event is triggered after the proxy receives the response from the target server.
- error: This event is triggered when an error occurs during the proxying process.
Bindable events allow you to hook into the proxy lifecycle and perform custom actions at different stages of the proxying process. This can be useful for logging, modifying the proxy instance, or performing other custom tasks.
Configuring Http Middleware
To configure http-proxy-middleware in your Next.js project, you need to install the necessary packages. This includes installing http-proxy-middleware and next-http-proxy-middleware.
You'll also need to create a new file named middleware.js, where you'll configure the proxy settings. This file should be placed in the root of your project.
To set up the proxy settings, you'll import the required modules and use the createProxyMiddleware function to create a proxy instance. This instance will be used to forward requests to a target server.
You can configure the proxy settings to handle different paths or fallback scenarios using conditional logic. This allows you to handle complex request routing and customize how requests are handled.
Here's a step-by-step guide to configuring http-proxy-middleware:
1. Install the necessary packages: http-proxy-middleware and next-http-proxy-middleware
2. Create a new file named middleware.js in the root of your project
3. Import the required modules and use createProxyMiddleware to create a proxy instance
4. Configure the proxy settings to handle different paths or fallback scenarios using conditional logic
By following these steps, you'll be able to configure http-proxy-middleware in your Next.js project and start using it to manage proxy requests.
Creating and Handling
Creating a proxy in Next.js involves setting up middleware that intercepts requests from the client and forwards them to the target server. This allows you to handle API requests seamlessly without exposing your backend servers directly to the client.
To create a proxy, you need to define a middleware function that uses http-proxy-middleware. This function will configure the proxy settings, including the target server and any necessary options like path rewriting or changing the origin of the request.
You can set up a new file for the proxy configuration by creating a new file named proxy.js in the root of your project. In this file, use const proxy to define your proxy settings and create an http proxy instance.
Here's a step-by-step guide to integrating the proxy with your Next.js API routes:
- Create a new API route in your Next.js project that uses the proxy middleware.
- Use the createProxyMiddleware function to create an HTTP proxy instance with the target server set to https://example.com.
- Use the pathRewrite option to remove the /api prefix from the incoming request path.
Handling unresolved requests is crucial to maintain the robustness of your application. You can set up a fallback route in case the proxy fails to resolve a request. This ensures that the application can still respond appropriately even when the target server is unreachable.
Explanation of Reverse
Creating and Handling a reverse proxy is a crucial step in managing your web servers. A reverse proxy is a type of proxy server that forwards client requests to one or more backend servers.
It's particularly useful for load balancing, which means distributing incoming traffic among numerous servers to avoid overloading a single server. This is especially important when you have a high volume of traffic and don't want to overwhelm your servers.
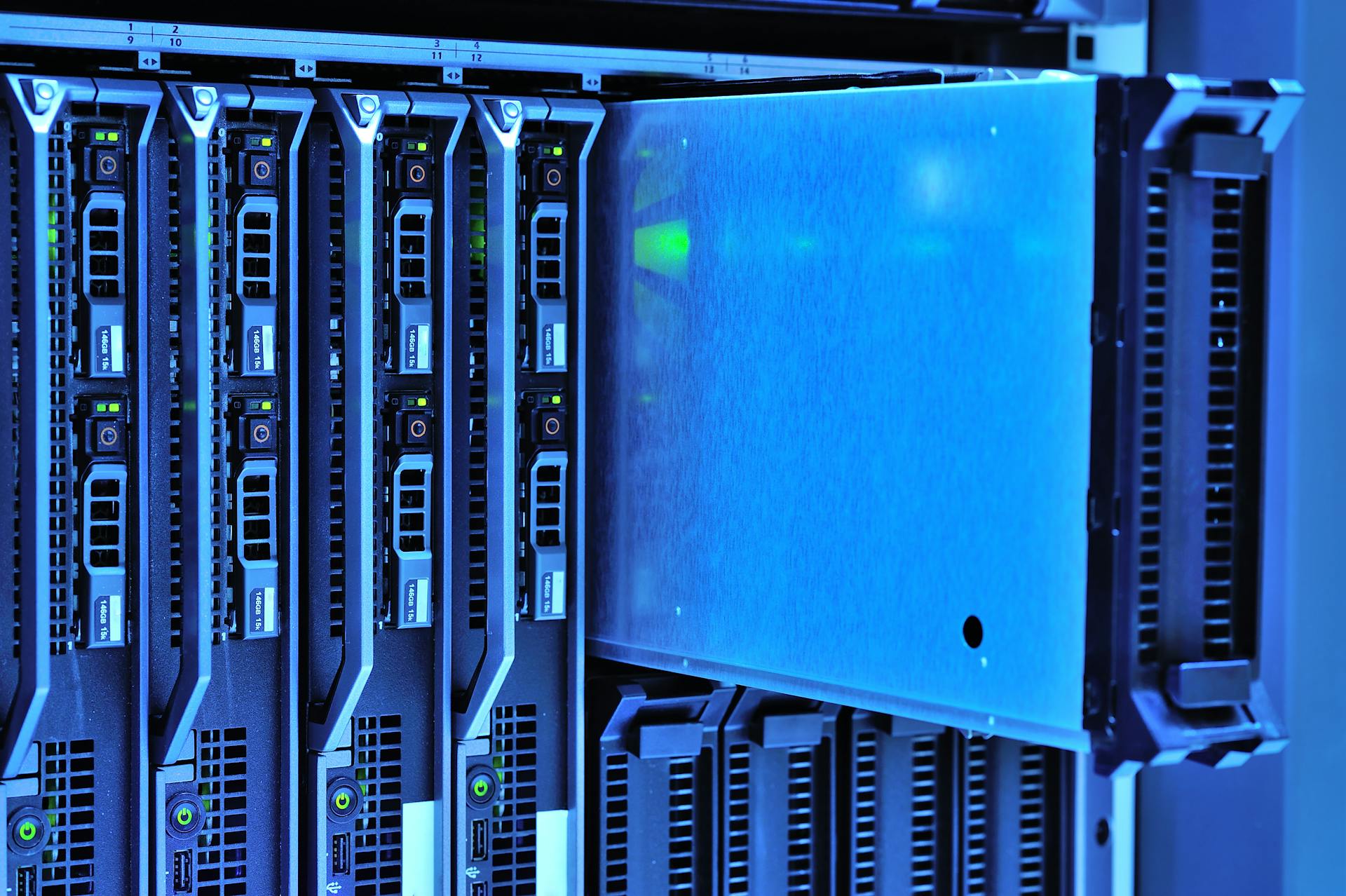
A reverse proxy also provides security by hiding the backend servers' details from the client, adding an extra layer of protection against potential threats. By hiding the backend servers, you're making it more difficult for hackers to target them.
One of the key benefits of using a reverse proxy is caching, which involves storing copies of responses to reduce load on backend servers and speed up response times. This can be a game-changer for websites that receive a lot of repeat traffic.
To give you a better idea of how a reverse proxy works, here are some of its key features:
- Load Balancing: Distributes incoming traffic among numerous servers.
- Security: Hides the backend servers' details from the client.
- Caching: Stores copies of responses to reduce load on backend servers.
- SSL Termination: Handles SSL encryption/decryption to offload this task from the backend servers.
Creating a
Creating a proxy in Next.js is a straightforward process that involves setting up middleware to intercept requests from the client and forward them to the target server. You can use http-proxy-middleware to configure and manage these proxies effectively.
To create a proxy, you'll need to define a middleware function that uses http-proxy-middleware. This function will configure the proxy settings, including the target server and any necessary options like path rewriting or changing the origin of the request.
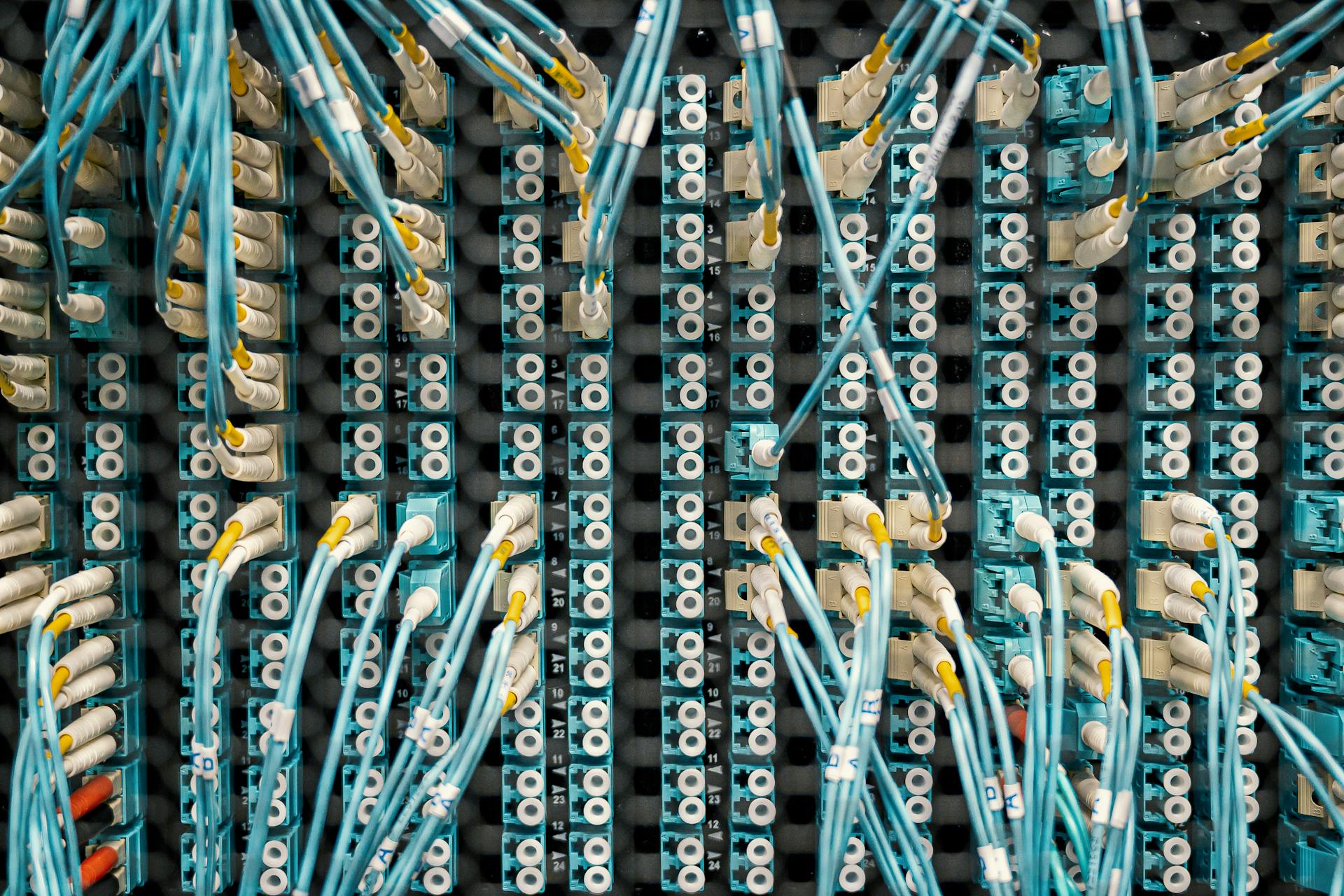
Here's a step-by-step guide to creating a proxy:
1. Set up a new file for the proxy configuration: Create a new file named proxy.js in the root of your project.
2. Import http-proxy-middleware and create the proxy: In proxy.js, use const proxy to define your proxy settings and create an http proxy instance.
Integrating the proxy with your Next.js API routes is the next step. You can create a new API route in your Next.js project that uses the proxy middleware.
Here's an example of how to use const proxy to define the proxy middleware configuration:
- The createProxyMiddleware function is used to create an HTTP proxy instance with the target server set to https://example.com.
- The pathRewrite option is used to remove the /api prefix from the incoming request path, ensuring the request URL matches the target server’s expected format.
By following these steps, you can create a proxy that seamlessly handles API requests and ensures secure and efficient communication with external services.
Frequently Asked Questions
What is proxy in Nodejs?
A proxy in Node.js is an intermediary that forwards client requests to servers and handles responses, enabling various uses in the ecosystem. This intermediary layer can be leveraged for a range of purposes, from caching to security.
Sources
- https://www.dhiwise.com/post/nextjs-proxy-simplifying-api-requests-and-routing
- https://kinsta.com/blog/err_too_many_redirects/
- https://nextjs.org/docs/pages/building-your-application/routing/middleware
- https://nextjs.org/docs/pages/api-reference/next-config-js/headers
- https://nextjs.org/docs/pages/api-reference/next-config-js/redirects
Featured Images: pexels.com